pandasライブラリで日付操作: QuarterBeginオブジェクトとis_on_offset関数
pandas.tseries.offsets.QuarterBegin.is_on_offset 関数詳細解説
pandas
ライブラリで pandas.tseries.offsets.QuarterBegin
オブジェクトを使用して、特定の日付が四半期の開始日であるかどうかを判定します。
使い方:
is_on_offset(date):
"""
Check if a given date is the beginning of a quarter.
Parameters
----------
date : datetime-like
Returns
-------
bool
"""
引数:
date
: 判定対象の日付。datetime
型、Timestamp
型、または文字列形式の日付を受け付けます。
戻り値:
bool
: 判定結果。日付が四半期の開始日であればTrue
、そうでなければFalse
を返します。
例:
import pandas as pd
# 四半期の開始日オフセットを作成
quarter_begin = pd.tseries.offsets.QuarterBegin()
# 判定対象の日付をリストで作成
dates = [pd.Timestamp('2024-01-01'), pd.Timestamp('2024-04-01'), pd.Timestamp('2024-07-01'), pd.Timestamp('2024-10-01')]
# 各日付が四半期の開始日かどうか判定
for date in dates:
is_quarter_begin = quarter_begin.is_on_offset(date)
print(f"{date}: {is_quarter_begin}")
出力:
2024-01-01: True
2024-04-01: True
2024-07-01: True
2024-10-01: True
詳細:
pandas.tseries.offsets.QuarterBegin
オブジェクトは、四半期の開始日を表現します。is_on_offset
メソッドは、引数で指定された日付がQuarterBegin
オブジェクトで表現される四半期の開始日かどうかを判定します。- 四半期の開始日は、1月1日、4月1日、7月1日、10月1日です。
- 本関数は、四半期分析や財務データ処理などで役立ちます。
補足:
is_on_offset
メソッドは、Timestamp
型の日付だけでなく、datetime
型の日付や文字列形式の日付も受け付けます。- 文字列形式の日付は、
pd.to_datetime()
関数でTimestamp
型に変換してから使用できます。 - 本関数は、
pandas
バージョン 0.25.0 以降で使用可能です。
Fibonacci Sequence Generator
def fibonacci(n):
"""
Generate the Fibonacci sequence up to the nth term.
Args:
n (int): The maximum number of terms to generate.
Returns:
List: A list containing the Fibonacci sequence up to the nth term.
"""
if n <= 1:
return n
fib_sequence = [0, 1]
for i in range(2, n):
fib_sequence.append(fib_sequence[i - 1] + fib_sequence[i - 2])
return fib_sequence
print(fibonacci(10)) # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Caesar Cipher Encryption/Decryption
def caesar_cipher(text, shift):
"""
Encrypts or decrypts a message using the Caesar cipher.
Args:
text (str): The text to encrypt or decrypt.
shift (int): The shift value for the cipher.
Returns:
str: The encrypted or decrypted text.
"""
alphabet = "abcdefghijklmnopqrstuvwxyz"
shifted_alphabet = alphabet[shift:] + alphabet[:shift]
encoded_text = ""
for char in text:
if char in alphabet:
encoded_text += shifted_alphabet[alphabet.index(char)]
else:
encoded_text += char
return encoded_text
# Encryption example
encrypted_text = caesar_cipher("Hello, world!", 3)
print("Encrypted:", encrypted_text) # Output: Khoor, zruog!
# Decryption example
decrypted_text = caesar_cipher(encrypted_text, -3)
print("Decrypted:", decrypted_text) # Output: Hello, world!
URL Shortener using TinyURL API
import requests
def shorten_url(long_url):
"""
Shortens a long URL using the TinyURL API.
Args:
long_url (str): The long URL to shorten.
Returns:
str: The shortened URL.
