C++でハッシュ値を生成: std::u16string_viewとstd::hash
C++ の Strings に関連する std::hash<std::u16string_view> プログラミング解説
この解説では、以下の内容について説明します。
- std::hash テンプレートクラス
- std::u16string_view 型
- std::hash<std::u16string_view> の使用方法
- 応用例
std::hash テンプレートクラス
std::hash テンプレートクラスは、コンテナ内の要素をハッシュ化するために使用されます。ハッシュ化とは、データを数値に変換する処理です。ハッシュ値は、オブジェクトを一意に識別するために使用できる数値です。
std::hash テンプレートクラスは、以下の要素をテンプレート引数として受け取ります。
- ハッシュ化したいオブジェクトの型
std::hash テンプレートクラスは以下のメンバ関数を提供します。
- operator()(const T& obj): オブジェクトをハッシュ値に変換します。
std::u16string_view 型
std::u16string_view 型は、UTF-16 エンコードされた文字列を表す読み取り専用ビューです。std::string_view 型と似ていますが、UTF-16 エンコードされた文字列を扱うために使用されます。
std::u16string_view 型は以下のメンバ関数を提供します。
- data(): 文字列のデータへのポインタを返します。
- size(): 文字列の長さを返します。
std::hash<std::u16string_view> の使用方法
std::hash<std::u16string_view> を使用するには、以下のコードのように記述します。
#include <iostream>
#include <string>
int main() {
std::u16string_view str = u"Hello, world!";
std::size_t hash = std::hash<std::u16string_view>()(str);
std::cout << "ハッシュ値: " << hash << std::endl;
return 0;
}
このコードは、"Hello, world!" という文字列をハッシュ化し、ハッシュ値を出力します。
応用例
std::hash<std::u16string_view> は、以下の用途に使用できます。
- マップやセットなどのコンテナで、キーとして std::u16string_view 型のオブジェクトを使用する場合
- オブジェクトの一意性を検証する場合
- データベースのインデックスを作成する場合
std::hash<std::u16string_view> のサンプルコード
#include <iostream>
#include <string>
int main() {
std::u16string_view str = u"Hello, world!";
std::size_t hash = std::hash<std::u16string_view>()(str);
std::cout << "ハッシュ値: " << hash << std::endl;
return 0;
}
マップでキーとして std::u16string_view 型のオブジェクトを使用する
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::u16string_view, int> mymap;
myapp[u"Hello"] = 1;
myapp[u"World"] = 2;
// キー "Hello" の値を取得
int value = mymap[u"Hello"];
std::cout << "値: " << value << std::endl;
return 0;
}
オブジェクトの一意性を検証する
#include <iostream>
#include <string>
#include <set>
class Person {
public:
Person(const std::u16string_view& name) : name_(name) {}
bool operator==(const Person& other) const {
return name_ == other.name_;
}
private:
std::u16string_view name_;
};
int main() {
std::set<Person> myset;
myset.insert(Person(u"John"));
myset.insert(Person(u"Mary"));
// "John" が存在するかどうかを確認
bool exists = myset.find(Person(u"John")) != myset.end();
std::cout << "存在する: " << exists << std::endl;
return 0;
}
データベースのインデックスを作成する
#include <iostream>
#include <string>
#include <vector>
class Database {
public:
void Add(const std::u16string_view& name, int age) {
// データベースに名前と年齢を追加
}
std::vector<int> FindByName(const std::u16string_view& name) {
// 名前で検索し、年齢のリストを返す
}
private:
// ハッシュテーブルを使用して、名前をインデックス化する
std::unordered_map<std::u16string_view, std::vector<int>> index_;
};
int main() {
Database db;
db.Add(u"John", 30);
db.Add(u"Mary", 25);
// "John" の年齢を取得
std::vector<int> ages = db.FindByName(u"John");
for (int age : ages) {
std::cout << age << std::endl;
}
return 0;
}
これらのコードはあくまでもサンプルです。実際の使用例は、状況によって異なります。
std::u16string_view をハッシュ化する他の方法
#include <iostream>
#include <string>
int main() {
std::u16string_view str = u"Hello, world!";
std::wstring wstr(str.begin(), str.end());
std::size_t hash = std::hash<std::wstring>()(wstr);
std::cout << "ハッシュ値: " << hash << std::endl;
return 0;
}
自作のハッシュ関数を使用する
#include <iostream>
#include <string>
size_t Hash(const std::u16string_view& str) {
// ハッシュアルゴリズムを実装
return 0;
}
int main() {
std::u16string_view str = u"Hello, world!";
std::size_t hash = Hash(str);
std::cout << "ハッシュ値: " << hash << std::endl;
return 0;
}
これらの方法は、それぞれ異なる長所と短所があります。
-
std::wstring と std::hash を使用する
- 最も簡単
- パフォーマンスは最適ではない
-
自作のハッシュ関数を使用する
- 最も高速
- 実装が複雑
-
3rd party ライブラリを使用する
- バランスが良い
- ライブラリの依存関係が必要
std::hash<std::u16string_view> は、C++ 標準ライブラリで提供される std::hash テンプレートクラスの特殊化であり、std::u16string_view 型のオブジェクトをハッシュ値に変換するために使用されます。ハッシュ値は、オブジェクトを一意に識別するために使用できる数値です。
上記以外にも、さまざまな方法で std::u16string_view をハッシュ化することができます。最適な方法は、状況によって異なります。
C++の「std::wcstoimax」でワイド文字列を整数に変換:詳細解説とサンプルコード
概要std::wcstoimax は、C++ の標準ライブラリに含まれる関数で、ワイド文字列 (wstring) を指定した基数に基づいて整数値に変換します。これは、std::stoi() 関数のワイド文字列バージョンと考えることができます。
std::wcstol 関数を使いこなして、C++ プログラミングをレベルアップ!
