C++ Strings で std::basic_string::npos を使用したサンプルコード
C++ の Strings における std::basic_string::npos の役割
npos の意味
- 最大値:
npos
は、size_t
型で表現可能な最大値に設定されています。 - 文字列の終端:
find()
やfind_first_of()
などの関数でnpos
を引数として渡すと、文字列の終端まで検索することを意味します。 - 検索失敗:
find()
やfind_first_of()
などの関数が検索対象を見つけられなかった場合、npos
を返します。
npos の使い方
文字列の終端を表す
std::string str = "Hello, world!";
// 文字列 "world" の位置を取得
size_t pos = str.find("world");
// "world" が見つかった場合は、その位置を出力
if (pos != std::basic_string::npos) {
std::cout << "Found at position " << pos << std::endl;
} else {
std::cout << "Not found" << std::endl;
}
検索失敗を表す
std::string str = "Hello, world!";
// 文字列 "xyz" の位置を取得
size_t pos = str.find("xyz");
// "xyz" は見つからないので、npos が返される
if (pos == std::basic_string::npos) {
std::cout << "Not found" << std::endl;
}
その他の用途
npos
は、文字列操作関数の引数としてだけでなく、さまざまな状況で使用できます。例えば、文字列の長さを比較したり、空の文字列かどうかをチェックしたりするのに役立ちます。
std::basic_string::npos
は、C++ の Strings における重要な概念です。その意味と使い方を理解することで、より効率的で正確なコードを書くことができます。
std::basic_string::npos のサンプルコード
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列 "world" の位置を取得
size_t pos = str.find("world");
// "world" が見つかった場合は、その位置を出力
if (pos != std::basic_string::npos) {
std::cout << "Found at position " << pos << std::endl;
} else {
std::cout << "Not found" << std::endl;
}
return 0;
}
検索失敗
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列 "xyz" の位置を取得
size_t pos = str.find("xyz");
// "xyz" は見つからないので、npos が返される
if (pos == std::basic_string::npos) {
std::cout << "Not found" << std::endl;
}
return 0;
}
文字列の長さの比較
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "World";
// str1 の長さが str2 より長い場合は "str1 is longer" を出力
if (str1.length() > str2.length()) {
std::cout << "str1 is longer" << std::endl;
} else {
std::cout << "str2 is longer" << std::endl;
}
return 0;
}
空の文字列かどうかチェック
#include <iostream>
#include <string>
int main() {
std::string str = "";
// str が空の文字列であれば "str is empty" を出力
if (str.empty()) {
std::cout << "str is empty" << std::endl;
} else {
std::cout << "str is not empty" << std::endl;
}
return 0;
}
文字列の連結
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "World";
// str1 と str2 を連結して "Hello World" を出力
std::string str3 = str1 + " " + str2;
std::cout << str3 << std::endl;
return 0;
}
部分文字列の検索
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列 "world" の位置を取得
size_t pos = str.find("world");
// "world" が見つかった場合は、その位置を出力
if (pos != std::basic_string::npos) {
std::cout << "Found at position " << pos << std::endl;
} else {
std::cout << "Not found" << std::endl;
}
return 0;
}
部分文字列の置換
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列 "world" を "universe" で置換
str.replace(pos, str.length() - pos, "universe");
// 結果: "Hello, universe!"
std::cout << str << std::endl;
return 0;
}
文字列の大文字・小文字変換
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// str をすべて小文字に変換
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
// 結果: "hello, world!"
std::cout << str << std::endl;
return 0;
}
**9. 文字列の分割
std::basic_string::npos を使用するその他の方法
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列 "world" が範囲内に収まるかどうかをチェック
if (pos + str.length() <= str.size()) {
// 範囲内に収まる
} else {
// 範囲外
}
return 0;
}
文字列の比較
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "World";
// str1 と str2 が同じかどうかをチェック
if (str1.compare(str2) == 0) {
// 同じ
} else {
// 異なる
}
return 0;
}
文字列のフォーマット
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, %s!";
// フォーマット文字列を使用して、"world" を挿入
std::string formatted_str = str.format("world");
// 結果: "Hello, world!"
std::cout << formatted_str << std::endl;
return 0;
}
文字列のサブ文字列の取得
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列 "world" をサブ文字列として取得
std::string substring = str.substr(pos, str.length() - pos);
// 結果: "world!"
std::cout << substring << std::endl;
return 0;
}
文字列のトリミング
#include <iostream>
#include <string>
int main() {
std::string str = " Hello, world! ";
// 文字列の両端の空白文字を削除
str.trim();
// 結果: "Hello, world!"
std::cout << str << std::endl;
return 0;
}
文字列への文字の追加
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
// 文字列の末尾に '!' を追加
str.push_back('!');
// 結果: "Hello!"
std::cout << str << std::endl;
return 0;
}
文字列への文字列の追加
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = " world!";
// str1 の末尾に str2 を追加
str1.append(str2);
// 結果: "Hello world!"
std::cout << str1 << std::endl;
return 0;
}
文字列の削除
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列 "world" を削除
str.erase(pos, str.length() - pos);
// 結果: "Hello"
std::cout << str << std::endl;
return 0;
}
文字列の挿入
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
// 文字列 "world" を "Hello" の後に挿入
str.insert(pos, "world");
// 結果: "Hello world!"
