Qt WidgetsのQRadioButton::QRadioButton()とは?
Qt WidgetsのQRadioButton::QRadioButton()徹底解説!
**QRadioButton::QRadioButton()**は、QRadioButtonオブジェクトのコンストラクタです。これは、新しいラジオボタンを作成するために使用されます。このコンストラクタには、いくつかの異なるオーバーロードがあります。
コンストラクタの詳細
デフォルトコンストラクタ
QRadioButton();
このコンストラクタは、デフォルトの値で初期化された新しいQRadioButtonオブジェクトを作成します。デフォルトでは、ラジオボタンはオフ状態になり、テキストは空になります。
親ウィジェットを指定するコンストラクタ
QRadioButton(QWidget *parent = nullptr);
このコンストラクタは、parent
で指定された親ウィジェットを持つ新しいQRadioButtonオブジェクトを作成します。親ウィジェットは、ラジオボタンが配置されるウィジェットです。
テキストと親ウィジェットを指定するコンストラクタ
QRadioButton(const QString &text, QWidget *parent = nullptr);
このコンストラクタは、text
で指定されたテキストとparent
で指定された親ウィジェットを持つ新しいQRadioButtonオブジェクトを作成します。
チェック状態と親ウィジェットを指定するコンストラクタ
QRadioButton(bool checked, QWidget *parent = nullptr);
このコンストラクタは、checked
で指定されたチェック状態とparent
で指定された親ウィジェットを持つ新しいQRadioButtonオブジェクトを作成します。
QRadioButtonオブジェクトには、以下の重要なプロパティがあります。
- text(): ラジオボタンのテキストを取得または設定します。
- isChecked(): ラジオボタンがチェックされているかどうかを取得します。
- setChecked(): ラジオボタンをチェックまたはオフにします。
- setEnabled(): ラジオボタンを有効または無効にします。
QRadioButtonオブジェクトは以下の重要なシグナルを発行します。
- toggled(): ラジオボタンのチェック状態が変化したときに発行されます。
コード例
#include <QtWidgets/QApplication>
#include <QtWidgets/QMainWindow>
#include <QtWidgets/QVBoxLayout>
#include <QtWidgets/QRadioButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// ウィンドウの作成
QMainWindow window;
// 中央ウィジェットの作成
QWidget *centralWidget = new QWidget;
window.setCentralWidget(centralWidget);
// QVBoxLayoutの作成
QVBoxLayout *layout = new QVBoxLayout;
centralWidget->setLayout(layout);
// ラジオボタンの作成
QRadioButton *radioButton1 = new QRadioButton("オプション1");
QRadioButton *radioButton2 = new QRadioButton("オプション2");
// ラジオボタンのレイアウトに追加
layout->addWidget(radioButton1);
layout->addWidget(radioButton2);
// ウィンドウの表示
window.show();
return app.exec();
}
このコードは、2つのラジオボタンを持つシンプルなウィンドウを作成します。ユーザーは、いずれかのラジオボタンを選択することができます。
QRadioButton::QRadioButton()は、Qt Widgetsでラジオボタンを作成するために使用されるコンストラクタです。このコンストラクタには、いくつかの異なるオーバーロードがあり、さまざまなオプションを指定することができます。
この解説が、Qt WidgetsのQRadioButton::QRadioButton()の理解に役立つことを願っています。
Qt WidgetsのQRadioButtonを使ったサンプルコード集
ラジオボタンの状態を取得
#include <QtWidgets/QApplication>
#include <QtWidgets/QMainWindow>
#include <QtWidgets/QVBoxLayout>
#include <QtWidgets/QRadioButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// ウィンドウの作成
QMainWindow window;
// 中央ウィジェットの作成
QWidget *centralWidget = new QWidget;
window.setCentralWidget(centralWidget);
