Qt WidgetsにおけるQTreeView::rowsInserted()の徹底解説
Qt WidgetsにおけるQTreeView::rowsInserted()の詳細解説
rowsInserted()シグナルの概要
シグナルの役割:
- モデルに新しい行が挿入されたことをビューに通知する
シグナルの引数:
parentIndex
: 新しい行が挿入された親インデックスstart
: 挿入された最初の行のインデックスend
: 挿入された最後の行のインデックス
シグナルの接続方法:
QObject::connect(model, &QAbstractItemModel::rowsInserted,
view, &QTreeView::onRowsInserted);
void QTreeView::onRowsInserted(const QModelIndex &parentIndex,
int start, int end) {
// 新しい行に対する処理を実装
}
シグナルの処理例
例1: 新しい行にウィジェットを追加する
void QTreeView::onRowsInserted(const QModelIndex &parentIndex,
int start, int end) {
for (int i = start; i <= end; ++i) {
QModelIndex index = model->index(i, 0, parentIndex);
// 新しい行にウィジェットを追加する処理
}
}
例2: ツリービューを更新する
void QTreeView::onRowsInserted(const QModelIndex &parentIndex,
int start, int end) {
// ツリービューを更新する処理
view->update(parentIndex);
}
- シグナルはモデル側から発せられるため、ビュー側から直接呼び出すことはできません。
- シグナルは挿入された行のインデックス情報などを提供します。
- シグナル処理内でモデルを変更する場合は、適切なロック機構を用いる必要があります。
rowsInserted()シグナルは、モデルに新しい行が挿入された時に発生するシグナルです。このシグナルを利用することで、ツリービューを更新したり、新しい行にウィジェットを追加したりすることができます。
Qt WidgetsにおけるQTreeView::rowsInserted()のサンプルコード
例1: 新しい行にウィジェットを追加する
#include <QtWidgets>
class MyModel : public QAbstractItemModel {
Q_OBJECT
public:
MyModel() {}
int rowCount(const QModelIndex &parent = QModelIndex()) const override {
return 10;
}
int columnCount(const QModelIndex &parent = QModelIndex()) const override {
return 1;
}
QVariant data(const QModelIndex &index, int role) const override {
if (role == Qt::DisplayRole) {
return QString("Item %1").arg(index.row());
}
return QVariant();
}
bool insertRows(int row, int count, const QModelIndex &parent = QModelIndex()) override {
beginInsertRows(parent, row, row + count - 1);
for (int i = 0; i < count; ++i) {
emit rowsInserted(parent, row + i, row + i);
}
endInsertRows();
return true;
}
};
class MyView : public QTreeView {
Q_OBJECT
public:
MyView() {
setModel(new MyModel());
}
protected:
void onRowsInserted(const QModelIndex &parentIndex,
int start, int end) override {
for (int i = start; i <= end; ++i) {
QModelIndex index = model()->index(i, 0, parentIndex);
// 新しい行にウィジェットを追加する処理
QLineEdit *lineEdit = new QLineEdit(this);
lineEdit->setText(QString("Line Edit %1").arg(i));
setItemWidget(index, lineEdit);
}
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
MyView view;
view.show();
return app.exec();
}
例2: ツリービューを更新する
#include <QtWidgets>
class MyModel : public QAbstractItemModel {
Q_OBJECT
public:
MyModel() {}
int rowCount(const QModelIndex &parent = QModelIndex()) const override {
return 10;
}
int columnCount(const QModelIndex &parent = QModelIndex()) const override {
return 1;
}
QVariant data(const QModelIndex &index, int role) const override {
if (role == Qt::DisplayRole) {
return QString("Item %1").arg(index.row());
}
return QVariant();
}
bool insertRows(int row, int count, const QModelIndex &parent = QModelIndex()) override {
beginInsertRows(parent, row, row + count - 1);
for (int i = 0; i < count; ++i) {
emit rowsInserted(parent, row + i, row + i);
}
endInsertRows();
return true;
}
};
class MyView : public QTreeView {
Q_OBJECT
public:
MyView() {
setModel(new MyModel());
}
protected:
void onRowsInserted(const QModelIndex &parentIndex,
int start, int end) override {
// ツリービューを更新する処理
update(parentIndex);
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
MyView view;
view.show();
// 新しい行を挿入
MyModel *model = view.model
Qt WidgetsにおけるQTreeView::rowsInserted()の代替方法
QAbstractItemModel::dataChanged()シグナルを使用する
void QTreeView::onDataChanged(const QModelIndex &topLeft,
const QModelIndex &bottomRight,
const QVector<int> &roles) {
// 変更された行をチェック
for (int row = topLeft.row(); row <= bottomRight.row(); ++row) {
if (model->hasChildren(model->index(row, 0))) {
// 子を持つ行は新しい行として処理
// ...
