std::basic_string::dataを使いこなして、C++プログラミングをもっと楽しく!
C++のStringsにおけるstd::basic_string::data
概要
std::basic_string::data
は、std::basic_string
オブジェクト内の文字列データへのポインタを返します。- 返されたポインタは、
const
であり、文字列データの変更はできません。 - 返されたポインタは、
std::basic_string
オブジェクトの生存期間中は有効です。 - 空の
std::basic_string
オブジェクトの場合、data()
は空のポインタを返します。
コード例
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列データへのポインタを取得
const char* data = str.data();
// ポインタを使用して文字列を出力
for (int i = 0; i < str.size(); ++i) {
std::cout << data[i];
}
std::cout << std::endl;
return 0;
}
出力例
Hello, world!
詳細
std::basic_string::data
は、const
メンバ関数であるため、std::basic_string
オブジェクトの状態を変更することはできません。- 返されたポインタは、
std::basic_string
オブジェクトの生存期間中は有効です。つまり、std::basic_string
オブジェクトがスコープ外に出ると、ポインタは無効になります。
注意事項
std::basic_string::data
で返されたポインタは、const
であるため、文字列データの変更はできません。
std::basic_string::data のサンプルコード
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列データへのポインタを取得
const char* data = str.data();
// ポインタを使用して文字列を出力
for (int i = 0; i < str.size(); ++i) {
std::cout << data[i];
}
std::cout << std::endl;
return 0;
}
出力例
Hello, world!
文字列データへのポインタを使用して、文字列を比較する
#include <string>
int main() {
std::string str1 = "Hello, world!";
std::string str2 = "Hello, world!";
// 文字列データへのポインタを取得
const char* data1 = str1.data();
const char* data2 = str2.data();
// ポインタを使用して文字列を比較
bool equal = (std::strcmp(data1, data2) == 0);
std::cout << "str1 and str2 are equal: " << std::boolalpha << equal << std::endl;
return 0;
}
出力例
str1 and str2 are equal: true
文字列データへのポインタを使用して、文字列を連結する
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "world!";
// 文字列データへのポインタを取得
const char* data1 = str1.data();
const char* data2 = str2.data();
// ポインタを使用して文字列を連結
size_t len1 = str1.size();
size_t len2 = str2.size();
char* concatenated_str = new char[len1 + len2 + 1];
std::memcpy(concatenated_str, data1, len1);
std::memcpy(concatenated_str + len1, data2, len2);
concatenated_str[len1 + len2] = '\0';
// 連結された文字列を出力
std::cout << concatenated_str << std::endl;
delete[] concatenated_str;
return 0;
}
出力例
Hello, world!
文字列データへのポインタを使用して、文字列を大文字に変換する
#include <string>
#include <cctype>
int main() {
std::string str = "hello, world!";
// 文字列データへのポインタを取得
char* data = str.data();
// ポインタを使用して文字列を大文字に変換
for (int i = 0; i < str.size(); ++i) {
data[i] = std::toupper(data[i]);
}
// 変換された文字列を出力
std::cout << str << std::endl;
return 0;
}
出力例
HELLO, WORLD!
文字列データへのポインタを使用して、文字列からスペースを除去する
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, world!";
// 文字列データへのポインタを取得
char* data = str.data();
// ポインタを使用して文字列からスペースを除去
str.erase(std::remove_if(data, data + str.size(), [](char c) { return c == ' '; }), str.end());
// スペースを除去した文字列を出力
std::cout << str << std::endl;
return 0;
}
出力例
Hello,world!
文字列データへのポインタを使用して、部分文字列を検索する
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, world!";
// 文字列データへのポインタを取得
const char* data = str.data();
// ポインタを使用して部分文字列を検索
auto
std::basic_string::data 以外の方法
文字列データへのアクセス
std::basic_string::operator[]
std::basic_string::at
文字列の操作
std::basic_string::assign
std::basic_string::append
文字列の変換
std::basic_string::to_upper
文字列データへのアクセス
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列データへのアクセス
char c1 = str[0]; // 'H'
char c2 = str.at(1); // 'e'
const char* c_str = str.c_str(); // "Hello, world!"
char c3 = str.front(); // 'H'
char c4 = str.back(); // '!'
std::cout << c1 << std::endl;
std::cout << c2 << std::endl;
std::cout << c_str << std::endl;
std::cout << c3 << std::endl;
std::cout << c4 << std::endl;
return 0;
}
出力例
H
e
Hello, world!
H
!
文字列の操作
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列の操作
str.assign("Goodbye, world!"); // "Goodbye, world!"
str.append("!"); // "Goodbye, world!!"
str.insert(7, " cruel "); // "Goodbye, cruel world!!"
str.erase(7, 6); // "Goodbye, world!!"
str.replace(7, 7, "beautiful"); // "Goodbye, beautiful world!!"
std::cout << str << std::endl;
return 0;
}
出力例
Goodbye, beautiful world!!
文字列の変換
#include <string>
int main() {
std::string str = "Hello, world!";
// 文字列の変換
std::string upper = str.to_upper(); // "HELLO, WORLD!"
std::string lower = str.to_lower(); // "hello, world!"
int i = std::stoi(str); // 0
double d = std::stod(str); // 0.0
float f = std::stof(str); // 0.0f
std::cout << upper << std::endl;
std::cout << lower << std::endl;
std::cout << i << std::endl;
std::cout << d << std::endl;
std::cout << f << std::endl;
return 0;
}
出力例
HELLO, WORLD!
hello, world!
0
0
0
C++ std::basic_string::ends_with 関数徹底解説
std::basic_string::ends_with 関数は、指定された文字列がストリングの末尾に一致するかどうかを検証します。一致する場合は true、一致しない場合は false を返します。構文パラメータsv: 一致させる文字列を表す std::basic_string_view オブジェクト
C++でハッシュ値を生成: std::u16string_viewとstd::hash
この解説では、以下の内容について説明します。std::hash テンプレートクラスstd::u16string_view 型std::hash<std::u16string_view> の使用方法応用例std::hash テンプレートクラスは、コンテナ内の要素をハッシュ化するために使用されます。ハッシュ化とは、データを数値に変換する処理です。ハッシュ値は、オブジェクトを一意に識別するために使用できる数値です。

