NumPy ufunc ループを C 言語で定義する: int PyUFunc_RegisterLoopForType() 関数の詳細解説
NumPy C-API: int PyUFunc_RegisterLoopForType() 関数の詳細解説
int PyUFunc_RegisterLoopForType()
は、NumPy C-API の重要な関数であり、特定の型に対して NumPy ufunc ループを登録するために使用されます。これは、NumPy 配列の要素間で実行される C 言語関数を登録するための強力なツールです。
関数宣言
int PyUFunc_RegisterLoopForType(PyUFuncObject *ufunc,
PyTypeObject *type,
PyUFuncLoopFunc *func,
int flags)
引数
- ufunc: ループを登録する ufunc オブジェクト
- type: ループが適用される NumPy 型
- func: 実行される C 言語関数
- flags: オプションフラグ
C 言語関数
func
は、以下の形式で記述する必要があります。
void func(char **args, npy_intp *dimensions, npy_intp *steps, void *data)
- args: 入力および出力配列へのポインタを含む配列
- dimensions: 各入力および出力配列の次元数
- steps: 各入力および出力配列における各次元の要素間の距離
- data: ユーザーデータ
詳細解説
PyUFunc_RegisterLoopForType()
は、以下の処理を行います。
- ufunc オブジェクトと型オブジェクトの検証
- ループ関数の検証
- ループ関数の登録
登録後の動作
ループ関数は、NumPy ufunc がその型に対して実行されるたびに呼び出されます。
例
以下の例は、PyUFunc_RegisterLoopForType()
を使用して、2 つの NumPy 配列の要素を乗算するループ関数を登録する方法を示しています。
#include <numpy/ufunc_object.h>
static void multiply_loop(char **args, npy_intp *dimensions, npy_intp *steps, void *data) {
npy_intp i;
npy_float64 *x = (npy_float64 *)args[0];
npy_float64 *y = (npy_float64 *)args[1];
npy_float64 *out = (npy_float64 *)args[2];
for (i = 0; i < dimensions[0]; i++) {
out[i] = x[i] * y[i];
}
}
static PyUFuncGenericFunction multiply_functions[] = {
{&multiply_loop, NULL, NULL}
};
static PyUFuncObject multiply_ufunc = {
.fn = multiply_functions,
.types = {PyArray_FLOAT64, PyArray_FLOAT64, PyArray_FLOAT64},
.ntypes = 3,
.name = "multiply",
.doc = "Multiply two NumPy arrays element-wise."
};
int main() {
PyUFunc_RegisterLoopForType(&multiply_ufunc, &PyArray_FLOAT64);
// ...
}
補足
PyUFunc_RegisterLoopForType()
は、高度な NumPy C-API 関数です。使用前に、NumPy C-API に関する十分な知識が必要です。- より簡単な方法で NumPy ufunc を定義したい場合は、
@vectorize
デコレータを使用することを検討してください。
NumPy C-API: int PyUFunc_RegisterLoopForType() 関数のサンプルコード
#include <numpy/ufunc_object.h>
static void add_loop(char **args, npy_intp *dimensions, npy_intp *steps, void *data) {
npy_intp i;
npy_int32 *x = (npy_int32 *)args[0];
npy_int32 *y = (npy_int32 *)args[1];
npy_int32 *out = (npy_int32 *)args[2];
for (i = 0; i < dimensions[0]; i++) {
out[i] = x[i] + y[i];
}
}
static PyUFuncGenericFunction add_functions[] = {
{&add_loop, NULL, NULL}
};
static PyUFuncObject add_ufunc = {
.fn = add_functions,
.types = {PyArray_INT32, PyArray_INT32, PyArray_INT32},
.ntypes = 3,
.name = "add",
.doc = "Add two NumPy arrays element-wise."
};
int main() {
PyUFunc_RegisterLoopForType(&add_ufunc, &PyArray_INT32);
// ...