}
}
}
この方法では、変更されたすべての行をチェックする必要があるため、rowsInserted()シグナルよりも処理が重くなります。
QAbstractItemView::modelChanged()シグナルを使用する
QAbstractItemView::modelAboutToBeChanged()シグナルとQAbstractItemView::modelChanged()シグナルの組み合わせを使用することができます。modelAboutToBeChanged()シグナルは、モデルが変更される前に発生し、modelChanged()シグナルは、モデルが変更された後に発生します。
void QTreeView::onModelAboutToBeChanged(const QModelIndex &topLeft,
const QModelIndex &bottomRight) {
// 変更前のモデルを保存
m_previousModel = model()->data(topLeft, Qt::DisplayRole);
}
void QTreeView::onModelChanged(const QModelIndex &topLeft,
const QModelIndex &bottomRight) {
// 変更後のモデルと比較
for (int row = topLeft.row(); row <= bottomRight.row(); ++row) {
if (model->hasChildren(model->index(row, 0))) {
// 子を持つ行は新しい行として処理
// ...
}
}
}
この方法では、変更された行のみをチェックする必要があるため、rowsInserted()シグナルよりも処理が軽くなります。
カスタムモデルを使用する
独自のモデルクラスを作成し、rowsInserted()シグナルを独自に実装することができます。
class MyModel : public QAbstractItemModel {
Q_OBJECT
public:
MyModel() {}
int rowCount(const QModelIndex &parent = QModelIndex()) const override {
return 10;
}
int columnCount(const QModelIndex &parent = QModelIndex()) const override {
return 1;
}
QVariant data(const QModelIndex &index, int role) const override {
if (role == Qt::DisplayRole) {
return QString("Item %1").arg(index.row());
}
return QVariant();
}
bool insertRows(int row, int count, const QModelIndex &parent = QModelIndex()) override {
beginInsertRows(parent, row, row + count - 1);
for (int i = 0; i < count; ++i) {
emit rowsInserted(parent, row + i, row + i);
// 独自の処理
}
endInsertRows();
return true;
}
};
この方法は、最も柔軟な方法ですが、最も複雑な方法でもあります。
- 処理速度が重要であれば、modelAboutToBeChanged()シグナルとmodelChanged()シグナルを使用する方が良いでしょう。
- 柔軟性が重要であれば、カスタムモデルを使用する方が良いでしょう。
rowsInserted()シグナルは、モデルに新しい行が挿入された時に発生する便利なシグナルです。しかし、場合によっては他の方法の方が
Qt GUIにおけるQUndoCommand::mergeWith()とは?
