C++ Stringsにおけるstd::basic_string::copy関数
C++のStringsにおけるstd::basic_string::copy関数
std::basic_string::copy
関数は、C++の標準ライブラリで提供されている関数の一つで、文字列オブジェクトの一部を別の文字列バッファにコピーするために使用されます。
機能
- ソース文字列の指定された位置から、指定された長さの文字列をコピーします。
- コピーされた文字列は、ターゲットバッファにヌル文字('\0')で終端されません。
- ターゲットバッファは、コピーされる文字列を格納するのに十分な大きさである必要があります。
構文
size_type copy(char_t* dest, size_type n, size_type pos = 0) const;
引数
dest
: コピー先の文字列バッファへのポインタn
: コピーする文字列の長さpos
: コピーを開始するソース文字列内の位置 (デフォルトは0)
戻り値
- コピーされた文字列の長さ
例
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
char buffer[10];
// 最初の5文字をコピー
str.copy(buffer, 5);
// コピーされた文字列を出力
std::cout << buffer << std::endl;
return 0;
}
この例では、str
オブジェクトの最初の5文字 ("Hello") が buffer
バッファにコピーされます。
注意事項
- ターゲットバッファは、コピーされる文字列を格納するのに十分な大きさである必要があります。
- コピーされた文字列は、ヌル文字で終端されません。必要に応じて、自分でヌル文字を追加する必要があります。
std::basic_string::copy 関数のサンプルコード
#include <iostream>
#include <string>
int main() {
std::string str = "This is a string.";
char buffer[20];
// 文字列 "is" をコピー
str.copy(buffer, 2, 7);
// コピーされた文字列を出力
std::cout << buffer << std::endl;
return 0;
}
出力:
is
文字列の末尾へのコピー
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
char buffer[10] = " world!";
// 文字列 "Hello" を " world!" の末尾に追加
str.copy(buffer + strlen(buffer), str.size());
// 結果を出力
std::cout << buffer << std::endl;
return 0;
}
出力:
Hello world!
ヌル文字の追加
#include <iostream>
#include <string>
int main() {
std::string str = "This is a string.";
char buffer[20];
// 文字列 "This is a string" をコピー
str.copy(buffer, str.size());
// 末尾にヌル文字を追加
buffer[str.size()] = '\0';
// コピーされた文字列を出力
std::cout << buffer << std::endl;
return 0;
}
出力:
This is a string
文字列の連結
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = " world!";
char buffer[20];
// 2つの文字列を連結
str1.copy(buffer, str1.size());
str2.copy(buffer + str1.size(), str2.size());
// 結果を出力
std::cout << buffer << std::endl;
return 0;
}
出力:
Hello world!
文字列の反転
#include <iostream>
#include <string>
int main() {
std::string str = "This is a string.";
char buffer[20];
// 文字列を反転
for (int i = str.size() - 1, j = 0; i >= 0; --i, ++j) {
buffer[j] = str[i];
}
// 結果を出力
std::cout << buffer << std::endl;
return 0;
}
出力:
.gnirts a si sihT
これらのサンプルコードは、std::basic_string::copy
関数のさまざまな使い方を示しています。
std::basic_string::copy関数の代替方法
標準ライブラリの関数を使う
std::strncpy
: 指定された長さの文字列をコピーします。std::strcpy
: 文字列全体をコピーします。
ループを使う
- ソース文字列の各文字をループで処理し、ターゲットバッファにコピーします。
自作の関数を使う
- 独自の要件に合わせて、文字列コピー関数を作成することができます。
それぞれの方法のメリットとデメリット
方法 | メリット | デメリット |
---|---|---|
std::basic_string::copy | 使いやすい | ヌル文字を追加する必要がある |
std::strncpy | ヌル文字を追加する必要がない | 長さを指定する必要がある |
std::strcpy | 使いやすい | ヌル文字を追加する必要がない |
ループ | 柔軟性が高い | コード量が増える |
自作関数 | 独自の要件に合わせられる | コード量が増える |
- 使いやすさを重視する場合は、
std::basic_string::copy
関数を使うのがおすすめです。 - ヌル文字を追加する必要がない場合は、
std::strncpy
関数やstd::strcpy
関数を使うことができます。 - 柔軟性が必要な場合は、ループや自作関数を使うことができます。
// std::strncpyを使う例
#include <iostream>
#include <string.h>
int main() {
char src[] = "This is a string.";
char dest[20];
strncpy(dest, src, sizeof(dest) - 1);
dest[sizeof(dest) - 1] = '\0';
// 結果を出力
std::cout << dest << std::endl;
return 0;
}
// std::strcpyを使う例
#include <iostream>
#include <string.h>
int main() {
char src[] = "This is a string.";
char dest[20];
strcpy(dest, src);
// 結果を出力
std::cout << dest << std::endl;
return 0;
}
// ループを使う例
#include <iostream>
int main() {
std::string str = "This is a string.";
char buffer[20];
// 文字列 "is" をコピー
for (int i = 0, j = 0; i < str.size() && j < sizeof(buffer) - 1; ++i, ++j) {
if (str[i] == ' ') {
continue;
}
buffer[j] = str[i];
}
// 結果を出力
std::cout << buffer << std::endl;
return 0;
}
// 自作関数を使う例
#include <iostream>
std::string copy_string(const std::string& src, size_t n, size_t pos = 0) {
std::string dest;
// ソース文字列の指定された位置から、指定された長さの文字列をコピー
for (int i = pos; i < pos + n && i < src.size(); ++i) {
dest += src[i];
}
return dest;
}
int main() {
std::string str = "This is a string.";
// 文字列 "is" をコピー
std::string dest = copy_string(str, 2, 7);
// 結果を出力
std::cout << dest << std::endl;
return 0;
}
これらのサンプルコードは、std::basic_string::copy
関数以外の方法で文字列をコピーする方法を示しています。
std::wstring_convertクラス:std::wcsrtombs関数のより安全な代替手段
std::wcsrtombs は、ワイド文字列をマルチバイト文字列に変換する関数です。これは、異なる文字エンコーディングを使用するシステム間で文字列データを交換する必要がある場合に役立ちます。機能std::wcsrtombs は以下の機能を提供します。
std::basic_string::dataを使いこなして、C++プログラミングをもっと楽しく!
