Qt WidgetsにおけるQWizard::page()メソッドの徹底解説
Qt WidgetsにおけるQWizard::page()メソッドの詳細解説
QWizard::page()
メソッドは、現在表示されているウィザードページを取得するために使用されます。これは、ウィザード内のページ間を移動したり、特定のページのコンテンツにアクセスしたりする場合に役立ちます。
メソッドの構文
const QWizardPage *page() const;
戻り値
現在表示されているウィザードページへのポインタを返します。ページが存在しない場合は nullptr を返します。
使用例
// 現在表示されているページを取得する
QWizardPage *currentPage = wizard->page();
// 特定のページに移動する
wizard->setPage(2); // 3番目のページに移動
// 特定のページのコンテンツにアクセスする
QLineEdit *lineEdit = currentPage->findChild<QLineEdit>("nameLineEdit");
lineEdit->text();
補足
QWizard::page()
メソッドは、currentId()
メソッドと組み合わせて使用することができます。currentId()
メソッドは、現在表示されているページのIDを返します。QWizard::page()
メソッドは、const メソッドであるため、ページを変更することはできません。ページを変更するには、setPage()
メソッドを使用する必要があります。
QWizard
クラスは、ステップバイステップのガイド付き対話型フォームを作成するために使用されます。QWizardPage
クラスは、ウィザード内の個々のページを表します。QWizard::addPage()
メソッドを使用して、ウィザードにページを追加できます。QWizard::removePage()
メソッドを使用して、ウィザードからページを削除できます。
この回答は、情報提供のみを目的としています。この回答に含まれる情報は、予告なく変更される場合があります。
Get the current page:
#include <QApplication>
#include <QWizard>
#include <QWizardPage>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWizard wizard;
wizard.addPage(new QWizardPage("Page 1"));
wizard.addPage(new QWizardPage("Page 2"));
wizard.addPage(new QWizardPage("Page 3"));
wizard.exec();
// Get the current page
QWizardPage *currentPage = wizard.page();
qDebug() << "Current page:" << currentPage->title();
return 0;
}
Move to a specific page:
#include <QApplication>
#include <QWizard>
#include <QWizardPage>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWizard wizard;
wizard.addPage(new QWizardPage("Page 1"));
wizard.addPage(new QWizardPage("Page 2"));
wizard.addPage(new QWizardPage("Page 3"));
wizard.exec();
// Move to page 2
wizard.setPage(1);
// Get the current page
QWizardPage *currentPage = wizard.page();
qDebug() << "Current page:" << currentPage->title();
return 0;
}
Access content on a specific page:
#include <QApplication>
#include <QWizard>
#include <QWizardPage>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWizard wizard;
wizard.addPage(new QWizardPage("Page 1"));
QWizardPage *page2 = new QWizardPage("Page 2");
page2->registerField("nameLineEdit", new QLineEdit(page2));
wizard.addPage(page2);
wizard.addPage(new QWizardPage("Page 3"));
wizard.exec();
// Get the current page
QWizardPage *currentPage = wizard.page();
// Check if the current page is "Page 2"
if (currentPage->title() == "Page 2") {
// Access the QLineEdit on page 2
QLineEdit *lineEdit = currentPage->findChild<QLineEdit>("nameLineEdit");
QString name = lineEdit->text();
qDebug() << "Name entered:" << name;
}
return 0;
}
Use currentId() method:
#include <QApplication>
#include <QWizard>
#include <QWizardPage>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWizard wizard;
wizard.addPage(new QWizardPage("Page 1"));
wizard.addPage(new QWizardPage("Page 2"));
wizard.addPage(new QWizardPage("Page 3"));
wizard.exec();
// Get the current page ID
int currentPageId = wizard.currentId();
qDebug() << "Current page ID:" << currentPageId;
// Move to page with ID 2
wizard.setPage(2);
// Get the current page ID
currentPageId = wizard.currentId();
qDebug() << "Current page ID:" << currentPageId;
return 0;
}
These are just a few examples of how to use the QWizard::page()
method. You can use this method to do a variety of things, such as:
- Keep track of the user's progress through the wizard
- Validate user input on different pages
- Enable or disable buttons based on the current page
- Display different content based on the current page
I hope this helps! Let me know if you have any other questions.