QUndoCommand::mergeWith() は、Qt GUIにおけるUndo/Redo機能をサポートするクラス QUndoCommand のメソッドの一つです。このメソッドは、2つの QUndoCommand オブジェクトが同じ操作を表しているかどうかを判断し、その場合はそれらを1つのコマンドに統合します。これにより、Undo/Redo履歴をより効率的に管理し、メモリ使用量を削減することができます。
Qt GUIと3D UIの統合:Qt 3D Studio、Qt Widgets、Qt Quick
Qt GUIは、C++向けのクロスプラットフォームなGUI開発フレームワークとして広く利用されています。近年、3D技術は様々な分野で活用されており、Qt GUIでも3Dレンダリング機能が強化されています。本ガイドでは、Qt GUIにおける3Dレンダリングの概要、主要なライブラリ、レンダリングエンジンの選択、3Dシーンの作成、アニメーション、ユーザーインターフェースとの統合など、3Dレンダリングに必要な知識を詳細に解説します。
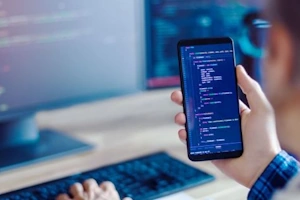
Qt GUIプログラミングの秘訣!QWindow::setFlag()でウィンドウを思い通りにカスタマイズ
この解説では、QWindow::setFlag()関数について、以下の内容を詳細に説明します。QWindow::setFlag()関数の役割: ウィンドウの動作やスタイルを制御するためのフラグを設定する関数設定可能なフラグの種類: ウィンドウタイプ、フレームスタイル、装飾、表示オプションなど、多様なフラグ
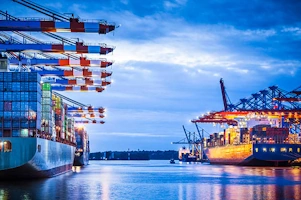
Qt GUI プログラミング: QUndoGroup::createUndoAction() で元に戻す機能を実装
QUndoGroup::createUndoAction() メソッドは、現在アクティブな QUndoStack に対する "元に戻す" アクションを作成します。このアクションは、QAction クラスの派生クラスであり、以下の機能を提供します:
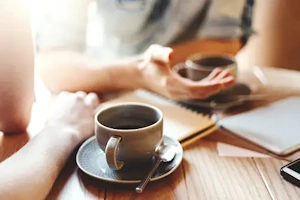
Qt GUIで入力エラーを防ぐ:QValidatorの使い方
概要QValidator::~QValidator() は、Qt GUIにおける入力検証クラス QValidator のデストラクタ関数です。この関数は、QValidator オブジェクトが破棄されるときに自動的に呼び出され、オブジェクトが占有していたメモリなどのリソースを解放します。
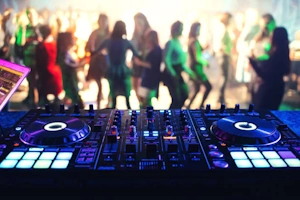
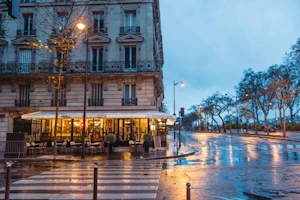
Qt GUI アプリケーションにおけるアクセシビリティ機能: QAccessibleInterface::setText() の詳細解説
QAccessibleInterface::setText()は、Qt GUIアプリケーションにおけるアクセシビリティ機能を提供するための重要な関数です。この関数は、視覚障碍者などのユーザーがスクリーンリーダーなどの支援技術を使用してアプリケーションを操作できるように、ウィジェットのテキスト情報を設定するために使用されます。
Qt GUIにおけるQTextDocument::documentLayout()詳解
QTextDocumentは、テキスト、画像、表などを含むリッチテキストドキュメントを表現するクラスです。documentLayout()関数は、ドキュメント内のテキストどのように配置するかを定義するQAbstractTextDocumentLayoutオブジェクトを返します。
Qt Widgetsでアイテムがフォーカスを失った時の処理 - QGraphicsScene::focusOutEvent()の詳細解説
QGraphicsScene::focusOutEvent()は、Qt Widgetsフレームワークで、QGraphicsScene内のアイテムがフォーカスを失ったときに発生するイベントを処理するための仮想関数です。このイベントは、ユーザーがキーボードやマウスを使って別のアイテムにフォーカスを移動させたときなど、さまざまな状況で発生します。
QCalendarWidget::updateCells()で実現!カレンダーの日付、スタイル、属性を動的に変更する
QCalendarWidget::updateCells()関数は、カレンダーウィジェット内の特定の範囲のセルを更新するために使用されます。これは、カレンダーの日付、スタイル、またはその他の属性を変更する必要がある場合に役立ちます。関数宣言
Qt Widgets の QLineEdit::insert() 関数:テキストエディットコントロールにテキストを挿入する
QLineEdit::insert() は、Qt Widgets モジュールで提供される QLineEdit クラスのメンバー関数です。この関数は、テキストエディットコントロールにテキストを挿入するために使用されます。機能QLineEdit::insert() は、以下の機能を提供します。