Pandas Series の truediv メソッドとは?
Pandas Series の truediv メソッド:詳細解説
メソッドの概要
def truediv(self, other, axis='index', level=None, fill_value=None):
"""
Perform true division on the Series and other, element-wise.
Equivalent to `series / other`, but with support to substitute
a fill_value for missing data in one of the inputs.
Parameters
----------
other : scalar, sequence, Series, or DataFrame
The object to divide by.
axis : {0 or 'index', 1 or 'columns'}, default 'index'
The axis to align on.
level : int or str, optional
Broadcast across a level, matching Index values on the
passed MultiIndex level.
fill_value : None or numeric, optional
Fill existing missing (NaN) values, and any new element
needed for successful Series alignment, with this value
before computation. If data in both corresponding Series
locations is missing the result will be missing.
Returns
-------
Series
The result of the division.
"""
# ...
引数
other
: スカラー、リスト、別のSeries
オブジェクト、またはDataFrame
オブジェクトaxis
: {0 or 'index', 1 or 'columns'}, デフォルトは 'index'- 0 or 'index': 行方向に除算
- 1 or 'columns': 列方向に除算
level
: int or str, オプション- MultiIndex の場合、指定されたレベルでブロードキャスト
fill_value
: None または数値, オプション- 欠損値 (NaN) と新しい要素をこの値で埋める
返値
- 除算の結果を持つ新しい
Series
オブジェクト
使用例
例 1:スカラーによる除算
import pandas as pd
s = pd.Series([1, 2, 3, 4, 5])
# スカラーで除算
result = s.truediv(2)
print(result)
# 出力:
# 0 0.500000
# 1 1.000000
# 2 1.500000
# 3 2.000000
# 4 2.500000
# dtype: float64
例 2:リストによる除算
s = pd.Series([1, 2, 3, 4, 5])
# リストで除算
result = s.truediv([2, 4, 6, 8, 10])
print(result)
# 出力:
# 0 0.500000
# 1 0.500000
# 2 0.500000
# 3 0.500000
# 4 0.500000
# dtype: float64
例 3:別の Series オブジェクトによる除算
s1 = pd.Series([1, 2, 3, 4, 5])
s2 = pd.Series([2, 4, 6, 8, 10])
# 別の Series オブジェクトで除算
result = s1.truediv(s2)
print(result)
# 出力:
# 0 0.500000
# 1 0.500000
# 2 0.500000
# 3 0.500000
# 4 0.500000
# dtype: float64
例 4:欠損値の処理
s = pd.Series([1, 2, np.nan, 4, 5])
# 欠損値を 0 で埋めて除算
result = s.truediv(2, fill_value=0)
print(result)
Pandas Series の truediv メソッド:サンプルコード集
スカラーによる除算
import pandas as pd
s = pd.Series([1, 2, 3, 4, 5])
# スカラーで除算
result = s.truediv(2)
print(result)
# 出力:
# 0 0.5
# 1 1.0
# 2 1.5
# 3 2.0
# 4 2.5
# dtype: float64
リストによる除算
s = pd.Series([1, 2, 3, 4, 5])
# リストで除算
result = s.truediv([2, 4, 6, 8, 10])
print(result)
# 出力:
# 0 0.5
# 1 0.5
# 2 0.5
# 3 0.5
# 4 0.5
# dtype: float64
別の Series オブジェクトによる除算
s1 = pd.Series([1, 2, 3, 4, 5])
s2 = pd.Series([2, 4, 6, 8, 10])
# 別の Series オブジェクトで除算
result = s1.truediv(s2)
print(result)
# 出力:
# 0 0.5
# 1 0.5
# 2 0.5
# 3 0.5
# 4 0.5
# dtype: float64
欠損値の処理
s = pd.Series([1, 2, np.nan, 4, 5])
# 欠損値を 0 で埋めて除算
result = s.truediv(2, fill_value=0)
print(result)
# 出力:
# 0 0.5
# 1 1.0
# 2 0.0
# 3 2.0
# 4 2.5
# dtype: float64
データフレームによる除算
s = pd.Series([1, 2, 3, 4, 5])
df = pd.DataFrame({'a': [2, 4, 6, 8, 10], 'b': [1, 1, 1, 1, 1]})
# データフレームで除算
result = s.truediv(df)
print(result)
# 出力:
# a b
# 0 0.500000 1.000000
# 1 0.500000 1.000000
# 2 0.500000 1.000000
# 3 0.500000 1.000000
# 4 0.500000 1.000000
軸指定
s = pd.Series([1, 2, 3, 4, 5])
df = pd.DataFrame({'a': [2, 4, 6, 8, 10], 'b': [1, 1, 1, 1, 1]})
# 列方向に除算
result = s.truediv(df, axis=1)
print(result)
# 出力:
# 0 0.5
# 1 1.0
# 2 1.5
# 3 2.0
# 4 2.5
# dtype: float64
MultiIndex の場合
