Django: auth.password_validation.password_validators_help_text_html() の詳細解説
Djangoのdjango.contrib.authモジュールにおけるauth.password_validation.password_validators_help_text_html()の解説
auth.password_validation.password_validators_help_text_html()
は、Djangoのdjango.contrib.auth
モジュールで提供される関数であり、パスワードバリデーションに使用されるすべてのバリデータのヘルプテキストをHTML形式で返します。このヘルプテキストは、ユーザーにパスワード設定時の要件をわかりやすく伝えるために使用されます。
機能
この関数は以下の機能を提供します。
- すべてのパスワードバリデータのヘルプテキストを取得する
- 取得したヘルプテキストをHTML形式に変換する
- 変換したHTML形式のヘルプテキストを返す
使い方
この関数は以下の引数を受け取ります。
password_validators
(省略可能): 使用するパスワードバリデータのリスト。省略した場合、デフォルトのバリデータが使用されます。
この関数は以下の値を返します。
- パスワードバリデータのヘルプテキストをHTML形式で表した文字列
例
from django.contrib.auth.password_validation import password_validators_help_text_html
help_text = password_validators_help_text_html()
print(help_text)
このコードを実行すると、以下のようなHTML形式のヘルプテキストが出力されます。
<ul>
<li>パスワードは少なくとも8文字の長さが必要です。</li>
<li>パスワードには英字と数字を含める必要があります。</li>
<li>パスワードには特殊文字を含める必要があります。</li>
<li>パスワードはよくあるパスワードのリストに含まれていない必要があります。</li>
<li>パスワードはユーザーの名前、電子メールアドレス、その他の個人情報と類似していない必要があります。</li>
</ul>
カスタマイズ
password_validators_help_text_html()
関数は、独自のパスワードバリデータを追加したり、デフォルトのバリデータのヘルプテキストを変更したりすることでカスタマイズできます。
補足
auth.password_validation.password_validators_help_text_html()
関数は、ユーザーに強固なパスワードを設定するように促すための重要なツールです。この関数を活用することで、より安全なWebアプリケーションを構築することができます。
Get HTML help text for default password validators:
from django.contrib.auth.password_validation import password_validators_help_text_html
help_text = password_validators_help_text_html()
print(help_text)
Get HTML help text for custom password validators:
from django.contrib.auth.password_validation import password_validators
from my_custom_validators import MyCustomPasswordValidator
password_validators.extend([MyCustomPasswordValidator()])
help_text = password_validators_help_text_html()
print(help_text)
Customize HTML help text for specific password validators:
from django.contrib.auth.password_validation import password_validators
from django.utils.translation import gettext
password_validators[0].get_help_text = lambda: gettext("My custom password help text")
help_text = password_validators_help_text_html()
print(help_text)
Use HTML help text in a template:
{% extends 'base.html' %}
{% block content %}
<h1>パスワード設定</h1>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<p>{{ password_validators_help_text_html }}</p>
<input type="submit" value="パスワードを設定">
</form>
{% endblock %}
These examples demonstrate how to use auth.password_validation.password_validators_help_text_html()
in various scenarios. By understanding its functionality and customization options, you can effectively inform users about password requirements and enhance the security of your Django applications.
Here are some general examples of different approaches and techniques that you might consider:
-
Alternative approaches to a specific task: If you are trying to solve a particular problem, there might be different ways to approach it. For instance, instead of using a traditional linear regression algorithm for machine learning, you could explore alternative methods like support vector machines, decision trees, or neural networks.
-
Different programming languages or frameworks: For software development, you might choose a different programming language or framework depending on the project's requirements, your expertise, and community support. For example, Python is a popular choice for web development, while Java is often used for enterprise applications.
-
Creative problem-solving techniques: When facing a challenge, you can employ various creative problem-solving techniques to generate new ideas and solutions. These techniques could include brainstorming, mind mapping, lateral thinking, and design thinking.
-
Diversifying perspectives and approaches: It's often beneficial to seek different perspectives and approaches to a problem. This could involve collaborating with people from diverse backgrounds, consulting experts in different fields, or researching alternative viewpoints.
Please provide more specific information about your situation or goal, and I'll be happy to assist you further.
