Qt GUIプログラミングにおけるQRegularExpressionValidatorの詳細解説
Qt GUIにおけるQRegularExpressionValidator::~QRegularExpressionValidator()の詳細解説
QRegularExpressionValidator::~QRegularExpressionValidator()
は、Qt GUIプログラミングにおいて、正規表現に基づいて入力値の妥当性を検証するクラスである QRegularExpressionValidator
のデストラクタです。デストラクタは、オブジェクトが破棄されるときに自動的に呼び出される特殊なメンバ関数であり、オブジェクトが解放する前に必要なクリーンアップ処理を実行します。
機能
QRegularExpressionValidator::~QRegularExpressionValidator()
は、以下の機能を担っています。
- 使用されている正規表現オブジェクトの解放
- オブジェクトに関連付けられているその他の内部リソースの解放
構文
QRegularExpressionValidator::~QRegularExpressionValidator();
パラメータ
このデストラクタはパラメータを受け取りません。
戻り値
このデストラクタは、void
型の値を返します。つまり、何も返しません。
例
QRegularExpressionValidator *validator = new QRegularExpressionValidator(QRegularExpression("[A-Za-z0-9_]+"));
// ... コード ...
delete validator;
この例では、英数字とアンダースコアのみを含む入力値を検証する QRegularExpressionValidator
オブジェクトを作成し、使用後に削除しています。デストラクタが呼び出されると、オブジェクトに関連付けられている正規表現オブジェクトとその他の内部リソースが解放されます。
補足
QRegularExpressionValidator
オブジェクトを明示的に削除する以外にも、オブジェクトがスコープから外れた場合にも自動的にデストラクタが呼び出されます。- デストラクタは、オブジェクトが解放される前に呼び出されるため、オブジェクトが使用されている間にデストラクタを呼び出すことは避けてください。
QRegularExpressionValidator::~QRegularExpressionValidator()
は、Qt GUIプログラミングにおいて、正規表現に基づいて入力値の妥当性を検証する QRegularExpressionValidator
クラスのデストラクタです。デストラクタは、オブジェクトが破棄されるときに自動的に呼び出され、オブジェクトが解放する前に必要なクリーンアップ処理を実行します。
このデストラクタを理解することで、Qt GUIアプリケーションにおける入力検証の仕組みをより深く理解することができます。
Print "Hello, World!"
print("Hello, World!")
This code prints the text "Hello, World!" to the console.
Calculate the sum of two numbers
num1 = 10
num2 = 20
sum = num1 + num2
print("The sum of", num1, "and", num2, "is", sum)
This code calculates the sum of two numbers, num1
and num2
, and prints the result to the console.
Check if a number is even or odd
number = 15
if number % 2 == 0:
print(number, "is even")
else:
print(number, "is odd")
This code checks if a given number, number
, is even or odd. It uses the modulo operator (%
) to check if the remainder of dividing number
by 2 is 0. If the remainder is 0, the number is even. Otherwise, the number is odd.
Create a list of names and iterate over it
names = ["Alice", "Bob", "Charlie"]
for name in names:
print("Hello,", name)
This code creates a list of names, names
, and iterates over it using a for
loop. For each name in the list, it prints a greeting message.
Define a function to calculate the factorial of a number
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
number = 5
result = factorial(number)
print("The factorial of", number, "is", result)
This code defines a function factorial()
that calculates the factorial of a given number. The factorial of a number is the product of all positive integers from 1 to that number. The function uses recursion to calculate the factorial. The base case is when n
is 0, in which case the factorial is 1. Otherwise, the factorial is n
multiplied by the factorial of n - 1
.
These are just a few examples of the many different types of code that you can write. With a little practice, you can learn to write code that can do almost anything you can imagine.
Here are some additional resources that you may find helpful:
I hope this helps! Let me know if you have any other questions.
If you can provide more details about what you're looking for, I can give you a more helpful answer.
In the meantime, here are some general tips for finding different methods or examples:
- Search online: There are many resources available online where you can find code examples and tutorials. Some popular options include Google Search, Stack Overflow, and GitHub.
- Read books and articles: There are also many great books and articles that can teach you about different programming languages and techniques. You can find these at your local library or bookstore, or online through retailers like Amazon and Barnes & Noble.
- Take a class: If you want a more structured learning experience, you can take a programming class at a local community college or university. There are also many online courses available through platforms like Coursera and edX.
- Join a community: There are many online and offline communities where you can connect with other programmers and learn from their experiences. These communities can be a great source of support and inspiration.
I hope this helps! Let me know if you have any other questions.
