pandas.Index.is_floating メソッドのサンプルコード
Pandas の Index オブジェクトにおける pandas.Index.is_floating メソッド
概要
- メソッド名:
is_floating
- 引数: なし
- 戻り値:
- True: Index オブジェクトが浮動小数点数型の場合
- False: Index オブジェクトが浮動小数点数型ではない場合
使用例
import pandas as pd
# 浮動小数点型の Index オブジェクトを作成
index = pd.Index([1.2, 3.4, 5.6])
# `is_floating` メソッドを使用して、Index オブジェクトが浮動小数点数型かどうかを確認
print(index.is_floating())
# 出力: True
詳細解説
pandas.Index.is_floating
メソッドは、Index
オブジェクトの dtype 属性をチェックすることで、Index オブジェクトが浮動小数点数型かどうかを判断します。- dtype 属性は、Index オブジェクトのデータ型を表す属性です。
- 浮動小数点数型のデータ型には、
float32
、float64
などがあります。
関連メソッド
pandas.Index.is_integer
:Index オブジェクトが整数型かどうかを判断するpandas.Index.is_object
:Index オブジェクトがオブジェクト型かどうかを判断するpandas.Index.dtype
:Index オブジェクトのデータ型を取得する
補足
pandas.Index.is_floating
メソッドは、Index オブジェクトの要素が全て浮動小数点数型かどうかを判断します。- Index オブジェクトの要素に欠損値 (NaN) が含まれている場合、
is_floating
メソッドはFalse
を返すことに注意が必要です。
例
import pandas as pd
# 欠損値を含む Index オブジェクトを作成
index = pd.Index([1.2, 3.4, np.nan])
# `is_floating` メソッドを使用して、Index オブジェクトが浮動小数点数型かどうかを確認
print(index.is_floating())
# 出力: False
Pandas の Index オブジェクトにおける pandas.Index.is_floating メソッドのサンプルコード
サンプルコード1: Index オブジェクトが浮動小数点数型かどうかを確認する
import pandas as pd
# 浮動小数点型の Index オブジェクトを作成
index = pd.Index([1.2, 3.4, 5.6])
# `is_floating` メソッドを使用して、Index オブジェクトが浮動小数点数型かどうかを確認
print(index.is_floating())
# 出力: True
サンプルコード2: 欠損値を含む Index オブジェクトが浮動小数点数型かどうかを確認する
import pandas as pd
import numpy as np
# 欠損値を含む Index オブジェクトを作成
index = pd.Index([1.2, 3.4, np.nan])
# `is_floating` メソッドを使用して、Index オブジェクトが浮動小数点数型かどうかを確認
print(index.is_floating())
# 出力: False
サンプルコード3: 文字列を含む Index オブジェクトが浮動小数点数型かどうかを確認する
import pandas as pd
# 文字列を含む Index オブジェクトを作成
index = pd.Index(["a", "b", "c"])
# `is_floating` メソッドを使用して、Index オブジェクトが浮動小数点数型かどうかを確認
print(index.is_floating())
# 出力: False
サンプルコード4: データフレームの Index オブジェクトが浮動小数点数型かどうかを確認する
import pandas as pd
# データフレームを作成
df = pd.DataFrame({"a": [1, 2, 3], "b": [4.5, 6.7, 8.9]})
# データフレームの Index オブジェクトを取得
index = df.index
# `is_floating` メソッドを使用して、Index オブジェクトが浮動小数点数型かどうかを確認
print(index.is_floating())
# 出力: False
サンプルコード5: Series の Index オブジェクトが浮動小数点数型かどうかを確認する
import pandas as pd
# Series を作成
series = pd.Series([1, 2, 3])
# Series の Index オブジェクトを取得
index = series.index
# `is_floating` メソッドを使用して、Index オブジェクトが浮動小数点数型かどうかを確認
print(index.is_floating())
# 出力: False
Pandas の Index オブジェクトが浮動小数点数型かどうかを確認する他の方法
dtype 属性を確認する
Index
オブジェクトの dtype
属性は、Index オブジェクトのデータ型を表します。
import pandas as pd
# 浮動小数点型の Index オブジェクトを作成
index = pd.Index([1.2, 3.4, 5.6])
# `dtype` 属性を使用して、Index オブジェクトのデータ型を確認
print(index.dtype)
# 出力: dtype('float64')
isinstance
関数は、オブジェクトが特定の型かどうかを判断するのに使用できます。
