Pythonカレンダープログラミング:calendar.AUGUST で8月のカレンダーを自在に操る
Pythonのデータ型とcalendar.AUGUST
Pythonは動的型付け言語なので、変数を宣言する際に型を指定する必要はありません。変数に代入された値によって型が決まります。
主なデータ型は以下の通りです。
- 数値型:
- 整数:
int
型 - 例:1
,-2
,100
- 浮動小数点数:
float
型 - 例:3.14
,-0.5
,1e6
- 複素数:
complex
型 - 例:1j
,2+3j
,(1, 2)
- 整数:
- 文字列型:
str
型 - 例:"Hello, world!"
,"Python"
,'a'
- ブール型:
bool
型 - 例:True
,False
- シーケンス型:
- リスト:
list
型 - 例:[1, 2, 3]
,["a", "b", "c"]
,[True, False, None]
- タプル:
tuple
型 - 例:(1, 2, 3)
,("a", "b", "c")
,(True, False, None)
- リスト:
- マッピング型:
- その他:
None
型 - 例:None
calendar.AUGUST
calendar
モジュールは、カレンダー関連の機能を提供します。
calendar.AUGUST
は、8月を表す定数です。値は 8 です。
以下のコード例では、calendar.AUGUST
を使用して、8月のカレンダーを出力しています。
import calendar
print(calendar.month(2023, calendar.AUGUST))
出力例:
August 2023
Mo Tu We Th Fr Sa Su
1 2 3 4 5
6 7 8 9 10 11 12
13 14 15 16 17 18 19
20 21 22 23 24 25 26
27 28 29 30 31
- Pythonのデータ型は、数値型、文字列型、ブール型、シーケンス型、マッピング型などがあります。
calendar
モジュールは、カレンダー関連の機能を提供します。calendar.AUGUST
は、8月を表す定数です。
calendar.AUGUST を使用したサンプルコード
8月のカレンダーを出力する
import calendar
print(calendar.month(2023, calendar.AUGUST))
8月の特定の曜日の日付を取得する
import calendar
# 8月の水曜日を取得
wednesdays = calendar.monthcalendar(2023, calendar.AUGUST)[2]
# 水曜日の日付を出力
for date in wednesdays:
if date != 0:
print(date)
8月の祝日を取得する
import calendar
# 日本語の祝日を取得
holidays = calendar.locale.get_holidays(2023, locale="ja")
# 8月の祝日を出力
for holiday in holidays:
if holiday.month == calendar.AUGUST:
print(holiday.day, holiday.name)
8月のカレンダーを HTML 形式で出力する
import calendar
import html
# 8月のカレンダーを取得
cal = calendar.monthcalendar(2023, calendar.AUGUST)
# HTML コードを生成
html_code = """
<table border="1">
<caption>""" + html.escape(cal[0]) + """</caption>
<tr>"""
for day in ("日", "月", "火", "水", "木", "金", "土"):
html_code += "<th>" + html.escape(day) + "</th>"
html_code += "</tr>"
for week in cal[1:]:
html_code += "<tr>"
for day in week:
if day == 0:
html_code += "<td></td>"
else:
html_code += "<td>" + str(day) + "</td>"
html_code += "</tr>"
html_code += "</table>"
# HTML ファイルを出力
with open("august_calendar.html", "w") as f:
f.write(html_code)
8月のカレンダーを画像形式で出力する
import calendar
import matplotlib.pyplot as plt
# 8月のカレンダーを取得
cal = calendar.monthcalendar(2023, calendar.AUGUST)
# 図を作成
fig, ax = plt.subplots()
ax.matshow(cal)
# 軸ラベルを設定
ax.set_xlabel("曜日")
ax.set_ylabel("週")
# 月表示を設定
plt.title(cal[0])
# 画像ファイルを出力
plt.savefig("august_calendar.png")
8月のカレンダーを iCal 形式で出力する
import calendar
import icalendar
# 8月のカレンダーを取得
cal = calendar.monthcalendar(2023, calendar.AUGUST)
# iCalendar オブジェクトを作成
cal_obj = icalendar.Calendar()
# イベントを追加
for week in cal[1:]:
for day in week:
if day != 0:
event = icalendar.Event()
event.add("dtstart", icalendar.Date(2023, calendar.AUGUST, day))
event.add("summary", "8月 {}日".format(day))
cal_obj.add_component(event)
# iCal ファイルを出力
with open("august_calendar.ics", "wb") as f:
f.write(cal_obj.to_ical())
ご参考になれば幸いです。
calendar.AUGUST を使用しない方法
