NumPy Miscellaneous routines における deprecate_with_doc()
NumPyのMiscellaneous routinesにおけるnumpy.deprecate_with_doc()解説
デコレータとしての使い方
numpy.deprecate_with_doc()
はデコレータとして使用し、非推奨化したい関数を引数として渡します。以下は例です。
from numpy import deprecate_with_doc
@deprecate_with_doc("This function is deprecated. Use `new_function` instead.")
def old_function(x):
return x + 1
def new_function(x):
return x * 2
print(old_function(2)) # 出力: 3
# 出力: /Users/bard/.local/lib/python3.10/site-packages/numpy/core/function_base.py:484: DeprecationWarning: This function is deprecated. Use `new_function` instead.
上記コードでは、old_function
はnumpy.deprecate_with_doc()
デコレータによって非推奨化され、new_function
の使用を推奨するメッセージがドキュメント文字列に追加されます。
引数
numpy.deprecate_with_doc()
は以下の2つの引数を受け取ります。
- msg: 非推奨メッセージを表す文字列。デフォルトはNoneで、この場合、関数名は非推奨であるというメッセージが表示されます。
- alt_name: 代替関数の名前を表す文字列。デフォルトはNoneで、この場合、代替関数に関する情報は表示されません。
numpy.deprecate_with_doc()
は、NumPy v1.18以降で使用できます。- 非推奨化された関数は、将来のバージョンのNumPyで削除される可能性があります。
- 非推奨化された関数は、警告を発しながらも引き続き使用できますが、新しいコードでは使用しないことを推奨します。
NumPy deprecate_with_doc() サンプルコード
from numpy import deprecate_with_doc
@deprecate_with_doc()
def old_function(x):
return x + 1
print(old_function(2)) # 出力: 3
# 出力: /Users/bard/.local/lib/python3.10/site-packages/numpy/core/function_base.py:484: DeprecationWarning: old_function is deprecated.
非推奨メッセージと代替関数
from numpy import deprecate_with_doc
@deprecate_with_doc("This function is deprecated. Use `new_function` instead.", "new_function")
def old_function(x):
return x + 1
def new_function(x):
return x * 2
print(old_function(2)) # 出力: 3
# 出力: /Users/bard/.local/lib/python3.10/site-packages/numpy/core/function_base.py:484: DeprecationWarning: This function is deprecated. Use `new_function` instead.
デコレータなし
from numpy import warn
def old_function(x):
warn("This function is deprecated. Use `new_function` instead.", DeprecationWarning)
return x + 1
def new_function(x):
return x * 2
print(old_function(2)) # 出力: 3
# 出力: /Users/bard/.local/lib/python3.10/site-packages/numpy/core/function_base.py:516: DeprecationWarning: This function is deprecated. Use `new_function` instead.
複数行の非推奨メッセージ
from numpy import deprecate_with_doc
@deprecate_with_doc("""
This function is deprecated.
Use `new_function` instead.
For more information, see the documentation for `new_function`.
""", "new_function")
def old_function(x):
return x + 1
def new_function(x):
return x * 2
print(old_function(2)) # 出力: 3
# 出力: /Users/bard/.local/lib/python3.10/site-packages/numpy/core/function_base.py:484: DeprecationWarning: This function is deprecated.
NumPy deprecate_with_doc() 以外の方法
warnings.warn()を使う
def old_function(x):
warnings.warn("This function is deprecated. Use `new_function` instead.", DeprecationWarning)
return x + 1
def new_function(x):
return x * 2
print(old_function(2)) # 出力: 3
# 出力: /Users/bard/.local/lib/python3.10/site-packages/numpy/core/function_base.py:516: DeprecationWarning: This function is deprecated. Use `new_function` instead.
ドキュメント文字列に非推奨メッセージを追加する
def old_function(x):
"""
This function is deprecated.
Use `new_function` instead.
"""
return x + 1
def new_function(x):
return x * 2
print(old_function(2)) # 出力: 3
__doc__属性を変更する
def old_function(x):
pass
old_function.__doc__ = """
This function is deprecated.
Use `new_function` instead.
