Djangoの django.db.migrations.operations.AlterUniqueTogether をわかりやすく解説!
What is AlterUniqueTogether?
AlterUniqueTogether
is a Django migration operation that is used to modify the unique_together
constraint on a model's Meta
class. The unique_together
constraint ensures that no two instances of a model can have the same combination of values for the specified fields.
When to use AlterUniqueTogether?
You should use AlterUniqueTogether
when you need to:
- Add a new unique constraint to a model
- Remove an existing unique constraint from a model
- Change the fields that are included in an existing unique constraint
Syntax of AlterUniqueTogether
The AlterUniqueTogether
operation takes two arguments:
name
: The name of the model to modifyunique_together
: A list of tuples, where each tuple specifies the fields that should be included in the unique constraint
Example of AlterUniqueTogether
Here is an example of how to use AlterUniqueTogether
to add a new unique constraint to the Author
model:
from django.db.migrations import operations
class Migration(migrations.Migration):
dependencies = [
('myapp', '0001_initial'),
]
operations = [
operations.AlterUniqueTogether(
name='Author',
unique_together=[('first_name', 'last_name')],
),
]
This migration will add a unique constraint to the Author
model that ensures that no two authors can have the same first name and last name.
Additional Notes
- The
AlterUniqueTogether
operation is reversible. This means that you can migrate your database back to the previous state if you need to. - The
AlterUniqueTogether
operation will automatically create or drop the appropriate database constraints. - If you are using a database that does not support unique constraints, the
AlterUniqueTogether
operation will create indexes instead.
I hope this explanation is helpful. Please let me know if you have any other questions.
If you're looking for something specific, please let me know and I'll be happy to help.
Please provide more details so I can assist you better.
Django 汎用表示ビューとその他のAPI開発方法の比較
Djangoの汎用表示ビューは、以下の4つの主要なクラスで構成されています。ListView: モデルのオブジェクト一覧を表示します。DetailView: モデルの個別のオブジェクトを表示します。CreateView: モデルの新しいオブジェクトを作成します。
Django フォームフィールド API のサンプルコード
フォームフィールドは、ユーザー入力を受け取るための個別の要素です。名前、メールアドレス、パスワードなど、さまざまな種類のデータに対応できます。主なフォームフィールドの種類:CharField: テキスト入力EmailField: メールアドレス入力
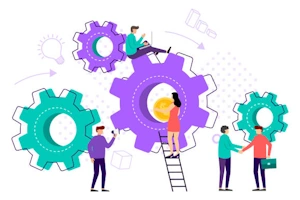
Django フォーム レンダリング API を使わない方がいい場合
テンプレートベースのレンダリング: フォームは、Django テンプレートエンジンを使用して HTML にレンダリングされます。これにより、フォームの外観と動作を完全にカスタマイズできます。ウィジェット: フォームフィールドは、さまざまなウィジェットを使用してレンダリングされます。各ウィジェットは、特定の種類の入力フィールド (テキスト入力、選択リストなど) をレンダリングします。
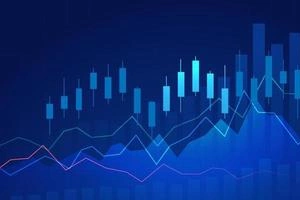
FeedBurnerで簡単フィード配信!Djangoとの連携方法
Djangoでフィードを作成するには、以下の手順を行います。django. contrib. syndication モジュールをインポートする。フィードの内容となるモデルを定義する。フィードクラスを作成する。フィードのURLパターンを設定する。
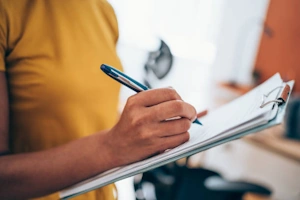
Django システムチェックフレームワーク: あなたのプロジェクトを守るための必須ツール
仕組みシステムチェックフレームワークは、以下の3つのステップで動作します。チェックの収集: Djangoは、データベース接続、キャッシュバックエンド、テンプレートエンジンなど、さまざまなコンポーネントに関するチェックを自動的に収集します。チェックの実行: 収集されたチェックは、1つずつ実行されます。
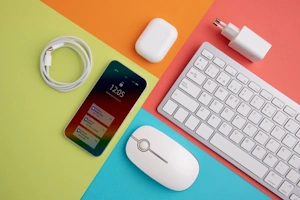
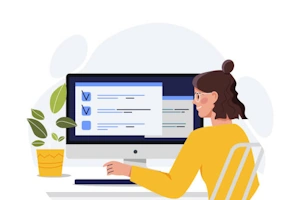
Django django.db.models.ForeignKey.to_field 詳細解説
django. db. models. ForeignKey. to_fieldは、Django ORMで使用する外部キーの関連フィールドを指定するオプションです。デフォルトでは関連モデルのプライマリキーを参照しますが、to_fieldを指定することで、別のフィールドを参照することができます。
Django admin.ModelAdmin.readonly_fields 詳細解説
django. contrib. admin の admin. ModelAdmin. readonly_fields は、Django 管理サイトで特定のフィールドを 読み取り専用 に設定するための強力なツールです。この属性を使用すると、ユーザーが編集できないフィールドを指定できます。
django.contrib.postgres.forms.BaseRangeField.base_field 属性の詳細解説
BaseRangeFieldクラスにはbase_fieldという属性があります。これは、範囲フィールドを構成する基盤となるフィールドを表します。つまり、範囲フィールドは2つの基盤フィールドで構成されており、base_field属性はそのうちの一つを参照します。
Django forms.BoundField.form に関する参考資料
forms. BoundField. formは、Djangoフォームフレームワークにおける重要な属性です。これは、フォームフィールドとその関連するフォームインスタンス間の接続を提供します。この属性への理解は、Djangoフォームの動作を理解し、フォームをより効果的に使用するために不可欠です。
Django QuerySet.difference() メソッドで差集合を効率的に取得
QuerySet. difference()メソッドは、2つのQuerySetから共通する要素を除いた結果を返すメソッドです。つまり、2つのQuerySetの差集合を求めることができます。使い方QuerySet. difference()メソッドは、次の形式で使用します。