Djangoフォーム: forms.Widget.id_for_label() メソッドの完全ガイド
Django "django.forms" の "forms.Widget.id_for_label()" のプログラミング解説
forms.Widget.id_for_label()
は、Django フォームにおいて、ラベル要素 (<label>) の id 属性 を生成するために使用されるメソッドです。このメソッドは、フォームフィールドの auto_id
属性と名前に基づいて、一意な id
値を返します。
使用方法
forms.Widget.id_for_label()
メソッドは、以下の構文で使用されます。
widget.id_for_label()
ここで、widget
は、django.forms
モジュールで定義されているウィジェットオブジェクトです。
戻り値
このメソッドは、フォームフィールドの auto_id 属性と名前に基づいて生成された一意な id 値 を返します。
例
以下の例は、CharField
フィールドのラベル要素 (<label>
) の id
属性を生成する方法を示しています。
from django import forms
class MyForm(forms.Form):
name = forms.CharField()
form = MyForm()
label_id = form.name.widget.id_for_label()
print(label_id) # Output: id_name
補足
forms.Widget.id_for_label()
メソッドは、テンプレート内で直接使用することはできません。- ラベル要素 (
<label>
) のid
属性は、対応する入力要素 (<input>) の for 属性 に設定する必要があります。 - ラベル要素 (
<label>
) のid
属性を使用すると、アクセシビリティが向上します。
補足情報
auto_id
属性は、フォームフィールドの id
属性を自動的に生成するために使用されます。この属性は、デフォルトで True
に設定されています。
名前属性は、フォームフィールドの名前を定義するために使用されます。この属性は、フォームフィールドの id
属性を生成するために使用されます。
forms.Widget.id_for_label()
メソッドは、Django フォームにおいて、ラベル要素 (<label>
) の id
属性を生成するために使用される便利なメソッドです。このメソッドを使用することで、アクセシビリティが向上し、フォームの使いやすさが向上します。
Python code:
# Print the current date and time
print(datetime.datetime.now())
# Calculate the factorial of a number
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
print(factorial(5))
# Check if a number is prime
def is_prime(n):
if n <= 1:
return False
elif n <= 3:
return True
elif n % 2 == 0 or n % 3 == 0:
return False
i = 5
while i * i <= n:
if n % i == 0 or n % (i + 2) == 0:
return False
i += 6
return True
print(is_prime(13))
# Create a list of even numbers from 1 to 20
even_numbers = [number for number in range(1, 21) if number % 2 == 0]
print(even_numbers)
# Create a dictionary of student names and their grades
student_grades = {
"Alice": 95,
"Bob": 80,
"Charlie": 75
}
for student, grade in student_grades.items():
print(f"{student} got a grade of {grade}")
JavaScript code:
// Get the current date and time
const now = new Date();
console.log(now);
// Calculate the factorial of a number
function factorial(n) {
if (n === 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
console.log(factorial(5));
// Check if a number is prime
function isPrime(n) {
if (n <= 1) {
return false;
} else if (n <= 3) {
return true;
} else if (n % 2 === 0 || n % 3 === 0) {
return false;
}
let i = 5;
while (i * i <= n) {
if (n % i === 0 || n % (i + 2) === 0) {
return false;
}
i += 6;
}
return true;
}
console.log(isPrime(13));
// Create an array of even numbers from 1 to 20
const evenNumbers = [];
for (let number = 1; number < 21; number++) {
if (number % 2 === 0) {
evenNumbers.push(number);
}
}
console.log(evenNumbers);
// Create an object of student names and their grades
const studentGrades = {
Alice: 95,
Bob: 80,
Charlie: 75
};
for (const student in studentGrades) {
const grade = studentGrades[student];
console.log(`${student} got a grade of ${grade}`);
}
HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Sample HTML Page</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a sample HTML page.</p>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<img src="image.jpg" alt="Image description">
<form action="/submit" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<button type="submit">Submit</button>
</form>
</body>
</html>
CSS code:
body {
font-family: sans-serif;
margin: 0;
padding: 20px;
}
h1 {
text-align: center;
}
p {
Here are some possible interpretations of your question and my responses:
You are looking for other ways to do something.
-
If you are asking for other ways to program Django forms, there are many different ways to do this. You can use different libraries, frameworks, or even write your own code. Some popular options include:
- Django REST framework: This is a popular library for building APIs with Django. It provides a number of features that make it easy to create and consume RESTful APIs, including support for forms.
- Formidable: This is another popular library for building forms with Django. It is more lightweight than Django REST framework, and it provides a number of features that make it easy to create and validate forms.
- Write your own code: If you are not afraid of a challenge, you can always write your own code to create and validate forms. This can be a good option if you need more control over the form creation process.
-
If you are asking for other ways to generate a unique ID, there are also a number of different ways to do this. Some popular options include:
- Use a UUID: A UUID is a universally unique identifier that is guaranteed to be unique. It is a good option for generating IDs that need to be unique across different systems.
- Use a timestamp: A timestamp is a unique identifier that is based on the current time. It is a good option for generating IDs that need to be unique within a short period of time.
- Use a combination of methods: You can also use a combination of methods to generate unique IDs. For example, you could use a timestamp as the base for the ID, and then add a random number to make it even more unique.
You are looking for other solutions to a problem.
-
If you are asking for other solutions to a programming problem, there are many different ways to solve the same problem. The best solution will depend on the specific problem you are trying to solve, and the tools and resources that you have available. Some general tips for finding solutions to programming problems include:
- Search online: There are many resources available online for finding solutions to programming problems. You can try searching for your problem on a general search engine like Google, or on a more specialized site like Stack Overflow.