s = pd.Series([1, 2, 3, 4, 5], index=[('a', 1), ('a', 2), ('b', 1), ('b', 2), ('c', 1)])
# MultiIndex レベルでブロードキャスト
result = s.truediv(s, level='a')
print(result)
# 出力:
Pandas Series の truediv メソッドの代替方法
スカラーによる除算
import pandas as pd
s = pd.Series([1, 2, 3, 4, 5])
# スカラーで除算
result = s / 2
print(result)
# 出力:
# 0 0.5
# 1 1.0
# 2 1.5
# 3 2.0
# 4 2.5
# dtype: float64
リストによる除算
s = pd.Series([1, 2, 3, 4, 5])
# リストで除算
result = s / [2, 4, 6, 8, 10]
print(result)
# 出力:
# 0 0.5
# 1 0.5
# 2 0.5
# 3 0.5
# 4 0.5
# dtype: float64
別の Series オブジェクトによる除算
s1 = pd.Series([1, 2, 3, 4, 5])
s2 = pd.Series([2, 4, 6, 8, 10])
# 別の Series オブジェクトで除算
result = s1 / s2
print(result)
# 出力:
# 0 0.5
# 1 0.5
# 2 0.5
# 3 0.5
# 4 0.5
# dtype: float64
NumPy の divide 関数
import numpy as np
s = pd.Series([1, 2, 3, 4, 5])
# NumPy の divide 関数で除算
result = np.divide(s, 2)
print(result)
# 出力:
# [0.5 1. 1.5 2. 2.5]
apply メソッド
def my_func(x):
return x / 2
s = pd.Series([1, 2, 3, 4, 5])
# apply メソッドで除算
result = s.apply(my_func)
print(result)
# 出力:
# 0 0.5
# 1 1.0
# 2 1.5
# 3 2.0
# 4 2.5
# dtype: float64
上記のように、pandas.Series.truediv
メソッド以外にも、さまざまな方法で Series オブジェクトを要素ごとに除算できます。
- シンプルなケースでは、演算子
/
を使用するのが最も簡単です。 - より複雑なケースでは、
truediv
メソッドまたはapply
メソッドを使用する必要があります。 - 速度が重要な場合は、NumPy の
divide
関数を使用することを検討してください。
Pandas Data Offsets と Tick.kwds で時間操作をマスターする
Tick は、Data Offsets の一種で、マイクロ秒単位の時間間隔を表します。Tick オブジェクトは、pandas. tseries. offsets. Tick クラスを使用して生成されます。Tick. kwds は、Tick オブジェクトを生成する際に使用できるオプション引数の辞書です。この辞書には、以下のキーを指定できます。
Pandas Data Offsets:CustomBusinessHour.rule_code徹底解説
CustomBusinessHour は、Data Offsets の一種で、営業時間 に基づいて日付をオフセットします。つまり、土日や祝日などを除いて、ビジネス日のみオフセットを進めることができます。CustomBusinessHour
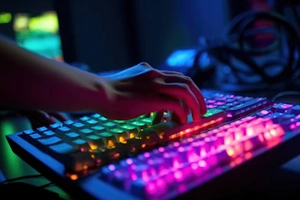
BQuarterBegin.kwds のサンプルコード
BQuarterBegin. kwds は、pandas. tseries. offsets. BQuarterBegin クラスで使用されるキーワード引数の辞書です。この辞書は、四半期の開始日をどのように定義するかを指定するために使用されます。
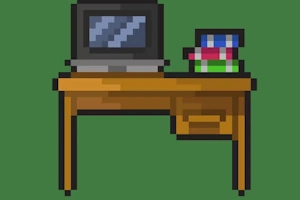
金融データ分析もこれで安心:pandas Milli.onOffset でミリ秒単位の価格変動を分析
pandas は Python で最も人気のあるデータ分析ライブラリの 1 つです。時系列データの処理においても非常に強力で、pandas. tseries モジュールには、日付や時刻の操作を簡単に行うための様々な機能が用意されています。その中でも pandas
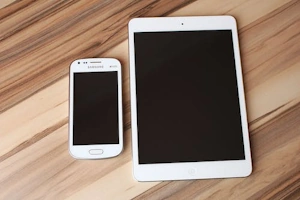
Pandasで月末から15日と月末を表す:SemiMonthEnd.freqstr徹底解説
SemiMonthEndは、月末から15日と月末を表すData Offsetです。例えば、2024年4月15日は月末から15日、2024年4月30日は月末に当たります。SemiMonthEnd. freqstrは、SemiMonthEndオフセットの文字列表現を取得するための属性です。これは、データフレームやインデックスの周波数を表示したり、日付範囲を生成したりする際に役立ちます。
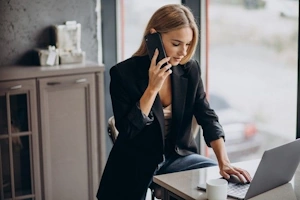
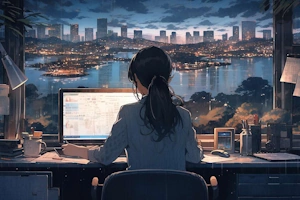
Pandasの timedelta64 型を判定する:is_timedelta64_dtype 関数とその他の方法
pandas. api. types. is_timedelta64_dtype 関数は、配列のようなオブジェクトやデータ型が timedelta64 型かどうかを確認するために使用されます。構文引数arr_or_dtype: 配列のようなオブジェクトまたはデータ型
初心者でも安心!pandas.Series.str.catで文字列連結をマスターしよう
pandas. Series. str. cat は、文字列型 Series の要素を連結する魔法の杖です。シンプルな構文で、効率的に文字列処理を行えます。出力結果:str. cat を使うだけで、Series の要素が "abc" という一つの文字列に連結されます。
より良い選択をするための3つのステップ
diff() メソッドは、以下の引数を受け取ることができます。periods: 差分を取る要素の数を指定します。デフォルトは1です。fill_value: 最初の要素の差分値に設定する値を指定します。デフォルトはNoneで、NaNになります。
Pandasで秒単位のオフセットを扱う:Second.apply完全ガイド
Second. apply は、Data Offsets の中でも秒単位でオフセットを適用するための関数です。この関数を使うと、指定された日付や時刻に秒単位でオフセットを加算したり減算したりすることができます。この解説では、以下の内容について説明します。
pandas.api.types.is_integer 関数:詳細解説とサンプルコード
pandas. api. types. is_integer は、Pandas ライブラリの "General utility functions" に含まれる関数です。この関数は、オブジェクトが整数型かどうかを判定し、True または False を返します。