Python テキスト処理:re.Match.groups() とその他のグループ化機能の比較
Python テキスト処理:re.Match.groups() の詳細解説
re.Match.groups()
は Python のテキスト処理モジュール re
で使用される関数で、正規表現パターンにマッチした部分文字列を取得します。
使い方
re.Match.groups()
は、re.match()
や re.search()
などの正規表現検索関数によって返される Match
オブジェクトに対して呼び出します。
import re
text = "This is a string with a number 1234 and a word Python."
# 正規表現パターン
pattern = r"\d+|\w+"
# Matchオブジェクトを取得
match = re.search(pattern, text)
# マッチした部分文字列をグループとして取得
groups = match.groups()
# 結果を出力
print(groups)
このコードは、以下の結果を出力します。
('1234', 'Python')
グループ
正規表現パターン内で括弧 ()
を使用すると、その部分文字列がグループとして抽出されます。
pattern = r"(\d+) (\w+)"
match = re.search(pattern, text)
groups = match.groups()
print(groups)
このコードは、以下の結果を出力します。
('1234', 'Python')
詳細
re.Match.groups()
は、マッチした部分文字列をタプルとして返します。- グループ番号を指定することで、特定のグループの文字列を取得できます。
- グループ名を使用している場合は、グループ名で文字列を取得できます。
- デフォルトでは、すべてのグループが返されます。
- 空のグループは、空の文字列
''
として返されます。
例
- 特定のグループの文字列を取得する
group_number = 1
# グループ番号を指定して文字列を取得
group_string = match.group(group_number)
print(group_string)
- グループ名で文字列を取得する
group_name = "number"
# グループ名で文字列を取得
group_string = match.group(group_name)
print(group_string)
- 空のグループ
pattern = r"(a)(\d+)(b)"
text = "ab"
match = re.search(pattern, text)
groups = match.groups()
print(groups)
このコードは、以下の結果を出力します。
('', '', '')
re.Match.groups()
は、テキスト処理において非常に便利な関数です。- 正規表現パターンを理解することで、より複雑なテキスト処理を行うことができます。
- 上記の例を参考に、さまざまなテキスト処理を試してみてください。
Python テキスト処理:re.Match.groups() サンプルコード集
import re
text = "This is a string with a number 1234 and a word Python."
# 数字と文字列を抽出するパターン
pattern = r"\d+|\w+"
# Matchオブジェクトを取得
match = re.search(pattern, text)
# マッチした部分文字列をグループとして取得
groups = match.groups()
# 結果を出力
print(groups)
出力:
('1234', 'Python')
メールアドレスを抽出する
import re
text = "My email address is [email protected] and yours is [email protected]."
# メールアドレス抽出パターン
pattern = r"[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+"
# Matchオブジェクトを取得
match = re.search(pattern, text)
# マッチした部分文字列をグループとして取得
groups = match.groups()
# 結果を出力
print(groups)
出力:
('[email protected]', '[email protected]')
URLを抽出する
import re
text = "This website has a URL https://www.example.com and another https://google.com."
# URL抽出パターン
pattern = r"https?://[a-zA-Z0-9-_.]+\.[a-zA-Z0-9-_.]+"
# Matchオブジェクトを取得
match = re.search(pattern, text)
# マッチした部分文字列をグループとして取得
groups = match.groups()
# 結果を出力
print(groups)
出力:
('https://www.example.com', 'https://google.com')
日付を抽出する
import re
text = "The meeting will be held on 2023-12-25 and the deadline is 2024-01-01."
# 日付抽出パターン
pattern = r"\d{4}-\d{1,2}-\d{1,2}"
# Matchオブジェクトを取得
match = re.search(pattern, text)
# マッチした部分文字列をグループとして取得
groups = match.groups()
# 結果を出力
print(groups)
出力:
('2023-12-25', '2024-01-01')
時間を抽出する
import re
text = "The meeting starts at 10:00 AM and ends at 3:00 PM."
