torch.nn.modules.module.register_module_forward_hook の徹底解説
PyTorchのニューラルネットワークにおける torch.nn.modules.module.register_module_forward_hook 解説
torch.nn.modules.module.register_module_forward_hook
は、PyTorchのニューラルネットワークにおいて、モジュールのフォワードパスにフックを登録するための関数です。フックは、モジュールの入出力データや中間層の出力などにアクセスできるコールバック関数です。
主な用途
- 中間層の出力の可視化
- デバッグ
- 勾配計算の変更
- カスタム層の実装
使い方
register_module_forward_hook
は、モジュールの forward
メソッドにフック関数を登録します。フック関数は、以下の引数を受け取ります。
module
: フックが登録されたモジュールinput
: モジュールの入力データoutput
: モジュールの出力データ
以下の例は、forward
パスでモジュールの入力と出力をログに出力するフック関数を登録する例です。
def hook_fn(module, input, output):
print(f"モジュール名: {module}")
print(f"入力: {input}")
print(f"出力: {output}")
model.register_module_forward_hook(hook_fn)
注意事項
- フック関数は、
forward
パスの実行速度を遅くする可能性があります。 - フック関数は、モジュールの状態を変更しないようにする必要があります。
関連キーワード
- PyTorch
- ニューラルネットワーク
- フック
- コールバック関数
- フォワードパス
- 中間層
- 勾配計算
- カスタム層
PyTorch register_module_forward_hook サンプルコード
中間層出力の可視化
import torch
class MyModel(torch.nn.Module):
def __init__(self):
super().__init__()
self.fc1 = torch.nn.Linear(10, 10)
self.fc2 = torch.nn.Linear(10, 10)
def forward(self, x):
x = x.view(-1)
x = self.fc1(x)
x = torch.relu(x)
x = self.fc2(x)
return x
def hook_fn(module, input, output):
print(f"モジュール名: {module}")
print(f"入力: {input}")
print(f"出力: {output}")
model = MyModel()
model.register_module_forward_hook(hook_fn)
# 入力データ
x = torch.randn(10, 10)
# モデルの推論
model(x)
デバッグ
import torch
class MyModel(torch.nn.Module):
def __init__(self):
super().__init__()
self.fc1 = torch.nn.Linear(10, 10)
self.fc2 = torch.nn.Linear(10, 10)
def forward(self, x):
x = x.view(-1)
x = self.fc1(x)
x = torch.relu(x)
x = self.fc2(x)
return x
def hook_fn(module, input, output):
if torch.isnan(output).any():
raise RuntimeError("NaN検出")
model = MyModel()
model.register_module_forward_hook(hook_fn)
# 入力データ
x = torch.randn(10, 10)
# モデルの推論
try:
model(x)
except RuntimeError as e:
print(e)
勾配計算の変更
import torch
class MyModel(torch.nn.Module):
def __init__(self):
super().__init__()
self.fc1 = torch.nn.Linear(10, 10)
self.fc2 = torch.nn.Linear(10, 10)
def forward(self, x):
x = x.view(-1)
x = self.fc1(x)
x = torch.relu(x)
x = self.fc2(x)
return x
def hook_fn(module, input, output):
if module == model.fc2:
# 勾配を2倍にする
output.backward = lambda grad: grad * 2
model = MyModel()
model.register_module_forward_hook(hook_fn)
# 入力データ
x = torch.randn(10, 10)
# モデルの推論
loss = model(x).sum()
loss.backward()
# fc2層の重みの勾配を確認
print(model.fc2.weight.grad)
カスタム層の実装
import torch
class MyCustomLayer(torch.nn.Module):
def __init__(self):
super().__init__()
def forward(self, x):
# ここにカスタム処理を記述
return x
def hook_fn(module, input, output):
if isinstance(module, MyCustomLayer):
# カスタム層の処理をフックで変更
output = ...
model = torch.nn.Sequential(
torch.nn.Linear(10, 10),
MyCustomLayer(),
torch.nn.Linear(10, 10),
)
model.register_module_forward_hook(hook_fn)
# 入力データ
x = torch.randn(10, 10)
# モデルの推論
model(x)
PyTorch の公式ドキュメントやチュートリアルを参照して、詳細を確認することをおすすめします。
PyTorch ニューラルネットワークにおけるモジュールの出力取得
モジュールの属性への直接アクセス
モジュールの出力は、モジュールの属性 output
としてアクセスできます。
import torch
class MyModel(torch.nn.Module):
def __init__(self):
super().__init__()
self.fc1 = torch.nn.Linear(10, 10)
self.fc2 = torch.nn.Linear(10, 10)
def forward(self, x):
x = x.view(-1)
x = self.fc1(x)
x = torch.relu(x)
x = self.fc2(x)
return x
model = MyModel()
# 入力データ
x = torch.randn(10, 10)
# モデルの推論
out = model(x)
# fc2層の出力
fc2_out = model.fc2.output
torch.nn.utils.model_to_graph
を使用して、モデルのグラフ表現を取得できます。グラフ表現から、各モジュールの出力を取り出すことができます。
import torch
from torch.nn.utils import model_to_graph
class MyModel(torch.nn.Module):
def __init__(self):
super().__init__()
self.fc1 = torch.nn.Linear(10, 10)
self.fc2 = torch.nn.Linear(10, 10)
def forward(self, x):
x = x.view(-1)
x = self.fc1(x)
x = torch.relu(x)
x = self.fc2(x)
return x
model = MyModel()
# モデルのグラフ表現を取得
graph = model_to_graph(model, example_inputs=torch.randn(10, 10))
# fc2層の出力ノードを取得
fc2_out_node = next(n for n in graph.nodes() if n.op == "aten::linear")
# fc2層の出力
fc2_out = fc2_out_node.outputs[0]
torch.onnx.export
を使用して、モデルを ONNX 形式でエクスポートできます。ONNX 形式は、ニューラルネットワークモデルを表現するためのオープンフォーマットです。ONNX ファイルから、各モジュールの出力を取り出すことができます。
import torch
import torch.onnx
class MyModel(torch.nn.Module):
def __init__(self):
super().__init__()
self.fc1 = torch.nn.Linear(10, 10)
self.fc2 = torch.nn.Linear(10, 10)
def forward(self, x):
x = x.view(-1)
x = self.fc1(x)
x = torch.relu(x)
x = self.fc2(x)
return x
model = MyModel()
# モデルを ONNX 形式でエクスポート
torch.onnx.export(model, torch.randn(10, 10), "model.onnx")
