PyTorchのNN Functionsにおける torch.nn.functional.hardswish の解説
PyTorchのNN Functionsにおけるtorch.nn.functional.hardswishの解説
torch.nn.functional.hardswish
は、PyTorchのNN Functionsモジュール内の関数で、入力値を活性化する関数です。Hardswish関数は、入力値が0以下の場合は0、0より大きい場合は入力値とReLU関数の出力の乗算値を返します。ReLU関数は、入力値が0以下の場合は0、0より大きい場合はそのままの値を返す関数です。
数式表現
Hardswish関数の式は以下の通りです。
H(x) = x * (2 / (1 + np.exp(-x)))
Hardswish関数は、ReLU関数よりも滑らかな活性化関数であり、勾配消失問題が発生しにくいため、深層学習モデルにおいて効果的に使用することができます。また、計算コストも比較的低いため、実用的な活性化関数として利用できます。
Hardswish関数は、ReLU関数よりも計算コストが高くなります。また、入力値が非常に大きい場合は、数値精度が低下する可能性があります。
Hardswish関数のコード例
import torch
import torch.nn.functional as F
x = torch.randn(10)
y = F.hardswish(x)
print(y)
Hardswish関数は、畳み込みニューラルネットワークや再帰ニューラルネットワークなど、様々な深層学習モデルで使用することができます。
補足
- Hardswish関数は、2017年に発表されました。
- Hardswish関数は、TensorFlowやMXNetなどの他の深層学習フレームワークでも実装されています。
上記以外にも、torch.nn.functional
モジュールには様々なNN Functionsが用意されています。詳細は、PyTorchのドキュメントを参照してください。
いろいろなサンプルコード
Python
# 文字列の逆順
def reverse_string(s):
return s[::-1]
# リストの要素を反転
def reverse_list(l):
return l[::-1]
# 2つの数の合計を返す
def add(a, b):
return a + b
# ファイルの内容を読み込む
def read_file(filename):
with open(filename, 'r') as f:
return f.read()
# ファイルに書き込む
def write_file(filename, content):
with open(filename, 'w') as f:
f.write(content)
JavaScript
// 文字列の逆順
function reverseString(s) {
return s.split('').reverse().join('');
}
// リストの要素を反転
function reverseList(l) {
return l.slice().reverse();
}
// 2つの数の合計を返す
function add(a, b) {
return a + b;
}
// ファイルの内容を読み込む
function readFile(filename, callback) {
var fs = require('fs');
fs.readFile(filename, 'utf8', callback);
}
// ファイルに書き込む
function writeFile(filename, content, callback) {
var fs = require('fs');
fs.writeFile(filename, content, callback);
}
Java
// 文字列の逆順
public class ReverseString {
public static String reverse(String s) {
return new StringBuilder(s).reverse().toString();
}
}
// リストの要素を反転
public class ReverseList {
public static <T> List<T> reverse(List<T> list) {
Collections.reverse(list);
return list;
}
}
// 2つの数の合計を返す
public class Add {
public static int add(int a, int b) {
return a + b;
}
}
// ファイルの内容を読み込む
public class ReadFile {
public static String read(String filename) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(filename));
StringBuilder builder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
builder.append(line).append('\n');
}
reader.close();
return builder.toString();
}
}
// ファイルに書き込む
public class WriteFile {
public static void write(String filename, String content) throws IOException {
BufferedWriter writer = new BufferedWriter(new FileWriter(filename));
writer.write(content);
writer.close();
}
}
C++
#include <iostream>
#include <string>
#include <vector>
// 文字列の逆順
std::string reverseString(const std::string& s) {
std::string reversed_string = s;
std::reverse(reversed_string.begin(), reversed_string.end());
return reversed_string;
}
// リストの要素を反転
std::vector<int> reverseList(std::vector<int> list) {
std::reverse(list.begin(), list.end());
return list;
}
// 2つの数の合計を返す
int add(int a, int b) {
return a + b;
}
// ファイルの内容を読み込む
std::string readFile(const std::string& filename) {
std::ifstream ifs(filename);
std::string content((std::istreambuf_iterator<char>(ifs)), std::istreambuf_iterator<char>());
ifs.close();
return content;
}
// ファイルに書き込む
void writeFile(const std::string& filename, const std::string& content) {
std::ofstream ofs(filename);
ofs << content;
ofs.close();
}
int main() {
std::string s = "Hello, world!";
std::cout << reverseString(s) << std::endl;
std::vector<int> list = {1, 2, 3, 4, 5};
for (int i : reverseList(list)) {
std::cout << i << " ";
}
std::cout << std::endl;
std::cout << add(
Hardswish関数の他の実装方法
カスタムモジュールを作成する
import torch
