std::basic_string_view vs std::string : どっちを選ぶべき?
C++ の文字列に関連する operator<<(std::basic_string_view) の解説
std::basic_string_view
は、C++20で導入された新しい型で、文字列の参照を表します。これは、従来の std::string
型と異なり、メモリを所有せず、軽量で効率的な操作が可能です。
operator<<(std::basic_string_view)
は、以下の形式で使用されます。
std::ostream& operator<<(std::ostream& os, std::basic_string_view str);
ここで、
os
は、挿入先のストリームを表すstd::ostream
型のオブジェクトです。str
は、挿入する文字列を表すstd::basic_string_view
型のオブジェクトです。
この演算子は、str
の内容を os
に挿入します。具体的には、str
の文字列データが os
に書き込まれます。
例
#include <iostream>
#include <string_view>
int main() {
std::string_view str = "Hello, world!";
std::cout << str << std::endl;
return 0;
}
このコードは、"Hello, world!" という文字列を標準出力 (コンソール) に出力します。
利点
operator<<(std::basic_string_view)
を使用すると、以下の利点があります。
- 効率性:
std::basic_string_view
はメモリを所有しないため、従来のstd::string
型よりも効率的に使用できます。 - 安全性:
std::basic_string_view
は、std::string
型とは異なり、null文字 (\0
) を含む文字列を安全に扱えます。 - 汎用性:
std::basic_string_view
は、std::string
型だけでなく、char*
やconst char*
などの他の文字列型にも使用できます。
operator<<(std::basic_string_view)
は、C++20で導入された新しい演算子で、std::basic_string_view
型のオブジェクトをストリームに挿入するために使用されます。この演算子は、効率性、安全性、汎用性などの利点があります。
C++ で文字列を扱う際は、operator<<(std::basic_string_view)
の使用を検討することをお勧めします。
operator<<(std::basic_string_view) のサンプルコード
標準出力への出力
#include <iostream>
#include <string_view>
int main() {
std::string_view str = "Hello, world!";
std::cout << str << std::endl;
return 0;
}
ファイルへの出力
#include <fstream>
#include <string_view>
int main() {
std::ofstream file("output.txt");
std::string_view str = "This is some text to write to the file.";
file << str << std::endl;
file.close();
return 0;
}
このコードは、"This is some text to write to the file." という文字列を "output.txt" というファイルに書き込みます。
文字列の連結
#include <iostream>
#include <string_view>
int main() {
std::string_view str1 = "Hello, ";
std::string_view str2 = "world!";
std::string_view str3 = str1 + str2;
std::cout << str3 << std::endl;
return 0;
}
このコードは、"Hello, world!" という文字列を標準出力 (コンソール) に出力します。
文字列の比較
#include <iostream>
#include <string_view>
int main() {
std::string_view str1 = "Hello, world!";
std::string_view str2 = "Hello, world!";
if (str1 == str2) {
std::cout << "The strings are equal." << std::endl;
} else {
std::cout << "The strings are not equal." << std::endl;
}
return 0;
}
このコードは、"The strings are equal." という文字列を標準出力 (コンソール) に出力します。
サブ文字列の検索
#include <iostream>
#include <string_view>
int main() {
std::string_view str = "Hello, world!";
std::string_view sub_str = "world";
auto pos = str.find(sub_str);
if (pos != std::string_view::npos) {
std::cout << "The substring \"" << sub_str << "\" was found at position " << pos << std::endl;
} else {
std::cout << "The substring \"" << sub_str << "\" was not found." << std::endl;
}
return 0;
}
このコードは、"The substring "world" was found at position 7" という文字列を標準出力 (コンソール) に出力します。
これらのサンプルコードは、operator<<(std::basic_string_view)
の基本的な使用方法を示しています。詳細については、C++ の標準ライブラリのドキュメントを参照してください。
operator<<(std::basic_string_view) 以外の方法
std::endl
マニピュレータは、文字列をストリームに挿入し、その後改行文字 (\n
) を挿入します。
#include <iostream>
#include <string_view>
int main() {
std::string_view str = "Hello, world!";
std::cout << str << std::endl;
return 0;
}
このコードは、"Hello, world!" という文字列を標準出力 (コンソール) に出力し、その後改行します。
std::stringstream
クラスは、文字列ストリームを表します。このクラスを使用して、文字列をストリームに挿入し、その後ストリームの内容を別のストリームに挿入することができます。
#include <iostream>
#include <sstream>
#include <string_view>
int main() {
std::string_view str = "Hello, world!";
std::stringstream ss;
ss << str;
std::string str2 = ss.str();
std::cout << str2 << std::endl;
return 0;
}
このコードは、"Hello, world!" という文字列を std::stringstream
オブジェクトに挿入し、その後 std::stringstream
オブジェクトの内容を std::string
オブジェクトに挿入します。最後に、std::string
オブジェクトの内容を標準出力 (コンソール) に出力します。
C 標準ライブラリの printf()
や fprintf()
などの関数を使用して、文字列をストリームに挿入することもできます。
#include <stdio.h>
#include <string_view>
int main() {
std::string_view str = "Hello, world!";
printf("%s\n", str);
return 0;
}
このコードは、"Hello, world!" という文字列を標準出力 (コンソール) に出力します。
これらの方法はそれぞれ異なる利点と欠点があります。operator<<(std::basic_string_view)
は最もシンプルで効率的な方法ですが、他の方法の方が汎用性が高い場合があります。
具体的な状況に応じて、最適な方法を選択する必要があります。
C++ の Strings における std::wcslen 関数の詳細解説
std::wcslen 関数の使い方std::wcslen 関数の使い方は非常に簡単です。以下のコード例のように、取得したいワイド文字列の先頭アドレスを関数に渡すだけです。std::wcslen 関数の詳細引数: str: ワイド文字列の先頭アドレス
C++の std::basic_string::push_back を駆使して、C++の文字列操作を自由自在に!