# 時間抽出パターン
pattern = r"\d{1,2}:\d{2} (AM|PM)"
# Matchオブジェクトを取得
match = re.search(pattern, text)
# マッチした部分文字列をグループとして取得
groups = match.groups()
# 結果を出力
print(groups)
出力:
('10:00', 'AM', '3:00', 'PM')
電話番号を抽出する
import re
text = "My phone number is 03-1234-5678 and yours is 090-9876-5432."
# 電話番号抽出パターン
pattern = r"(\d{2,3})-(\d{4})-(\d{4})"
# Matchオブジェクトを取得
match = re.search(pattern, text)
# マッチした部分文字列をグループとして取得
groups = match.groups()
# 結果を出力
print(groups)
出力:
('03', '1234', '5678', '090', '9876', '5432')
郵便番号を抽出する
import re
text = "My zip code is 123-4567 and yours is 890-1234."
# 郵便番号抽出パターン
pattern = r"\d{3}-\d{4}"
# Matchオブジェクトを取得
match = re.search(pattern, text)
# マッチした部分文字列をグループとして取得
groups = match.groups()
# 結果を出力
print(groups
Python テキスト処理:re.Match.groups() の代替方法
re.findall()
は、正規表現パターンにマッチしたすべての部分文字列をリストとして返します。
import re
text = "This is a string with a number 1234 and a word Python."
# 数字と文字列を抽出するパターン
pattern = r"\d+|\w+"
# マッチした部分文字列のリストを取得
groups = re.findall(pattern, text)
# 結果を出力
print(groups)
出力:
['1234', 'Python']
**2. re.sub()
re.sub()
は、正規表現パターンにマッチした部分文字列を置換文字列で置き換えます。
import re
text = "This is a string with a number 1234 and a word Python."
# 数字と文字列を抽出するパターン
pattern = r"\d+|\w+"
# マッチした部分文字列を置換
replaced_text = re.sub(pattern, "**", text)
# 結果を出力
print(replaced_text)
出力:
This is a string with a number ** and a word **.
手動で抽出
単純なパターンであれば、手動で部分文字列を抽出することも可能です。
text = "This is a string with a number 1234 and a word Python."
# 数字と文字列を抽出
number = text.split(" ")[3]
word = text.split(" ")[5]
# 結果を出力
print(number, word)
出力:
1234 Python
パーサーライブラリ
lxml
などのパーサーライブラリを使用すれば、より複雑な構造のテキストから部分文字列を抽出することができます。
from lxml import etree
text = """
<html>
<head>
<title>This is a title</title>
</head>
<body>
This is a string with a number 1234 and a word Python.
</body>
</html>
"""
# HTMLをパース
root = etree.fromstring(text)
# タイトルを取得
title = root.find("head/title").text
# 数字と文字列を取得
number = root.find("body").text.split(" ")[3]
word = root.find("body").text.split(" ")[5]
# 結果を出力
print(title, number, word)
出力:
This is a title 1234 Python
方法の選択
どの方法を使用するかは、以下の要素を考慮する必要があります。
- パターン
- テキストの構造
- 処理速度
- コードの簡潔さ
