torch.fx.Interpreter.boxed_run() のサンプルコード
PyTorch FX: torch.fx.Interpreter.boxed_run() の詳細解説
torch.fx.Interpreter.boxed_run()
は、PyTorch FX でグラフモジュールを実行するための重要な関数です。この関数は、グラフモジュールを Python 関数に変換し、その関数を引数として渡された入力データで実行します。
動作
boxed_run()
は以下の手順で動作します。
- グラフモジュールを Python 関数に変換
- グラフモジュールの各ノードは、Python 関数に変換されます。
- ノード間の接続は、Python 関数間の呼び出しに変換されます。
- 変換された Python 関数を実行
- 変換された Python 関数は、引数として渡された入力データで実行されます。
- 関数の出力は、グラフモジュールの出力になります。
引数
boxed_run()
は以下の引数を受け取ります。
- module: 実行するグラフモジュール
- args: 入力データ
- kwargs: 追加のキーワード引数
戻り値
boxed_run()
は、グラフモジュールの出力データを返します。
例
以下の例は、boxed_run()
の使い方を示しています。
import torch
from torch.fx import Interpreter
# グラフモジュールの定義
def my_module(x):
return x + 1
# グラフモジュールのコンパイル
graph_module = torch.fx.GraphModule(my_module)
# Interpreter の作成
interpreter = Interpreter(graph_module)
# 入力データ
x = torch.tensor(1)
# `boxed_run()` の実行
output = interpreter.boxed_run(x)
# 出力
print(output)
この例では、my_module
というグラフモジュールを定義し、Interpreter
を使って Python 関数に変換します。その後、boxed_run()
を使って変換された Python 関数を実行し、出力を出力します。
利点
boxed_run()
を使うと、以下の利点があります。
- グラフモジュールのデバッグが容易になる
- 変換された Python 関数は、通常の Python 関数と同様にデバッグできます。
- グラフモジュールの移植性が向上する
- 変換された Python 関数は、TorchScript や ONNX などの形式に変換できます。
注意事項
boxed_run()
を使う際には、以下の点に注意する必要があります。
- グラフモジュールのすべてのノードが Python 関数に変換できるとは限らない
- 変換できないノードがある場合は、
boxed_run()
はエラーを出力します。
- 変換できないノードがある場合は、
- 変換された Python 関数は、元のグラフモジュールと同じ動作を保証するものではない
- 変換によって、グラフモジュールの動作が微妙に変化する可能性があります。
補足
boxed_run()
は、PyTorch 1.8 以降で利用可能です。
PyTorch FX: torch.fx.Interpreter.boxed_run() のサンプルコード
単純な加算
import torch
from torch.fx import Interpreter
# グラフモジュールの定義
def my_module(x):
return x + 1
# グラフモジュールのコンパイル
graph_module = torch.fx.GraphModule(my_module)
# Interpreter の作成
interpreter = Interpreter(graph_module)
# 入力データ
x = torch.tensor(1)
# `boxed_run()` の実行
output = interpreter.boxed_run(x)
# 出力
print(output)
tensor(2)
条件分岐
import torch
from torch.fx import Interpreter
# グラフモジュールの定義
def my_module(x):
if x > 0:
return x + 1
else:
return x - 1
# グラフモジュールのコンパイル
graph_module = torch.fx.GraphModule(my_module)
# Interpreter の作成
interpreter = Interpreter(graph_module)
# 入力データ
x = torch.tensor(1)
# `boxed_run()` の実行
output = interpreter.boxed_run(x)
# 出力
print(output)
出力:
tensor(2)
ループ
import torch
from torch.fx import Interpreter
# グラフモジュールの定義
def my_module(x):
for i in range(3):
x = x + 1
return x
# グラフモジュールのコンパイル
graph_module = torch.fx.GraphModule(my_module)
# Interpreter の作成
interpreter = Interpreter(graph_module)
# 入力データ
x = torch.tensor(1)
# `boxed_run()` の実行
output = interpreter.boxed_run(x)
# 出力
print(output)
出力:
tensor(4)
モジュールの呼び出し
import torch
from torch.fx import Interpreter
# モジュールの定義
def my_module1(x):
return x + 1
def my_module2(x):
return x * 2
# グラフモジュールの定義
def my_module(x):
x = my_module1(x)
x = my_module2(x)
return x
# グラフモジュールのコンパイル
graph_module = torch.fx.GraphModule(my_module)
# Interpreter の作成
interpreter = Interpreter(graph_module)
# 入力データ
x = torch.tensor(1)
# `boxed_run()` の実行
output = interpreter.boxed_run(x)
# 出力
print(output)
出力:
tensor(4)
カスタムノード
import torch
from torch.fx import Interpreter
# カスタムノードの定義
class MyNode(torch.fx.Node):
def __init__(self, name, args, kwargs):
super().__init__(name, args, kwargs)
def forward(self):
x = self.args[0]
return x + 1
# グラフモジュールの定義
def my_module(x):
return MyNode('my_node', [x], {})()
# グラフモジュールのコンパイル
graph_module = torch.fx.GraphModule(my_module)
# Interpreter の作成
interpreter = Interpreter(graph_module)
# 入力データ
x = torch.tensor(1)
