Qt GUI で QTextList::itemNumber() 関数を使用して特定の項目にアクセスする方法
Qt GUI における QTextList::itemNumber() 関数の詳細解説
概要
QTextList::itemNumber()
関数は、QTextList オブジェクト内の特定の QTextBlock がリスト内のどの項目に対応しているのかを調べ、そのインデックスを返します。もし、その QTextBlock
がリスト内に存在しない場合は、-1
を返します。
用途
この関数は、以下の場面で役立ちます。
- リスト内の特定の項目にアクセスしたい場合
- リスト内の項目の順序を変更したい場合
- リスト内の項目を削除したい場合
関数の詳細
int QTextList::itemNumber(const QTextBlock &block) const
引数
block
: 対象となるQTextBlock
オブジェクト
戻り値
- リスト内の
QTextBlock
のインデックス。もし、そのQTextBlock
がリスト内に存在しない場合は、-1
を返します。
例
QTextList list;
list.append("項目1");
list.append("項目2");
list.append("項目3");
QTextBlock block = list.item(1); // "項目2" に相当する QTextBlock を取得
int index = list.itemNumber(block);
if (index != -1) {
// "項目2" はリスト内の 1 番目の項目であることが確認できました。
}
補足
QTextList
オブジェクト内の項目は、0 から始まるインデックスで管理されています。QTextList::itemNumber()
関数は、const 関数であるため、リストの内容を変更することはできません。- リスト内の項目にアクセスするには、
QTextList::item()
関数を使用します。
上記以外にも、QTextList
クラスには、様々な機能を提供する関数が用意されています。詳しくは、Qt 公式ドキュメントを参照してください。
Sure, here are some sample code snippets that demonstrate the usage of QTextList::itemNumber()
function in various scenarios:
Accessing a Specific Item in the List
This code snippet retrieves the second item from the list, which is "Item 2", and then prints its index:
QTextList list;
list.append("Item 1");
list.append("Item 2");
list.append("Item 3");
QTextBlock block = list.item(1);
int index = list.itemNumber(block);
if (index != -1) {
std::cout << "The index of the block is: " << index << std::endl;
}
Checking if an Item Exists in the List
This code snippet checks if the item "Item 4" exists in the list and prints a message accordingly:
QTextList list;
list.append("Item 1");
list.append("Item 2");
list.append("Item 3");
QTextBlock block("Item 4");
int index = list.itemNumber(block);
if (index != -1) {
std::cout << "The item 'Item 4' exists in the list at index: " << index << std::endl;
} else {
std::cout << "The item 'Item 4' does not exist in the list." << std::endl;
}
Modifying the List Based on Item Index
This code snippet modifies the text of the item located at index 1 (which is "Item 2") to "Modified Item 2":
QTextList list;
list.append("Item 1");
list.append("Item 2");
list.append("Item 3");
int index = 1;
QTextBlock block = list.item(index);
block.setText("Modified Item 2");
std::cout << "The modified list contents:" << std::endl;
for (int i = 0; i < list.count(); ++i) {
std::cout << list.item(i).text().toStdString() << std::endl;
}
Removing an Item from the List Based on Index
This code snippet removes the item located at index 1 (which is "Item 2") from the list:
QTextList list;
list.append("Item 1");
list.append("Item 2");
list.append("Item 3");
int index = 1;
list.removeItem(index);
std::cout << "The list contents after removing item at index 1:" << std::endl;
for (int i = 0; i < list.count(); ++i) {
std::cout << list.item(i).text().toStdString() << std::endl;
}
These examples illustrate the basic usage of QTextList::itemNumber()
function in different contexts. You can adapt and extend these snippets to suit your specific programming needs and requirements.
While QTextList::itemNumber()
is a straightforward and efficient method for determining the index of a specific QTextBlock
within a QTextList
, there are alternative approaches that can be employed in certain situations. Here are a couple of options to consider:
Iterating Through the List
If you need to access multiple items in the list or perform operations based on their order, iterating through the list using a loop might be a more suitable approach. This method allows you to examine each QTextBlock
individually and apply the necessary actions.
QTextList list;
list.append("Item 1");
list.append("Item 2");
list.append("Item 3");
for (int i = 0; i < list.count(); ++i) {
QTextBlock block = list.item(i);
// Perform operations on the current block
std::cout << "Item at index " << i << ": " << block.text().toStdString() << std::endl;
}
Using QTextBlock::findBlockByNumber()`
The QTextList
class provides an alternative function, findBlockByNumber()
, which takes an index as input and returns the corresponding QTextBlock
if it exists. This function can be useful if you already know the index of the item you want to access.
QTextList list;
list.append("Item 1");
list.append("Item 2");
list.append("Item 3");
int index = 1; // Assuming you know the index of the desired item
QTextBlock block = list.findBlockByNumber(index);
if (block.isValid()) {
// Access the retrieved block
std::cout << "Item at index " << index << ": " << block.text().toStdString() << std::endl;
} else {
std::cout << "No block found at index: " << index << std::endl;
}
Choosing the Right Approach
The choice between these methods depends on the specific context and requirements of your program. If you need to access a specific item based on its content, QTextList::itemNumber()
is a direct and efficient approach. However, if you need to iterate through the list or perform operations based on item order, using a loop or findBlockByNumber()
might be more suitable.