パラメータ:ch: 末尾に追加する文字。charT 型は、std::string が使用する文字型を表します。std::basic_string オブジェクトの内部ストレージに十分なスペースがあるかを確認します。スペースがない場合は、必要に応じてストレージを拡張します。
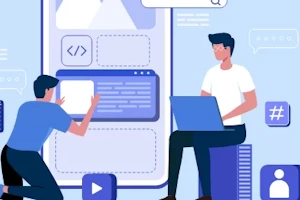
C++ Stringsにおけるstd::basic_string_view::rfind
概要std::basic_string_view::rfind は、部分文字列と検索を開始する位置を受け取り、部分文字列が最後に出現する位置を返します。部分文字列が見つからない場合は、std::basic_string_view::npos が返されます。
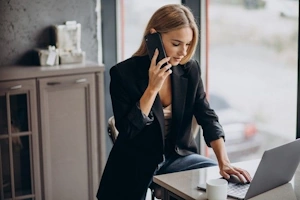
Strings ライブラリを使いこなす:主要メソッドとサンプルコード
C++ では、文字列リテラルは二重引用符で囲まれた文字列として表現されます。例えば、 "Hello, world!" は文字列リテラルです。しかし、C 言語の文字列配列とは異なり、C++ では文字列リテラルは直接変更できません。文字列を編集するには、std::string クラスのオブジェクトを作成する必要があります。

std::basic_string::append_range を選択する際のポイント
std::basic_string::append_rangeは、C++標準ライブラリで提供される関数で、文字列オブジェクトに別の範囲(range)の文字列を追加します。std::stringクラスだけでなく、std::wstringなど他の文字列クラスでも使用できます。
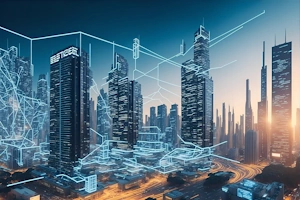
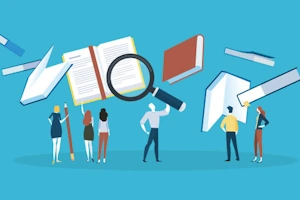
C++のStringsにおけるstd::basic_string::cbeginを使いこなす
機能: std::basic_stringオブジェクトの先頭文字へのconstイテレータを取得戻り値: const_iterator型引数: なし関連する関数: begin(), end(), cend()この例では、strオブジェクトの先頭文字へのconstイテレータを取得し、ループ処理を使って文字列を出力しています。
C++ Strings: std::basic_string::assign_range 関数徹底解説
std::basic_string::assign_range は、C++ 標準ライブラリ std::string クラスのメンバー関数で、指定された範囲の要素を使って文字列の内容を置き換えます。これは、文字列を効率的に初期化または変更したい場合に便利な関数です。
std::basic_string::find_first_ofとfind_first_not_ofの違い
std::basic_string::find_first_of は、C++標準ライブラリで提供される関数の一つで、文字列内における特定の文字列や文字集合の最初の出現位置を検索します。この関数は、文字列操作において非常に便利でよく使用される関数の一つです。
C++のstd::basic_string::assign関数:初心者向けチュートリアル
この関数は、initializer list を受け取り、その内容を std::string に割り当てます。initializer list は、カンマで区切られた値のリストです。assign 関数は、initializer list 以外にも様々なパラメータを受け取ることができます。
C++ Null 終端マルチバイト文字列:基礎から応用まで
概要文字列は連続したバイトの列で表される。各バイトは 1 文字を表す。文字列の最後のバイトはヌル文字('\0')で終端される。ヌル文字は文字列の終わりを示す。マルチバイト文字列は、1 バイト以上のバイトで構成される文字を表すことができる。例