string.punctuation の基本的な使い方
Pythonにおける"string.punctuation"とテキスト処理
string.punctuation
は、Python標準ライブラリに含まれるモジュール string
の一部で、句読点やその他の記号などの 区切り文字 のセットを表す変数です。テキスト処理において、単語やフレーズを区切ったり、特殊文字を処理したりする際に役立ちます。
使い方
string.punctuation
は、文字列として直接使用できます。例えば、以下のコードは、文字列 sentence
から句読点を取り除きます。
import string
sentence = "Hello, world! This is a great day."
# 句読点を取り除く
punctuation = string.punctuation
no_punctuation = "".join(ch for ch in sentence if ch not in punctuation)
print(no_punctuation) # 出力: Hello world This is a great day
応用例
- 単語のリストを作成する
- 特殊文字をエスケープする
- テキストから数字を抽出する
- データをクリーンアップする
例
以下のコードは、上記の応用例をいくつか示しています。
import string
# 単語のリストを作成する
text = "This is a text with punctuation."
words = text.split(string.punctuation)
print(words) # 出力: ['This', 'is', 'a', 'text', 'with', 'punctuation']
# 特殊文字をエスケープする
special_chars = "!@#$%^&*"
text = "This text contains special characters."
escaped_text = "".join(ch.replace("\\", "\\\\") for ch in text if ch in special_chars)
print(escaped_text) # 出力: This text contains special characters\\.
# テキストから数字を抽出する
text = "The price is $19.99."
digits = "".join(ch for ch in text if ch in string.digits)
print(digits) # 出力: 1999
# データをクリーンアップする
text = "Data, such as this, often contains noise."
cleaned_text = "".join(ch.lower() for ch in text if ch.isalnum() or ch in string.whitespace)
print(cleaned_text) # 出力: data such as this often contains noise
補足
string.punctuation
の内容は、ロケールによって異なる場合があります。- 句読点以外の記号も含まれていることに注意してください。
- 特定の記号のみを処理したい場合は、
str.translate()
関数とカスタム翻訳テーブルを使用することができます。
この説明が、Pythonにおける "string.punctuation" とテキスト処理に関する理解を深めるのに役立つことを願っています。
Pythonにおける"string.punctuation"を使った様々なサンプルコード
以下のコードは、句読点のうち、カンマとピリオドのみを削除する例です。
import string
text = "Hello, world! This is a great day. How are you?"
punctuation = string.punctuation
remove_chars = ",." # 削除する句読点
# 特定の句読点のみを削除
no_punctuation = "".join(ch for ch in text if ch not in remove_chars)
print(no_punctuation) # 出力: Hello world This is a great day How are you
文字列内の空白をすべて削除する
以下のコードは、文字列内の空白をすべて削除する例です。
import string
text = " This text has many spaces. "
punctuation = string.punctuation + string.whitespace # 空白も句読点に含める
# 空白を含む句読点すべてを削除
no_whitespace = "".join(ch for ch in text if ch not in punctuation)
print(no_whitespace) # 出力: Thistexthasmanyspaces
URLからクエリパラメータを抽出する
以下のコードは、URLからクエリパラメータを抽出する例です。
import string
url = "https://www.example.com/search?q=python&page=2"
punctuation = string.punctuation + "=" # クエリパラメータの区切り文字
# クエリパラメータ部分を抽出
query_params = url.split("?")[-1]
# 各パラメータをキーと値のペアに分割
params = dict(pair.split("=") for pair in query_params.split("&"))
print(params) # 出力: {'q': 'python', 'page': '2'}
HTMLタグを取り除く
以下のコードは、HTMLタグを取り除く例です。
import string
html = "<p>This is some HTML text with <b>tags</b>.</p>"
punctuation = string.punctuation + "<" + ">" # HTMLタグも句読点に含める
# HTMLタグを含む句読点すべてを削除
no_html = "".join(ch for ch in html if ch not in punctuation)
print(no_html) # 出力: This is some HTML text with tags.
改行コードを変換する
以下のコードは、改行コードを別の文字列に変換する例です。
import string
text = "This is a text\nwith multiple\nlines."
punctuation = string.punctuation + "\n" # 改行コードも句読点に含める
# 改行コードを指定の文字列に置き換える
new_lines = " "
converted_text = "".join(ch.replace("\n", new_lines) for ch in text)
print(converted_text) # 出力: This is a text with multiple lines.
これらの例は、string.punctuation
を様々なテキスト処理タスクに応用する方法を示しています。
上記以外にも、string.punctuation
を利用した様々な処理が可能です。例えば、
- 特殊文字をHTMLエンティティに変換する
- 文字列を大文字または小文字に変換する
- 余計なスペースを削除する
など、様々な用途に活用できます。
ご自身のニーズに合わせて、string.punctuation
を様々な方法で活用してみてください。
"string.punctuation" 以外の代替手段
カスタム文字セット
特定のタスクに必要ない句読点のみを削除したい場合は、string.punctuation
から不要な文字を削除したカスタム文字セットを作成することができます。
import string
# 不要な句読点を削除したカスタム文字セット
custom_punctuation = string.punctuation.replace(",", "").replace(".", "")
# カスタム文字セットを使用して句読点を除去
text = "Hello, world! This is a great day."
no_punctuation = "".join(ch for ch in text if ch not in custom_punctuation)
print(no_punctuation) # 出力: Hello world This is a great day
正規表現
より複雑なパターンで句読点を含む文字列を処理したい場合は、正規表現を使用することができます。
import re
text = "This text has various punctuation: ?!@#$.%"
pattern = re.compile("[%s]" % re.escape(string.punctuation)) # 正規表現で句読点のパターンを定義
# 正規表現を使用して句読点を削除
no_punctuation = pattern.sub("", text)
print(no_punctuation) # 出力: This text has various punctuation
文字列メソッド
特定の文字や文字クラスを処理したい場合は、str
オブジェクトのメソッドを使用することができます。
text = "Hello, world! This is a great day."