}
乗算
#include <numpy/ufunc_object.h>
static void multiply_loop(char **args, npy_intp *dimensions, npy_intp *steps, void *data) {
npy_intp i;
npy_float64 *x = (npy_float64 *)args[0];
npy_float64 *y = (npy_float64 *)args[1];
npy_float64 *out = (npy_float64 *)args[2];
for (i = 0; i < dimensions[0]; i++) {
out[i] = x[i] * y[i];
}
}
static PyUFuncGenericFunction multiply_functions[] = {
{&multiply_loop, NULL, NULL}
};
static PyUFuncObject multiply_ufunc = {
.fn = multiply_functions,
.types = {PyArray_FLOAT64, PyArray_FLOAT64, PyArray_FLOAT64},
.ntypes = 3,
.name = "multiply",
.doc = "Multiply two NumPy arrays element-wise."
};
int main() {
PyUFunc_RegisterLoopForType(&multiply_ufunc, &PyArray_FLOAT64);
// ...
}
論理否定
#include <numpy/ufunc_object.h>
static void logical_not_loop(char **args, npy_intp *dimensions, npy_intp *steps, void *data) {
npy_intp i;
npy_bool *x = (npy_bool *)args[0];
npy_bool *out = (npy_bool *)args[1];
for (i = 0; i < dimensions[0]; i++) {
out[i] = !x[i];
}
}
static PyUFuncGenericFunction logical_not_functions[] = {
{&logical_not_loop, NULL, NULL}
};
static PyUFuncObject logical_not_ufunc = {
.fn = logical_not_functions,
.types = {PyArray_BOOL, PyArray_BOOL},
.ntypes = 2,
.name = "logical_not",
.doc = "Logical NOT of a NumPy array element-wise."
};
int main() {
PyUFunc_RegisterLoopForType(&logical_not_ufunc, &PyArray_BOOL);
// ...
}
最大値
#include <numpy/ufunc_object.h>
static void maximum_loop(char **args, npy_intp *dimensions, npy_intp *steps, void *data) {
npy_intp i;
npy_int32 *x = (npy_int32 *)args[0];
npy_int32 *y = (npy_int32 *)args
NumPy C-API: int PyUFunc_RegisterLoopForType() 以外の方法
@vectorize デコレータ
NumPy には、@vectorize
デコレータと呼ばれる、C 言語関数を NumPy ufunc に変換する便利な機能があります。@vectorize
は、PyUFunc_RegisterLoopForType()
よりも簡単に NumPy ufunc を定義できます。
@vectorize
def add(x, y):
return x + y
# 加算 ufunc を利用
x = np.array([1, 2, 3])
y = np.array([4, 5, 6])
z = add(x, y)
print(z)
# [5 7 9]
numpy.frompyfunc
関数は、C 言語関数を NumPy ufunc オブジェクトに変換する関数です。numpy.frompyfunc
は、@vectorize
よりも柔軟性がありますが、複雑で習得に時間がかかります。
def add_c(x, y):
return x + y
add_ufunc = np.frompyfunc(add_c, 2, 1)
# 加算 ufunc を利用
x = np.array([1, 2, 3])
y = np.array([4, 5, 6])
z = add_ufunc(x, y)
print(z)
# [5 7 9]
NumPy ufunc の拡張
NumPy は、ufunc.add
や ufunc.multiply
などの事前定義された ufunc を多数提供しています。これらの ufunc を拡張して、独自のニーズに合わせてカスタマイズできます。
def my_add(x, y):
return x + y + 1
# 加算 ufunc を拡張
np.add.at = my_add
# 拡張された加算 ufunc を利用
x = np.array([1, 2, 3])
y = np.array([4, 5, 6])
z = np.add(x, y)
print(z)
# [6 8 10]
その他のライブラリ
NumPy 以外にも、ufunc を定義するためのライブラリがいくつかあります。以下はその例です。
int PyUFunc_RegisterLoopForType()
関数は、NumPy ufunc ループを登録する強力な方法ですが、複雑で習得に時間がかかります。上記のような代替方法も検討することで、ニーズに合った方法を選択できます。
NumPy.tri() 関数を使ったその他の方法
numpy. tri()関数は以下の4つのパラメータを受け取ります。N: 作成する配列の行数M: 作成する配列の列数 (省略可。デフォルトはNと同じ)k: 対角線の位置 (デフォルトは0。0の場合は主対角線、負の場合は主対角線より下、正の場合は主対角線より上)
NumPy Array Creation Routinesにおけるnumpy.diagflat() 解説
NumPyのnumpy. diagflat()関数は、1次元配列を対角線要素とする2次元配列を作成します。これは、対角行列の作成や、特定のオフセットを持つ対角線要素を持つ配列の作成など、さまざまな場面で役立ちます。引数v:1次元配列またはスカラ値。対角線要素として使用されます。
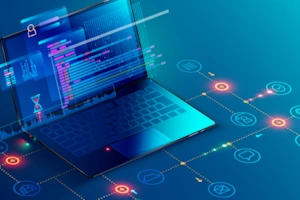
NumPy の empty() とは?