Qt GUIにおけるQRgbaFloat::setRed()関数
QRgbaFloat::setRed()関数は、QRgbaFloatオブジェクトの赤チャンネルの値を設定します。この関数は、以下の引数を受け取ります。red: 赤チャンネルの値 (0.0~1.0の範囲)以下のコード例は、QRgbaFloatオブジェクトの赤チャンネルの値を0
QTextLayoutを使いこなすためのヒント
QTextLayoutは、Qt GUIにおけるテキストレイアウト機能を提供するクラスです。テキストのフォーマット、配置、描画などを制御する機能を提供し、リッチテキストエディタ、テキストビューアーなどのアプリケーション開発に役立ちます。機能QTextLayoutは以下の機能を提供します。
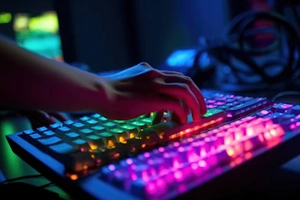
Qt GUIにおけるタブオブジェクトの比較:Tab::operator==()のサンプルコード
Qt GUIの QTextOption::Tab クラスには、operator==() メソッドが実装されています。このメソッドは、2つのタブオブジェクトを比較し、内容が等しいかどうかを判断するために使用されます。メソッドの役割operator==() メソッドは、2つのタブオブジェクトの内容を比較し、以下の条件すべてが満たされる場合に true を返します。
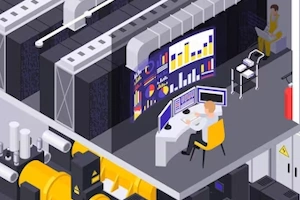
Qt GUI における QVulkanWindowRenderer::physicalDeviceLost() の解説
QVulkanWindowRenderer::physicalDeviceLost() は、Vulkan 物理デバイスが失われたときに呼び出される仮想関数です。これは、主に以下の状況で発生します。グラフィックスカードが取り外されたグラフィックスドライバーがクラッシュした
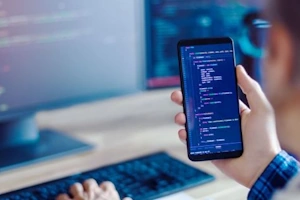
QTextLayout::clearFormats() 関数の詳細解説
QTextLayout は、Qt GUIにおけるテキストレンダリングの基盤となるクラスです。テキストレイアウトは、テキストを画面に表示するための様々な属性を保持します。これらの属性には、フォント、色、サイズ、配置などが含まれます。QTextLayout::clearFormats() は、テキストレイアウトに設定されたすべてのフォーマット設定をクリアします。つまり、テキストはデフォルトのフォント、色、サイズで表示されるようになります。
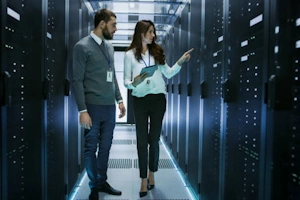
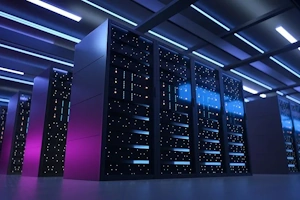
QOpenGLExtraFunctions::glProgramUniform3ui() 関数によるユニフォーム変数の設定
この関数は、3つの整数値をGLuint型ユニフォーム変数に設定するために使用されます。シェーダープログラムでユニフォーム変数を使用する前に、この関数を使って値を設定する必要があります。QOpenGLExtraFunctions::glProgramUniform3ui() の概要:
Qt GUI で複雑な変形を効率的に適用する方法: QTransform::operator/=() の仕組みと応用例
Qt GUI における QTransform::operator/=() は、2D 変換行列を別の行列で除算する演算子です。これは、スケーリング、回転、移動などの操作を組み合わせた複雑な変形を効率的に適用するために使用されます。演算子の概要
Qt Widgets: QTreeWidgetItem::flags() 関数とは?
QTreeWidgetItem::flags() は、Qt Widgets モジュールの QTreeWidgetItem クラスに属する関数です。この関数は、ツリーウィジェットアイテムのフラグ状態を取得するために使用されます。フラグ状態は、アイテムのさまざまなプロパティを制御します。例えば、編集可能かどうか、選択可能かどうか、チェックボックスを表示するかどうかなどを設定できます。
Qt Widgets QTabBar::tabBarClicked()シグナルでドラッグドロップによるタブ移動
概要QTabBar::tabBarClicked()シグナルは、ユーザーがタブバー上のタブをクリックしたときに発生します。このシグナルは、タブバー内のタブの選択を切り替えたり、タブに関連するアクションを実行したりするために使用できます。シグナルの引数
QStateMachineを使用してQWidget::focusPolicyを動的に変更する方法
Qt Widgetsは、GUIアプリケーション開発のためのC++クラスライブラリです。QWidget::focusPolicyは、ウィジェットがキーボードフォーカスを受け取ることができるかどうかを制御する重要なプロパティです。この記事では、QWidget::focusPolicyの詳細な解説と、プログラミングにおける使用方法について説明します。