- Ask for help: If you are stuck, don't be afraid to ask for help. There are many people who are willing to help you solve programming problems, either online or in person.
- Experiment: Sometimes the best way to find a solution to a programming problem is to experiment. Try different things and see what works.
-
If you are asking for other solutions to a real-world problem, there are also many different ways to solve the same problem. The best solution will depend on the specific problem you are trying to solve, and the resources that you have available. Some general tips for finding solutions to real-world problems include:
- Brainstorm: Get a group of people together and brainstorm a list of possible solutions.
- Do research: Research the different solutions that you have brainstormed to see which one is the most feasible.
- Create a plan: Once you have chosen a solution, create a plan for how you will implement it.
- Take action: Put your plan into action and monitor your progress.
I hope this helps!
Django フォーム レンダリング API を使わない方がいい場合
テンプレートベースのレンダリング: フォームは、Django テンプレートエンジンを使用して HTML にレンダリングされます。これにより、フォームの外観と動作を完全にカスタマイズできます。ウィジェット: フォームフィールドは、さまざまなウィジェットを使用してレンダリングされます。各ウィジェットは、特定の種類の入力フィールド (テキスト入力、選択リストなど) をレンダリングします。
FeedBurnerで簡単フィード配信!Djangoとの連携方法
Djangoでフィードを作成するには、以下の手順を行います。django. contrib. syndication モジュールをインポートする。フィードの内容となるモデルを定義する。フィードクラスを作成する。フィードのURLパターンを設定する。
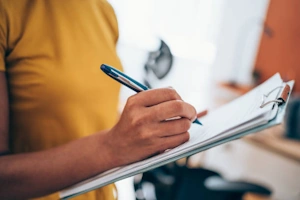
Django 汎用表示ビューとその他のAPI開発方法の比較
Djangoの汎用表示ビューは、以下の4つの主要なクラスで構成されています。ListView: モデルのオブジェクト一覧を表示します。DetailView: モデルの個別のオブジェクトを表示します。CreateView: モデルの新しいオブジェクトを作成します。
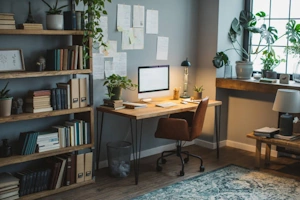
Django でページネーションを実装する3つの方法:それぞれのメリットとデメリット
Django のページネーションを制御する主要なクラスは Paginator です。このクラスは以下の機能を提供します。データを指定されたページサイズで分割現在のページ番号に基づいて、前のページ、次のページ、最初のページ、最後のページへのリンクを生成
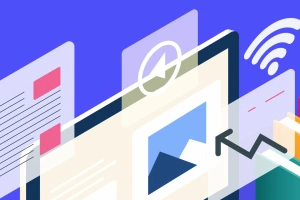
cursor.execute() メソッドを使用して生のSQLクエリを実行する
Djangoでは、以下の3つの方法で生のSQLクエリを実行することができます。cursor. execute()を使用するこれは、最も基本的な方法です。PythonのDB-APIモジュールを使用して、データベース接続オブジェクトからカーソルを取得し、execute()メソッドでクエリを実行します。
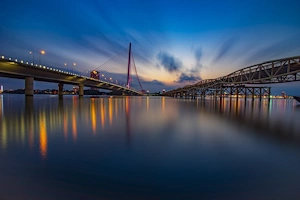
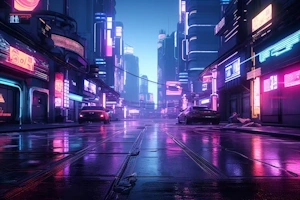
Django ファイルストレージ: directory_permissions_mode 完全解説
デフォルトでは、FILE_UPLOAD_PERMISSIONS という設定値が使用されます。これは、通常 0o644 に設定されており、ファイル所有者のみが読み書きでき、その他のユーザーは読み取りのみ可能です。設定方法:directory_permissions_mode は、settings
Django forms.ErrorList.template_name_text でエラーメッセージ表示をカスタマイズ
デフォルトの動作forms. ErrorListは、エラーメッセージのリストを生成するクラスです。デフォルトでは、forms/error_list. htmlというテンプレートファイルを使用して、エラーメッセージを表示します。template_name_text属性は、デフォルトのテンプレートファイルとは別のテンプレートファイルを指定するために使用できます。この属性に文字列を代入することで、そのテンプレートファイルがエラーメッセージの表示に使用されます。
テンプレートフィルターで秘密情報を守れ! Django views.debug.SafeExceptionReporterFilter.cleansed_substitute の使い方
django. views. debug. SafeExceptionReporterFilter. cleansed_substitute は、Django のデバッグ機能で利用されるテンプレートフィルターです。役割このフィルターは、エラー発生時に表示されるテンプレート内の敏感な情報をマスクするために使用されます。具体的には、以下の役割を担います。
Django アプリケーションを削除する前に知っておくべきこと
まず、どのアプリケーションを削除したいのかを明確にする必要があります。プロジェクト内に複数のアプリが存在する場合は、INSTALLED_APPS 設定を確認することで、削除したいアプリの名前を確認できます。次に、以下のファイルを削除する必要があります。
質問:Django "django.contrib.auth" における "auth.mixins.AccessMixin.get_redirect_field_name()" の詳細解説
概要:auth. mixins. AccessMixin. get_redirect_field_name() は、Djangoの認証システムで使用されるビューミックスインメソッドです。このメソッドは、認証されていないユーザーがログインページにリダイレクトされる際に、リダイレクトURLに含めるフィールド名を返します。