// QVBoxLayoutの作成
QVBoxLayout *layout = new QVBoxLayout;
centralWidget->setLayout(layout);
// ラジオボタンの作成
QRadioButton *radioButton1 = new QRadioButton("オプション1");
QRadioButton *radioButton2 = new QRadioButton("オプション2");
// ラジオボタンのレイアウトに追加
layout->addWidget(radioButton1);
layout->addWidget(radioButton2);
// ラジオボタンの状態を取得
bool isRadioButton1Checked = radioButton1->isChecked();
bool isRadioButton2Checked = radioButton2->isChecked();
// ラジオボタンの状態を出力
qDebug() << "ラジオボタン1のチェック状態:" << isRadioButton1Checked;
qDebug() << "ラジオボタン2のチェック状態:" << isRadioButton2Checked;
// ウィンドウの表示
window.show();
return app.exec();
}
ラジオボタンのチェック状態を設定
#include <QtWidgets/QApplication>
#include <QtWidgets/QMainWindow>
#include <QtWidgets/QVBoxLayout>
#include <QtWidgets/QRadioButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// ウィンドウの作成
QMainWindow window;
// 中央ウィジェットの作成
QWidget *centralWidget = new QWidget;
window.setCentralWidget(centralWidget);
// QVBoxLayoutの作成
QVBoxLayout *layout = new QVBoxLayout;
centralWidget->setLayout(layout);
// ラジオボタンの作成
QRadioButton *radioButton1 = new QRadioButton("オプション1");
QRadioButton *radioButton2 = new QRadioButton("オプション2");
// ラジオボタンのレイアウトに追加
layout->addWidget(radioButton1);
layout->addWidget(radioButton2);
// ラジオボタンのチェック状態を設定
radioButton1->setChecked(true);
// ウィンドウの表示
window.show();
return app.exec();
}
このコードは、2つのラジオボタンを持つウィンドウを作成します。radioButton1
はデフォルトでチェック状態になります。
ラジオボタンの有効/無効設定
#include <QtWidgets/QApplication>
#include <QtWidgets/QMainWindow>
#include <QtWidgets/QVBoxLayout>
#include <QtWidgets/QRadioButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// ウィンドウの作成
QMainWindow window;
// 中央ウィジェットの作成
QWidget *centralWidget = new QWidget;
window.setCentralWidget(centralWidget);
// QVBoxLayoutの作成
QVBoxLayout *layout = new QVBoxLayout;
centralWidget->setLayout(layout);
// ラジオボタンの作成
QRadioButton *radioButton1 = new QRadioButton("オプション1");
QRadioButton *radioButton2 = new QRadioButton("オプション2");
// ラジオボタンのレイアウトに追加
layout->addWidget(radioButton1);
layout->addWidget(radioButton2);
// ラジオボタン1を無効にする
radioButton1->setEnabled(false);
// ウィンドウの表示
window.show();
return app.exec();
}
このコードは、2つのラジオボタンを持つウィンドウを作成します。radioButton1
は無効化され、ユーザーは選択できません。
ラジオボタンのグループ化
#include <QtWidgets/QApplication>
#include <QtWidgets/QMainWindow>
#include <QtWidgets/QVBoxLayout>
#include <QtWidgets/QRadioButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// ウィンドウの作成
