PyTorch Profiler で torch.profiler._KinetoProfile.export_stacks() 関数を使ってスタックトレースを書き出す
torch.profiler._KinetoProfile.export_stacks()
関数は、PyTorch Profilerを使用して取得したプロファイリング結果から、各イベントのスタックトレースをファイルに書き出すための関数です。この関数は、パフォーマンスのボトルネックを特定し、コードの問題をデバッグするのに役立ちます。
使用方法
export_stacks()
関数は、以下の引数を受け取ります。
- path: スタックトレースを書き出すファイルパス
- metric: スタックトレースに含めるメトリクス。デフォルトは
self_cpu_time_total
です。
例
import torch
from torch.profiler import profile
with profile(activities=[ProfilerActivity.CPU, ProfilerActivity.CUDA], with_stack=True) as prof:
model(inputs)
prof.export_stacks("/tmp/profiler/stacks_cpu.txt", "self_cpu_time_total")
prof.export_stacks("/tmp/profiler/stacks_cuda.txt", "self_cuda_time_total")
上記コードは、CPUとCUDAの両方のパフォーマンスを計測し、それぞれのスタックトレースをstacks_cpu.txt
とstacks_cuda.txt
ファイルに書き出す例です。
出力ファイルには、各イベントのスタックトレースがJSON形式で書き出されます。各イベントには、以下の情報が含まれます。
- イベント名
- 開始時間
- 終了時間
- 経過時間
- スタックトレース
スタックトレースの読み方
スタックトレースは、イベントが発生した時点のコードの呼び出し履歴を表します。各フレームには、以下の情報が含まれます。
- ファイル名
- 行番号
- 関数名
注意事項
export_stacks()
関数は、with_stack=True
オプションを指定してプロファイリングを実行した場合にのみ使用できます。- 出力ファイルは、Kineto Profilerなどのツールで読み込むことができます。
応用例
- パフォーマンスのボトルネックを特定する
- コードの問題をデバッグする
- 異なるコードパスのパフォーマンスを比較する
torch.profiler._KinetoProfile.export_stacks()
関数は、PyTorch Profilerを使用して取得したプロファイリング結果から、各イベントのスタックトレースをファイルに書き出すための関数です。この関数は、パフォーマンスのボトルネックを特定し、コードの問題をデバッグするのに役立ちます。
torch.profiler._KinetoProfile.export_stacks() 関数のサンプルコード
CPUとCUDAの両方のパフォーマンスを計測し、それぞれのスタックトレースをファイルに書き出す
import torch
from torch.profiler import profile
with profile(activities=[ProfilerActivity.CPU, ProfilerActivity.CUDA], with_stack=True) as prof:
model(inputs)
prof.export_stacks("/tmp/profiler/stacks_cpu.txt", "self_cpu_time_total")
prof.export_stacks("/tmp/profiler/stacks_cuda.txt", "self_cuda_time_total")
特定のイベントのスタックトレースのみをファイルに書き出す
import torch
from torch.profiler import profile
with profile(activities=[ProfilerActivity.CPU], with_stack=True) as prof:
model(inputs)
# 特定のイベントのスタックトレースのみを書き出す
event = prof.kineto_profile.events[0]
event.export_stacks("/tmp/profiler/stacks_event.txt", "self_cpu_time_total")
スタックトレースに含めるメトリクスを指定する
import torch
from torch.profiler import profile
with profile(activities=[ProfilerActivity.CPU], with_stack=True) as prof:
model(inputs)
prof.export_stacks("/tmp/profiler/stacks.txt", "self_cpu_time_total,nvtx_time_total")
出力ファイル形式を指定する
import torch
from torch.profiler import profile
with profile(activities=[ProfilerActivity.CPU], with_stack=True) as prof:
model(inputs)
prof.export_stacks("/tmp/profiler/stacks.json", "self_cpu_time_total", output_format="json")
上記コードは、出力ファイル形式をJSON形式に指定する例です。
Kineto Profiler でスタックトレースを開く
kineto_profiler -open /tmp/profiler/stacks.json
上記コマンドは、Kineto Profiler で stacks.json
ファイルを開く例です。
- 上記のサンプルコードは、PyTorch Profiler の基本的な使い方を示しています。
- 詳細については、PyTorch Profiler のドキュメントを参照してください。
torch.profiler._KinetoProfile.export_stacks() 関数の代替方法
torch.profiler.export_stacks() 関数を使う
import torch
from torch.profiler import profile, export_stacks
with profile(activities=[ProfilerActivity.CPU], with_stack=True) as prof:
model(inputs)