概要std::basic_string::data は、std::basic_string オブジェクト内の文字列データへのポインタを返します。返されたポインタは、const であり、文字列データの変更はできません。返されたポインタは、std::basic_string オブジェクトの生存期間中は有効です。

C++で文字コード変換をマスターしよう!std::btowcの使い方とサンプルコード
この関数を使うことで、異なるエンコード間で文字列を効率的に変換したり、マルチバイト文字を扱うプログラムを作成することができます。std::btowcは以下の形式で定義されています。c: 変換する単一バイト文字std::wint_t: 変換結果のワイド文字
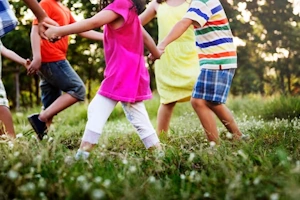
C++の「std::wcstoimax」でワイド文字列を整数に変換:詳細解説とサンプルコード
概要std::wcstoimax は、C++ の標準ライブラリに含まれる関数で、ワイド文字列 (wstring) を指定した基数に基づいて整数値に変換します。これは、std::stoi() 関数のワイド文字列バージョンと考えることができます。

C++ Stringsにおけるstd::basic_string::substr
std::basic_string::substrは以下の形式で呼び出されます。pos: 部分文字列の開始位置を指定する整数値です。len: 部分文字列の長さを指定する整数値です。例上記の例では、strの7番目から6文字分の部分文字列を取得し、substr変数に格納しています。
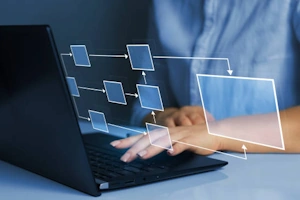
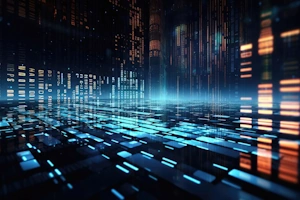
std::basic_string::crbegin関数とstd::reverse_iteratorの比較
std::basic_string::crbegin は、C++ 標準ライブラリで提供されている std::basic_string クラスのメンバ関数です。この関数は、文字列の逆順を指す 読み取り専用 イテレータを返します。つまり、文字列の最後の文字から最初の文字に向かってイテレートすることができます。
C++ std::basic_string::find完全ガイド:部分文字列検索をマスターしよう!
std::basic_string::find 関数は、C++ の std::string クラスにおいて、部分文字列の検索を行うための強力なツールです。この関数は、検索対象となる文字列と、検索開始位置を指定することで、部分文字列が見つかった最初の位置を返します。
C++ の Strings と std::basic_string::basic_string
std::basic_string::basic_string は、std::basic_string クラスのコンストラクタです。文字列を初期化する際に使用します。このコンストラクタは以下の引数を受け取ります。str: コピー元の文字列alloc: 使用するメモリ割り当て器 (省略可能)
C++ プログラマー必見!std::basic_string::empty 関数の詳細解説
概要機能: 文字列が空かどうかを判定戻り値: 空の場合: true 空でない場合: false空の場合: true空でない場合: false引数: なし使用例:動作の詳細empty() 関数は、文字列の length() が 0 かどうかをチェックします。
C++ Stringsにおけるstd::basic_string_view::rfind
概要std::basic_string_view::rfind は、部分文字列と検索を開始する位置を受け取り、部分文字列が最後に出現する位置を返します。部分文字列が見つからない場合は、std::basic_string_view::npos が返されます。