std::basic_string_view::find_last_of の使い方:C++で文字列の最後の出現位置を探す
引数: str: 検索対象となる文字列 pos: 検索を開始する位置(省略可能、デフォルトは文字列末尾)str: 検索対象となる文字列pos: 検索を開始する位置(省略可能、デフォルトは文字列末尾)返値: 見つかった場合は、最後の出現位置 見つからない場合は、std::basic_string_view::npos

C++ の Strings における std::basic_string::resize の詳細解説
この解説では、以下の内容を詳細に説明します:std::basic_string::resize の概要: 機能 引数 戻り値 例機能引数戻り値例メモリ管理: 文字列の拡張と縮小 デフォルト初期化 明示的な初期化文字列の拡張と縮小デフォルト初期化
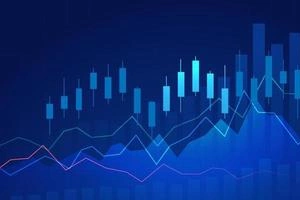
std::wstring_convertクラス:std::wcsrtombs関数のより安全な代替手段
std::wcsrtombs は、ワイド文字列をマルチバイト文字列に変換する関数です。これは、異なる文字エンコーディングを使用するシステム間で文字列データを交換する必要がある場合に役立ちます。機能std::wcsrtombs は以下の機能を提供します。
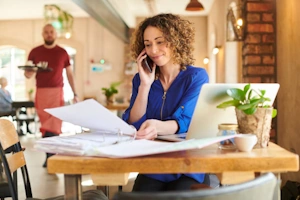

std::basic_string::erase のサンプルコード
std::basic_string::erase は、C++ 標準ライブラリ std::string クラスのメンバ関数であり、文字列の一部を削除するために使用されます。この関数は、文字列の長さを短縮し、その内容を変更します。使い方std::basic_string::erase は、以下の3つの方法で呼び出すことができます。
C++の「std::wcstoimax」でワイド文字列を整数に変換:詳細解説とサンプルコード
概要std::wcstoimax は、C++ の標準ライブラリに含まれる関数で、ワイド文字列 (wstring) を指定した基数に基づいて整数値に変換します。これは、std::stoi() 関数のワイド文字列バージョンと考えることができます。
C++ の Strings における std::basic_string::crend の詳細解説
std::basic_string::crend は、C++ 標準ライブラリ std::string クラスのメンバー関数であり、逆順イテレータ を返します。このイテレータは、文字列の最後の文字の次を指します。つまり、文字列の逆順の終わり を表します。
C++で文字コード変換をマスターしよう!std::btowcの使い方とサンプルコード
この関数を使うことで、異なるエンコード間で文字列を効率的に変換したり、マルチバイト文字を扱うプログラムを作成することができます。std::btowcは以下の形式で定義されています。c: 変換する単一バイト文字std::wint_t: 変換結果のワイド文字
std::basic_string_view のサンプルコード
std::basic_string_view は、文字列データへの読み取り専用ビューを提供する軽量なクラスです。std::string オブジェクトや char 配列など、さまざまな文字列データソースを参照することができます。主な特徴:読み取り専用: 文字列内容を変更することはできません。