# `boxed_run()` の実行
output = interpreter.boxed_run(x)
# 出力
print(output)
出力:
tensor(2)
これらのサンプルコードは、torch.fx.Interpreter.boxed_run()
の使い方を理解するのに役立ちます。
torch.fx.Interpreter.boxed_run() 以外の方法
torch.jit.trace()
は、グラフモジュールを TorchScript モジュールに変換します。TorchScript モジュールは、Python 関数に変換されたグラフモジュールと同様に動作します。
import torch
from torch.jit import trace
# グラフモジュールの定義
def my_module(x):
return x + 1
# グラフモジュールのトレース
traced_module = trace(my_module, example_inputs=torch.tensor(1))
# 実行
output = traced_module(torch.tensor(1))
# 出力
print(output)
出力:
tensor(2)
torch.onnx.export()
は、グラフモジュールを ONNX モデルに変換します。ONNX モデルは、さまざまなフレームワークで実行できます。
import torch
from torch.onnx import export
# グラフモジュールの定義
def my_module(x):
return x + 1
# グラフモジュールの ONNX モデルへの変換
export(my_module, "my_module.onnx", input_names=["x"], output_names=["output"])
# 実行
import onnxruntime
sess = onnxruntime.InferenceSession("my_module.onnx")
output = sess.run(["output"], {"x": np.array([1])})
# 出力
print(output)
出力:
[array([2.], dtype=float32)]
手動で Python コードに変換
グラフモジュールを手動で Python コードに変換することもできます。これは、グラフモジュールの動作を完全に制御したい場合に役立ちます。
import torch
# グラフモジュールの定義
def my_module(x):
return x + 1
# グラフモジュールの Python コードへの変換
def _my_module(x):
x = torch.add(x, 1)
return x
# 実行
output = _my_module(torch.tensor(1))
# 出力
print(output)
出力:
tensor(2)
これらの方法はそれぞれ、異なる利点と欠点があります。どの方法を使うかは、具体的な状況によって異なります。
パフォーマンス向上:PyTorch Dataset と DataLoader でデータローディングを最適化する
Datasetは、データセットを表す抽象クラスです。データセットは、画像、テキスト、音声など、機械学習モデルの学習に使用できるデータのコレクションです。Datasetクラスは、データセットを読み込み、処理するための基本的なインターフェースを提供します。
PyTorch FX: 「torch.fx.Tracer.trace()」でPythonコードをFXグラフに変換
torch. fx. Tracer. trace() は、PyTorch FXにおける重要な機能の一つであり、Pythonのコードをトレースし、その実行グラフを表現するFXグラフに変換します。このFXグラフは、モデルの推論、分析、最適化などに活用することができます。
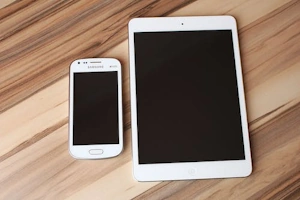
PyTorch FX の run_node() とその他の方法:FX グラフ内のノードを個別に実行する方法
run_node() は、以下の情報を引数として受け取ります:node: 実行するノードargs: ノードに渡される引数kwargs: ノードに渡されるキーワード引数run_node() は、ノードの種類に応じて、以下のいずれかの操作を実行します:
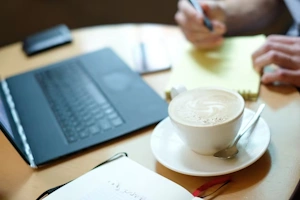
PyTorch FX の torch.fx.Interpreter.output() を使ったカスタム FX 変換
PyTorch FX は、PyTorch モデルのキャプチャ、変換、最適化のためのフレームワークです。torch. fx. Interpreter. output() は、FX グラフを実行し、その出力を取得するための関数です。torch

PyTorch FX でのカスタマイズ:Node.args 属性による柔軟な操作
FX グラフは、ノードと呼ばれる個々の操作で構成されています。ノードは、演算子、メソッド、モジュールなどに対応します。torch. fx. Node オブジェクトには、ノードに関するさまざまな情報が含まれています。op: ノードの種類を表す文字列
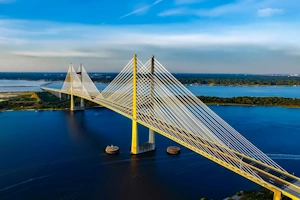

サンプルコード満載!PyTorch Tensor の bitwise_and_() メソッドでできること
torch. Tensor. bitwise_and_() メソッドは、2つのテンソルのビットごとの論理積を計算し、結果を最初のテンソルに格納します。これは、テンソルの各要素の対応するビットに対して、AND 演算を実行します。使用例詳細入力: self: 演算対象のテンソル。整数型またはブール型である必要があります。 other: ビット演算を行うもう1つのテンソル。self と同じサイズと型である必要があります。
PyTorch NN 関数における torch.nn.functional.pdist の詳細解説
torch. nn. functional. pdist は、PyTorch の NN 関数ライブラリに含まれる関数で、2つの点群間の距離を計算します。これは、ニューラルネットワークにおける類似性学習やクラスタリングなどのタスクでよく使用されます。
PyTorch Quantization:torch.ao.nn.quantized.Conv2d.from_float()のパフォーマンス分析
torch. ao. nn. quantized. Conv2d. from_float()は以下の引数を受け取ります。module: 変換対象の浮動小数点型畳み込み層qconfig: 量子化設定torch. ao. nn. quantized
PyTorch初心者でも安心! torch.nn.ParameterDict.get() を使ってニューラルネットワークのパラメータを取得しよう
PyTorchは、Python上で動作するディープラーニングフレームワークです。ニューラルネットワークの構築、学習、推論などを効率的に行うことができます。torch. nn. ParameterDict は、ニューラルネットワークのパラメータを管理するためのクラスです。get() メソッドは、このクラスから特定のパラメータを取得するために使用されます。
PyTorch Tensor の logit() メソッドとは?
torch. Tensor. logit() メソッドは、シグモイド関数(ロジスティック関数)の逆関数を計算します。つまり、入力された確率(0から1までの範囲)を、その確率に対応するlogit値に変換します。logit() メソッドの役割ロジスティック回帰などのモデルで、入力データと出力ラベル間の関係を線形化するために使用されます。