std::wcstol は、以下の引数を受け取ります。str: 変換対象となるワイド文字列へのポインターstr_end: 変換が終了した後の文字列へのポインター (省略可能)base: 数値の基数 (省略時は 10)この関数は、str で指定されたワイド文字列を解析し、指定された基数に基づいて長整型値に変換します。変換が成功すると、変換結果が返されます。変換が失敗した場合、0 が返されます。
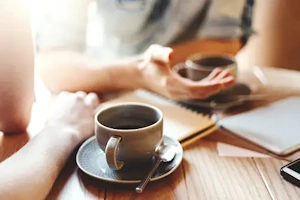
C++ プログラマー必見! ワイド文字列と浮動小数点数の変換テクニック: std::wcstold 関数
std::wcstold 関数は、ワイド文字列 (wstring) から double 型の浮動小数点数を解釈し、変換するものです。ワイド文字列とは、wchar_t 型の文字列で、通常の文字列 (char) よりも広範囲の文字を表すことができます。
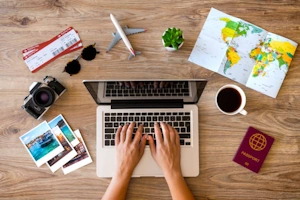
C++ Strings の魔法使い:std::stoi で文字列を整数に変換する
この解説では、std::stoi の使い方を分かりやすく説明し、さらにその仕組みや注意点についても詳しく掘り下げていきます。std::stoi は、string 型の文字列を受け取り、それを int 型の整数に変換する関数です。使い方はとても簡単で、以下のコードのように記述します。
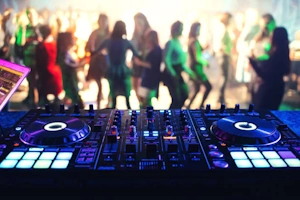
C++ の Strings における std::wcslen 関数の詳細解説
std::wcslen 関数の使い方std::wcslen 関数の使い方は非常に簡単です。以下のコード例のように、取得したいワイド文字列の先頭アドレスを関数に渡すだけです。std::wcslen 関数の詳細引数: str: ワイド文字列の先頭アドレス
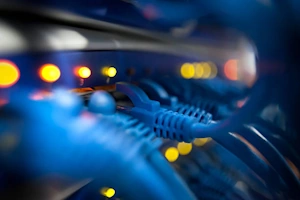

C++ の Strings における std::basic_string::crend の詳細解説
std::basic_string::crend は、C++ 標準ライブラリ std::string クラスのメンバー関数であり、逆順イテレータ を返します。このイテレータは、文字列の最後の文字の次を指します。つまり、文字列の逆順の終わり を表します。
C++のstd::basic_string::assign関数:初心者向けチュートリアル
この関数は、initializer list を受け取り、その内容を std::string に割り当てます。initializer list は、カンマで区切られた値のリストです。assign 関数は、initializer list 以外にも様々なパラメータを受け取ることができます。
C++ Stringsにおけるstd::basic_string_view::rfind
概要std::basic_string_view::rfind は、部分文字列と検索を開始する位置を受け取り、部分文字列が最後に出現する位置を返します。部分文字列が見つからない場合は、std::basic_string_view::npos が返されます。
std::basic_string::dataを使いこなして、C++プログラミングをもっと楽しく!
概要std::basic_string::data は、std::basic_string オブジェクト内の文字列データへのポインタを返します。返されたポインタは、const であり、文字列データの変更はできません。返されたポインタは、std::basic_string オブジェクトの生存期間中は有効です。
C++ Strings の魔法使い:std::stoi で文字列を整数に変換する
この解説では、std::stoi の使い方を分かりやすく説明し、さらにその仕組みや注意点についても詳しく掘り下げていきます。std::stoi は、string 型の文字列を受け取り、それを int 型の整数に変換する関数です。使い方はとても簡単で、以下のコードのように記述します。