Qt WidgetsでQPlainTextEdit::plainTextを使ってテキストを操作する
QPlainTextEdit is a widget in Qt that is used to edit and display plain text. It is a subclass of QAbstractScrollArea, which means that it can be used to display large amounts of text without performance problems.
QPlainTextEdit::plainText is a property that gets and sets the plain text contents of the text editor. The plain text is the text without any formatting, such as font styles, colors, or embedded images.
Getting the Plain Text
To get the plain text contents of a QPlainTextEdit widget, you can use the following code:
QString text = plainTextEdit->toPlainText();
This code will get the plain text contents of the plainTextEdit widget and store them in the text variable.
Setting the Plain Text
To set the plain text contents of a QPlainTextEdit widget, you can use the following code:
plainTextEdit->setPlainText(text);
This code will set the plain text contents of the plainTextEdit widget to the text stored in the text variable.
Example
The following code shows an example of how to use QPlainTextEdit::plainText to get and set the plain text contents of a text editor:
QPlainTextEdit *plainTextEdit = new QPlainTextEdit();
plainTextEdit->setPlainText("This is some plain text.");
QString text = plainTextEdit->toPlainText();
qDebug() << text;
plainTextEdit->setPlainText("This is some different plain text.");
text = plainTextEdit->toPlainText();
qDebug() << text;
This code will output the following text to the console:
This is some plain text.
This is some different plain text.
Additional Notes
- The plainText property is always available, even if the text editor is read-only.
- Setting the plainText property will clear the undo/redo history of the text editor.
I hope this explanation is helpful. Please let me know if you have any other questions.
Getting the text from a file
The following code shows how to get the plain text contents of a file and display them in a QPlainTextEdit widget:
QPlainTextEdit *plainTextEdit = new QPlainTextEdit();
QFile file("myfile.txt");
if (file.open(QIODevice::ReadOnly)) {
QTextStream in(&file);
plainTextEdit->setPlainText(in.readAll());
file.close();
} else {
qDebug() << "Error opening file";
}
This code will open the file myfile.txt and read its contents into the text variable. The text variable will then be used to set the plain text contents of the plainTextEdit widget.
Saving the text to a file
The following code shows how to save the plain text contents of a QPlainTextEdit widget to a file:
QPlainTextEdit *plainTextEdit = new QPlainTextEdit();
QFile file("myfile.txt");
if (file.open(QIODevice::WriteOnly)) {
QTextStream out(&file);
out << plainTextEdit->toPlainText();
file.close();
} else {
qDebug() << "Error opening file";
}
This code will open the file myfile.txt and write the plain text contents of the plainTextEdit widget to the file.
Highlighting specific text
The following code shows how to highlight specific text in a QPlainTextEdit widget:
QPlainTextEdit *plainTextEdit = new QPlainTextEdit();
plainTextEdit->setPlainText("This is some text. Some of it is important.");
QTextDocument *document = plainTextEdit->document();
QTextFinder *finder = new QTextFinder(document);
finder->setSearchText("important");
QTextCursor cursor = finder->first();
while (cursor.isValid()) {
QTextCharFormat format;
format.setBackgroundColor(Qt::yellow);
cursor.setCharFormat(format);
cursor = finder->next();
}
This code will search the plain text contents of the plainTextEdit widget for the text "important" and highlight all occurrences of the text in yellow.
Responding to user input
The following code shows how to respond to user input in a QPlainTextEdit widget:
QPlainTextEdit *plainTextEdit = new QPlainTextEdit();
plainTextEdit->connect(plainTextEdit, &QPlainTextEdit::textChanged, this, &MyClass::textChanged);
This code will connect the textChanged signal of the plainTextEdit widget to the textChanged slot of the MyClass class. The textChanged slot will be called whenever the text in the plainTextEdit widget changes.
In the textChanged slot, you can do whatever you want with the new text, such as:
- Save the text to a file
- Send the text to a server
- Update a database
I hope these examples are helpful. Please let me know if you have any other questions.
However, here are some general tips for finding other methods:
- Search online: Use a search engine like Google to find examples of code that does what you need. Be sure to use specific keywords in your search query.
- Check documentation: The documentation for your programming language or library may contain examples of how to use the features you need.