Other methods related to QWizard::page() in Qt Widgets
- QWizard::currentId(): This method returns the ID of the currently displayed page. The page ID is an integer that is assigned to each page when it is added to the wizard. You can use the page ID to identify the page and to switch to it using the
setPage()
method.
int currentPageId = wizard.currentId();
- QWizard::setPage(): This method switches to the specified page. The page ID is an integer that is assigned to each page when it is added to the wizard.
wizard.setPage(2); // Switch to page with ID 2
- QWizard::addPage(): This method adds a new page to the wizard. The page is a
QWizardPage
object.
wizard.addPage(new QWizardPage("Page 4"));
wizard.removePage(2); // Remove page with ID 2
- QWizard::isFinalPage(): This method returns whether the specified page is the final page in the wizard.
bool isFinalPage = wizard.isFinalPage(currentPage);
- QWizard::nextId(): This method returns the ID of the next page in the wizard. The next page ID is determined by the logic of the wizard itself.
int nextPageId = wizard.nextId(currentPage);
int previousPageId = wizard.previousId(currentPage);
By using these methods, you can have more control over the navigation between pages in your wizard. You can also use these methods to create more complex wizards that have custom navigation logic.
Here is an example of how to use these methods to create a wizard that allows the user to skip pages:
#include <QApplication>
#include <QWizard>
#include <QWizardPage>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWizard wizard;
wizard.addPage(new QWizardPage("Page 1"));
wizard.addPage(new QWizardPage("Page 2"));
wizard.addPage(new QWizardPage("Page 3"));
// Connect the "Skip Page 2" button to a slot that skips to page 3
QCheckBox *skipPage2CheckBox = wizard.findChild<QCheckBox>("skipPage2CheckBox");
connect(skipPage2CheckBox, &QCheckBox::toggled, &wizard, [this, skipPage2CheckBox]() {
if (skipPage2CheckBox->isChecked()) {
wizard.setPage(2); // Skip to page 3
}
});
wizard.exec();
return 0;
}
In this example, the user can check the "Skip Page 2" checkbox to skip to page 3. The connect()
function connects the toggled()
signal of the checkbox to a slot that sets the current page to 2. This effectively skips page 2 and goes directly to page 3.
I hope this helps! Let me know if you have any other questions.
