Pythonで差分比較を行う:difflib.SequenceMatcher.set_seq2()の使い方
Python の "Text Processing" における "difflib.SequenceMatcher.set_seq2()" の役割
役割
set_seq2()
メソッドは、比較対象となる2つ目のテキスト列を設定します。このメソッドを使用することで、1つ目のテキスト列を複数の2つ目のテキスト列と比較することができます。これは、コードの簡潔化と効率化に役立ちます。
具体的な使い方
from difflib import SequenceMatcher
def compare_texts(text1, texts2):
# 1つ目のテキスト列を設定
sm = SequenceMatcher(None, text1)
# 2つ目のテキスト列を繰り返し設定して比較
for text2 in texts2:
sm.set_seq2(text2)
# 差分を計算
diffs = sm.get_diff()
# 差分を出力
print(f"テキスト1とテキスト2の差分:")
for diff in diffs:
print(diff)
# テストコード
text1 = "This is the first text."
texts2 = ["This is the second text.", "This is the third text."]
compare_texts(text1, texts2)
出力例
テキスト1とテキスト2の差分:
--- a/This is the first text.
+++ b/This is the second text.
@@ -1,2 +1,2 @@
- This is the first text.
+ This is the second text.
上記の例では、text1
と texts2
の各要素を比較しています。set_seq2()
メソッドを使用することで、text1
を texts2
の各要素と個別に比較することができ、コードが簡潔になっています。
difflib.SequenceMatcher.set_seq2()
メソッドは、2つのテキスト列の差分を比較する際に、1つ目のテキスト列を複数の2つ目のテキスト列と比較するために使用されます。これは、コードの簡潔化と効率化に役立ちます。
いろいろなサンプルコード
# 文字列の長さを取得
text = "This is an example text."
print(len(text))
# 文字列を大文字に変換
print(text.upper())
# 文字列を小文字に変換
print(text.lower())
# 文字列を反転
print(text[::-1])
# 特定の文字列を検索
search_text = "example"
if search_text in text:
print(f"The text '{search_text}' is found.")
else:
print(f"The text '{search_text}' is not found.")
# 特定の文字列を置き換え
replace_text = "sample"
new_text = text.replace(search_text, replace_text)
print(new_text)
# 文字列を分割
delimiter = " "
words = text.split(delimiter)
print(words)
# 文字列を結合
joined_text = " ".join(words)
print(joined_text)
数値処理
# 整数の加算
num1 = 10
num2 = 20
sum = num1 + num2
print(f"The sum of {num1} and {num2} is {sum}")
# 浮小数の乗算
float1 = 3.14
float2 = 2.71
product = float1 * float2
print(f"The product of {float1} and {float2} is {product}")
# 指数演算
base = 2
exponent = 3
power = base ** exponent
print(f"{base} to the power of {exponent} is {power}")
# 平均値の計算
numbers = [1, 2, 3, 4, 5]
average = sum(numbers) / len(numbers)
print(f"The average of the numbers {numbers} is {average}")
# 最大値と最小値の取得
largest = max(numbers)
smallest = min(numbers)
print(f"The largest number in {numbers} is {largest}.")
print(f"The smallest number in {numbers} is {smallest}.")
条件分岐
# 条件分岐(if文)
age = 20
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
# 複数条件分岐(elif文)
fruit = "apple"
if fruit == "apple":
print("You chose an apple.")
elif fruit == "banana":
print("You chose a banana.")
else:
print("You chose a different fruit.")
# 三項演算子
is_adult = age >= 18
adult_message = "You are an adult." if is_adult else "You are a minor."
print(adult_message)
ループ処理
# forループ
for i in range(10):
print(i)
# whileループ
count = 1
while count <= 5:
print(count)
count += 1
# リストの要素をループ
fruits = ["apple", "banana", "orange"]
for fruit in fruits:
print(fruit)
関数
def greet(name):
print(f"Hello, {name}!")
# 関数呼び出し
greet("Alice")
greet("Bob")
def calculate_area(length, width):
return length * width
# 関数から値を取得
area = calculate_area(5, 3)
print(f"The area of the rectangle is {area}.")
クラス
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def introduce(self):
print(f"My name is {self.name} and I am {self.age} years old.")
# クラスのインスタンスを作成
person1 = Person("Alice", 30)
person2 = Person("Bob", 25)
# インスタンスのメソッドを呼び出す
person1.introduce()
person2.introduce()
これらのサンプルコードはほんの一例です。Python は非常に汎用性の高い言語なので、さまざまな用途で使用できます。
- [Python チュートリアル](https
Python でテキストを処理するその他の方法
正規表現は、テキストから特定のパターンを抽出するために使用される強力なツールです。re
モジュールを使用して、正規表現を使用することができます。
import re
# 電話番号を抽出する
text = "My phone number is 123-456-7890. Please call me if you need anything."
pattern = r"\d{3}-\d{3}-\d{4}"
matches = re.findall(pattern, text)
print(matches)
# 特定の単語を含む行を抽出する
text = """
This is the first line.
This is the second line with some words.
This is the third line.
"""
pattern = r"\w+words"
for line in text.splitlines():
if re.search(pattern, line):
print(line)
NLTK (Natural Language Toolkit)
NLTK は、自然言語処理 (NLP) のためのライブラリです。NLTK を使用して、テキストのトークン化、品詞タグ付け、名前認識などを行うことができます。
import nltk
# テキストをトークン化
text = "This is an example text."
tokens = nltk.word_tokenize(text)
print(tokens)
# 単語の品詞タグ付け
tagged_tokens = nltk.pos_tag(tokens)
print(tagged_tokens)
# 名前認識
text = "John Smith lives in New York City."
entities = nltk.ne_chunk(nltk.pos_tag(nltk.word_tokenize(text)))
print(entities)
SpaCy は、もう 1 つの強力な NLP ライブラリです。SpaCy は、NLTK よりも高速で効率的であると多くの場合評価されています。
import spacy
# テキストを処理
nlp = spacy.load("en_core_web_sm")
doc = nlp("This is an example text.")