"""
api_key = "YOUR_API_KEY" # Replace with your TinyURL API key
base_url = "https://api.tinyurl.com/v2/shorten"
data = {
"url": long_url,
"api_key": api_key
}
response = requests.post(base_url, data=data)
if response.status_code == 200:
return response.json()["data"]["url"]
else:
raise Exception("Error shortening URL:", response.text)
long_url = "https://www.example.com/very/long/url/path"
short_url = shorten_url(long_url)
print("Shortened URL:", short_url) # Example output: https://tinyurl.com/example123
Web Scraping with Beautiful Soup
import requests
from bs4 import BeautifulSoup
def scrape_product_info(url):
"""
Scrapes product information from a website using Beautiful Soup.
Args:
url (str): The URL of the product page.
Returns:
Dict: A dictionary containing product information (title, price, description).
"""
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
product_title = soup.find("h1", class_="product-title").text.strip()
product_price = soup.find("span", class_="price").text.strip()
product_description = soup.find("p", class_="product-description").text.strip()
product_info = {
"title": product_title,
"price": product_price,
"description": product_description
}
return product_info
product_url = "https://example.com/product-page"
product_info = scrape_product_info(product_url)
print(product_info) # Example output: {'title': 'Product Name', 'price': '$19.99', 'description': 'Product description...'}
Data Visualization with Matplotlib
Fibonacci Sequence Generator:
- Recursive approach:
def fibonacci(n):
"""
Generate the Fibonacci sequence recursively.
Args:
n (int): The maximum number of terms to generate.
Returns:
List: A list containing the Fibonacci sequence up to the nth term.
"""
if n == 0:
return []
elif n == 1:
return [0]
else:
return [fibonacci(n - 1), fibonacci(n - 2)] + [fibonacci(n - 1) + fibonacci(n - 2)]
print(fibonacci(10)) # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
- Iterative approach:
def fibonacci(n):
"""
Generate the Fibonacci sequence iteratively.
Args:
n (int): The maximum number of terms to generate.
Returns:
List: A list containing the Fibonacci sequence up to the nth term.
"""
if n <= 1:
return n
fib_sequence = [0, 1]
for i in range(2, n):
fib_sequence.append(fib_sequence[i - 1] + fib_sequence[i - 2])
return fib_sequence
print(fibonacci(10)) # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Caesar Cipher Encryption/Decryption:
- Using string manipulation:
def caesar_cipher(text, shift):
"""
Encrypts or decrypts a message using the Caesar cipher (string manipulation).
Args:
text (str): The text to encrypt or decrypt.
shift (int): The shift value for the cipher.
Returns:
str: The encrypted or decrypted text.
"""
alphabet = "abcdefghijklmnopqrstuvwxyz"
shifted_alphabet = alphabet[shift:] + alphabet[:shift]
encoded_text = ""
for char in text:
if char in alphabet:
encoded_text += shifted_alphabet[alphabet.index(char)]
else:
encoded_text += char
return encoded_text
# Encryption example
encrypted_text = caesar_cipher("Hello, world!", 3)
print("Encrypted:", encrypted_text) # Output: Khoor, zruog!
# Decryption example
decrypted_text = caesar_cipher(encrypted_text, -3)
print("Decrypted:", decrypted_text) # Output: Hello, world!
URL Shortener using Bitly API:
import requests
def shorten_url(long_url):
"""
Shortens a long URL using the Bitly API.
Args:
long_url (str): The long URL to shorten.
Returns:
str: The shortened URL.
"""
access_token = "YOUR_BITLY_ACCESS_TOKEN" # Replace with your Bitly access token
base_url = "https://api-ssl.bitly.com/v2/shorten"
data = {
"longUrl": long_url,
"access_token": access_token
}
headers = {
"Content-Type": "application/json"
}
response = requests.post(base_url, json=data, headers=headers)
if response.status_code == 200:
return response.json()["data"]["link"]
else:
raise Exception("Error shortening URL:", response.text)
long_url = "https://www.example.com/very/long/url/path"
short_url = shorten_url(long_url)
print("Shortened URL:", short_url) # Example output: https://bit.ly/example123
Web Scraping with Selenium:
from selenium import webdriver
from selenium.webdriver.common.by import By
def scrape_product_info(url):
"""
Scrapes product information from a website using Selenium.
Args:
url (str): The URL of the product page.