export_stacks(prof, "/tmp/profiler/stacks.txt", "self_cpu_time_total")
上記コードは、torch.profiler.export_stacks()
関数を使ってスタックトレースをファイルに書き出す例です。
自作のコードでスタックトレースを取得する
import torch
from torch.profiler import profile
with profile(activities=[ProfilerActivity.CPU], with_stack=True) as prof:
model(inputs)
for event in prof.kineto_profile.events:
# イベントの開始時間と終了時間を使って、自作のコードでスタックトレースを取得
start_time = event.start_time
end_time = event.start_time + event.duration
stacks = get_stacks(start_time, end_time)
# スタックトレースをファイルに書き出す
with open("/tmp/profiler/stacks.txt", "a") as f:
f.write(f"Event: {event.name}\n")
for frame in stacks:
f.write(f" {frame.filename}:{frame.lineno} {frame.function}\n")
上記コードは、自作のコードでスタックトレースを取得し、ファイルに書き出す例です。
これらのツールは、PyTorch Profiler だけでなく、その他のフレームワークや言語で実行されるプログラムのパフォーマンスを分析することができます。
torch.profiler._KinetoProfile.export_stacks()
関数は、PyTorch Profiler で取得したプロファイリング結果からスタックトレースをファイルに書き出すための便利な関数です。しかし、いくつかの代替方法も存在します。これらの方法を理解することで、ニーズに合った方法を選択することができます。
パフォーマンス向上:PyTorch Dataset と DataLoader でデータローディングを最適化する
Datasetは、データセットを表す抽象クラスです。データセットは、画像、テキスト、音声など、機械学習モデルの学習に使用できるデータのコレクションです。Datasetクラスは、データセットを読み込み、処理するための基本的なインターフェースを提供します。
PyTorch Miscellaneous モジュール:ディープラーニング開発を効率化するユーティリティ
このモジュールは、以下のサブモジュールで構成されています。データ処理torch. utils. data:データセットの読み込み、バッチ化、シャッフルなど、データ処理のためのツールを提供します。 DataLoader:データセットを効率的に読み込み、イテレートするためのクラス Dataset:データセットを表す抽象クラス Sampler:データセットからサンプルを取得するためのクラス
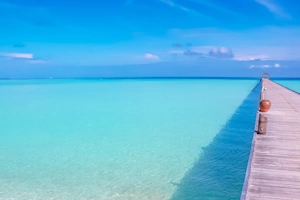
PyTorchで事前学習済みモデルを使う:torch.utils.model_zoo徹底解説
torch. utils. model_zoo でモデルをロードするには、以下のコードを使用します。このコードは、ImageNet データセットで事前学習済みの ResNet-18 モデルをダウンロードしてロードします。torch. utils

PyTorch Miscellaneous: torch.testing.assert_close() の詳細解説
torch. testing. assert_close() は、PyTorch テストモジュール内にある関数で、2つのテンソルの要素がほぼ等しいことを確認するために使用されます。これは、テストコードで計算結果の正確性を検証する際に役立ちます。
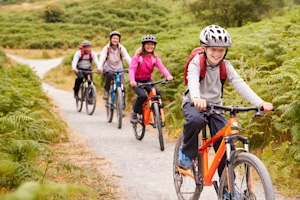
PyTorch Miscellaneous: torch.utils.cpp_extension.get_compiler_abi_compatibility_and_version() の概要
torch. utils. cpp_extension. get_compiler_abi_compatibility_and_version() は、C++ 拡張モジュールをビルドする際に、現在のコンパイラが PyTorch と互換性があるかどうかを確認するために使用されます。
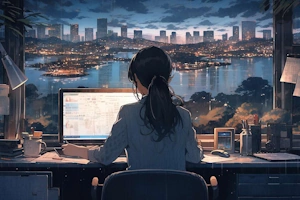
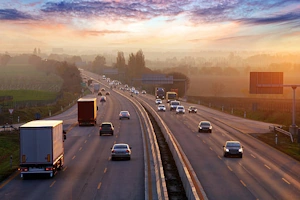
PyTorch Storage とは?Tensor との関係を分かりやすく解説
torch. TypedStorage. long()は、torch. Storage オブジェクトを作成する関数です。この関数は、以下の引数を受け取ります。size: 作成されるストレージのバイト数dtype: ストレージ内のデータ型torch
PyTorchでニューラルネットワークのバックプロパゲーションを制御する方法
このチュートリアルでは、PyTorchのニューラルネットワークにおける重要な機能の一つであるバックプロパゲーションフックについて、特にtorch. nn. Module. register_full_backward_hook()メソッドに焦点を当てて詳細に解説します。
PyTorch Tensor の特異値分解 (torch.Tensor.svd) のサンプルコード
torch. Tensor. svd は、PyTorch の Tensor に対して特異値分解 (SVD) を実行する関数です。SVD は、行列を 3 つの行列の積に分解する方法です。式SVD は、以下の式で表されます。ここで、A: 元の行列
PyTorch torch.renorm 関数:勾配クリッピング、ニューラルネットワークの安定化、L_p ノルム制限など
機能概要対象となるテンソル内の各行または列に対して L_p ノルムを計算します。指定された maxnorm 値を超えるノルムを持つ行または列を、maxnorm 値でスケーリングします。入力テンソルと同じ形状の出力テンソルを返します。引数input: 処理対象の入力テンソル
torch.distributions.dirichlet.Dirichlet.mean メソッドによる計算
PyTorchは、Pythonで機械学習を行うためのオープンソースライブラリです。torch. distributionsモジュールは、確率分布を扱うための機能を提供します。Dirichletクラスは、ディリクレ分布を表すクラスです。Dirichlet分布とは