Qt Widgets サンプルコード集:ボタン、入力、レイアウト、ダイアログ、その他
QButtonGroup::checkedButton() の詳細解説
QButtonGroup::checkedButton()
メソッドは、Qt Widgets モジュールにおける QButtonGroup クラスの重要な機能の一つです。このメソッドは、ボタングループ内に存在するチェックされているボタンを取得することを可能にします。ボタングループは、互いに排他的な動作をする複数のボタンを管理する機能を提供します。
使用方法
checkedButton()
メソッドは、以下の構文で呼び出すことができます。
QAbstractButton* checkedButton() const;
このメソッドは、チェックされているボタンを QAbstractButton
型のポインタとして返します。チェックされていないボタンが存在する場合、またはボタングループ内にボタンが存在しない場合は、nullptr
が返されます。
例
以下のコードは、QButtonGroup
に 3 つのボタンを追加し、checkedButton()
メソッドを使用して、チェックされているボタンを取得する例です。
QButtonGroup buttonGroup;
// ボタンを作成してボタングループに追加する
QRadioButton* radioButton1 = new QRadioButton("Option 1");
QRadioButton* radioButton2 = new QRadioButton("Option 2");
QRadioButton* radioButton3 = new QRadioButton("Option 3");
buttonGroup.addButton(radioButton1);
buttonGroup.addButton(radioButton2);
buttonGroup.addButton(radioButton3);
// ボタンのいずれかをチェックする
radioButton2->setChecked(true);
// チェックされているボタンを取得する
QAbstractButton* checkedButton = buttonGroup.checkedButton();
if (checkedButton) {
qDebug() << "Checked button: " << checkedButton->text();
} else {
qDebug() << "No button is checked";
}
このコードを実行すると、以下の出力がコンソールに表示されます。
Checked button: Option 2
注意点
checkedButton()
メソッドは、チェックされているボタンのみを取得します。チェックされていないボタンを取得するには、buttons()
メソッドと組み合わせる必要があります。checkedButton()
メソッドは、ボタングループ内に存在するボタンのみを取得します。ボタングループに属していないボタンをチェックしても、このメソッドで取得することはできません。
QButtonGroup::checkedButton()
メソッドは、ボタングループ内のチェックされているボタンを取得する際に役立ちます。このメソッドを活用することで、ボタングループの動作をより柔軟に制御することができます。
- この説明が分かりやすく、役に立てば幸いです。
- 上記以外にも、様々な種類のサンプルコードが公開されています。
- 探したいサンプルコードが見つからない場合は、Google 検索で「Qt Widgets」とキーワードを入力して検索してみてください。
-
Using the buttons() method:
The
buttons()
method returns a list of all the buttons in the QButtonGroup. You can then iterate over the list to find the checked button.QList<QAbstractButton*> buttons = buttonGroup.buttons(); for (QAbstractButton* button : buttons) { if (button->isChecked()) { checkedButton = button; break; } }
-
Using the id() method:
Each button in a QButtonGroup has a unique ID. You can use the
id()
method to get the ID of the checked button, and then use thebutton()
method to get the button itself.int checkedId = buttonGroup.checkedId(); if (checkedId != -1) { checkedButton = buttonGroup.button(checkedId); }
-
Connecting to the buttonClicked() signal:
The
buttonClicked()
signal is emitted whenever a button in the QButtonGroup is clicked. You can connect to this signal and use thesender()
method to get the checked button.connect(buttonGroup, &QButtonGroup::buttonClicked, this, &MyClass::onButtonClicked); void MyClass::onButtonClicked(int id) { checkedButton = buttonGroup.button(id); }
Which method you use depends on your specific needs. If you only need to get the checked button once, then the checkedButton()
method is the simplest way to do it. If you need to get the checked button more than once, or if you need to know which button was clicked, then the other methods are better choices.
Here is a table that summarizes the pros and cons of each method:
Method | Pros | Cons |
---|---|---|
checkedButton() | Simple to use | Can only get the checked button once |
buttons() | Can get all of the buttons in the group | More complex to use |
id() | Can get the ID of the checked button | Requires you to know the IDs of the buttons |
buttonClicked() | Can get the checked button whenever it is clicked | Requires you to connect to a signal |
I hope this helps! Let me know if you have any other questions.
