Qt Widgets: 複雑なテーブルビュー操作も楽々 - QTableView::verticalOffset() を駆使する
QTableView::verticalOffset() in Qt Widgets: A Comprehensive Explanation
In the realm of Qt programming, QTableView
is a versatile widget that enables the presentation and manipulation of tabular data. It serves as a cornerstone for building interactive data grids and tables within Qt applications. Among its extensive repertoire of functionalities, the verticalOffset()
method plays a crucial role in managing the vertical positioning of table rows.
Understanding verticalOffset()
The verticalOffset()
method, as part of the QTableView
class, retrieves the current vertical offset of the table view's contents. This offset represents the displacement of the displayed rows relative to the topmost row of the table. In simpler terms, it indicates how far the table has been scrolled vertically.
Significance of verticalOffset()
The verticalOffset()
method holds immense significance in various aspects of table view management:
-
Scrolling Control: By accessing the current vertical offset, you can effectively control the scrolling behavior of the table view. This allows you to programmatically scroll the table to specific rows or positions.
-
Row Positioning: Utilizing the
verticalOffset()
value, you can determine the exact location of any visible row within the table view. This enables precise row manipulation and selection. -
Viewport Management: The
verticalOffset()
information proves invaluable when managing the viewport, the visible portion of the table. By adjusting the offset, you can bring specific rows into view or hide them from sight.
Practical Application of verticalOffset()
To illustrate the practical application of verticalOffset()
, consider the following scenario:
Imagine you have a QTableView
displaying a large dataset of customer records. You want to programmatically scroll the table to display the record corresponding to customer ID 12345.
To achieve this, you can follow these steps:
-
Retrieve the customer ID of the currently selected row using the
selectedIndexes()
method. -
Compare the retrieved customer ID with 12345.
-
If the IDs match, call the
verticalOffset()
method to determine the vertical offset required to bring the row corresponding to customer ID 12345 into view. -
Utilize the
scrollTo()
method of theQTableView
object to adjust the vertical scroll position to the calculated offset.
Conclusion
The verticalOffset()
method stands as a cornerstone of effective table view management in Qt applications. By understanding its purpose and applications, you can enhance your ability to control the scrolling behavior, row positioning, and viewport management within your table views.
I hope this comprehensive explanation has provided you with a clear understanding of QTableView::verticalOffset()
and its significance in Qt Widgets programming. Feel free to ask if you have any further questions.
Sample Code Examples for QTableView::verticalOffset()
Scenario 1: Scrolling to a Specific Row
In this scenario, we want to programmatically scroll the table view to display the row corresponding to customer ID 12345.
#include <QTableView>
#include <QModelIndex>
// ... (assume you have a QTableView object named 'tableView' and a QModelIndex object representing the row with customer ID 12345)
// Retrieve the vertical offset required to bring the row into view
int requiredOffset = tableView.verticalOffset() + modelIndex.row() * tableView.rowHeight();
// Scroll the table view to the calculated offset
tableView.scrollTo(0, requiredOffset);
Scenario 2: Determining the Position of a Visible Row
In this scenario, we want to determine the vertical offset of the currently selected row within the table view.
#include <QTableView>
#include <QModelIndex>
// ... (assume you have a QTableView object named 'tableView' and a QModelIndex object representing the currently selected row)
// Retrieve the vertical offset of the selected row
int rowOffset = tableView.verticalOffset() + modelIndex.row() * tableView.rowHeight();
// Use the rowOffset value for further processing
// ...
Scenario 3: Managing Viewport Visibility
In this scenario, we want to programmatically bring a specific row into view, ensuring it is visible within the table view's viewport.
#include <QTableView>
#include <QModelIndex>
// ... (assume you have a QTableView object named 'tableView' and a QModelIndex object representing the row you want to bring into view)
// Calculate the vertical offset required to bring the row into view
int requiredOffset = tableView.verticalOffset() + modelIndex.row() * tableView.rowHeight();
// Adjust the vertical scroll position to ensure the row is visible
if (requiredOffset < tableView.verticalOffset()) {
tableView.scrollTo(0, requiredOffset);
} else if (requiredOffset + tableView.rowHeight() > tableView.verticalOffset() + tableView.viewportHeight()) {
tableView.scrollTo(0, requiredOffset - tableView.viewportHeight() + tableView.rowHeight());
}
These code snippets provide a foundation for utilizing QTableView::verticalOffset()
in various practical scenarios. You can adapt and extend these examples to suit your specific requirements and table view management tasks.
