Djangoの「django.views」における「views.generic.base.TemplateResponseMixin.response_class」属性の徹底解説
Djangoの「django.views」における「views.generic.base.TemplateResponseMixin.response_class」の詳細解説
django.views.generic.base.TemplateResponseMixin
クラスのresponse_class
属性は、テンプレートレンダリング時に使用されるレスポンスクラスを決定します。これは、Djangoのジェネリックビューで使用される重要な属性です。
デフォルトでは、response_class
はdjango.template.response.TemplateResponse
に設定されています。これは、テンプレートをレンダリングして、HTTPレスポンスとして返します。
カスタマイズ
response_class
属性をカスタマイズすることで、独自のレスポンスクラスを使用することができます。これは、以下のような場合に役立ちます。
- 特定のコンテンツタイプを設定したい場合
- レスポンスヘッダーをカスタマイズしたい場合
- カスタムのレンダリングロジックを実装したい場合
例
以下の例では、response_class
属性を使用して、JSONレスポンスを返すカスタムのJsonResponse
クラスを使用しています。
from django.views.generic import View
from django.http import JsonResponse
class MyView(View):
def get(self, request):
data = {'message': 'Hello, world!'}
return JsonResponse(data)
この例では、MyView
クラスはView
クラスを継承し、get()
メソッドをオーバーライドしています。get()
メソッドは、JsonResponse
クラスを使用して、data
ディクショナリーを含むJSONレスポンスを返します。
response_class属性を使用する際の注意点
- 使用するカスタムレスポンスクラスは、
django.template.response.TemplateResponse
クラスと同じインターフェースを実装する必要があります。 - カスタムレスポンスクラスは、
django.utils.decorators.method_decorator
デコレータを使用して、get_template_names()
、get_context_data()
、render_to_response()
などのメソッドをラップする必要があります。
補足
- 上記の情報に加えて、以下の点にも注意が必要です。
response_class
属性は、テンプレートレンダリングにのみ使用されます。他の種類のビューでは使用されません。response_class
属性をカスタマイズする場合は、パフォーマンスへの影響を考慮する必要があります。
django.views.generic.base.TemplateResponseMixin.response_class
属性について、さらに質問がある場合は、遠慮なく聞いてください。
Djangoの「django.views」における「views.generic.base.TemplateResponseMixin.response_class」属性のサンプルコード
from django.views.generic import View
from django.http import JsonResponse
class MyView(View):
def get(self, request):
data = {'message': 'Hello, world!'}
return JsonResponse(data)
カスタムのコンテンツタイプを設定
from django.views.generic import View
from django.http import HttpResponse
class MyView(View):
def get(self, request):
response = HttpResponse('Hello, world!')
response['Content-Type'] = 'text/plain'
return response
レスポンスヘッダーをカスタマイズ
from django.views.generic import View
from django.http import HttpResponse
class MyView(View):
def get(self, request):
response = HttpResponse('Hello, world!')
response['Cache-Control'] = 'max-age=600'
return response
カスタムのレンダリングロジックを実装
from django.views.generic import View
from django.template import loader
class MyView(View):
def get(self, request):
template = loader.get_template('my_template.html')
context = {'message': 'Hello, world!'}
html = template.render(context)
return HttpResponse(html)
テンプレートレンダリングを無効化
from django.views.generic import View
class MyView(View):
def get(self, request):
return HttpResponse('Hello, world!')