# 特定の文字を削除
no_commas = text.replace(",", "")
print(no_commas) # 出力: Hello world! This is a great day
# 文字クラスを使用して空白を削除
no_whitespace = text.translate(str.maketrans("", "", string.whitespace))
print(no_whitespace) # 出力: Hello,world!Thisisalgreatday
サードライブラリ
より高度なテキスト処理機能が必要な場合は、pandas
や NLTK
などのサードライブラリを使用することができます。
これらのライブラリは、正規表現や統計分析などの高度なツールを提供しており、複雑なテキスト処理タスクを効率的に処理することができます。
string.punctuation
は、シンプルなテキスト処理タスクに適していますが、より複雑な処理には str
メソッド、正規表現、カスタム文字セット、サードライブラリなどの代替手段を検討する必要があります。
それぞれの方法の長所と短所を理解し、状況に応じて適切な方法を選択することが重要です。
SystemErrorとその他の例外
SystemErrorの詳細発生条件: インタプリタ内部でエラーが発生した場合原因: インタプリタのバグ深刻度: 致命的ではないが、プログラムの動作に影響を与える可能性がある関連値: エラーが発生した場所を示す文字列対処方法: 使用中の Python インタプリタのバージョンとエラーメッセージを報告する 可能であれば、代替の解決策を見つける 問題が修正されるまで、プログラムの使用を中止する
スレッドのネイティブIDを取得: Pythonにおける「thread.get_native_id()」
thread. get_native_id() は、Python の threading モジュールで提供される関数で、現在のスレッドのネイティブIDを取得するために使用されます。ネイティブIDは、オペレーティングシステムによって割り当てられるスレッドの一意な識別番号です。
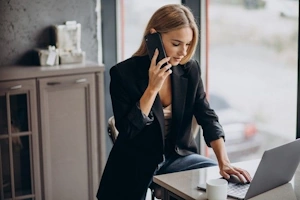
Pythonの同時実行におけるsubprocess.Popen.stderrの詳細解説
Pythonの subprocess モジュールは、外部コマンドをサブプロセスとして実行するための強力なツールです。Popen クラスは、サブプロセスの起動、入出力の制御、終了ステータスの取得などを可能にします。この解説では、Popen クラスの stderr 属性に焦点を当て、同時実行における役割と使用方法について詳しく説明します。

Pythonのthread.lock.release()を使いこなして、安定性の高いマルチスレッドプログラムを作成
**thread. lock. release()**は、スレッドがロックを解放するための関数です。ロックの必要性複数のスレッドが同じ共有リソースにアクセスする場合、データ競合と呼ばれる問題が発生する可能性があります。データ競合とは、複数のスレッドが同時に同じデータを変更しようとすることで、データの整合性が失われる状態を指します。

Pythonで並行処理をマスター!スレッド、マルチプロセス、非同期プログラミングの比較
Concurrent Execution において、thread. get_ident() は以下の用途で使用されます。1. スレッドの識別:複数のスレッドが同時に実行されている場合、thread. get_ident() を使用して個々のスレッドを区別することができます。これは、ログ記録やデバッグを行う際に役立ちます。
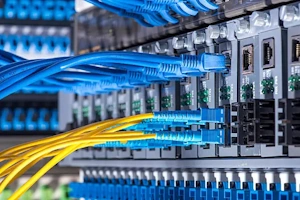
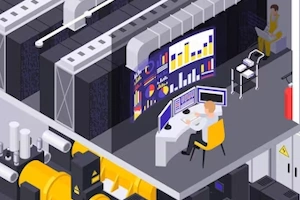
Python Text Processing における readline.read_init_file() 関数
read_init_file() 関数 は、readline モジュールが提供する関数の一つで、初期化ファイルを読み込み、設定を適用するために使用されます。この関数は、以下の役割を果たします。指定された初期化ファイルを読み込み、設定を解析します。
RLock、Semaphore、BoundedSemaphore、Conditionを使いこなしてスレッドを制御しよう!
Pythonのマルチスレッドプログラミングにおいて、thread. LockTypeは共有リソースへのアクセスを制御し、データ競合を防ぐための重要なツールです。この解説では、thread. LockTypeの仕組みと、さまざまな種類のロックオブジェクトの使い方を、分かりやすく例を交えて説明します。
複雑な並行処理をシンプルに! contextvars モジュールによるコンテキスト管理
スレッドローカルな状態をより簡単に管理できるcontextvarsモジュールでは、コンテキスト変数を定義し、そのスコープ内でアクセスすることができます。従来のthreading. localモジュールでは、スレッドローカルな属性を直接アクセスする必要がありましたが、contextvarsモジュールでは、より自然な構文でコンテキスト変数を扱えます。
Pythonでタイムゾーン情報を扱うベストプラクティス
Pythonのdatetimeモジュールは、日付と時刻を扱うための標準ライブラリです。このモジュールには、タイムゾーン情報を扱うためのzoneinfoサブモジュールも含まれています。ZoneInfoは、世界中のタイムゾーンに関する情報を含むデータベースです。このデータベースは、IANA (Internet Assigned Numbers Authority) によって管理されています。
Pythonの「Concurrent Execution」における「threading.Barrier」の徹底解説
Pythonの「threading. Barrier」は、マルチスレッドプログラミングにおいて、複数のスレッドが特定のポイントに到達するまで待機させるための同期オブジェクトです。この解説では、「threading. Barrier. broken」属性に焦点を当て、以下の内容を分かりやすく説明します。