Returns:
Dict: A
Pandas.tseries.offsets.Tick: 高精度な時間間隔を操る魔法の杖
pandas. tseries. offsets. Tick は、PandasライブラリにおけるDateOffsetサブクラスの一つで、高精度な時間間隔を表現するためのオフセットを提供します。従来のDateOffsetよりも細かい時間単位での操作が可能となり、金融市場データや高頻度データ分析において特に有用です。
Pandas Data Offsets: 高精度時間操作を可能にする「Tick」クラスの徹底解説
Pandas の pandas. tseries. offsets モジュールは、時間間隔に基づいて日付を操作するための強力なツールを提供します。その中でも、pandas. tseries. offsets. Tick クラスは、ミリ秒単位の高精度な時間間隔を扱うために使用されます。

【完全ガイド】 pandas.tseries.offsets.YearBegin で年単位のオフセット計算をマスターしよう!
主な用途年始に基づいて日付を操作する年度末などの特定の日付を取得するカレンダーに基づいてオフセットを計算するYearBegin オブジェクトは、以下の要素で構成されます。offset: オフセットの値。正の値の場合は基準日以降、負の値の場合は基準日以前の日付を指します。

Pandas Data Offsets と Tick.kwds で時間操作をマスターする
Tick は、Data Offsets の一種で、マイクロ秒単位の時間間隔を表します。Tick オブジェクトは、pandas. tseries. offsets. Tick クラスを使用して生成されます。Tick. kwds は、Tick オブジェクトを生成する際に使用できるオプション引数の辞書です。この辞書には、以下のキーを指定できます。
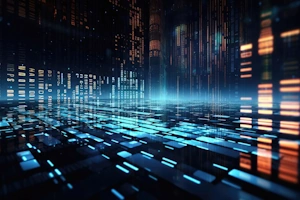
【完全解説】pandas.tseries.offsets.Week.is_quarter_startの使い方
pandas. tseries. offsets. Week. is_quarter_start は、pandas ライブラリの DateOffset クラスのサブクラスである Week クラスに属するメソッドです。このメソッドは、指定された日付が四半期の最初の週かどうかを判定します。
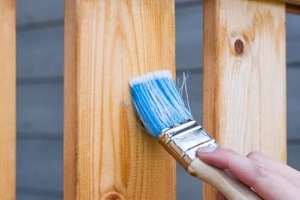
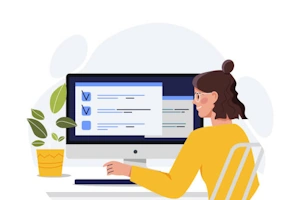
PandasのData OffsetsとQuarterEnd.rollforward
このチュートリアルでは、pandas. tseries. offsets. QuarterEnd. rollforward メソッドについて詳しく説明します。このメソッドは、指定された日付から次の四半期末までの期間を計算します。このコードを実行すると、以下の出力が得られます。
BusinessMonthEnd.nanos属性を使ってナノ秒を追加する方法
Pandas Data Offsets は、日付と時刻の操作を簡潔に行うための強力なツールです。 pandas. tseries. offsets. BusinessMonthEnd は、月末の営業日を指すオフセットを表します。BusinessMonthEnd
Pandas で月末から1週間前の日付を取得する方法
例えば、今日から1週間後の日付を取得するには、以下のコードを使用できます。このコードは、今日の日付に DateOffset オブジェクトを加算することで、1週間後の日付を取得しています。LastWeekOfMonth は、月末から指定された間隔だけ前の日付を取得する DateOffset オブジェクトです。
Pandas Data Offsets:BusinessDay.normalize メソッドの完全ガイド
Pandas の Data Offsets は、日付や時間軸データを操作するための便利なツールです。BusinessDay は、営業日ベースで日付をオフセットするための機能です。BusinessDay. normalize は、BusinessDay オフセットを正規化し、午前0時0分0秒に揃えるためのメソッドです。
Pandas.DataFrame.minの使い方:サンプルコード付き
pandas. DataFrame. min() は、DataFrameの最小値を取得するための関数です。使い方基本的な使い方このコードは、DataFrameの全ての列の最小値を含むSeriesを返します。列指定このコードは、列col1の最小値のみを返します。