DjangoのテストクライアントにおけるJSONレスポンスの解析: 従来の方法と test.Response.json() の比較
What is test.Response.json()?
test.Response.json()
is a method added to the Response
object in Django's test client in Django 4.2. It simplifies the process of parsing JSON responses from Django views in unit tests.
How does it work?
When you make a request to a Django view using the test client, the response object contains the JSON data that the view returned. The test.Response.json()
method automatically parses this JSON data into a Python dictionary, making it easier to test and verify the response.
Why use it?
Before test.Response.json()
, you had to manually parse the JSON data from the response object using the json
module. This was more cumbersome and error-prone. test.Response.json()
makes the process more streamlined and less error-prone.
How to use it?
To use test.Response.json()
, simply call the method on the response object. For example:
response = self.client.get('/my-view/')
data = response.json()
self.assertEqual(data['status'], 'success')
self.assertEqual(data['data']['id'], 123)
In this example, data
will be a Python dictionary containing the JSON data from the response. You can then use the dictionary to test and verify the response.
Here's an example of how to use test.Response.json() to test a view that returns a list of products:
class ProductListViewTest(TestCase):
def test_product_list_view(self):
response = self.client.get('/products/')
products = response.json()
self.assertEqual(response.status_code, 200)
self.assertEqual(len(products), 3)
for product in products:
self.assertIn('id', product)
self.assertIn('name', product)
self.assertIn('price', product)
Additional notes:
- The
test.Response.json()
method also supports theheaders
parameter, which allows you to specify the headers to be included when parsing the JSON data. - If the response is not JSON, the
test.Response.json()
method will raise aJSONDecodeError
exception.
I hope this explanation is clear and helpful. Please let me know if you have any other questions.
Testing a view that returns a single product:
class ProductDetailViewTest(TestCase):
def test_product_detail_view(self):
product = Product.objects.create(name='My Product', price=9.99)
response = self.client.get(f'/products/{product.id}/')
product_data = response.json()
self.assertEqual(response.status_code, 200)
self.assertEqual(product_data['id'], product.id)
self.assertEqual(product_data['name'], product.name)
self.assertEqual(product_data['price'], product.price)
Testing a view that creates a new product:
class ProductCreateViewTest(TestCase):
def test_product_create_view(self):
data = {
'name': 'My New Product',
'price': 19.99,
}
response = self.client.post('/products/', data=data)
self.assertEqual(response.status_code, 201)
product = Product.objects.get(id=response.json()['id'])
self.assertEqual(product.name, data['name'])
self.assertEqual(product.price, data['price'])
Testing a view that updates an existing product:
class ProductUpdateViewTest(TestCase):
def test_product_update_view(self):
product = Product.objects.create(name='My Product', price=9.99)
data = {
'name': 'My Updated Product',
'price': 14.99,
}
response = self.client.put(f'/products/{product.id}/', data=data)
self.assertEqual(response.status_code, 200)
product.refresh_from_db()
self.assertEqual(product.name, data['name'])
self.assertEqual(product.price, data['price'])
Testing a view that deletes a product:
class ProductDeleteViewTest(TestCase):
def test_product_delete_view(self):
product = Product.objects.create(name='My Product', price=9.99)
response = self.client.delete(f'/products/{product.id}/')
self.assertEqual(response.status_code, 204)
self.assertFalse(Product.objects.filter(id=product.id).exists())
These are just a few examples of how to use test.Response.json()
to test Django views that return JSON data. The possibilities are endless!
I hope these additional samples are helpful. Please let me know if you have any other questions.
Using the json module:
The traditional approach before test.Response.json()
was to manually parse the JSON data from the response object using the json
module. This involves the following steps:
- Import the
json
module:
import json
- Decode the JSON data from the response object:
json_data = json.loads(response.content)
- Access the data using dictionary-like syntax:
data = json_data['key1']
data = json_data['key2']['nested_key']
Example:
def test_product_list_view(self):
response = self.client.get('/products/')
json_data = json.loads(response.content)
self.assertEqual(response.status_code, 200)
self.assertEqual(len(json_data['products']), 3)
for product in json_data['products']:
self.assertIn('id', product)
self.assertIn('name', product)
self.assertIn('price', product)
Using a third-party library:
There are several third-party libraries available for parsing JSON data in Python, such as simplejson
and ujson
. These libraries may offer performance advantages or additional features compared to the built-in json
module.
Example using simplejson:
import simplejson
def test_product_list_view(self):
response = self.client.get('/products/')
data = simplejson.loads(response.content)
self.assertEqual(response.status_code, 200)
self.assertEqual(len(data['products']), 3)
for product in data['products']:
self.assertIn('id', product)
self.assertIn('name', product)
self.assertIn('price', product)
Considerations:
-
Simplicity:
test.Response.json()
offers the simplest and most straightforward approach, as it directly integrates with the test client and simplifies the process. -
Performance: If you're dealing with large volumes of JSON data, consider using a high-performance JSON parsing library like
ujson
. -
Third-party dependencies: Using a third-party library introduces an additional dependency to your project.
