C++ Stringsにおけるstd::basic_string::cend関数
C++のStringsにおけるstd::basic_string::cend
cend関数の役割
cend
関数は、主に以下の2つの役割で使用されます。
- 文字列の末尾を検出する:
- ループ処理などで、文字列の末尾まで処理を行う際に使用します。
- 例えば、以下のコードは、
str
内の全ての文字を反復処理し、それぞれの文字を出力します。
std::string str = "Hello, world!";
for (auto it = str.begin(); it != str.cend(); ++it) {
std::cout << *it;
}
- 文字列の範囲を計算する:
std::distance
関数と組み合わせて、文字列の特定の部分の範囲を計算することができます。- 例えば、以下のコードは、
str
内の最初の5文字の範囲を計算します。
std::string str = "Hello, world!";
auto begin = str.begin();
auto end = str.cend();
auto range = std::distance(begin, end);
std::cout << "The range of the first 5 characters is: " << range << std::endl;
cend関数の戻り値
cend
関数は、const_iterator
型オブジェクトを返します。const_iterator
型オブジェクトは、読み取り専用のイテレータであり、文字列の内容を変更することはできません。
cend関数とend関数の違い
cend
関数とend
関数は、どちらも文字列の末尾を指すイテレータを返します。しかし、cend
関数はconst_iterator型オブジェクトを返し、end関数は**iterator型オブジェクトを返します。
iterator
型オブジェクトは、読み書き可能なイテレータであり、文字列の内容を変更することができます。一方、const_iterator
型オブジェクトは、読み取り専用であり、文字列の内容を変更することはできません。
cend関数の使用例
以下に、cend
関数の使用例をいくつか示します。
// 文字列の末尾を検出する
std::string str = "Hello, world!";
for (auto it = str.begin(); it != str.cend(); ++it) {
std::cout << *it;
}
// 文字列の範囲を計算する
std::string str = "Hello, world!";
auto begin = str.begin();
auto end = str.cend();
auto range = std::distance(begin, end);
std::cout << "The range of the first 5 characters is: " << range << std::endl;
// 文字列の末尾に文字を追加する
std::string str = "Hello, world!";
str.push_back('!');
std::cout << str << std::endl;
// 文字列の末尾の文字を取得する
std::string str = "Hello, world!";
char last_char = *str.cend() - 1;
std::cout << "The last character is: " << last_char << std::endl;
まとめ
std::basic_string::cend
関数は、C++標準ライブラリで提供される文字列クラスstd::basic_string
のメンバ関数です。この関数は、文字列の末尾の次の位置を指すconst_iterator
型オブジェクトを返します。
cend
関数は、文字列の末尾を検出したり、文字列の範囲を計算したりするなど、様々な場面で使用することができます。
C++のStringsにおけるstd::basic_string::cendのサンプルコード
文字列の末尾を検出する
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
for (auto it = str.begin(); it != str.cend(); ++it) {
std::cout << *it;
}
std::cout << std::endl;
return 0;
}
文字列の範囲を計算する
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, world!";
auto begin = str.begin();
auto end = str.cend();
auto range = std::distance(begin, end);
std::cout << "The range of the first 5 characters is: " << range << std::endl;
return 0;
}
このコードは、str
内の最初の5文字の範囲を計算します。
文字列の末尾に文字を追加する
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
str.push_back('!');
std::cout << str << std::endl;
return 0;
}
このコードは、str
の末尾に!
文字を追加します。
文字列の末尾の文字を取得する
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
char last_char = *str.cend() - 1;
std::cout << "The last character is: " << last_char << std::endl;
return 0;
}
このコードは、str
の末尾の文字を取得します。
cendとendの違い
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// cend returns a const_iterator
auto it1 = str.cend();
// end returns an iterator
auto it2 = str.end();
// it1 cannot be used to modify the string
// *it1 = '!'; // error: cannot assign to a variable of const type
// it2 can be used to modify the string
*it2 = '!';
std::cout << str << std::endl;
return 0;
}
このコードは、cend
とend
の違いを示しています。cend
はconst_iterator
型オブジェクトを返し、end
はiterator
型オブジェクトを返します。
const_iterator
型オブジェクトは読み取り専用であり、文字列の内容を変更することはできません。一方、iterator
型オブジェクトは読み書き可能であり、文字列の内容を変更することができます。
- 文字列を逆順に反復処理する
- 文字列内の特定の文字列を検索する
- 文字列を部分文字列に分割する
- 文字列を大文字または小文字に変換する
これらのサンプルコードは、インターネット上やC++の教科書で簡単に見つけることができます。
std::basic_string::cend
関数は、文字列の末尾の次の位置を指すconst_iterator
型オブジェクトを返します。この関数は、文字列の末尾を検出したり、文字列の範囲を計算したりするなど、様々な場面で使用することができます。
C++のStringsにおけるstd::basic_string::cendの代替方法
std::string::size()関数とstd::string::begin()関数
std::string::size()
