QUndoStack::undoTextChanged()関数によるUndo/Redo機能の実装
Qt GUIにおけるQUndoStack::undoTextChanged()の解説
機能概要
QUndoStack::undoTextChanged()
は以下の機能を提供します。
- テキスト編集操作を1つずつ元に戻す/やり直す
- 元に戻す/やり直す履歴を管理
- 元に戻す/やり直す操作をプログラムで制御
使用例
以下のコードは、QUndoStack
とQTextEdit
を使用して、テキスト編集操作を元に戻す/やり直す機能を実装する例です。
#include <QtWidgets>
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow() {
// ウィジェットの作成
QWidget *widget = new QWidget;
setCentralWidget(widget);
// テキストエディタの作成
QTextEdit *textEdit = new QTextEdit;
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(textEdit);
widget->setLayout(layout);
// 元に戻す/やり直すスタックの作成
QUndoStack *undoStack = new QUndoStack;
// テキスト変更時の処理
connect(textEdit, &QTextEdit::textChanged, [undoStack, textEdit](const QString &text) {
// テキスト変更を元に戻すスタックに追加
undoStack->push(new QUndoCommand([textEdit, text] {
textEdit->setText(text);
}));
});
// 元に戻すボタンの作成
QPushButton *undoButton = new QPushButton("元に戻す");
undoButton->setEnabled(false);
connect(undoButton, &QPushButton::clicked, undoStack, &QUndoStack::undo);
// やり直すボタンの作成
QPushButton *redoButton = new QPushButton("やり直す");
redoButton->setEnabled(false);
connect(redoButton, &QPushButton::clicked, undoStack, &QUndoStack::redo);
// ツールバーの作成
QToolBar *toolBar = new QToolBar;
toolBar->addWidget(undoButton);
toolBar->addWidget(redoButton);
addToolBar(Qt::TopToolBarArea, toolBar);
// 元に戻す/やり直すスタックの状態変化時の処理
connect(undoStack, &QUndoStack::canUndoChanged, undoButton, &QPushButton::setEnabled);
connect(undoStack, &QUndoStack::canRedoChanged, redoButton, &QPushButton::setEnabled);
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
MainWindow mainWindow;
mainWindow.show();
return app.exec();
}
このコードでは、以下の処理が行われています。
QUndoStack
オブジェクトを作成し、undoStack
変数に格納します。QTextEdit
オブジェクトを作成し、textEdit
変数に格納します。- テキスト変更時に
QUndoStack::push()
を使用して、テキスト変更操作を元に戻すスタックに追加します。 QPushButton
オブジェクトを2つ作成し、それぞれ「元に戻す」、「やり直す」ボタンとして設定します。- ツールバーを作成し、「元に戻す」、「やり直す」ボタンを追加します。
QUndoStack::canUndoChanged
、QUndoStack::canRedoChanged
シグナルに接続し、「元に戻す」、「やり直す」ボタンの有効状態を更新します。
詳細
QUndoStack::undoTextChanged()
関数には、以下の引数があります。
text
: 元に戻したいテキスト
この関数を使用する際は、以下の点に注意する必要があります。
- テキスト変更操作を元に戻すスタックに追加するには、
QUndoStack::push()
を使用する必要があります。 - 元に戻す/やり直す操作をプログラムで制御するには、
QUndoStack::undo()
、QUndoStack::redo()
関数を
Qt GUIにおけるQUndoStack::undoTextChanged()のサンプルコード
基本的な使用例
#include <QtWidgets>
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow() {
// ウィジェットの作成
QWidget *widget = new QWidget;
setCentralWidget(widget);
// テキストエディタの作成
QTextEdit *textEdit = new QTextEdit;
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(textEdit);
widget->setLayout(layout);
// 元に戻す/やり直すスタックの作成
QUndoStack *undoStack = new QUndoStack;
// テキスト変更時の処理
connect(textEdit, &QTextEdit::textChanged, [undoStack, textEdit](const QString &text) {
// テキスト変更を元に戻すスタックに追加
undoStack->push(new QUndoCommand([textEdit, text] {
textEdit->setText(text);