re.Match.groups()
以外にも、さまざまな方法でテキスト処理を行うことができます。それぞれの方法の特徴を理解し、状況に応じて適切な方法を選択することが重要です。
デバッガーで Python ResourceWarning の原因を徹底分析! 問題解決への近道
ResourceWarningは、以下の状況で発生する可能性があります。メモリリーク: プログラムが不要になったメモリを解放しない場合、メモリリークが発生します。ファイルハンドルリーク: プログラムが不要になったファイルハンドルを閉じない場合、ファイルハンドルリークが発生します。
Pythonで潜む罠:RecursionErrorの正体と完全攻略マニュアル
Pythonでは、再帰呼び出しの最大回数に制限を設けています。これは、無限ループによるスタックオーバーフローを防ぐためです。デフォルトでは、この最大回数は1000です。再帰呼び出しが最大回数をを超えると、RecursionError例外が発生します。
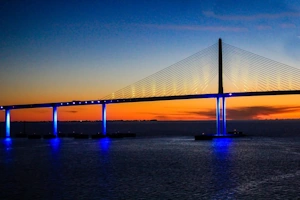
SystemErrorとその他の例外
SystemErrorの詳細発生条件: インタプリタ内部でエラーが発生した場合原因: インタプリタのバグ深刻度: 致命的ではないが、プログラムの動作に影響を与える可能性がある関連値: エラーが発生した場所を示す文字列対処方法: 使用中の Python インタプリタのバージョンとエラーメッセージを報告する 可能であれば、代替の解決策を見つける 問題が修正されるまで、プログラムの使用を中止する
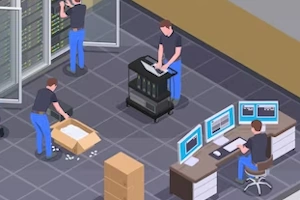
Pythonで並行処理をマスター!スレッド、マルチプロセス、非同期プログラミングの比較
Concurrent Execution において、thread. get_ident() は以下の用途で使用されます。1. スレッドの識別:複数のスレッドが同時に実行されている場合、thread. get_ident() を使用して個々のスレッドを区別することができます。これは、ログ記録やデバッグを行う際に役立ちます。
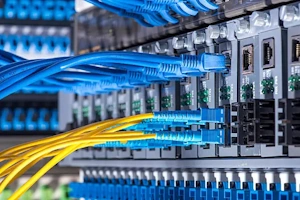
sched.scheduler.cancel()の動作メカニズム
この関数の動作メカニズムcancel() 関数にタスク識別子を渡します。識別子は、sched. scheduler. enter() 関数でタスクをスケジューリングする際に設定したものです。スケジューラは、実行待ちのタスクキューを調べます。一致するタスクが見つかれば、そのタスクはキューから削除されます。
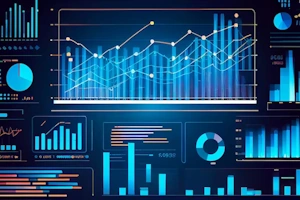

readline.get_history_length():Python Text Processingにおけるコマンド履歴操作の基礎
概要readlineモジュールは、対話型インターフェースにおけるコマンド履歴機能を提供します。get_history_length() は、その履歴の長さを整数で返します。履歴の長さは、ユーザーが過去に入力したコマンドの数です。コード例出力例
collections.abc モジュールを使用した具体的なユースケース
Collections abstract base classes (collections. abc) は、これらの共通操作を定義した抽象基底クラスの集合です。抽象基底クラスは、具体的な実装を提供するのではなく、インターフェースを定義します。
Pythonカレンダープログラミング:calendar.AUGUST で8月のカレンダーを自在に操る
Pythonは動的型付け言語なので、変数を宣言する際に型を指定する必要はありません。変数に代入された値によって型が決まります。主なデータ型は以下の通りです。数値型: 整数: int 型 - 例: 1, -2, 100 浮動小数点数: float 型 - 例: 3.14
multiprocessing.connection.Connection.close() の注意事項
マルチプロセッシングでは、複数のプロセス間でデータを共有したり、タスクを実行したりするために、接続を使用します。しかし、処理が終了した後、接続を閉じてリソースを解放しないと、以下の問題が発生する可能性があります。メモリリーク: 接続オブジェクトはメモリを占有するため、閉じていない接続が多数存在すると、メモリ不足が発生する可能性があります。
Pythonの並行実行におけるsubprocess.CalledProcessErrorの処理方法
この解説では、以下の内容について分かりやすく説明します。subprocess. CalledProcessErrorの概要 発生原因 属性 例外処理発生原因属性例外処理並行実行における影響 エラーの検出と処理 デバッグと原因特定エラーの検出と処理