Consider the following factors when making your decision:
- Number of items to access: If you only need to access a few specific items,
itemNumber()
might be sufficient. For accessing multiple items or iterating through the entire list, a loop orfindBlockByNumber()
could be more efficient. - Operations on items: If you need to perform specific operations on each item based on its order or content, a loop or
findBlockByNumber()
would allow for more granular control. - Performance considerations: If performance is critical,
itemNumber()
might be slightly more efficient for accessing individual items, as it directly retrieves the index without iterating through the list.
Ultimately, the best approach depends on the specific needs of your program and the trade-offs between simplicity, efficiency, and flexibility.
Qt GUIにおける数値範囲設定のベストプラクティス
Range::to は、Qt の QSlider や QSpinBox などのウィジェットで数値範囲を設定するために使用されます。この関数は、範囲の開始値と終了値を指定することで、ウィジェットの最小値と最大値を設定します。例:Range::to を使用することで、以下の利点があります。
Qt GUI でセルが表の右端にあるかどうかを QTextTableCell::column() 関数で確認する方法
QTextTable クラスは、テキストベースの表を作成および管理するために使用されます。QTextTableCell クラスは、表内の個々のセルを表します。QTextTableCell::column() 関数は、以下の情報を提供します。
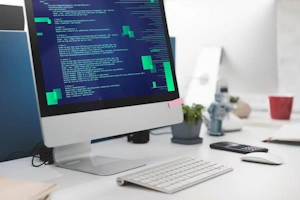
Qt GUI の QValidator::locale() 関数
この関数は以下の情報を提供します:小数点記号: 数字の区切り文字(例:カンマ "," またはピリオド ".")千分位記号: 3桁ごとに数字を区切る文字(例:カンマ "," またはピリオド ".")負数の符号: 負の数を表す記号(例:マイナス "-" またはプラス "+")

QTextListFormat::numberPrefix()で番号の前に文字列を挿入
QTextListFormat::numberPrefix()は、Qt GUIで箇条書きリストの番号の前に表示される文字列を設定するための関数です。機能この関数を使うと、デフォルトの番号ではなく、独自の文字列を番号の前に挿入することができます。例えば、以下のような設定が可能です。
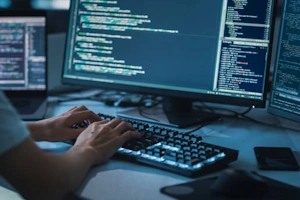
Qt GUI アプリケーションにおけるフォーカス管理:QWindow::focusObjectChanged() シグナルの徹底解説
QWindow::focusObjectChanged() は、Qt GUI アプリケーションにおける重要なシグナルの一つです。これは、フォーカスを受け取るオブジェクトが変更されたときに発生し、開発者がそれに応じて適切な処理を行うための機能を提供します。
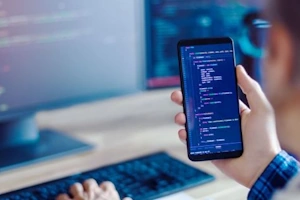
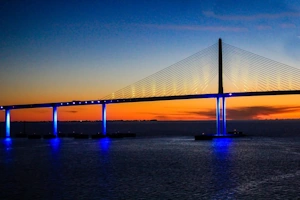
Qt Widgetsでツールボタンスタイルを自在に操る: QMainWindow::toolButtonStyleChanged() の活用指南
QMainWindow::toolButtonStyleChanged()は、QMainWindowウィジェットのツールボタンスタイルが変更されたときにemitされるシグナルです。このシグナルは、アプリケーション全体の外観の一貫性を保つために、他のコンポーネントに接続することができます。
Qt Widgets: QTableWidget::event() 関数の詳細解説
QTableWidget::event() は、Qt Widgets モジュールの QTableWidget クラスで使用される重要な仮想関数です。この関数は、ウィジェットに送信されたイベントを処理するために使用されます。イベントには、マウスのクリックやキーの押下など、ユーザーとのやり取りに関するものが含まれます。
Qt WidgetsにおけるQProxyStyle::layoutSpacing()とは
QProxyStyle::layoutSpacing()は、Qt Widgetsで使用される関数で、ウィジェット間のレイアウトスペースを取得するために使用されます。この関数は、ウィジェット間のデフォルトの水平方向および垂直方向のスペースをピクセル単位で返します。
【初心者向け】Qt GUI プログラミング: QBackingStore::paintDevice() をマスターしよう!
QBackingStore::paintDevice() は、Qt GUI の重要な機能である QBackingStore クラスのメソッドの一つです。このメソッドは、QPainter を用いて QWindow に描画するためのペイントデバイスを取得するために使用されます。
QOpenGLExtraFunctions::glGetProgramPipelineInfoLog() 関数の詳細解説
関数の概要役割: OpenGLパイプラインの情報ログを取得するヘッダーファイル: QOpenGLFunctionsプロトタイプ:引数: programPipeline: 情報を取得するパイプラインオブジェクト bufSize: 情報ログバッファのサイズ length: 実際に書き込まれた情報ログの長さ infoLog: 情報ログを出力するバッファ