C++ std::basic_string::find完全ガイド:部分文字列検索をマスターしよう!
C++ の std::basic_string::find 関数:文字列検索をマスターしよう!
std::basic_string::find
関数は、C++ の std::string
クラスにおいて、部分文字列の検索を行うための強力なツールです。この関数は、検索対象となる文字列と、検索開始位置を指定することで、部分文字列が見つかった最初の位置を返します。
使い方
std::basic_string::find
関数の基本的な使い方は以下の通りです。
std::string str = "Hello, world!";
// "world" を検索
size_t pos = str.find("world");
if (pos != std::string::npos) {
std::cout << "world は " << pos << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "world は見つかりませんでした。" << std::endl;
}
詳細
str.find("world")
の部分は、検索対象となる部分文字列を指定します。pos
変数は、部分文字列が見つかった最初の位置を格納します。std::string::npos
は、部分文字列が見つからなかったことを示す特別な値です。
応用例
- 特定の文字列が含まれているかどうかを確認する
- 部分文字列の位置を取得する
- 部分文字列を置換する
- 部分文字列を抽出する
その他のオプション
std::basic_string::find_first_of
関数:指定された文字のいずれか最初の出現位置を検索
補足
std::basic_string::find
関数は、大文字と小文字を区別します。- 検索対象となる部分文字列は、空文字列でも構いません。
- 検索開始位置は、文字列の長さよりも大きい値を指定することもできます。
練習問題
- 文字列 "stressed" の中で、最初の "s" と最後の "s" の位置をそれぞれ出力するプログラムを書いてみましょう。
- 文字列 "1234567890" の中で、数字 "5" の出現位置をすべて出力するプログラムを書いてみましょう。
std::basic_string::find
関数は、C++ の文字列処理において非常に重要な関数です。この関数を理解し使いこなすことで、様々な文字列処理を行うことができます。
std::basic_string::find 関数のサンプルコード
std::string str = "Hello, world!";
if (str.find("world") != std::string::npos) {
std::cout << "world は含まれています。" << std::endl;
} else {
std::cout << "world は含まれていません。" << std::endl;
}
部分文字列の位置を取得する
std::string str = "Hello, world!";
size_t pos = str.find("world");
if (pos != std::string::npos) {
std::cout << "world は " << pos << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "world は見つかりませんでした。" << std::endl;
}
部分文字列を置換する
std::string str = "Hello, world!";
str.replace(str.find("world"), 5, "universe");
std::cout << str << std::endl; // "Hello, universe!"
部分文字列を抽出する
std::string str = "Hello, world!";
std::string substring = str.substr(str.find("world"));
std::cout << substring << std::endl; // "world!"
大文字と小文字を区別しない検索
std::string str = "Hello, World!";
size_t pos = str.find("world", 0, std::locale::classic());
if (pos != std::string::npos) {
std::cout << "world は " << pos << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "world は見つかりませんでした。" << std::endl;
}
検索開始位置を指定する
std::string str = "Hello, world!";
size_t pos = str.find("world", 7);
if (pos != std::string::npos) {
std::cout << "world は " << pos << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "world は見つかりませんでした。" << std::endl;
}
空文字列の検索
std::string str = "Hello, world!";
size_t pos = str.find("");
if (pos != std::string::npos) {
std::cout << "空文字列は " << pos << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "空文字列は見つかりませんでした。" << std::endl;
}
検索対象文字列が空文字列の場合
std::string str = "Hello, world!";
std::string empty_str = "";
size_t pos = str.find(empty_str);
if (pos != std::string::npos) {
std::cout << "空文字列は " << pos << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "空文字列は見つかりませんでした。" << std::endl;
}
検索開始位置が文字列の長さよりも大きい場合
std::string str = "Hello, world!";
size_t pos = str.find("world", 13);
if (pos != std::string::npos) {
std::cout << "world は " << pos << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "world は見つかりませんでした。" << std::endl;
}
- C++ Standard - std::basic_string::find: [https://en.cppreference.