関数は、文字列の長さを返します。std::string::begin()
関数は、文字列の先頭の位置を指すiterator
型オブジェクトを返します。これらの2つの関数を組み合わせて、文字列の末尾を取得することができます。
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
auto it = str.begin() + str.size();
std::cout << *it << std::endl;
return 0;
}
このコードは、str
の末尾の文字を出力します。
std::string::rbegin()関数とstd::string::rend()関数
std::string::rbegin()
関数は、文字列の末尾を指すreverse_iterator
型オブジェクトを返します。std::string::rend()
関数は、文字列の先頭を指すreverse_iterator
型オブジェクトを返します。これらの2つの関数を組み合わせて、文字列の範囲を取得することができます。
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, world!";
auto begin = str.rbegin();
auto end = str.rend();
auto range = std::distance(begin, end);
std::cout << "The range of the last 5 characters is: " << range << std::endl;
return 0;
}
このコードは、str
の最後の5文字の範囲を計算します。
独自のイテレータクラスを作成して、文字列の末尾を指すようにすることもできます。
#include <iostream>
#include <string>
class StringIterator {
public:
StringIterator(const std::string& str) : str_(str), pos_(str.size()) {}
bool operator!=(const StringIterator& other) const {
return pos_ != other.pos_;
}
void operator++() {
++pos_;
}
char operator*() const {
return str_[pos_];
}
private:
const std::string& str_;
size_t pos_;
};
int main() {
std::string str = "Hello, world!";
StringIterator it(str);
while (it != StringIterator()) {
std::cout << *it;
++it;
}
std::cout << std::endl;
return 0;
}
このコードは、str
内の全ての文字を反復処理し、それぞれの文字を出力します。
std::basic_string::cend
関数以外にも、文字列の末尾を取得したり、文字列の範囲を計算したりする方法はいくつかあります。どの方法を使用するかは、状況によって異なります。
C++ Strings で std::basic_string::npos を使用したサンプルコード
npos の意味最大値: npos は、size_t 型で表現可能な最大値に設定されています。文字列の終端: find() や find_first_of() などの関数で npos を引数として渡すと、文字列の終端まで検索することを意味します。
std::basic_string::find_first_ofとfind_first_not_ofの違い
std::basic_string::find_first_of は、C++標準ライブラリで提供される関数の一つで、文字列内における特定の文字列や文字集合の最初の出現位置を検索します。この関数は、文字列操作において非常に便利でよく使用される関数の一つです。
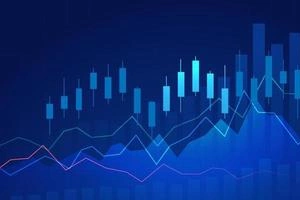
質問:C++で「std::basic_string_view::size」関数を用いて文字列の長さを取得する方法
概要std::basic_string_view::size 関数は、std::basic_string_view オブジェクトが保持する文字列の長さを取得します。これは、文字列の要素数に相当します。戻り値この関数は、保持している文字列の長さを size_type 型で返します。size_type 型は、文字列の長さを表現するために使用される符号なし整数型です。
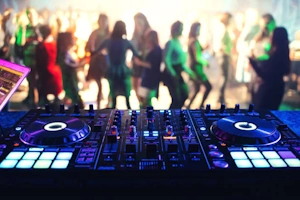
C++ストリングクラスにおけるstd::basic_string::find_first_not_of関数:詳細解説とサンプルコード
std::basic_string::find_first_not_of関数は、指定された文字列または文字範囲内で、最初の除外文字が現れる位置を検索します。除外文字とは、検索対象文字列に含まれていない文字のことです。詳細解説関数宣言引数s: 除外文字列または文字範囲の先頭ポインタ
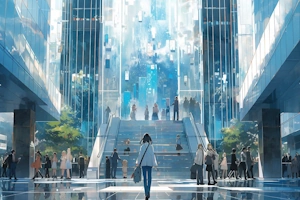
Strings ライブラリを使いこなす:主要メソッドとサンプルコード
C++ では、文字列リテラルは二重引用符で囲まれた文字列として表現されます。例えば、 "Hello, world!" は文字列リテラルです。しかし、C 言語の文字列配列とは異なり、C++ では文字列リテラルは直接変更できません。文字列を編集するには、std::string クラスのオブジェクトを作成する必要があります。

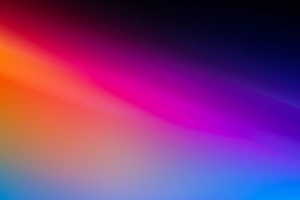
C++の文字列操作をマスターしよう! std::basic_string::capacity 関数徹底解説
メモリ確保と効率性std::basic_string は、動的にメモリを確保して文字列を格納します。文字列に追加や削除を行うたびに、必要に応じてメモリ領域を再割り当てします。しかし、メモリ再割り当ては処理速度の低下を招きます。capacity 関数は、メモリ再割り当てを減らし、コードの効率性を向上させるために役立ちます。
C++ Stringsで空白**以外**の最後の文字を見つける:find_last_not_of関数
この関数は、以下の2つの重要な役割を果たします。特定の文字列以外の最後の文字を見つける検索対象となる文字列をstr、検索対象となる文字列をnot_ofとします。find_last_not_of関数は、strの中でnot_ofに含まれない最後の文字の位置を返します。
C++ Strings: std::basic_string::assign_range 関数徹底解説
std::basic_string::assign_range は、C++ 標準ライブラリ std::string クラスのメンバー関数で、指定された範囲の要素を使って文字列の内容を置き換えます。これは、文字列を効率的に初期化または変更したい場合に便利な関数です。
std::basic_string::back以外の最後の文字取得方法:at(), operator[], イテレータなど
概要機能: 文字列の最後の文字への参照を返す戻り値: 最後の文字への参照引数: なし使用例:詳細std::basic_string::back は、文字列クラス std::basic_string のメンバー関数です。この関数は、文字列の最後の文字への参照を返します。
std::basic_string::find_first_ofとfind_first_not_ofの違い
std::basic_string::find_first_of は、C++標準ライブラリで提供される関数の一つで、文字列内における特定の文字列や文字集合の最初の出現位置を検索します。この関数は、文字列操作において非常に便利でよく使用される関数の一つです。