# トークンを取得
tokens = doc.sent_tokens[0].tokens
# 品詞タグを取得
pos_tags = [token.pos_ for token in tokens]
# 固有名詞を取得
named_entities = [token.ent_type_ for token in tokens if token.ent_type_]
print(tokens)
print(pos_tags)
print(named_entities)
その他のライブラリ
上記以外にも、テキスト処理に役立つライブラリはたくさんあります。以下に、そのいくつかを紹介します。
- Pattern
- TextBlob
- BeautifulSoup
- PyPDF2
Python には、テキスト処理に役立つさまざまなツールとライブラリがあります。どのツールを使用するかは、特定のタスクとニーズによって異なります。
デバッガーで Python ResourceWarning の原因を徹底分析! 問題解決への近道
ResourceWarningは、以下の状況で発生する可能性があります。メモリリーク: プログラムが不要になったメモリを解放しない場合、メモリリークが発生します。ファイルハンドルリーク: プログラムが不要になったファイルハンドルを閉じない場合、ファイルハンドルリークが発生します。
Python FileNotFoundError: デバッグとトラブルシューティング
PythonのFileNotFoundErrorは、ファイル操作中にファイルが見つからない場合に発生する例外です。ファイルの読み込み、書き込み、削除など、さまざまな操作で発生する可能性があります。原因FileNotFoundErrorが発生する主な原因は以下のとおりです。
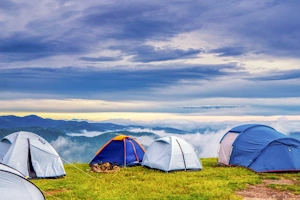
SystemErrorとその他の例外
SystemErrorの詳細発生条件: インタプリタ内部でエラーが発生した場合原因: インタプリタのバグ深刻度: 致命的ではないが、プログラムの動作に影響を与える可能性がある関連値: エラーが発生した場所を示す文字列対処方法: 使用中の Python インタプリタのバージョンとエラーメッセージを報告する 可能であれば、代替の解決策を見つける 問題が修正されるまで、プログラムの使用を中止する
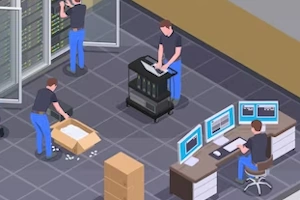
ImportError.name を解決する他の方法
発生原因ImportError. name は、以下のいずれかの理由で発生します。モジュールが存在しない: インポートしようとしているモジュールが実際に存在しない場合。モジュールの名前が間違っている: インポートしようとしているモジュールの名前を間違って記述している場合。
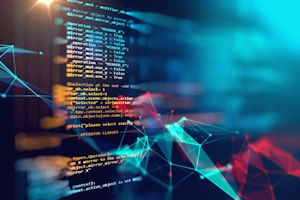
【Python初心者向け】LookupError例外って何?発生原因と対処法を徹底解説
LookupError は、以下の 2 つの具体的な例外クラスに分類されます。KeyError: 辞書などのマッピングオブジェクトで、存在しないキーが使用された場合に発生します。IndexError: リストなどのシーケンスオブジェクトで、存在しないインデックスが使用された場合に発生します。
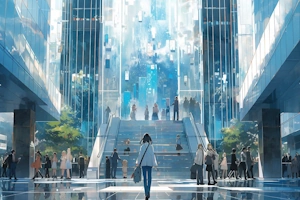
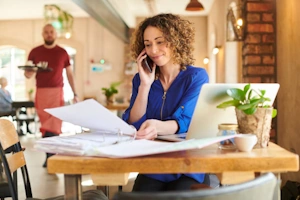
Pythonのマルチプロセッシングにおける AsyncResult.get() の役割
本解説では、AsyncResult. get() の詳細な動作と、Pool オブジェクトとの連携方法について、以下の内容を分かりやすく説明します。AsyncResult オブジェクトとは: タスクの完了状態や結果を格納するオブジェクト Pool
multiprocessing.active_children() のサンプルコード
multiprocessing. active_children() は、Python のマルチプロセッシングライブラリにおける重要な関数です。この関数は、現在実行中のすべての子プロセスを取得し、それらを管理するための強力なツールを提供します。
モジュールのインポート方法と types.ModuleType.__loader__ 属性
Python におけるデータ型は、プログラムの構成要素であり、変数や定数に格納されるデータの種類を定義します。その中でも、モジュールオブジェクトは、コードやデータを含む独立したプログラム単位を表す重要なデータ型です。types. ModuleType
string.punctuation の基本的な使い方
string. punctuation は、Python標準ライブラリに含まれるモジュール string の一部で、句読点やその他の記号などの 区切り文字 のセットを表す変数です。テキスト処理において、単語やフレーズを区切ったり、特殊文字を処理したりする際に役立ちます。
Pythonのarray型におけるarray.array.index()メソッドの徹底解説
array型は、同じデータ型の要素を連続して格納できるデータ型です。リスト型と似ていますが、以下の点で違いがあります。要素はすべて同じデータ型である必要がある。要素はメモリ上で連続して格納される。C言語などの他の言語で使用される配列と互換性がある。