def render_to_response(self, context):
return super().render_to_response(context, template_name=None)
Djangoのテンプレートレンダリングを行う他の方法
from django.template import loader
template = loader.get_template('my_template.html')
context = {'message': 'Hello, world!'}
html = template.render(context)
return HttpResponse(html)
django.shortcuts.render()を使用する
from django.shortcuts import render
return render(request, 'my_template.html', {'message': 'Hello, world!'})
カスタムビューを使用する
from django.views.generic import View
class MyView(View):
def get(self, request):
template = loader.get_template('my_template.html')
context = {'message': 'Hello, world!'}
html = template.render(context)
return HttpResponse(html)
それぞれの方法の比較
方法 | 利点 | 欠点 |
---|---|---|
django.template.loader.render_to_string() | シンプルで使いやすい | ビューロジックをテンプレートファイルに混入させる必要がある |
django.shortcuts.render() | ビューロジックとテンプレートを分離できる | コード量が少し多くなる |
カスタムビュー | 柔軟性が高い | コード量が多くなる |
- シンプルなテンプレートレンダリングの場合は、
django.template.loader.render_to_string()
を使用するのがおすすめです。 - ビューロジックとテンプレートを分離したい場合は、
django.shortcuts.render()
を使用するのがおすすめです。 - より柔軟なテンプレートレンダリングが必要な場合は、カスタムビューを使用するのがおすすめです。
- Djangoには、テンプレートレンダリングを行うための他にも様々な方法があります。
- 詳細については、Djangoドキュメントを参照してください。
Django フォームのサンプルコード
このガイドでは、以下の内容をより詳細に、分かりやすく解説します。フォームの作成フォームは forms. py ファイルで定義します。ここでは、フォームの各フィールドとその属性を記述します。フィールドの種類 文字列型 (CharField) テキストエリア (TextField) 選択肢 (ChoiceField) チェックボックス (BooleanField) ファイルアップロード (FileField) その他多数
MonthArchiveView を使ってブログ記事のアーカイブページを作成する
MonthArchiveView を使用するには、以下の手順が必要です。モデルを定義する: MonthArchiveView は、日付フィールドを持つモデルが必要です。モデルは、django. db. models. Model から継承する必要があります。
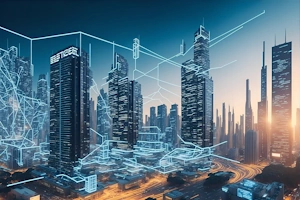
django.views.generic.base.TemplateResponseMixin.template_name 以外のテンプレートファイルの指定方法
django. views. generic. base. TemplateResponseMixin. template_name は、Djangoのジェネリックビューでテンプレートファイルを指定するために使用する属性です。この属性は、テンプレート名を文字列として設定し、ビューがレンダリングするテンプレートを決定します。
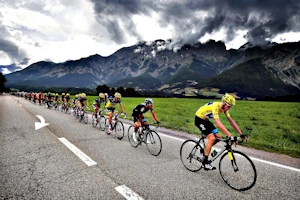
Django views.generic.dates.WeekMixin.get_next_week() の徹底解説
このメソッドは、以下の引数を受け取ります:date: 現在の週の日付を表す datetime. date オブジェクトweek_delta: 次の週までの週数差を表す整数get_next_week() は、以下の処理を行います:現在の週の日付に week_delta を加算します。
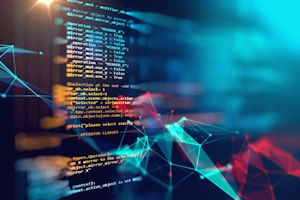
Django views.generic.edit.ModelFormMixin.form_invalid() サンプルコード集
django. views. generic. edit. ModelFormMixin. form_invalid() は、Django のジェネリックビューにおいて、モデルフォームのバリデーションが失敗した場合に呼び出されるメソッドです。このメソッドは、エラーメッセージの表示やフォームの再描画など、バリデーションエラー後の処理をカスタマイズするために使用されます。
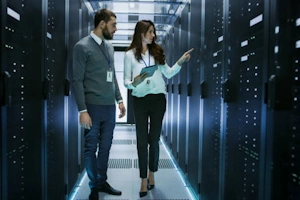
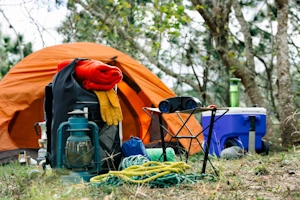
InlineModelAdmin.model と関連する属性
django. contrib. admin. InlineModelAdmin. model は、Django 管理画面でインライン編集機能を提供するために使用する重要な属性です。この属性は、インライン編集で扱いたい関連モデルを指定するために使用されます。
utils.functional.keep_lazy_text() デコレータ:Django テンプレートのパフォーマンスを向上させる方法
django. utils. functional. keep_lazy_text() は、Django テンプレート内で遅延評価されるテキストを保持するためのデコレータです。これは、テンプレートがレンダリングされる前に、テキストの評価を遅らせることで、パフォーマンスを向上させるのに役立ちます。
django.utils.decorators.method_decorator() のサンプルコード
概要method_decorator() は、デコレータをメソッドに適用できるようにするラッパーです。デコレータは、メソッド呼び出しの前に実行されるコードを追加する特殊な関数です。クラスベースビューでは、メソッドデコレータを使用して、ビューロジックを実行する前に特定の処理を実行できます。
Django forms.DateTimeField をマスターして、より高度なフォームを作成しよう
django. forms. DateTimeField. input_formats は、Django フォームでユーザーが入力した日付時刻文字列を datetime. datetime オブジェクトに変換するために使用されるフォーマットのリストです。デフォルトでは、以下のフォーマットが設定されています。
DjangoのWeekArchiveViewで特定の週に公開された記事を表示する
WeekArchiveViewは、Djangoのviews. generic. datesモジュールにあるクラスベースビューです。これは、特定の週に公開されたオブジェクトのリストを表示するビューを作成するための便利なツールです。機能特定の週に公開されたオブジェクトのリストを表示します。