Remember:
- Replace
tableView
andmodelIndex
with the actual objects representing your table view and row selection. - Adjust the code to fit your data structures and table view configuration.
I hope these additional code examples further enhance your understanding of QTableView::verticalOffset()
and its practical applications. Feel free to ask if you have any specific modifications or scenarios in mind.
Utilizing scrollTo() with Row Index:
Instead of calculating the required offset manually, you can directly leverage the scrollTo()
method of QTableView
to scroll to a specific row based on its index.
#include <QTableView>
#include <QModelIndex>
// ... (assume you have a QTableView object named 'tableView' and a QModelIndex object representing the row you want to scroll to)
// Scroll to the row using its index
tableView.scrollTo(modelIndex.row(), 0);
Employing rowAt() for Row Position:
To determine the vertical offset of a visible row, you can utilize the rowAt()
method in conjunction with verticalOffset()
.
#include <QTableView>
#include <QModelIndex>
// ... (assume you have a QTableView object named 'tableView' and a QModelIndex object representing the row you want to find the offset for)
// Calculate the vertical offset using rowAt() and verticalOffset()
int rowOffset = tableView.verticalOffset() + tableView.rowAt(modelIndex.row()) * tableView.rowHeight();
// Use the rowOffset value for further processing
// ...
Leveraging indexAt() for Offset-Based Row Identification:
Given a vertical offset, you can identify the corresponding row using the indexAt()
method.
#include <QTableView>
// ... (assume you have a QTableView object named 'tableView' and a vertical offset value)
// Retrieve the row index corresponding to the offset
QModelIndex rowIndex = tableView.indexAt(offset, 0);
// Use the rowIndex object for further processing
// ...
Combining verticalOffset() with Geometry Information:
To manage viewport visibility, you can combine verticalOffset()
with geometric information like viewport height and row height.
#include <QTableView>
// ... (assume you have a QTableView object named 'tableView', a vertical offset value, and a row index)
// Calculate the top and bottom positions of the row in the viewport
int rowTop = tableView.verticalOffset() + rowIndex.row() * tableView.rowHeight();
int rowBottom = rowTop + tableView.rowHeight();
// Adjust the vertical scroll position based on row visibility
if (rowTop < tableView.verticalOffset()) {
tableView.scrollTo(0, rowTop);
} else if (rowBottom > tableView.verticalOffset() + tableView.viewportHeight()) {
tableView.scrollTo(0, rowBottom - tableView.viewportHeight());
}
These alternative approaches provide additional techniques for utilizing QTableView::verticalOffset()
in your Qt applications. The specific approach you choose will depend on the context and requirements of your task.
Key Takeaways:
scrollTo()
offers a direct method for scrolling to a specific row based on its index.rowAt()
enables determining the vertical offset of a visible row.indexAt()
allows identifying the row corresponding to a given vertical offset.- Combining
verticalOffset()
with geometric information provides fine-grained control over viewport visibility.
I hope these alternative methods further expand your understanding of QTableView::verticalOffset()
and its versatility in Qt programming. Feel free to ask if you have any specific use cases or scenarios in mind.
Qt GUI アプリケーションにおける QWindow::surfaceType() 関数の詳細解説
QWindow::surfaceType() 関数は、Qt GUI アプリケーションでウィンドウの描画に使用されるサーフェスタイプを取得します。これは、ウィンドウのレンダリング方法を決定する重要なプロパティです。この関数は次の情報を提供します:
逆変換でQt GUIの2Dグラフィックスを自在に操る:QTransform::adjoint()徹底解説
概要:QTransformクラスは、2D座標系の変換を表すためのクラスです。adjoint()は、QTransformオブジェクトの逆行列の転置行列を計算します。逆行列の転置行列は、逆変換を行うために使用されます。逆変換は、元の座標系に戻すための操作です。
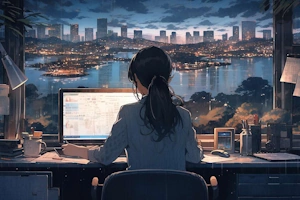
Qt GUIにおけるQWindow::wheelEvent()関数とは?