std::basic_string::find 関数の代替方法
std::search
関数:部分文字列の最初の出現位置を検索する汎用関数std::find_if
関数:条件を満たす最初の要素を見つける汎用関数
自作関数
- ループを使って部分文字列を検索する
- Boyer-Moore アルゴリズムなどの高速な検索アルゴリズムを実装する
正規表現
std::regex
クラスを使って、部分文字列を検索する
各方法の比較
方法 | 利点 | 欠点 |
---|---|---|
std::basic_string::find | シンプルで使いやすい | 高速な検索アルゴリズムではない |
std::search | 汎用性が高い | std::basic_string::find よりも複雑 |
std::find_if | 条件を指定して検索できる | std::basic_string::find よりも複雑 |
自作関数 | 高速な検索アルゴリズムを実装できる | 複雑で実装に時間がかかる |
正規表現 | 複雑なパターン検索に使える | 習得に時間がかかる |
std::search 関数
std::string str = "Hello, world!";
std::string substring = "world";
auto it = std::search(str.begin(), str.end(), substring.begin(), substring.end());
if (it != str.end()) {
std::cout << "world は " << (it - str.begin()) << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "world は見つかりませんでした。" << std::endl;
}
std::find_if 関数
std::string str = "Hello, world!";
size_t pos = std::find_if(str.begin(), str.end(), [](char c) { return c == 'w'; });
if (pos != str.end()) {
std::cout << "w は " << pos << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "w は見つかりませんでした。" << std::endl;
}
自作関数
size_t find_substring(const std::string& str, const std::string& substring) {
for (size_t i = 0; i < str.length() - substring.length() + 1; ++i) {
bool found = true;
for (size_t j = 0; j < substring.length(); ++j) {
if (str[i + j] != substring[j]) {
found = false;
break;
}
}
if (found) {
return i;
}
}
return std::string::npos;
}
int main() {
std::string str = "Hello, world!";
std::string substring = "world";
size_t pos = find_substring(str, substring);
if (pos != std::string::npos) {
std::cout << "world は " << pos << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "world は見つかりませんでした。" << std::endl;
}
return 0;
}
正規表現
std::string str = "Hello, world!";
std::regex re("world");
std::smatch m;
if (std::regex_search(str, m, re)) {
std::cout << "world は " << m.position() << " 番目に見つかりました。" << std::endl;
} else {
std::cout << "world は見つかりませんでした。" << std::endl;
}
- Boyer-Moore アルゴリズム: [https://ja.wikipedia
C++の「std::wcstoimax」でワイド文字列を整数に変換:詳細解説とサンプルコード
概要std::wcstoimax は、C++ の標準ライブラリに含まれる関数で、ワイド文字列 (wstring) を指定した基数に基づいて整数値に変換します。これは、std::stoi() 関数のワイド文字列バージョンと考えることができます。
C++ の Strings における std::wcslen 関数の詳細解説
std::wcslen 関数の使い方std::wcslen 関数の使い方は非常に簡単です。以下のコード例のように、取得したいワイド文字列の先頭アドレスを関数に渡すだけです。std::wcslen 関数の詳細引数: str: ワイド文字列の先頭アドレス
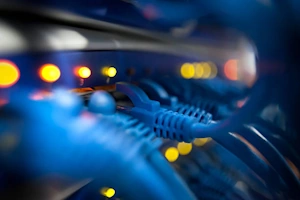
C++ Stringsにおけるstd::basic_string_view::rfind
概要std::basic_string_view::rfind は、部分文字列と検索を開始する位置を受け取り、部分文字列が最後に出現する位置を返します。部分文字列が見つからない場合は、std::basic_string_view::npos が返されます。
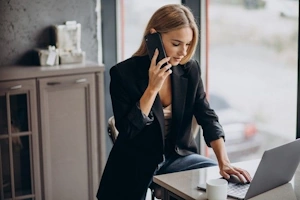
C++ストリングクラスにおけるstd::basic_string::find_first_not_of関数:詳細解説とサンプルコード
std::basic_string::find_first_not_of関数は、指定された文字列または文字範囲内で、最初の除外文字が現れる位置を検索します。除外文字とは、検索対象文字列に含まれていない文字のことです。詳細解説関数宣言引数s: 除外文字列または文字範囲の先頭ポインタ
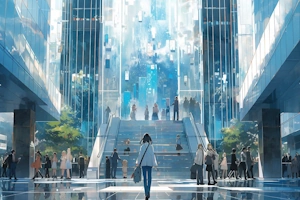
std::basic_string_view::find_last_of の使い方:C++で文字列の最後の出現位置を探す
引数: str: 検索対象となる文字列 pos: 検索を開始する位置(省略可能、デフォルトは文字列末尾)str: 検索対象となる文字列pos: 検索を開始する位置(省略可能、デフォルトは文字列末尾)返値: 見つかった場合は、最後の出現位置 見つからない場合は、std::basic_string_view::npos

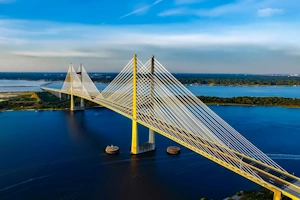
std::basic_string_view のサンプルコード
std::basic_string_view は、文字列データへの読み取り専用ビューを提供する軽量なクラスです。std::string オブジェクトや char 配列など、さまざまな文字列データソースを参照することができます。主な特徴:読み取り専用: 文字列内容を変更することはできません。
C++ の Strings における std::basic_string::crend の詳細解説
std::basic_string::crend は、C++ 標準ライブラリ std::string クラスのメンバー関数であり、逆順イテレータ を返します。このイテレータは、文字列の最後の文字の次を指します。つまり、文字列の逆順の終わり を表します。
C++ プログラマー必見!std::basic_string::empty 関数の詳細解説
概要機能: 文字列が空かどうかを判定戻り値: 空の場合: true 空でない場合: false空の場合: true空でない場合: false引数: なし使用例:動作の詳細empty() 関数は、文字列の length() が 0 かどうかをチェックします。
std::basic_stringを使いこなして、C++で文字列を自在に操る
std::basic_stringは、C++標準ライブラリで提供される汎用的な文字列クラスです。文字列の格納、操作、比較など、文字列処理に必要な機能を網羅しています。主な特徴:様々な文字型に対応:char、wchar_t、char16_t、char32_tなど
C++ の Strings における std::basic_string_view 推論ガイド
推論ガイドは、テンプレートクラスの型引数を自動的に推論するための機能です。std::basic_string_view クラスには、以下の推論ガイドが用意されています。文字列リテラルから推論このコードでは、文字列リテラル "Hello, world!" から std::basic_string_view<char> 型のオブジェクトが自動的に生成されます。