FeedBurnerで簡単フィード配信!Djangoとの連携方法
Djangoでフィードを作成するには、以下の手順を行います。django. contrib. syndication モジュールをインポートする。フィードの内容となるモデルを定義する。フィードクラスを作成する。フィードのURLパターンを設定する。
Django 汎用表示ビューとその他のAPI開発方法の比較
Djangoの汎用表示ビューは、以下の4つの主要なクラスで構成されています。ListView: モデルのオブジェクト一覧を表示します。DetailView: モデルの個別のオブジェクトを表示します。CreateView: モデルの新しいオブジェクトを作成します。
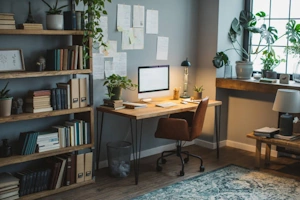
Django組み込みビューとは?
組み込みビューは、Django が提供する事前定義済みのビュー関数です。一般的な CRUD 操作(作成、読み取り、更新、削除)や汎用的な機能を実行するためのビューが用意されており、開発者はこれらのビューを拡張したり、独自のカスタムビューを作成したりして、アプリケーションのニーズに合わせた API を構築することができます。
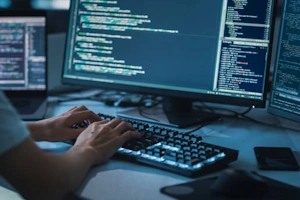
Django テンプレート: 組み込みタグとフィルタを使いこなす
Django テンプレートには、さまざまな機能を提供する多数の組み込みテンプレートタグがあります。以下は、いくつかの主要なテンプレートタグの例です。{% for %} ループ: データのリストを繰り返し処理し、各要素に対してテンプレートの一部をレンダリングします。
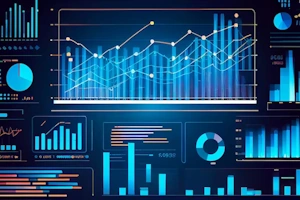
Django フォームフィールド API のサンプルコード
フォームフィールドは、ユーザー入力を受け取るための個別の要素です。名前、メールアドレス、パスワードなど、さまざまな種類のデータに対応できます。主なフォームフィールドの種類:CharField: テキスト入力EmailField: メールアドレス入力
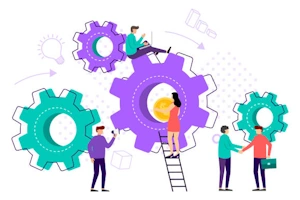

django.views.generic.dates.BaseDateListView.get_dated_queryset() のサンプルコード
django. views. generic. dates. BaseDateListView. get_dated_queryset()は、DjangoのジェネリックビューBaseDateListViewで使用されるメソッドです。このメソッドは、日付ベースのクエリセットを生成し、ビューで使用するために返します。
django.contrib.gis の BaseSpatialField.spatial_index 属性の解説
django. contrib. gis は、Django に空間データ型と空間データベース機能を追加するモジュールです。 gis. db. models. BaseSpatialField. spatial_index は、空間フィールドに空間インデックスを作成するかどうかを制御する属性です。
関連オブジェクト削除からログ記録まで!Django post_delete シグナルの多様な活用例
django. db. models. signals. post_deleteは、Djangoモデルオブジェクトが削除された後に実行されるシグナルです。このシグナルは、モデル削除後の処理を実行するために使用できます。例えば、関連オブジェクトの削除、ログ記録、メール送信などを行うことができます。
テンプレートフィルターで秘密情報を守れ! Django views.debug.SafeExceptionReporterFilter.cleansed_substitute の使い方
django. views. debug. SafeExceptionReporterFilter. cleansed_substitute は、Django のデバッグ機能で利用されるテンプレートフィルターです。役割このフィルターは、エラー発生時に表示されるテンプレート内の敏感な情報をマスクするために使用されます。具体的には、以下の役割を担います。
Djangoの django.core.cache.cache.delete() メソッド完全解説
使い方:パラメータ:key: 削除したいキャッシュエントリのキー。文字列型である必要があります。戻り値:削除が成功した場合は True、存在しないキーを削除しようとした場合は False が返されます。注意事項:delete() メソッドは、デフォルトのキャッシュバックエンドに対してのみ動作します。