QMainWindow window;
// 中央ウィジェットの作成
QWidget *centralWidget = new QWidget;
window.setCentralWidget(centralWidget
Qt WidgetsのQRadioButtonを使うその他の方法
QButtonGroupを使う
#include <QtWidgets/QApplication>
#include <QtWidgets/QMainWindow>
#include <QtWidgets/QVBoxLayout>
#include <QtWidgets/QRadioButton>
#include <QtWidgets/QButtonGroup>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// ウィンドウの作成
QMainWindow window;
// 中央ウィジェットの作成
QWidget *centralWidget = new QWidget;
window.setCentralWidget(centralWidget);
// QVBoxLayoutの作成
QVBoxLayout *layout = new QVBoxLayout;
centralWidget->setLayout(layout);
// QButtonGroupの作成
QButtonGroup *buttonGroup = new QButtonGroup;
// ラジオボタンの作成
QRadioButton *radioButton1 = new QRadioButton("オプション1");
QRadioButton *radioButton2 = new QRadioButton("オプション2");
// ラジオボタンをグループに追加
buttonGroup->addButton(radioButton1);
buttonGroup->addButton(radioButton2);
// ラジオボタンのレイアウトに追加
layout->addWidget(radioButton1);
layout->addWidget(radioButton2);
// ウィンドウの表示
window.show();
return app.exec();
}
このコードは、2つのラジオボタンを持つウィンドウを作成します。2つのラジオボタンは同じグループに属するため、ユーザーは同時に1つだけ選択することができます。
**QRadioButton::toggled()**シグナルは、ラジオボタンのチェック状態が変化したときに発行されます。このシグナルを使って、ラジオボタンの状態変化に応じた処理を行うことができます。
#include <QtWidgets/QApplication>
#include <QtWidgets/QMainWindow>
#include <QtWidgets/QVBoxLayout>
#include <QtWidgets/QRadioButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// ウィンドウの作成
QMainWindow window;
// 中央ウィジェットの作成
QWidget *centralWidget = new QWidget;
window.setCentralWidget(centralWidget);
// QVBoxLayoutの作成
QVBoxLayout *layout = new QVBoxLayout;
centralWidget->setLayout(layout);
// ラジオボタンの作成
QRadioButton *radioButton1 = new QRadioButton("オプション1");
QRadioButton *radioButton2 = new QRadioButton("オプション2");
// ラジオボタンのレイアウトに追加
layout->addWidget(radioButton1);
layout->addWidget(radioButton2);
// ラジオボタンの状態変化に応じた処理
QObject::connect(radioButton1, &QRadioButton::toggled, [](bool checked) {
if (checked) {
// オプション1が選択された処理
} else {
// オプション1が選択解除された処理
}
});
QObject::connect(radioButton2, &QRadioButton::toggled, [](bool checked) {
if (checked) {
// オプション2が選択された処理
} else {
// オプション2が選択解除された処理
}
});
// ウィンドウの表示
window.show();
return app.exec();
}
このコードは、2つのラジオボタンを持つウィンドウを作成します。toggled()
シグナルを使って、ユーザーがどちらかのラジオボタンを選択した
QTextCharFormatとQTextTableFormatを組み合わせて、さらに高度な書式設定