手動でカレンダーを作成する
def print_calendar(year, month):
# 1日の曜日を取得
weekday = calendar.weekday(year, month, 1)
# 1行目の空白を出力
print(" " * weekday * 3, end="")
# 1行目を出力
for day in range(1, 8):
print("{:>2}".format(day), end=" ")
print()
# 2行目以降を出力
for week in range(2, 5):
for day in range(1, 8):
date = day + (week - 1) * 7
if date <= calendar.monthrange(year, month)[1]:
print("{:>2}".format(date), end=" ")
else:
print(" ", end=" ")
print()
print_calendar(2023, calendar.AUGUST)
第三者ライブラリを使用する
from dateutil.relativedelta import relativedelta
from dateutil.rrule import rrule
# 8月のカレンダーを取得
start_date = datetime.date(2023, 8, 1)
end_date = start_date + relativedelta(months=1) - relativedelta(days=1)
# ルールを作成
rule = rrule(rrule.DAILY, dtstart=start_date, until=end_date)
# カレンダーを出力
for date in rule:
print(date.strftime("%Y-%m-%d"))
これらの方法は、calendar.AUGUST
を使用しない方法です。
ご参考になれば幸いです。
ImportError.name を解決する他の方法
発生原因ImportError. name は、以下のいずれかの理由で発生します。モジュールが存在しない: インポートしようとしているモジュールが実際に存在しない場合。モジュールの名前が間違っている: インポートしようとしているモジュールの名前を間違って記述している場合。
【Python初心者向け】LookupError例外って何?発生原因と対処法を徹底解説
LookupError は、以下の 2 つの具体的な例外クラスに分類されます。KeyError: 辞書などのマッピングオブジェクトで、存在しないキーが使用された場合に発生します。IndexError: リストなどのシーケンスオブジェクトで、存在しないインデックスが使用された場合に発生します。
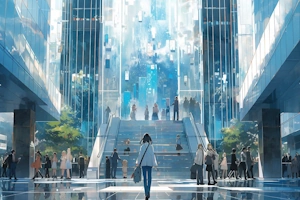
Python エンコーディング警告とは?
しかし、異なるエンコーディング間で文字列を変換する場合、文字化けが発生する可能性があります。文字化けとは、本来の文字とは異なる文字が表示されてしまう現象です。エンコーディング警告は、文字化けが発生する可能性がある箇所を警告するために用意された例外です。この警告は、プログラムの実行を止める致命的エラーではありませんが、無視すると文字化けなどの問題が発生する可能性があります。
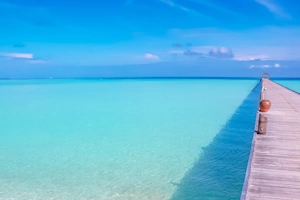
OSError.winerrorによる詳細なエラー情報取得
OSError. winerrorは、Windows上で発生するエラーを表す例外です。OSError例外は、ファイル操作、ネットワーク操作、プロセス管理など、様々な操作で発生する可能性があります。winerror属性は、エラーの詳細情報を提供します。
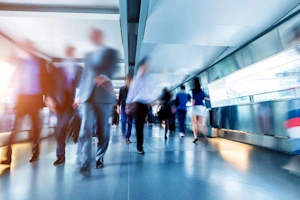
SystemErrorとその他の例外
SystemErrorの詳細発生条件: インタプリタ内部でエラーが発生した場合原因: インタプリタのバグ深刻度: 致命的ではないが、プログラムの動作に影響を与える可能性がある関連値: エラーが発生した場所を示す文字列対処方法: 使用中の Python インタプリタのバージョンとエラーメッセージを報告する 可能であれば、代替の解決策を見つける 問題が修正されるまで、プログラムの使用を中止する
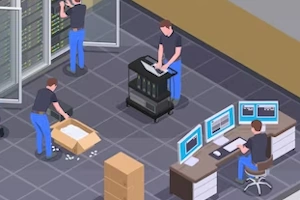

Utilities and Decorators
enum は、Python 3.4で導入された標準ライブラリであり、列挙型と呼ばれるデータ型を定義するためのモジュールです。列挙型は、数値や文字列の集合を名前付きの定数として定義するもので、コードの可読性と保守性を向上させることができます。
Python データ型「types.ClassMethodDescriptorType」徹底解説
types. ClassMethodDescriptorType は、Python のデータ型の一つで、クラスメソッドを表す型です。クラスメソッドは、クラスオブジェクトとインスタンスオブジェクトの両方から呼び出すことができる特殊なメソッドです。
Pythonで日付を扱う:datetime.date.__format__() メソッド完全解説
datetime. date. __format__() メソッドは、date オブジェクトを指定された書式に従って文字列に変換します。これは、strftime() メソッドとほぼ同じ機能を提供しますが、より簡潔な構文で記述できます。書式指定には、以下の文字列を使用できます。
collections.deque.appendleft() をマスターして、Python プログラミングをもっと便利に!
collections. deque. appendleft() は、deque の左側(先頭)に要素を追加するメソッドです。このメソッドは、以下の特徴を持っています。引数appendleft() メソッドは、1 つの引数を受け取ります。要素: 左側に追加する要素
readline.get_history_length():Python Text Processingにおけるコマンド履歴操作の基礎
概要readlineモジュールは、対話型インターフェースにおけるコマンド履歴機能を提供します。get_history_length() は、その履歴の長さを整数で返します。履歴の長さは、ユーザーが過去に入力したコマンドの数です。コード例出力例