Qt WidgetsでQStyleOptionViewItem::indexを活用する:アイテム属性取得、状態判定、カスタム描画のすべてを可能にする
Qt WidgetsにおけるQStyleOptionViewItem::indexプログラミング解説
QStyleOptionViewItem::index
は、Qt Widgets
フレームワークにおいて、ビュー項目を描画するためのオプション構造体QStyleOptionViewItem
内に存在するメンバ変数です。この変数は、描画対象となるモデルインデックスを表し、アイテムの属性や状態に関する情報を提供します。
役割
QStyleOptionViewItem::index
は、以下の役割を担います。
- アイテムの属性取得: モデルインデックスから、アイテムのテキスト、アイコン、チェック状態などの属性を取得できます。
- アイテムの状態判定: 選択状態、編集状態、フォーカス状態などのアイテムの状態を判定できます。
- カスタム描画: アイテムデリゲートにおいて、
QStyleOptionViewItem::index
を利用して、アイテムを個別にカスタマイズした描画を行うことができます。
例
以下のコード例は、QStyledItemDelegate
のpaint()
関数におけるQStyleOptionViewItem::index
の利用例です。
void MyDelegate::paint(QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const
{
if (option.state & QStyle::State_Selected) {
painter->setBrush(Qt::red);
} else {
painter->setBrush(Qt::white);
}
painter->drawRect(option.rect);
// アイテムのテキストを描画
painter->drawText(option.rect, Qt::AlignCenter, index.data().toString());
}
このコード例では、QStyleOptionViewItem::index
のstate
メンバ変数を使用して、アイテムが選択されているかどうかを判定し、選択されている場合は赤い矩形を描画しています。また、index.data().toString()
を使用して、アイテムのテキストを取得し、中央揃えで描画しています。
補足
QStyleOptionViewItem::index
は、ビューアイテムを描画する際に常に渡されるわけではありません。アイテムデリゲートが使用されている場合のみ、paint()
関数に渡されます。
注意事項
上記の情報は、Qt 5.15.5時点の情報に基づいています。最新の情報については、Qt 公式ドキュメントを参照してください。
Fibonacci sequence generator
def fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n - 1) + fibonacci(n - 2)
print(fibonacci(10))
This code generates the Fibonacci sequence up to the 10th term.
Caesar cipher
def caesar_cipher(text, shift):
alphabet = "abcdefghijklmnopqrstuvwxyz"
shifted_alphabet = alphabet[shift:] + alphabet[:shift]
encoded_text = ""
for char in text:
if char in alphabet:
encoded_text += shifted_alphabet[alphabet.index(char)]
else:
encoded_text += char
return encoded_text
print(caesar_cipher("Hello, world!", 3))
This code encrypts the text "Hello, world!" using the Caesar cipher with a shift of 3. The encrypted text is "Khoor, zruog!".
Sorting algorithms
Here are some examples of sorting algorithms:
- Bubble sort:
def bubble_sort(array):
for i in range(len(array) - 1):
for j in range(len(array) - i - 1):
if array[j] > array[j + 1]:
array[j], array[j + 1] = array[j + 1], array[j]
array = [5, 2, 4, 1, 3]
bubble_sort(array)
print(array)
- Selection sort:
def selection_sort(array):
for i in range(len(array)):
min_index = i
for j in range(i + 1, len(array)):
if array[j] < array[min_index]:
min_index = j
array[i], array[min_index] = array[min_index], array[i]
array = [5, 2, 4, 1, 3]
selection_sort(array)
print(array)
- Insertion sort:
def insertion_sort(array):
for i in range(1, len(array)):
current_value = array[i]
j = i - 1
while j >= 0 and array[j] > current_value:
array[j + 1] = array[j]
j -= 1
array[j + 1] = current_value
array = [5, 2, 4, 1, 3]
insertion_sort(array)
print(array)
These are just a few examples of the many different types of sample codes that are available. With a little creativity, you can come up with your own unique and interesting codes.
I hope this helps! Let me know if you have any other questions.
Here are some possible interpretations of your question and potential responses:
You are looking for alternative ways to do something.
In this case, I would need to know what you are trying to do in order to provide specific suggestions. For example, if you are asking for alternative ways to cook an egg, I could suggest boiling, frying, or scrambling it.
You are looking for different perspectives on a topic.
In this case, I would need to know what topic you are interested in. I could then provide you with links to articles, videos, or other resources that offer different perspectives on the topic.
You are looking for a way to solve a problem.
In this case, I would need to know more about the problem you are trying to solve. I could then provide you with suggestions for how to solve the problem, or I could connect you with someone who can help.
You are simply saying "other methods" or "other ways".
In this case, I would need more context to provide a specific response. Please provide more details about what you are talking about.
Please let me know if any of these interpretations are correct or if you have any other questions. I am always happy to help!