主な機能枠線 スタイル、幅、色を設定スタイル、幅、色を設定余白 上、下、左、右の余白を設定上、下、左、右の余白を設定配置 フレームをページ内での配置フレームをページ内での配置高さ フレームの高さを設定フレームの高さを設定背景 背景色、画像、パターンを設定
Qt GUIにおけるQStandardItem::isAutoTristate():チェックボックス付きアイテムの三状態モードを理解する
三状態モードとは、チェックボックスがオン、オフ、中間の3つの状態を持つことができるモードです。中間状態は、アイテムの状態がまだ決まっていない場合や、部分的に選択されている場合などに使用されます。**QStandardItem::isAutoTristate()**は、以下の状況で役立ちます。
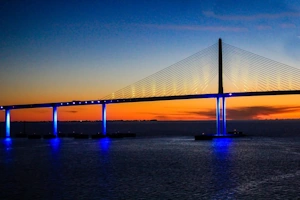
Qt GUIプログラミングにおけるQRegularExpressionValidatorの詳細解説
QRegularExpressionValidator::~QRegularExpressionValidator() は、Qt GUIプログラミングにおいて、正規表現に基づいて入力値の妥当性を検証するクラスである QRegularExpressionValidator のデストラクタです。デストラクタは、オブジェクトが破棄されるときに自動的に呼び出される特殊なメンバ関数であり、オブジェクトが解放する前に必要なクリーンアップ処理を実行します。
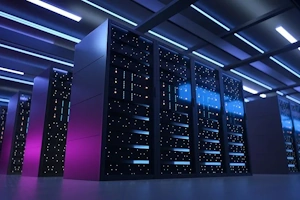
Qt GUIにおけるQTextBlockFormat::setAlignment()の解説
QTextBlockFormat::setAlignment()は、Qt GUIフレームワークでテキストブロックの配置を制御する関数です。テキストブロックとは、テキストエディタなどのウィジェットで一連のテキスト行をまとめて扱うための単位です。
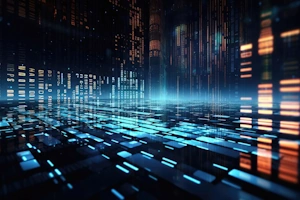
Qt GUI:QTextCharFormat::superScriptBaseline() を使って上付き文字を正確に配置する
QTextCharFormat::superScriptBaseline() は、Qt GUIフレームワークにおけるテキストフォーマット設定に関わる関数です。上付き文字のベースライン位置を制御し、文字配置を調整する際に役立ちます。機能この関数は、上付き文字のベースラインを、通常の文字ベースラインからのオフセット値としてピクセル単位で返します。正の値は上方向へのオフセット、負の値は下方向へのオフセットを表します。
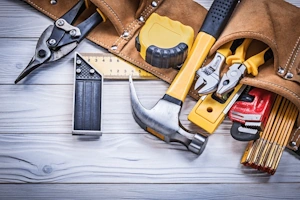
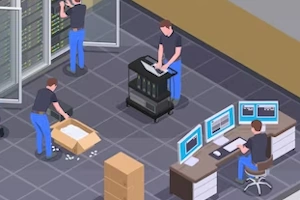
Qt WidgetsにおけるQGraphicsTextItem::inputMethodEvent()の詳細解説
QGraphicsTextItem::inputMethodEvent()は、Qt Widgetsフレームワークにおける重要な関数の一つです。これは、テキスト入力処理に関連するイベントを処理するために使用されます。この関数を理解することで、ユーザー入力に対するテキストアイテムの反応をより細かく制御できるようになります。
QGraphicsSimpleTextItem::opaqueArea() 関数の代替方法
不透明領域とは、テキストアイテム内でレンダリングされる部分の領域を指します。この領域は、テキストの色、フォント、およびその他の属性によって決定されます。QGraphicsSimpleTextItem::opaqueArea() 関数は、QRectF 型のオブジェクトを返します。このオブジェクトは、不透明領域の左上の座標、幅、および高さを表します。
QInputDevice::availableVirtualGeometry()のサンプルコード
QInputDevice::availableVirtualGeometry() は、Qt GUIにおける入力デバイスの仮想デスクトップ上の利用可能領域を取得するための関数です。これは、タッチスクリーンやペンタブレットなどの入力デバイスが仮想デスクトップ上のどの領域にアクセスできるかを判断するために使用されます。
Qt Widgetsでスピンボックスを操作する:QAbstractSpinBox::mousePressEvent() の詳細解説
QAbstractSpinBox::mousePressEvent()は、Qt Widgetsフレームワークにおける重要なイベントハンドラです。スピンボックス内の特定の領域がマウスでクリックされたときに呼び出され、さまざまな操作の実行に使用できます。
Vulkanレンダリングを成功させるためのQt GUI:QVulkanWindow::graphicsQueueFamilyIndex()の役割
概要:機能: Vulkanレンダリング用のグラフィックスキューファミリーのインデックスを取得引数: なし戻り値: グラフィックスキューファミリーのインデックス関連クラス: QVulkanWindow詳細:Vulkanでは、異なる種類の処理を行うための複数のキューファミリーが存在します。QVulkanWindow::graphicsQueueFamilyIndex()関数は、その中でもグラフィックスレンダリングに特化したグラフィックスキューファミリーのインデックスを取得します。