- Ask for help: If you can't find what you need on your own, you can ask for help from other programmers. There are many online forums and communities where you can post questions and get help from others.
I hope this helps!
Rich Text でテキストフォーマットを識別する方法: QTextFormat::objectIndex() の使いかた
QTextFormat::objectIndex() 関数は、Qt GUI における Rich Text 機能で、テキストフォーマットオブジェクトに固有のインデックス番号を取得するために使用されます。このインデックス番号は、テキストフォーマットオブジェクトを識別し、テキスト内の特定のテキスト領域に適用されているフォーマットを管理する際に役立ちます。
Qt GUIにおけるQTextTableCellFormat::setTopPadding()の詳細解説
QTextTableCellFormat::setTopPadding()は、Qt GUIフレームワークにおいて、テーブルセルの上部余白を設定するための関数です。この関数を用いることで、セル内のテキストと上部の境界線との間に垂直方向のスペースを調整できます。
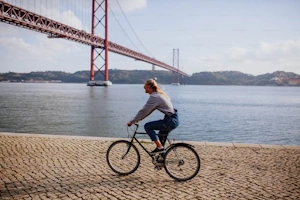
QAbstractTextDocumentLayout::setIndentWidth() 関数を使う
QTextDocument::setIndentWidth()関数は、Qt GUIでテキストドキュメントのインデント幅を設定するために使用します。インデントとは、テキストの先頭部分に空白を挿入することで、段落の開始位置を視覚的に強調する機能です。
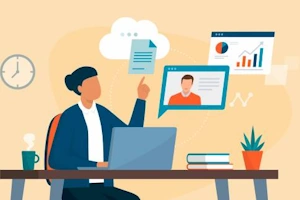
Qt GUIにおけるQRgba64::setGreen()メソッド以外の緑色表現方法
QRgba64::setGreen()メソッドは、Qt GUIライブラリにおいて、QRgba64構造体の緑色成分を指定した値に設定するために使用されます。QRgba64構造体は、64ビットのデータ構造であり、赤、緑、青、アルファの4つの16ビットカラーチャンネルを保持します。

QKeySequence::fromString() 関数による設定
QShortcut::keys() 関数は、以下の役割を果たします。ショートカットキーの取得: 現在のショートカットキーを取得します。ショートカットキーの設定: 新しいショートカットキーを設定します。QShortcut::keys() 関数の使い方は、以下の通りです。
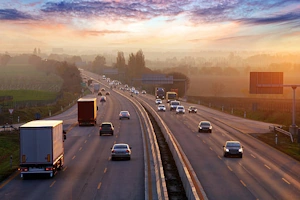

QFont::Weightとは何か?
QFont::Weightの詳細型: 列挙型役割: フォントの太さを設定する使用場所: Qt GUI関連クラス: QFontQFont::Weightの定数QFont::Weightの使用例補足上記の例では、QFont::Boldという定数を使用して、ラベルのテキストを太字に設定しています。
Qt GUI アプリケーションにおける OpenGL グラフィックスプログラミングの基礎:QOpenGLContext::functions() の使い方
QOpenGLContext::functions() は、Qt GUI で OpenGL グラフィックスを使用する際に、OpenGL 関数へのアクセスを提供する重要な関数です。この関数は、OpenGL バージョンに基づいた適切な関数ポインタを取得し、アプリケーション内で安全に使用できるようにします。
【初心者向け】Qt GUI プログラミング: QBackingStore::paintDevice() をマスターしよう!
QBackingStore::paintDevice() は、Qt GUI の重要な機能である QBackingStore クラスのメソッドの一つです。このメソッドは、QPainter を用いて QWindow に描画するためのペイントデバイスを取得するために使用されます。
Qt WidgetsにおけるQScrollerProperties::operator==()の解説
QScrollerProperties::operator==() は、2 つの QScrollerProperties オブジェクトの内容を比較し、等価かどうかを判断するための演算子です。この演算子は、== 演算子を使用して呼び出すことができます。
Qt Widgetsでパフォーマンスを向上させる:QListView::batchSizeとその他の方法の比較
バッチ処理とは、複数の処理をまとめて実行することで、個別に実行するよりも効率的に処理を行う手法です。QListView::batchSize プロパティは、このバッチ処理を QListView でどのように行うかを制御します。QListView::batchSize の役割