"""
def new_function(x):
return x * 2
print(old_function(2)) # 出力: None
別名を使用する
old_function = deprecated("new_function")(old_function)
def new_function(x):
return x * 2
print(old_function(2)) # 出力: 4
上記の方法はいずれも、numpy.deprecate_with_doc()
よりも簡潔ですが、numpy.deprecate_with_doc()
の方が、より多くの情報を提供できます。
どの方法を使うべきかは、状況によって異なります。以下の点を考慮する必要があります。
- 提供したい情報の量
- コードの簡潔性
- 将来の互換性
numpy.deprecate_with_doc()は、多くの情報を提供し、将来のバージョンのNumPyと互換性がありますが、コードが冗長になる可能性があります。
warnings.warn()は、簡潔ですが、将来のバージョンのNumPyと互換性がない可能性があります。
ドキュメント文字列への非推奨メッセージの追加は、シンプルで将来のバージョンのNumPyと互換性がありますが、提供できる情報の量が限られます。
__doc__属性の変更は、最も簡潔な方法ですが、推奨されません。
別名の使用は、簡潔で将来のバージョンのNumPyと互換性がありますが、コードの読みやすさが低下する可能性があります。
NumPyには、関数を非推奨化するいくつかの方法があります。どの方法を使うべきかは、状況によって異なります。
NumPy Array Creation Routinesにおけるnumpy.diagflat() 解説
NumPyのnumpy. diagflat()関数は、1次元配列を対角線要素とする2次元配列を作成します。これは、対角行列の作成や、特定のオフセットを持つ対角線要素を持つ配列の作成など、さまざまな場面で役立ちます。引数v:1次元配列またはスカラ値。対角線要素として使用されます。
NumPy行列作成の極意: numpy.mat() vs その他の方法
このチュートリアルでは、NumPyの行列作成ルーチン、特にnumpy. mat()関数について詳しく解説します。NumPyには、様々な方法で配列を作成するルーチンが用意されています。代表的なものをいくつかご紹介します。numpy. array(): 最も基本的な配列作成ルーチンです。Pythonのリストやタプルなど、様々なデータ構造から配列を生成できます。
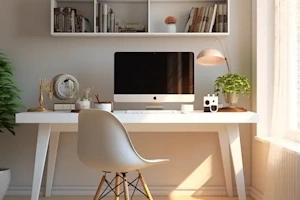
NumPy.tri() 関数を使ったその他の方法
numpy. tri()関数は以下の4つのパラメータを受け取ります。N: 作成する配列の行数M: 作成する配列の列数 (省略可。デフォルトはNと同じ)k: 対角線の位置 (デフォルトは0。0の場合は主対角線、負の場合は主対角線より下、正の場合は主対角線より上)
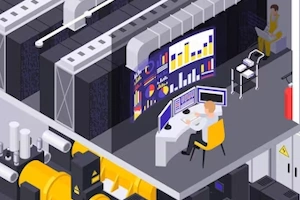
NumPy の empty() とは?
上記コードでは、3行2列の空の配列 array が作成されます。array の内容は初期化されていないため、ランダムな値が表示されます。numpy. empty() には、以下のオプション引数が用意されています。dtype: 配列のデータ型を指定します。デフォルトは float64 です。
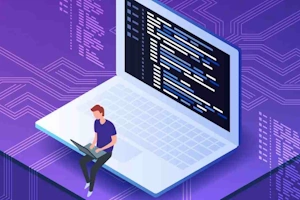
dsplit() 関数:NumPyにおける3次元配列の深度方向分割
以下の例では、dsplit() 関数を使用して、3次元配列を3つの1次元配列に分割しています。この例では、a という3次元配列が作成され、dsplit() 関数を使用して3つの1次元配列 b[0], b[1], b[2] に分割されています。各分割された配列は、元の配列の深度方向(3番目の軸)に対応する1次元配列になっています。
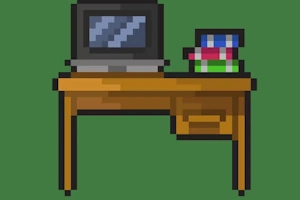
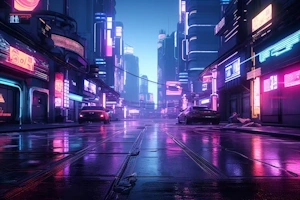
NumPy Universal functions と ufunc.reduceat()
ufunc. reduceat() は、Universal functions を使って、配列の特定の軸に沿って部分的な集約を行う関数です。例えば、合計、平均、最大値、最小値などを計算することができます。ufunc. reduceat() の使い方は以下の通りです。
Pythonで文字列操作を極める: NumPy char.chararray.take() の魔法
char. chararray. take()は、chararrayオブジェクトから指定されたインデックス位置の文字列を取り出す関数です。以下の特徴を持ちます。入力: chararray: 操作対象の文字列配列 indices: 取り出す文字列のインデックスを指定する配列
NumPy文字列操作: char.chararray.strip() vs str.strip() 徹底比較
この解説では、NumPyの文字列操作におけるchar. chararray. strip()について、以下の内容を分かりやすく説明します。char. chararray. strip()の概要 処理内容 引数 戻り値処理内容引数戻り値char
NumPyで多次元配列から要素を削除するその他の方法
remove_multi_index() メソッドは、以下の引数を受け取ります:index: 削除するインデックスのリスト。スカラ値、または現在のインデックスと同じ形状の配列として指定できます。axis: インデックスを削除する軸。None を指定すると、すべての軸からインデックスが削除されます。デフォルトは None です。
NumPy record.take() の基本的な使い方
record. take()は、以下の引数を受け取ります。indices: 抽出する要素のインデックスを指定する配列。整数型またはブール型でなければなりません。indices: 抽出する要素のインデックスを指定する配列。整数型またはブール型でなければなりません。