QWindow::wheelEvent()は、Qt GUIフレームワークにおいて、マウスホイールイベントを処理するための重要な関数です。この関数は、ウィジェットにマウスホイールイベントが送信された際に呼び出され、ユーザーがホイールを回転させた方向や回転量に基づいて、ウィジェットの動作を制御することができます。

Qt GUIにおけるQVulkanInstance::removeDebugOutputFilter()解説
QVulkanInstance::removeDebugOutputFilter()は、Vulkanデバッグ出力のフィルタリング機能を無効にするためのQt GUIクラスの関数です。詳細機能: デバッグ出力フィルタは、Vulkan APIからのデバッグメッセージをフィルタリングする機能を提供します。 特定のメッセージレベルやカテゴリのメッセージを出力しないように設定できます。
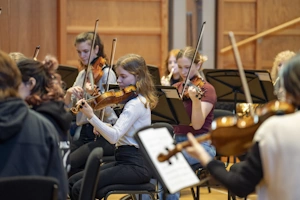
Qt GUIにおけるQTextDocument::setSuperScriptBaseline()徹底解説
QTextDocument::setSuperScriptBaseline() は、Qt GUI ライブラリにおけるテキスト描画機能の一つで、上付き文字のベースラインを設定するための関数です。上付き文字は、通常の文字よりも小さく、文字の上部に配置されます。この関数は、上付き文字のベースラインを、通常の文字のベースラインとは異なる位置に設定することで、上付き文字の位置をより細かく調整することができます。
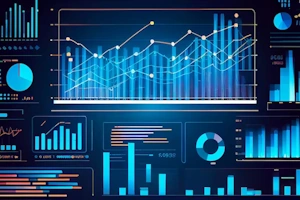
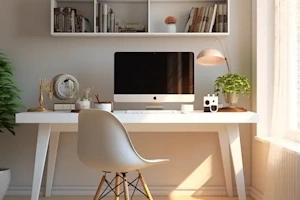
QPlainTextEdit::cut()のサンプルコード
QPlainTextEdit::cut()は、Qt Widgetsライブラリで提供されるプレーンテキスト編集ウィジェットQPlainTextEditのメソッドです。このメソッドは、現在選択されているテキストを切り取り、クリップボードにコピーし、テキストエディタから削除します。選択されたテキストがない場合は、何も起こりません。
Qt Widgetsでウィザードの前のページへ移動する方法:QWizard::back()とその他の方法
QWizard::back()を使用するには、以下の2つの方法があります。シグナルとスロットQWizardクラスには、backRequested()というシグナルが提供されています。このシグナルは、ユーザーが「戻る」ボタンを押下した際に送信されます。このシグナルにスロットを接続することで、ユーザーが「戻る」ボタンを押下した際に処理を実行することができます。
QRasterPaintEngine::drawStaticTextItem() 以外のテキスト描画方法
QRasterPaintEngine::drawStaticTextItem() は、Qt GUI フレームワークにおいて、静的なテキストアイテムを描画するために使用される重要な関数です。この関数は、テキスト文字列、フォント、色、その他の属性を指定することで、高品質なテキストレンダリングを実現します。
Qt GUI で OpenGL 対応サーフェスを判定する方法:QSurface::supportsOpenGL() 関数 vs その他の方法
QSurface::supportsOpenGL() は、Qt GUI モジュールで提供される関数です。この関数は、指定されたサーフェスが OpenGL に対応しているかどうかを判定します。詳細Qt では、ウィンドウやオフスクリーンサーフェスなど、さまざまな種類のレンダリングサーフェスをサポートしています。これらのサーフェスは、それぞれ異なるレンダリングエンジンを使用します。
Qt Widgets: QProgressDialog::autoReset プロパティを使いこなして、プログレスダイアログをレベルアップ!
デフォルト値: false以下のコード例のように、setAutoReset() メソッドを使って設定できます。このコード例では、autoReset プロパティが true に設定されているため、setValue() メソッドで設定された値が最大値に達すると、自動的にリセットされ、プログレスバーは0から再スタートします。