# ONNX ファイルから fc2 層の出力を取り出す
# ...
上記の方法のどれを選択するかは、目的に応じて異なります。
- シンプルな方法で出力を取り出す場合は、モジュールの属性への直接アクセスがおすすめです。
- より複雑な処理を行う場合は、
torch.nn.utils.model_to_graph
またはtorch.onnx.export
の利用を検討してください。
パフォーマンス向上:PyTorch Dataset と DataLoader でデータローディングを最適化する
Datasetは、データセットを表す抽象クラスです。データセットは、画像、テキスト、音声など、機械学習モデルの学習に使用できるデータのコレクションです。Datasetクラスは、データセットを読み込み、処理するための基本的なインターフェースを提供します。
PyTorch Miscellaneous: torch.testing.assert_close() の詳細解説
torch. testing. assert_close() は、PyTorch テストモジュール内にある関数で、2つのテンソルの要素がほぼ等しいことを確認するために使用されます。これは、テストコードで計算結果の正確性を検証する際に役立ちます。
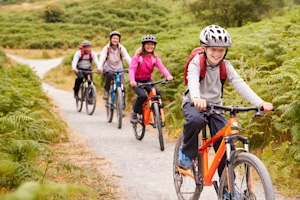
PyTorchのC++バックトレースを取得:torch.utils.get_cpp_backtraceの使い方
torch. utils. get_cpp_backtrace は、PyTorch の C++ バックトレースを取得するための関数です。これは、C++ コードで発生したエラーのデバッグに役立ちます。機能この関数は、現在のスレッドの C++ バックトレースをリストとして返します。各要素は、フレームの情報を含むディクショナリです。
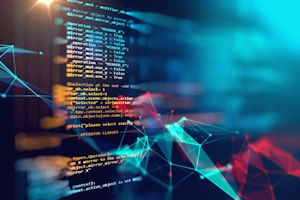
PyTorchで事前学習済みモデルを使う:torch.utils.model_zoo徹底解説
torch. utils. model_zoo でモデルをロードするには、以下のコードを使用します。このコードは、ImageNet データセットで事前学習済みの ResNet-18 モデルをダウンロードしてロードします。torch. utils

PyTorch Miscellaneous: 隠れた機能 torch.overrides.wrap_torch_function()
PyTorchは、機械学習アプリケーション開発のためのオープンソースライブラリです。torch. overrides. wrap_torch_function() は、PyTorchの「Miscellaneous」カテゴリに属する関数で、既存のPyTorch関数をオーバーライドするための機能を提供します。
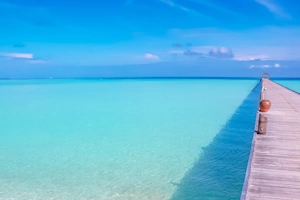
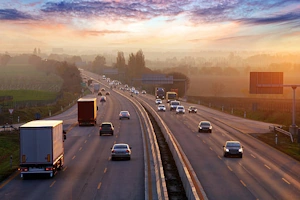
PyTorchのisinstance関数とtorch.is_tensor関数の違いとは?
torch. is_tensor関数は、以下の引数を受け取ります。obj: テンサーであるかどうかを確認したいオブジェクトこの関数は、以下のいずれかの条件を満たす場合にTrueを返し、それ以外の場合はFalseを返します。obj が torch
【初心者向け】PyTorchで自動微分を使いこなす!「torch.autograd.function.FunctionCtx.mark_dirty()」の役割と使い方
PyTorchは、機械学習タスクに広く利用されているオープンソースのライブラリです。その中でも、「Automatic Differentiation」と呼ばれる機能は、勾配計算を自動的に行うことで、ニューラルネットワークなどのモデルの訓練を効率化します。
PyTorchでガンマ分布のエントロピーを計算する: torch.distributions.gamma.Gamma.entropy() 関数徹底解説
torch. distributions. gamma. Gamma. entropy()は、PyTorchの確率分布モジュールにおいて、ガンマ分布のエントロピーを計算する関数です。この関数は、確率密度関数の対数を取って期待値を計算することで、ガンマ分布のエントロピーを計算します。
PyTorch Linear Algebra: torch.linalg.vander() の徹底解説
torch. linalg. vander は、Vandermonde行列を生成する関数です。Vandermonde行列は、ベクトルの各要素のべき乗を列ベクトルとして並べた行列です。この関数は、PyTorchの線形代数ライブラリ torch
PyTorch FX の torch.fx.Interpreter.output() を使ったカスタム FX 変換
PyTorch FX は、PyTorch モデルのキャプチャ、変換、最適化のためのフレームワークです。torch. fx. Interpreter. output() は、FX グラフを実行し、その出力を取得するための関数です。torch