strip_tags() 関数でHTMLタグを効率的に除去
django.utils.html.strip_tags()
関数は、HTML文字列から指定されたタグを除去し、テキストのみを抽出する強力なツールです。テンプレートやビューで安全かつ効率的にHTMLタグを除去したい場合に役立ちます。
機能
- 指定されたHTMLタグをすべて除去します。
- タグ属性も除去します。
- エンティティはそのまま残します。
- テキストノード間の空白文字を保持します。
- 複数行のテキストを正しく処理します。
使い方
from django.utils.html import strip_tags
# 全てのHTMLタグを除去
text = strip_tags("<p>This is a paragraph.</p>")
# 特定のタグのみを除去
text = strip_tags("<p>This is a paragraph.</p>", ["p"])
# タグ属性も除去
text = strip_tags("<p title='This is a title'>This is a paragraph.</p>")
# エンティティはそのまま残す
text = strip_tags("<p>© This is a copyright notice.</p>")
# テキストノード間の空白文字を保持
text = strip_tags("<p>This is a paragraph. \tThis is another paragraph.</p>")
# 複数行のテキストを正しく処理
text = strip_tags("""
<p>This is a paragraph.
This is another paragraph.</p>
""")
詳細
strip_tags()
関数は、引数としてHTML文字列を受け取ります。- オプション引数として、除去したいタグの名前をリストで渡すことができます。
- 除去したいタグの名前は、小文字で指定する必要があります。
注意点
strip_tags()
関数は、HTMLの構造を壊してしまう可能性があります。- テンプレートで
strip_tags()
関数を使用する場合は、autoescape
を有効にする必要があります。 - エンティティを保持したい場合は、
escape()
関数と組み合わせて使用する必要があります。
補足
strip_tags()
関数は、HTML文字列からテキストのみを抽出したい場合に役立ちます。- テンプレートやビューで安全かつ効率的にHTMLタグを除去したい場合に利用できます。
- ご質問やご不明な点がありましたら、お気軽にお問い合わせください。
Djangoの「utils.html.strip_tags()」関数:サンプルコード
from django.utils.html import strip_tags
html = """
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
"""
text = strip_tags(html)
print(text)
出力:
This is a heading
This is a paragraph.
特定のタグのみを除去
from django.utils.html import strip_tags
html = """
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
"""
text = strip_tags(html, ["h1"])
print(text)
出力:
This is a paragraph.
タグ属性も除去
from django.utils.html import strip_tags
html = """
<p title='This is a title'>This is a paragraph.</p>
"""
text = strip_tags(html)
print(text)
出力:
This is a paragraph.
エンティティはそのまま残す
from django.utils.html import strip_tags
html = """
<p>© This is a copyright notice.</p>
"""
text = strip_tags(html)
print(text)
出力:
© This is a copyright notice.
テキストノード間の空白文字を保持
from django.utils.html import strip_tags
html = """
<p>This is a paragraph. \tThis is another paragraph.</p>
"""
text = strip_tags(html)
print(text)
出力:
This is a paragraph. This is another paragraph.
複数行のテキストを正しく処理
from django.utils.html import strip_tags
html = """
<p>This is a paragraph.
This is another paragraph.</p>
"""
text = strip_tags(html)
print(text)
出力:
This is a paragraph.
This is another paragraph.
テンプレートでの使用例
{% load staticfiles %}
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>Django テンプレート</title>
</head>
<body>
<h1>{{ article.title }}</h1>
<p>{{ article.content|strip_tags }}</p>
</body>
</html>
ビューでの使用例
from django.views.generic import DetailView
class ArticleDetailView(DetailView):
model = Article
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['article_content'] = strip_tags(self.object.content)
return context
DjangoでHTMLタグを除去するその他の方法
- BeautifulSoup は、HTML を解析して操作するための Python ライブラリです。
strip_tags()
関数よりも柔軟で強力な機能を提供します。- 複雑な HTML 構造を処理する場合に役立ちます。
from bs4 import BeautifulSoup
html = """
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
"""
soup = BeautifulSoup(html, "html.parser")
text = soup.get_text()
print(text)
出力:
This is a heading
This is a paragraph.
lxml
- lxml は、XML と HTML を解析するための Python ライブラリです。
- BeautifulSoup よりも高速で効率的な処理が可能です。
- 大量の HTML データを処理する場合に役立ちます。
from lxml import etree
html = """
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
"""
root = etree.fromstring(html)
text = root.text
print(text)
出力:
This is a heading
This is a paragraph.
正規表現
- 正規表現は、テキストパターンを検索して置換するための強力なツールです。
- HTML タグを除去するために使用することができます。
- 複雑なパターン処理に役立ちます。
import re
html = """
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
"""
text = re.sub(r"<.*?>", "", html)
print(text)
出力:
This is a heading
This is a paragraph.
自作関数
- 上記の方法以外にも、自作関数を使って HTML タグを除去することができます。
- 具体的な処理内容を制御したい場合に役立ちます。
def strip_tags(html):
"""
HTMLタグを除去する関数
"""
return re.sub(r"<.*?>", "", html)
html = """
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
"""
text = strip_tags(html)
print(text)
出力:
This is a heading
This is a paragraph.