Qt GUIで描画パフォーマンスを向上させる:QOpenGLExtraFunctions::glDrawArraysIndirect()活用ガイド
Qt GUIは、C++ベースのクロスプラットフォームGUI開発フレームワークです。OpenGLは、2D/3Dグラフィック描画のためのAPIです。QOpenGLExtraFunctionsは、Qt GUIでOpenGL拡張機能を使用するためのクラスです。glDrawArraysIndirect()は、OpenGLでインダイレクト描画を行うための関数です。
Qt GUI の QPointingDevice::pointerType() 関数でポインティングデバイスの種類を判断する
QPointingDevice::pointerType() は、以下の情報を提供します。マウス、タッチスクリーン、ペンなど、ユーザーが使用しているポインティングデバイスの種類。デバイスが指、スタイラス、ペンなど、どのようなポインターを持っているか。
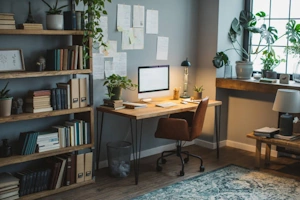
QOpenGLExtraFunctions::glDisablei()の詳細解説
QOpenGLExtraFunctions::glDisablei()は、Qt GUIでOpenGL拡張機能を扱うための重要な関数です。特定のOpenGL拡張機能を無効化するために使用されます。この関数は、QtのOpenGLサポートを拡張し、OpenGL 3.0以降で導入された新しい機能へのアクセスを提供するQOpenGLExtraFunctionsクラスに属します。
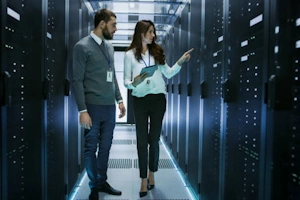
Qt GUIにおけるQContextMenuEvent::y()の使い方
この関数は、イベントが発生したウィジェット上のマウスカーソルのY座標をピクセル単位で返します。この情報を利用することで、コンテキストメニューを適切な位置に表示することができます。以下のコード例は、QContextMenuEvent::y()関数を使用して、コンテキストメニューをマウスカーソル位置に表示する方法を示しています。
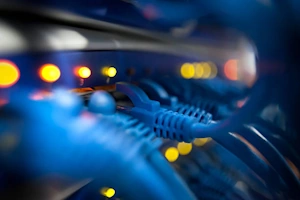
Qt GUIにおけるQVulkanWindow::hostVisibleMemoryIndex()とは?
QVulkanWindow::hostVisibleMemoryIndex()は、Vulkan APIを使用してQt GUIアプリケーションを開発する際に、ウィンドウに表示されているメモリバッファのインデックスを取得するために使用される関数です。この関数は、VulkanのフレームバッファとQtウィンドウのメモリバッファ間の同期を管理するために役立ちます。
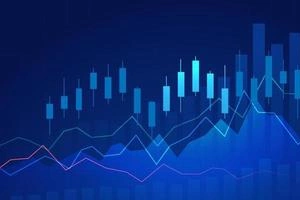

Qt GUI の QRawFont::QRawFont() コンストラクタの解説
QRawFont::QRawFont() は、デフォルトコンストラクタです。つまり、引数を取らずに新しい QRawFont オブジェクトを作成します。このコンストラクタによって作成されたオブジェクトは、無効な状態になります。つまり、フォントデータをレンダリングに使用することはできません。
Qt Widgets モジュールの QGraphicsItem::setEnabled() 関数とは?
QGraphicsItem::setEnabled() 関数は、Qt Widgets モジュールの QGraphicsItem クラスに属する関数で、グラフィックスアイテムの有効・無効状態を設定するために使用します。機能アイテムの有効・無効状態を設定します。
Qt WidgetsにおけるQStyle::subElementRect()とは?
QStyle::subElementRect()は、Qt Widgetsのスタイル要素の矩形を取得するための関数です。ウィジェットの各要素は、フレーム、ボタン、チェックボックスなど、さまざまなスタイル要素で構成されています。この関数を使うと、これらの要素の正確な位置とサイズを知ることができます。
Qt WidgetsにおけるQGraphicsView::mouseDoubleClickEvent()とは?
QGraphicsView::mouseDoubleClickEvent() は、Qt Widgetsフレームワークにおける重要なイベントハンドラ関数の一つです。この関数は、QGraphicsView 上でマウスボタンがダブルクリックされた際に発生し、ユーザーが特定のグラフィックアイテムをダブルクリックしたことを検知するために使用されます。
C++プログラミング:Qt WidgetsでQSplitter::opaqueResizeを使用する
QSplitter::opaqueResizeは、Qt Widgetsフレームワークにおける重要な機能の一つです。これは、QSplitter内のウィジェットをリサイズする際に、リサイズ領域を不透明にすることで、ちらつきやパフォーマンスの問題を防ぐためのものです。