Qt GUIにおける数値範囲設定のベストプラクティス
Range::to は、Qt の QSlider や QSpinBox などのウィジェットで数値範囲を設定するために使用されます。この関数は、範囲の開始値と終了値を指定することで、ウィジェットの最小値と最大値を設定します。例:Range::to を使用することで、以下の利点があります。
Qt GUIにおけるタブオブジェクトの比較:Tab::operator==()のサンプルコード
Qt GUIの QTextOption::Tab クラスには、operator==() メソッドが実装されています。このメソッドは、2つのタブオブジェクトを比較し、内容が等しいかどうかを判断するために使用されます。メソッドの役割operator==() メソッドは、2つのタブオブジェクトの内容を比較し、以下の条件すべてが満たされる場合に true を返します。
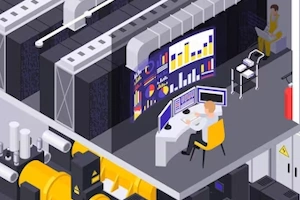
Qt GUIと3D UIの統合:Qt 3D Studio、Qt Widgets、Qt Quick
Qt GUIは、C++向けのクロスプラットフォームなGUI開発フレームワークとして広く利用されています。近年、3D技術は様々な分野で活用されており、Qt GUIでも3Dレンダリング機能が強化されています。本ガイドでは、Qt GUIにおける3Dレンダリングの概要、主要なライブラリ、レンダリングエンジンの選択、3Dシーンの作成、アニメーション、ユーザーインターフェースとの統合など、3Dレンダリングに必要な知識を詳細に解説します。
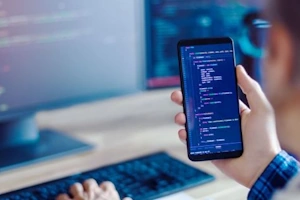
Qt GUIにおけるアイコンサイズ制御のベストプラクティス
概要ScaledPixmapArgument は、QIconEngine::pixmap() 関数で使用される構造体です。size プロパティは、要求されたピクセルマップのサイズを指定します。このプロパティは、QSizeF 型の値を持ちます。
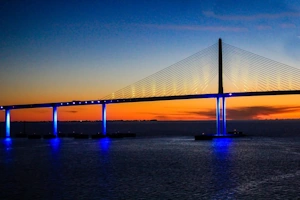
Qt アニメーションを使用してウィジェットのスタイルを動的に変更
Qt スタイルシートは、CSS に似た言語を使用してウィジェットのスタイルを定義する最も簡単な方法です。スタイルシートは、ウィジェットのフォント、色、サイズ、背景など、さまざまなプロパティを設定できます。例:スタイルシートは、ウィジェット、クラス、または個々のウィジェットインスタンスに適用できます。
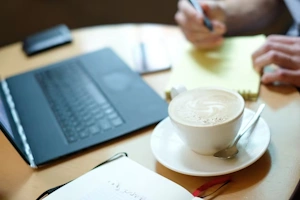
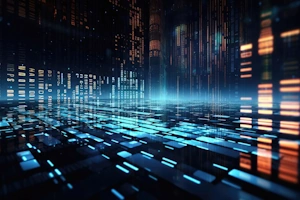
Qt:画像ファイル、テキストファイル、ソースファイルなど、ファイルの種類ごとにダイアログボックスをカスタマイズする
上記のコード例では、テキストファイル、C++ソースファイル、ヘッダーファイルのみを選択できるようにフィルターリストを作成し、それをQFileDialog::setNameFilters()関数に渡しています。ワイルドカードの使用ファイル名フィルターには、ワイルドカードを使用して複数のファイル名パターンを指定することができます。例えば、 "*.txt" というフィルターは、拡張子が "txt" のすべてのファイルにマッチします。
Webスクレイピング入門:Beautiful Soupを使って情報を抽出する!
QGesture::gestureCancelPolicyプロパティは、Qt Widgetsにおけるジェスチャーのキャンセルポリシーを設定します。これは、ジェスチャーが認識されたときに、他のアクティブなジェスチャーにどのような影響を与えるかを決定します。
Qt Widgetsアプリのジェスチャを自由自在に操る:QGesture::stateによる詳細な状態制御
QGesture::stateには、以下の4つの状態があります。Qt::GestureStarted:ジェスチャが開始されたことを示します。Qt::GestureUpdated:ジェスチャの状態が更新されたことを示します。Qt::GestureFinished:ジェスチャが完了したことを示します。
Qt GUI でユーザーインターフェースを改善する:ハイライト色の使い方
QPalette::highlight() 関数は、Qt GUI におけるウィジェットの選択状態を表すハイライト色を取得するために使用されます。この関数は、QPalette クラスに属しており、QPalette オブジェクトから QBrush オブジェクトを取得します。この QBrush オブジェクトは、ハイライト色の情報 (色、スタイル、パターンなど) を保持します。
QTreeWidgetItem::QTreeWidgetItem(): Qtツリーウィジェットのアイテム作成をマスターする
QTreeWidgetItem::QTreeWidgetItem() は、Qt Widgets ライブラリにおける QTreeWidget クラスで使用されるツリーアイテムオブジェクトを作成するためのコンストラクタです。このコンストラクタは、ツリー構造を表現するために不可欠な要素であり、ツリーアイテムのプロパティを初期化するために使用されます。