各方法の比較
方法 | 速度 | 柔軟性 | 複雑性 | 用途 |
---|---|---|---|---|
strip_tags() | 速い | 低い | 低い | 簡単な処理 |
BeautifulSoup | 中程度 | 高い | 中程度 | 複雑な構造 |
lxml | 速い | 中程度 | 中程度 | 大量のデータ |
正規表現 | 遅い | 高い | 高い | 複雑なパターン |
自作関数 | 可変 | 可変 | 高い | 細かい制御 |
最適な方法の選択
- 処理速度を重視する場合は、
strip_tags()
または lxml を使用します。 - 柔軟性と機能性を重視する場合は、BeautifulSoup を使用します。
- 複雑なパターン処理を行う場合は、正規表現または自作関数を使用します。
- 具体的な要件に応じて、最適な方法を選択してください。
Django フォームのサンプルコード
このガイドでは、以下の内容をより詳細に、分かりやすく解説します。フォームの作成フォームは forms. py ファイルで定義します。ここでは、フォームの各フィールドとその属性を記述します。フィールドの種類 文字列型 (CharField) テキストエリア (TextField) 選択肢 (ChoiceField) チェックボックス (BooleanField) ファイルアップロード (FileField) その他多数
Djangoテンプレートで現在の日付を表示する: django.utils.timezone.localdate() とテンプレートタグ
概要django. utils. timezone モジュールは、Djangoにおけるタイムゾーン機能を提供します。localdate() 関数は、現在のタイムゾーンに基づいて、datetime. date 型のオブジェクトを返します。この関数は、Djangoプロジェクトにおける日付処理において、重要な役割を果たします。
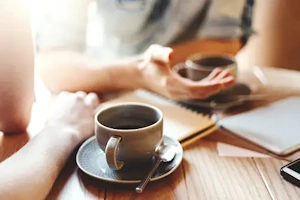
Django の翻訳機能をもっと深く理解したいあなたへ: gettext_noop() 関数の詳細解説
主な用途データベースや API などの外部システムから取得する文字列他のアプリケーションやライブラリで使用される文字列翻訳が不要な定数やメッセージ利点翻訳ファイルのサイズを小さくする翻訳の優先順位を設定するコードの読みやすさを向上させる使用方法
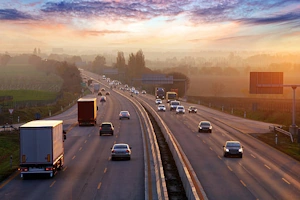
Djangoでエラーをメールで通知する方法 - utils.log.AdminEmailHandler.send_mail()
django. utils. log. AdminEmailHandler. send_mail() は、Django プロジェクトで発生したエラーを、サイト管理者にメールで通知するための関数です。仕組み:ログ記録にエラーが発生すると、AdminEmailHandler が呼び出されます。
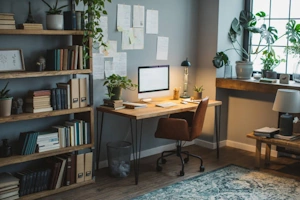
Djangoで複数言語対応を実現する: utils.translation.activate() の使い方
activate() は、以下のような状況で役立ちます。複数言語対応の Web サイトやアプリケーションを開発する場合特定の言語でメールやその他のメッセージを送信する場合テストコードで特定の言語設定を検証する場合activate() の使い方は以下のとおりです。
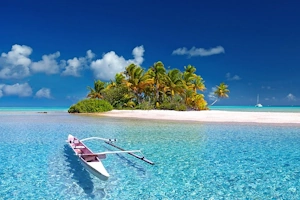
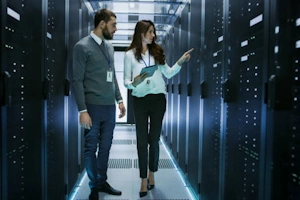
他のアプリケーションとフィールド情報を共有するために文字列に変換する
django. contrib. gis. gdal. Field. as_string() は、GDAL (Geospatial Data Abstraction Library) フィールドオブジェクトを文字列に変換するための関数です。この関数は、GDAL フィールドの属性情報 (名前、型、精度、幅など) を、データベースに保存したり、他のアプリケーションと共有したりするために使用できます。
CreateView の使い方
CreateView は、Django の ジェネリックビュー: [無効な URL を削除しました] の一つで、新しいオブジェクトを作成するためのビューです。具体的には、以下の機能を提供します。フォームを表示:モデルに基づいて自動的にフォームを生成し、HTMLテンプレートで表示します。
django.http.JsonResponse クラス
従来の HttpResponse オブジェクトよりも簡潔で読みやすいコード自動的に JSON エンコードステータスコードとその他の HTTP ヘッダーの設定標準の Django テンプレートエンジンとの統合django. http モジュールをインポート
Django フォームのサンプルコード
このガイドでは、以下の内容をより詳細に、分かりやすく解説します。フォームの作成フォームは forms. py ファイルで定義します。ここでは、フォームの各フィールドとその属性を記述します。フィールドの種類 文字列型 (CharField) テキストエリア (TextField) 選択肢 (ChoiceField) チェックボックス (BooleanField) ファイルアップロード (FileField) その他多数
Djangoのdjango.httpモジュールにおけるhttp.StreamingHttpResponse.reason_phraseの詳細解説
http. StreamingHttpResponse. reason_phrase は、Django フレームワークの django. http モジュールで定義されている属性です。これは、ストリーミング HTTP レスポンスの理由句を表します。