Qt GUIにおけるアイコンサイズ制御のベストプラクティス
概要ScaledPixmapArgument は、QIconEngine::pixmap() 関数で使用される構造体です。size プロパティは、要求されたピクセルマップのサイズを指定します。このプロパティは、QSizeF 型の値を持ちます。
【初心者向け】Qt GUI で QUndoGroup::canRedo() を使ってやり直し操作を理解しよう!
QUndoGroup::canRedo() は、Qt GUI における やり直し 操作が可能かどうかを判断するための関数です。QUndoGroup クラスは、複数の QUndoCommand オブジェクトをグループ化し、一括操作を可能にするものです。canRedo() 関数は、このグループ内にやり直せるコマンドが存在するかどうかを確認します。
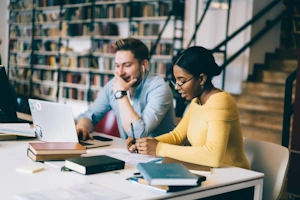
QSupportedWritingSystems::QSupportedWritingSystems() 関数のサンプルコード
QSupportedWritingSystems::QSupportedWritingSystems() は、Qt GUI アプリケーションで使用されるテキスト入力システム (TIS) に関する情報を提供する関数です。この関数は、特定のロケールや言語でサポートされている書記体系の一覧を取得するために使用できます。
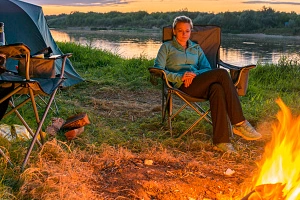
Qt GUIプログラミングにおけるVulkanスワップチェーンイメージビュー:応用例とベストプラクティス
QVulkanWindow::swapChainImageView()関数は、Vulkanスワップチェーンイメージに対応するイメージビューを取得するために使用されます。イメージビューは、シェーダープログラムでテクスチャとしてサンプリングしたり、レンダリングターゲットとして使用したりするために必要なオブジェクトです。
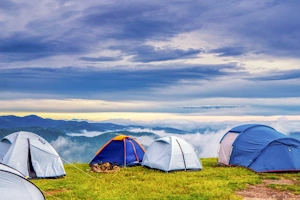
Qt GUI で複雑な変形を効率的に適用する方法: QTransform::operator/=() の仕組みと応用例
Qt GUI における QTransform::operator/=() は、2D 変換行列を別の行列で除算する演算子です。これは、スケーリング、回転、移動などの操作を組み合わせた複雑な変形を効率的に適用するために使用されます。演算子の概要
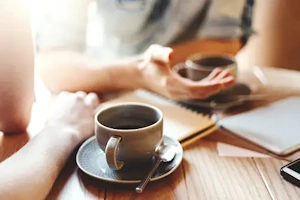
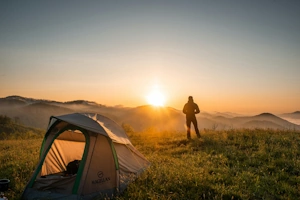
Qt GUI チュートリアル:QVector3D::operator+=() を使用して 3Dベクトルを加算する
使用方法この例では、v1 と v2 という 2つの QVector3D オブジェクトが定義されています。v1 += v2 という式は、v1 の各成分に v2 の対応する成分を加算します。結果として、v1 は (5.0f, 7.0f, 9.0f) という新しいベクトルになります。
ドラッグ中のウィジェットがどのモニターに入ったかを検知:QWidget::screen()関数でマルチモニター対応を強化
QWidget::screen()関数は、ウィジェットが属する画面情報を取得するために使用します。これは、マルチモニター環境でのウィジェット配置や、画面解像度に応じたウィジェットサイズ調整などに役立ちます。機能ウィジェットが属する画面オブジェクトへのポインタを返します。
Qt Widgetsで柔軟なデータマッピング:QDataWidgetMapper::setItemDelegate()の使い方
QDataWidgetMapper::setItemDelegate()は、モデル内のデータとウィジェット間のマッピングに柔軟性と高度なカスタマイズ性を追加する強力な関数です。この関数は、個々のウィジェットに独自のデリゲートを設定することで、データ表示と編集の処理をより細かく制御できます。
Qt GUIにおけるQOpenGLExtraFunctions::glGetProgramInterfaceiv()解説
QOpenGLExtraFunctions::glGetProgramInterfaceiv() は、OpenGLプログラムインターフェースに関する情報を取得するための関数です。Qt GUIでOpenGLを使用する際、プログラムオブジェクトやシェーダーオブジェクトの情報取得に役立ちます。
Qt GUI の QOffscreenSurface::surfaceType() 関数とは?
QOffscreenSurface::surfaceType() は、Qt GUI フレームワークにおけるオフスクリーンサーフェス QOffscreenSurface の種類を取得する関数です。この関数は、オフスクリーンレンダリングや OpenGL コンテキストの管理など、Qt GUI の高度な機能を使用する際に役立ちます。