上記コードでは、3行2列の空の配列 array が作成されます。array の内容は初期化されていないため、ランダムな値が表示されます。numpy. empty() には、以下のオプション引数が用意されています。dtype: 配列のデータ型を指定します。デフォルトは float64 です。
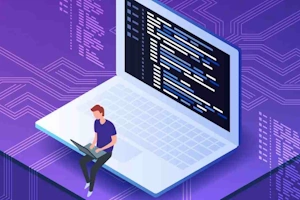
NumPy行列作成の極意: numpy.mat() vs その他の方法
このチュートリアルでは、NumPyの行列作成ルーチン、特にnumpy. mat()関数について詳しく解説します。NumPyには、様々な方法で配列を作成するルーチンが用意されています。代表的なものをいくつかご紹介します。numpy. array(): 最も基本的な配列作成ルーチンです。Pythonのリストやタプルなど、様々なデータ構造から配列を生成できます。
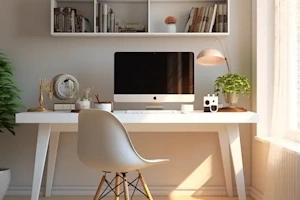
NumPy 配列分割:初心者から上級者まで役立つ完全ガイド
NumPy の numpy. split() 関数は、配列を指定された軸に沿って分割する便利な関数です。分割された各部分は、元の配列のビューとして保持されます。基本的な使い方引数array: 分割したいNumPy配列indices_or_sections: 分割するポイントを指定 整数の場合: 配列を等間隔に分割 配列の場合: 指定されたインデックスで分割
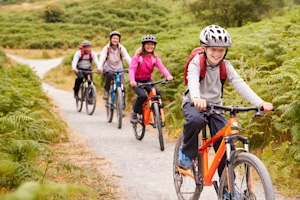
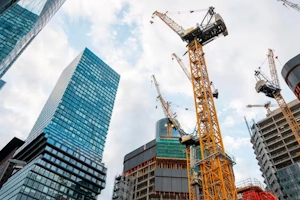
NumPyのrandom.Generator.triangular():三角形分布サンプリングの基礎
NumPyのrandom. Generator. triangular()は、三角形分布からランダムサンプルを生成する関数です。三角形分布は、最小値、最大値、モード(頂点)を持つ3つのパラメータで定義される連続確率分布です。使用方法パラメータ
NumPy C-API: PyArray_XDECREF() 関数の詳細解説
PyArray_XDECREF() は、NumPy オブジェクトの参照カウントを減らす関数です。 オブジェクトの参照カウントが 0 になると、メモリが解放されます。重要なポイント:PyArray_XDECREF() は、Py_DECREF() と似ていますが、NumPy オブジェクト専用です。
NumPy recarray.setflags() 関数:レコード配列のメモリレイアウトを自在に操る
上記の例では、rec_arrのwriteableフラグをFalseに設定することで、配列を書き込み不可にしています。その後、rec_arr[0].nameを変更しようとすると、エラーが発生します。recarray. setflags()は以下のフラグを設定できます。
NumPy.bartlett() の代替方法: 手動計算、SciPy、その他のライブラリ
窓関数は、有限長の離散信号を処理する際に、信号の端部における不連続性を滑らかにするために用いられる数学的な関数です。これは、信号処理における様々な場面で発生する 周波数漏れ や ギブス現象 といった問題を軽減するために役立ちます。NumPy には、Bartlett窓以外にも様々な窓関数を生成する関数が用意されています。代表的な窓関数には、以下のようなものがあります。
NumPy matrix.mean() 関数とは?
NumPy には、標準配列 (ndarray) 以外にもいくつかの配列サブクラスがあります。これらのサブクラスは、特定の種類のデータや操作を効率的に処理するために設計されています。主な標準配列サブクラスは以下の通りです。matrix: 行列演算用に最適化された配列