}));
});
// 元に戻すボタンの作成
QPushButton *undoButton = new QPushButton("元に戻す");
undoButton->setEnabled(false);
connect(undoButton, &QPushButton::clicked, undoStack, &QUndoStack::undo);
// やり直すボタンの作成
QPushButton *redoButton = new QPushButton("やり直す");
redoButton->setEnabled(false);
connect(redoButton, &QPushButton::clicked, undoStack, &QUndoStack::redo);
// ツールバーの作成
QToolBar *toolBar = new QToolBar;
toolBar->addWidget(undoButton);
toolBar->addWidget(redoButton);
addToolBar(Qt::TopToolBarArea, toolBar);
// 元に戻す/やり直すスタックの状態変化時の処理
connect(undoStack, &QUndoStack::canUndoChanged, undoButton, &QPushButton::setEnabled);
connect(undoStack, &QUndoStack::canRedoChanged, redoButton, &QPushButton::setEnabled);
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
MainWindow mainWindow;
mainWindow.show();
return app.exec();
}
アンドゥ/リドゥの回数の制限
#include <QtWidgets>
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow() {
// ウィジェットの作成
QWidget *widget = new QWidget;
setCentralWidget(widget);
// テキストエディタの作成
QTextEdit *textEdit = new QTextEdit;
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(textEdit);
widget->setLayout(layout);
// 元に戻す/やり直すスタックの作成
QUndoStack *undoStack = new QUndoStack(this);
undoStack->setUndoLimit(10); // アンドゥ回数の制限
// テキスト変更時の処理
connect(textEdit, &QTextEdit::textChanged, [undoStack, textEdit](const QString &text) {
// テキスト変更を元に戻すスタックに追加
undoStack->push(new QUndoCommand([textEdit, text] {
textEdit->setText(text);
}));
});
// ... (その他の処理)
}
};
アンドゥ/リドゥのスタックの状態監視
#include <QtWidgets>
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow() {
// ウィジェットの作成
QWidget *widget = new QWidget;
setCentralWidget(widget);
// テキストエディタの作成
QTextEdit *textEdit = new QTextEdit;
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(textEdit);
widget->setLayout(layout);
// 元に戻す/やり直すスタックの作成
QUndoStack *undoStack = new QUndoStack;
// テキスト変更時の処理
connect(textEdit, &QTextEdit::textChanged, [undoStack, textEdit](const QString &text) {
// テキスト変更を元に戻すスタックに追加
undoStack->push(new QUndoCommand([textEdit, text] {
textEdit->setText(text);
}));
});
// 元に戻す/やり直すスタックの状態変化時の処理
connect(undoStack, &QUndoStack::canUndoChanged, [this](bool canUndo) {
Qt GUIにおけるテキスト編集操作のUndo/Redo機能の実装方法
QTextDocument::undo() / redo()
QTextDocument
クラスには、undo()
とredo()
というメソッドがあり、これらのメソッドを使用して、テキスト編集操作を元に戻す/やり直すことができます。
QTextDocument *document = textEdit->document();
// テキスト編集操作を元に戻す
document->undo();
// テキスト編集操作をやり直す
document->redo();
自作のUndo/Redoスタック
QUndoStack
クラスを使用せず、自作のUndo/Redoスタックを実装することもできます。
class UndoStack {
public:
void push(const QString &text) {
// テキスト変更をスタックに追加
}
void undo() {
// スタックから最後のテキスト変更を取り出し、テキストを元に戻す
}
void redo() {
// スタックから取り出したテキスト変更を適用し、テキストを元に戻す
}
};
// ...
UndoStack undoStack;
// テキスト変更時の処理
connect(textEdit, &QTextEdit::textChanged, [undoStack, textEdit](const QString &text) {
undoStack.push(text);
});
// ...