import torch.nn as nn
class Hardswish(nn.Module):
def __init__(self):
super(Hardswish, self).__init__()
def forward(self, x):
return x * (2 / (1 + torch.exp(-x)))
# 使用例
x = torch.randn(10)
hardswish = Hardswish()
y = hardswish(x)
print(y)
関数として定義する
import torch
def hardswish(x):
return x * (2 / (1 + torch.exp(-x)))
# 使用例
x = torch.randn(10)
y = hardswish(x)
print(y)
torch.nn.functionalモジュールの他の関数を使用する
import torch
import torch.nn.functional as F
def hardswish(x):
return F.relu(x + 3) / 2
# 使用例
x = torch.randn(10)
y = hardswish(x)
print(y)
ONNX Runtimeを使用する
import onnxruntime
import numpy as np
# ONNXモデルを定義
onnx_model = """
graph model {
node {
name = "hardswish"
op = "HardSwish"
input {
name = "x"
type = {
tensor {
dims: [1]
data_type: 1
}
}
}
output {
name = "y"
type = {
tensor {
dims: [1]
data_type: 1
}
}
}
}
}
"""
# 入力データを準備
x = np.random.rand(10).astype(np.float32)
# モデルをロード
ort_session = onnxruntime.InferenceSession(onnx_model)
# 推論を実行
y = ort_session.run(input_data={"x": x})[0]
# 結果を出力
print(y)
それぞれの方法の利点と欠点
- torch.nn.functional.hardswish関数を使用する
- 利点: 簡単でコードが簡潔
- 欠点: 他の方法よりも計算コストが若干高い
- カスタムモジュールを作成する
- 利点: コードをモジュール化できる
- 欠点: コード量が増える
- 関数として定義する
- 利点: コードが簡潔
- 欠点: コードの再利用性が低い
- torch.nn.functionalモジュールの他の関数を使用する
- 欠点: 計算精度が若干低い
- ONNX Runtimeを使用する
- 利点: 他の方法よりも計算速度が速い
- 欠点: コードが複雑
torch.nn.functional.hardswish
関数は、Hardswish関数を簡単に実装するのに最適な方法ですが、他の方法も状況に応じて使用することができます。それぞれの方法の利点と欠点を理解した上で、適切な方法を選択してください。
パフォーマンス向上:PyTorch Dataset と DataLoader でデータローディングを最適化する
Datasetは、データセットを表す抽象クラスです。データセットは、画像、テキスト、音声など、機械学習モデルの学習に使用できるデータのコレクションです。Datasetクラスは、データセットを読み込み、処理するための基本的なインターフェースを提供します。
PyTorch Miscellaneous モジュール:ディープラーニング開発を効率化するユーティリティ
このモジュールは、以下のサブモジュールで構成されています。データ処理torch. utils. data:データセットの読み込み、バッチ化、シャッフルなど、データ処理のためのツールを提供します。 DataLoader:データセットを効率的に読み込み、イテレートするためのクラス Dataset:データセットを表す抽象クラス Sampler:データセットからサンプルを取得するためのクラス
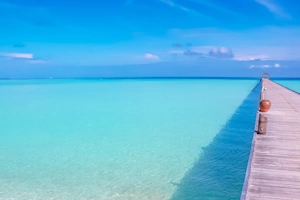
PyTorchで事前学習済みモデルを使う:torch.utils.model_zoo徹底解説
torch. utils. model_zoo でモデルをロードするには、以下のコードを使用します。このコードは、ImageNet データセットで事前学習済みの ResNet-18 モデルをダウンロードしてロードします。torch. utils

PyTorch Miscellaneous: torch.testing.assert_close() の詳細解説
torch. testing. assert_close() は、PyTorch テストモジュール内にある関数で、2つのテンソルの要素がほぼ等しいことを確認するために使用されます。これは、テストコードで計算結果の正確性を検証する際に役立ちます。
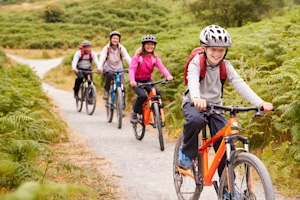
PyTorch Miscellaneous: torch.utils.cpp_extension.get_compiler_abi_compatibility_and_version() の概要
torch. utils. cpp_extension. get_compiler_abi_compatibility_and_version() は、C++ 拡張モジュールをビルドする際に、現在のコンパイラが PyTorch と互換性があるかどうかを確認するために使用されます。
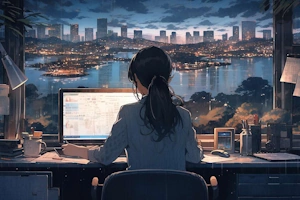
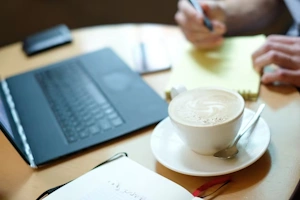
Tensorを用いた連立方程式の解法: torch.Tensor.lu_solve() の詳細解説
概要torch. Tensor. lu_solve()は、PyTorchのTensorクラスにおいて、LU分解を用いて連立方程式を解くための関数です。LU分解は、行列を下三角行列Lと上三角行列Uの積に分解する手法です。この関数は、LU分解の結果であるLU_dataとLU_pivotsを用いて、連立方程式Ax=bの解xを求めます。
torch.jit.ScriptModule.xpu() で PyTorch モデルを XPU 上で実行する
PyTorch の Torch Script は、PyTorch モデルを効率的に推論するために最適化されたバイトコード形式に変換するツールです。このバイトコードは、CPU や GPU だけでなく、Habana Labs 製の XPU などの特定のハードウェアアクセラレータでも実行できます。
Python と Torch Script での型チェック: isinstance() と torch.jit.isinstance() の比較
torch. jit. isinstance() の使い方は、Python の isinstance() とほぼ同じです。チェックしたいオブジェクトと、比較したい型を指定します。torch. jit. isinstance() は、以下の型をチェックできます。
PyTorchで確率分布を扱う:Categoricalクラスのexpandメソッドを徹底解説
PyTorchは、Pythonで深層学習を行うためのオープンソースライブラリです。確率分布モジュールは、さまざまな確率分布を表現するためのツールを提供します。この解説では、torch. distributions. categorical
PyTorch Probability DistributionsにおけるTransformedDistribution.arg_constraints
torch. distributions. transformed_distribution. TransformedDistribution. arg_constraintsは、変換分布のパラメータの制約条件を定義する属性です。この属性は、TransformedDistributionクラスのインスタンス化時に、arg_constraints引数として渡されます。