Ultimately, the best choice depends on your specific needs and preferences. If simplicity and ease of use are your priorities, test.Response.json()
is an excellent choice. For performance-critical scenarios or if you have specific requirements for JSON parsing, consider using a third-party library.
I hope this helps!
Django フォーム レンダリング API を使わない方がいい場合
テンプレートベースのレンダリング: フォームは、Django テンプレートエンジンを使用して HTML にレンダリングされます。これにより、フォームの外観と動作を完全にカスタマイズできます。ウィジェット: フォームフィールドは、さまざまなウィジェットを使用してレンダリングされます。各ウィジェットは、特定の種類の入力フィールド (テキスト入力、選択リストなど) をレンダリングします。
FeedBurnerで簡単フィード配信!Djangoとの連携方法
Djangoでフィードを作成するには、以下の手順を行います。django. contrib. syndication モジュールをインポートする。フィードの内容となるモデルを定義する。フィードクラスを作成する。フィードのURLパターンを設定する。
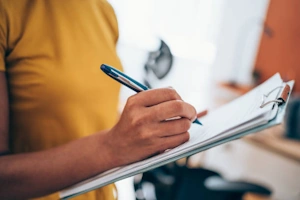
Django フォームのサンプルコード
このガイドでは、以下の内容をより詳細に、分かりやすく解説します。フォームの作成フォームは forms. py ファイルで定義します。ここでは、フォームの各フィールドとその属性を記述します。フィールドの種類 文字列型 (CharField) テキストエリア (TextField) 選択肢 (ChoiceField) チェックボックス (BooleanField) ファイルアップロード (FileField) その他多数
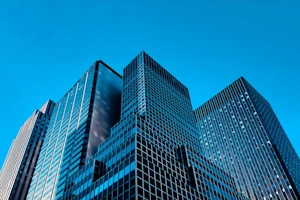
Django クラスベースビューでミックスイン: 効率的な開発のためのガイド
ミックスインは、コードの再利用を目的としたクラスです。共通の機能をまとめることで、コードを冗長化せず、さまざまなクラスに機能を追加することができます。Django では、クラスベースビューを使って、URL と処理を関連付けることができます。クラスベースビューでミックスインを使うには、mixins
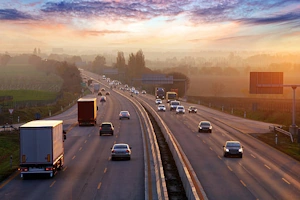
Django で翻訳を使用する:概要と基本
Django の標準的な翻訳フレームワークを使用する: これが最も簡単で一般的な方法です。このフレームワークでは、メッセージを . po ファイルに保存し、Django がそれらを適切な言語に翻訳することを処理します。カスタムソリューションを構築する: 独自の翻訳ソリューションを構築することもできます。これは、より複雑な要件がある場合や、より多くの制御が必要な場合に役立ちます。
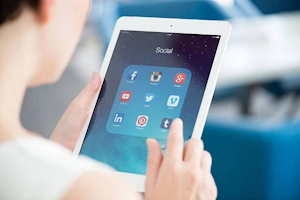
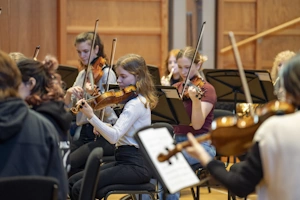
django.db.models.Avg.distinct のサンプルコード
django. db. models. Avg. distinct は、Django ORM でクエリを実行する際に、重複を除いた値の平均値 を計算するための関数です。使い方Avg. distinct は、aggregate() メソッドの引数として使用します。
Django の django.db.models.as_sql() メソッドを徹底解説!
django. db. models. as_sql() メソッドは、Django モデルのクエリを、データベースが実行できる SQL クエリに変換します。これは、クエリがどのように実行されるのかを理解したい場合や、生の SQL クエリを必要とする場合に役立ちます。
django.core.files.File.size 属性の徹底解説
django. core. files. Fileオブジェクトは、アップロードされたファイルやストレージ内のファイルを表すDjangoモデルです。このオブジェクトには、ファイルサイズを取得するためのsize属性があります。属性値size属性は、ファイルのサイズをバイト単位で返します。ファイルがまだメモリに読み込まれていない場合は、ファイルシステムから読み込まれてからサイズが返されます。
Django forms.Form.prefix を使ったフォームセットの表示例
django. forms. forms. Form. prefix は、Django フォームでフォームの名前空間を制御するための属性です。フォームの名前空間とは、フォームのフィールド名やエラーメッセージなどの識別子を生成するために使用されるプレフィックスです。
Django QuerySet.update() メソッドの使い方を理解するための参考資料
django. db. models. query. QuerySet. update() メソッドは、指定された条件に合致するレコードをまとめて更新するために使用されます。これは、個々のレコードをループして更新するよりも効率的で、大量のデータを更新する場合に特に役立ちます。