std::cout << str << std::endl;
return 0;
}
文字列の逆順
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列を逆順にする
std::reverse(str.begin(), str.end());
// 結果: "!dlrow ,olleH"
std::cout
C++ Strings の魔法使い:std::stoi で文字列を整数に変換する
この解説では、std::stoi の使い方を分かりやすく説明し、さらにその仕組みや注意点についても詳しく掘り下げていきます。std::stoi は、string 型の文字列を受け取り、それを int 型の整数に変換する関数です。使い方はとても簡単で、以下のコードのように記述します。
C++ Stringsにおけるstd::basic_string::substr
std::basic_string::substrは以下の形式で呼び出されます。pos: 部分文字列の開始位置を指定する整数値です。len: 部分文字列の長さを指定する整数値です。例上記の例では、strの7番目から6文字分の部分文字列を取得し、substr変数に格納しています。
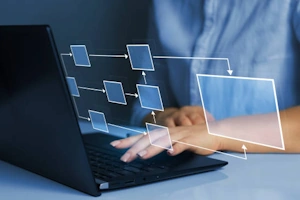
Strings ライブラリを使いこなす:主要メソッドとサンプルコード
C++ では、文字列リテラルは二重引用符で囲まれた文字列として表現されます。例えば、 "Hello, world!" は文字列リテラルです。しかし、C 言語の文字列配列とは異なり、C++ では文字列リテラルは直接変更できません。文字列を編集するには、std::string クラスのオブジェクトを作成する必要があります。

std::wcstol 関数を使いこなして、C++ プログラミングをレベルアップ!
std::wcstol は、以下の引数を受け取ります。str: 変換対象となるワイド文字列へのポインターstr_end: 変換が終了した後の文字列へのポインター (省略可能)base: 数値の基数 (省略時は 10)この関数は、str で指定されたワイド文字列を解析し、指定された基数に基づいて長整型値に変換します。変換が成功すると、変換結果が返されます。変換が失敗した場合、0 が返されます。
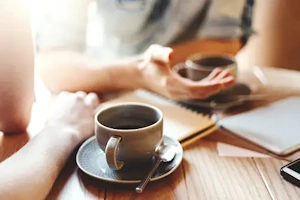
std::wstring_convertクラス:std::wcsrtombs関数のより安全な代替手段
std::wcsrtombs は、ワイド文字列をマルチバイト文字列に変換する関数です。これは、異なる文字エンコーディングを使用するシステム間で文字列データを交換する必要がある場合に役立ちます。機能std::wcsrtombs は以下の機能を提供します。
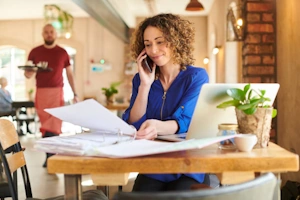
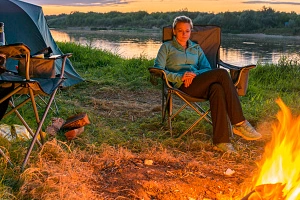
C++ Stringsで空白**以外**の最後の文字を見つける:find_last_not_of関数
この関数は、以下の2つの重要な役割を果たします。特定の文字列以外の最後の文字を見つける検索対象となる文字列をstr、検索対象となる文字列をnot_ofとします。find_last_not_of関数は、strの中でnot_ofに含まれない最後の文字の位置を返します。
std::stoi、std::stol、std::stoull:詳細解説
本解説では、以下の内容を詳細に説明します:std::atoll の概要: 動作 型 ヘッダーファイル 引数 戻り値動作型ヘッダーファイル引数戻り値std::atoll の使用方法: 基本的な例 エラー処理 文字列ストリームとの比較基本的な例
std::basic_string_view::find_last_of の使い方:C++で文字列の最後の出現位置を探す
引数: str: 検索対象となる文字列 pos: 検索を開始する位置(省略可能、デフォルトは文字列末尾)str: 検索対象となる文字列pos: 検索を開始する位置(省略可能、デフォルトは文字列末尾)返値: 見つかった場合は、最後の出現位置 見つからない場合は、std::basic_string_view::npos
C++ で Unicode 文字列を扱う:Null 終端ワイド文字列以外の方法
ワイド文字と Null 終端ワイド文字: Unicode 文字を表現するために使用されるデータ型 (wchar_t)。Null 終端: 文字列の終わりを示す特殊な文字コード (\0)。文字列リテラルワイド文字列リテラルは、L プレフィックスと二重引用符で囲まれた文字列です。例:
std::basic_string::clear を使いこなして C++ プログラミングをレベルアップ!
std::basic_string::clear の概要文字列オブジェクトからすべての文字を削除します。メモリ解放は行われません。容量は変更されない可能性が高いです。すべてのポインタ、参照、イテレータは無効化されます。std::basic_string::clear は、std::string::erase(begin(), end()) と同じように動作します。つまり、文字列の先頭から末尾までのすべての文字が削除されます。