// 元に戻すボタンの処理
connect(undoButton, &QPushButton::clicked, [&undoStack] {
undoStack.undo();
});
// やり直すボタンの処理
connect(redoButton, &QPushButton::clicked, [&undoStack] {
undoStack.redo();
});
その他のライブラリ
QUndoStack
クラス以外にも、Undo/Redo機能を提供するライブラリがあります。
これらのライブラリを使用することで、より簡単にUndo/Redo機能を実装することができます。
選択方法
どの方法を選択するかは、アプリケーションの要件と開発者の好みによって異なります。
- シンプルな実装で済む場合は、
QTextDocument::undo()
/redo()
メソッドを使用する。 - より柔軟な実装が必要な場合は、
QUndoStack
クラスを使用する。 - さらに高度な機能が必要な場合は、自作のUndo/Redoスタックを実装するか、他のライブラリを使用する。
QWindow::devicePixelRatio() 関数を使ったサンプルコード
QWindow::devicePixelRatio() 関数は、ウィンドウが属する画面のデバイスピクセル比を取得します。デバイスピクセル比とは、物理的なピクセルと論理的なピクセルの間の比率です。高解像度ディスプレイでは、この値が大きくなります。
Qt GUI アプリケーション開発:QWindow::flags で実現する多様なウィンドウ
QWindow::flags は、QWindow クラスのメンバー関数で、ウィンドウに適用するフラグのセットを取得または設定するために使用されます。これらのフラグは、ウィンドウの装飾、サイズ変更、スタック順序など、さまざまな属性を制御します。
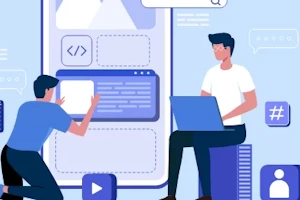
Qt GUI で OpenGL コンテキストを操作する: QWGLContext::nativeContext() 関数の詳細解説
QWGLContext::nativeContext() 関数は、Qt GUI フレームワークにおける OpenGL コンテキスト管理において重要な役割を果たします。この関数は、現在の OpenGL コンテキストのネイティブハンドルを取得するために使用されます。このハンドルは、プラットフォーム固有の API との相互作用や、OpenGL コンテキストを直接制御する必要がある場合に必要となります。
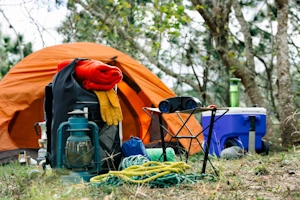
Qt GUI でデータのバインディングと QVector2D::operator QVariant()
QVector2D: 2D ベクトルを表すクラスoperator QVariant(): QVector2D オブジェクトを QVariant 型に変換する関数QVariant: Qt のさまざまなデータ型を汎用的に表現する型QVector2D::operator QVariant() は、さまざまな用途で使用されます。
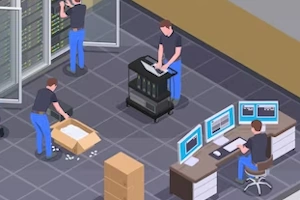
Qt GUIで3Dグラフィックスを扱うためのチュートリアル
QVector3D::toPointF()関数は、3次元ベクトルであるQVector3D型を2次元ポイントであるQPointF型に変換します。これは、3D空間上の点を2D画面上での座標に変換する際に必要となります。詳細QVector3D::toPointF()関数は、以下の式に基づいてQPointF型を生成します。
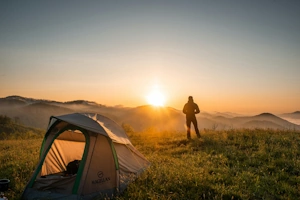
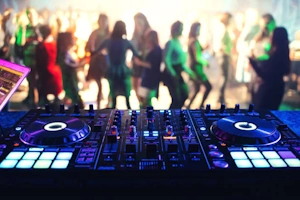
Qt Widgets アプリケーション全体のデフォルトフォントを設定するには?
QApplication::font() は、Qt Widgetsアプリケーション全体のデフォルトフォントを取得または設定するために使用される関数です。この関数は、すべてのウィジェットに適用されるフォントファミリ、サイズ、スタイルなどの属性を指定します。
QTextBlockFormat::QTextBlockFormat() を使ってテキストブロックの書式設定をカスタマイズする方法
テキストブロックのデフォルトの書式設定を定義します。文書内のすべてのテキストブロックに適用されます。個々のテキストブロックの書式設定は、このデフォルト設定を上書きすることができます。**QTextBlockFormat::QTextBlockFormat()**は、以下の引数を受け取りません。
Qt Widgetsでボタンを描画する:QStyleOption::operator=()の活用
Qt Widgetsライブラリは、QtフレームワークにおけるGUI開発用の基本的な要素を提供します。その中で、QStyleOptionクラスは、スタイルエンジンがウィジェットを描画するために必要な情報を格納する重要な役割を担います。QStyleOption::operator=は、この情報を別のQStyleOptionインスタンスにコピーするために使用される演算子です。
QImage::convertToFormat() 以外の画像フォーマット変換方法
宣言: QImage QImage::convertToFormat(QImage::Format format, Qt::ImageConversionFlags flags = Qt::ImageConversionFlags()) const
QAbstractItemView::itemDelegateForColumn()の完全ガイド
Qtのモデル/ビューアーアーキテクチャは、データと視覚表現を分離することで、柔軟で再利用可能なUI開発を可能にします。このアーキテクチャでは、以下の主要なコンポーネントが役割を果たします。モデル (QAbstractItemModel): データを格納し、データへのアクセスを提供します。