import pandas as pd
# 浮動小数点型の Index オブジェクトを作成
index = pd.Index([1.2, 3.4, 5.6])
# `isinstance` 関数を使用して、Index オブジェクトが浮動小数点数型かどうかを確認
print(isinstance(index, pd.Float64Index))
# 出力: True
np.issubdtype
関数は、オブジェクトのデータ型が NumPy のデータ型のサブタイプかどうかを判断するのに使用できます。
import pandas as pd
import numpy as np
# 浮動小数点型の Index オブジェクトを作成
index = pd.Index([1.2, 3.4, 5.6])
# `np.issubdtype` 関数を使用して、Index オブジェクトのデータ型が NumPy の float64 型のサブタイプかどうかを確認
print(np.issubdtype(index.dtype, np.float64))
# 出力: True
これらの方法は、pandas.Index.is_floating
メソッドよりも簡潔に記述できますが、pandas.Index.is_floating
メソッドの方がコードの可読性が高くなります。
Pandas Data Offsets と LastWeekOfMonth.is_month_end 以外の方法
Pandas Data Offsets は、日付や時刻を操作するための便利なツールです。特定の期間(日、週、月など)を簡単に追加したり、差を取ったりすることができます。LastWeekOfMonth. is_month_end は、特定の日付がその月の最後の週かどうかを示す属性です。
Pandas Data Offsets でカスタムビジネス月の末日を扱う:詳細解説とサンプルコード
pandas. tseries. offsets. CustomBusinessMonthEnd は、Pandas の Data Offsets ライブラリで提供されるクラスであり、カスタムビジネス月の末日 を基準とした日付オフセットを定義します。このオフセットは、指定された月数だけ、平日のみ を進めることができます。

Pandasで月末から2週間後の最初の月曜日を判定:SemiMonthBegin.onOffset徹底解説
pandas. tseries. offsets. SemiMonthBegin は、pandasライブラリで提供される日付オフセットの一つです。これは、月末から2週間後に発生する最初の月曜日を基準とするオフセットです。SemiMonthBegin
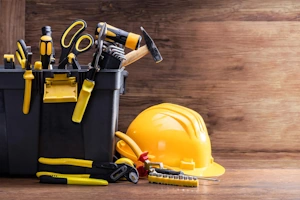
Pandas WeekOfMonth.is_quarter_start 属性のユースケース
この解説は、Python ライブラリ Pandas の Data Offsets 機能と、WeekOfMonth オブジェクトの is_quarter_start 属性について、プログラミング初心者にも分かりやすく説明することを目的としています。
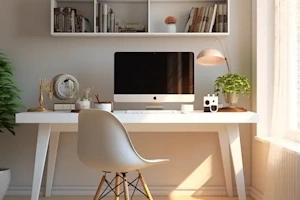
Pandas.tseries.offsets.CustomBusinessMonthBeginを使いこなす
pandas. tseries. offsets. CustomBusinessMonthBegin. rollback は、Pandasの「Data offsets」機能で、カスタムビジネス月始のオフセットを指定された日付から過去方向にロールバックするために使用されます。
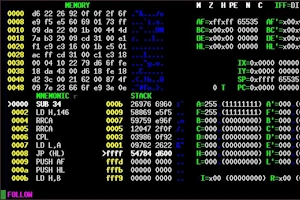
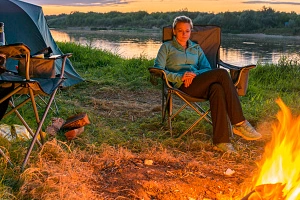
Pandas Series の append() メソッド:サンプルコード
append() メソッドは、以下の引数を受け取ります。to_append: 結合する Seriesignore_index: True の場合、結合後の Series のインデックスは元の Series のインデックスを無視します。デフォルトは False です。
IntervalIndex.get_loc メソッドのサンプルコード
pandas. IntervalIndex. get_loc メソッドは、IntervalIndex 内で特定の値の位置を取得するために使用されます。これは、データフレームの特定の行や列にアクセスしたり、データの分析や可視化を行ったりする際に役立ちます。
Pandas Data Offsets:BusinessDay.normalize メソッドの完全ガイド
Pandas の Data Offsets は、日付や時間軸データを操作するための便利なツールです。BusinessDay は、営業日ベースで日付をオフセットするための機能です。BusinessDay. normalize は、BusinessDay オフセットを正規化し、午前0時0分0秒に揃えるためのメソッドです。
Pandas DatetimeIndex.is_month_start 完全ガイド
pandas. DatetimeIndex. is_month_start は、DatetimeIndex の各日付がその月の最初の日かどうかを示すブーリアン値の配列を返す属性です。例出力の説明True は、その日付がその月の最初の日であることを示します。
Pandas DataFrame の replace メソッド vs その他の置換方法: 速度比較と使い分け
pandas. DataFrame. replace メソッドは、DataFrame 内の特定の値を別の値に置き換えるために使用されます。これは、データのクリーニング、欠損値の処理、または単純にデータの値を変更したい場合に役立ちます。基本的な使い方