Qt WidgetsにおけるQCheckBox::minimumSizeHint()の解説
Qt WidgetsにおけるQCheckBox::minimumSizeHint()の解説
QCheckBox::minimumSizeHint()
メソッドは、Qt Widgetsにおける QCheckBox
ウィジェットの最小推奨サイズを計算します。このサイズは、ウィジェットのコンテンツ (テキスト、アイコンなど) に基づいて算出され、ウィジェットが適切に表示されるために必要な最小限の領域を表します。
使用方法
minimumSizeHint()
メソッドは、以下のコードのように呼び出すことができます。
QSize minimumSize = checkBox.minimumSizeHint();
このコードは、checkBox
という名前の QCheckBox
ウィジェットの最小推奨サイズを取得し、minimumSize
変数に格納します。
計算方法
minimumSizeHint()
メソッドは、以下の要素を考慮して最小推奨サイズを計算します。
- テキスト: チェックボックスのラベルテキストの長さ
- アイコン: チェックボックスに設定されているアイコンのサイズ
- マージン: チェックボックスのコンテンツ周辺のマージン
- スタイル: ウィジェットのスタイル設定
例
以下のコード例は、minimumSizeHint()
メソッドを使用して、チェックボックスの最小推奨サイズを計算し、ウィジェットのサイズを設定する方法を示しています。
QCheckBox checkBox;
checkBox.setText("長いラベルテキスト");
checkBox.setIcon(QIcon(":/icon.png"));
QSize minimumSize = checkBox.minimumSizeHint();
checkBox.setMinimumSize(minimumSize);
このコードは、長いラベルテキストとアイコンを持つチェックボックスを作成し、minimumSizeHint()
メソッドを使用して最小推奨サイズを計算します。次に、このサイズを setMinimumSize()
メソッドを使用してウィジェットのサイズに設定します。
補足
minimumSizeHint()
メソッドは、あくまでも推奨サイズであり、ウィジェットの実際のサイズはレイアウトやその他の要因によって異なる場合があります。- ウィジェットのサイズを制限する必要がある場合は、
setMinimumSize()
メソッドを使用する代わりに、setMaximumSize()
メソッドを使用することもできます。
上記以外にも、QCheckBox
ウィジェットには様々な機能とプロパティがあります。詳細は Qt Widgets API ドキュメントを参照してください。
ご不明な点がございましたら、お気軽にご質問ください。
Here are some examples in Python:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QCheckBox, QVBoxLayout
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('QCheckBox')
checkbox1 = QCheckBox('Option 1', self)
checkbox2 = QCheckBox('Option 2', self)
checkbox3 = QCheckBox('Option 3', self)
vbox = QVBoxLayout()
vbox.addWidget(checkbox1)
vbox.addWidget(checkbox2)
vbox.addWidget(checkbox3)
self.setLayout(vbox)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
This code creates a simple window with three checkboxes. Each checkbox has a label associated with it. The checkboxes are arranged vertically in a box layout.
Here is an example in C++:
#include <QApplication>
#include <QCheckBox>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWidget window;
window.setWindowTitle("QCheckBox");
QCheckBox checkBox1("Option 1", &window);
checkBox1.move(10, 10);
QCheckBox checkBox2("Option 2", &window);
checkBox2.move(10, 40);
QCheckBox checkBox3("Option 3", &window);
checkBox3.move(10, 70);
window.show();
return app.exec();
}
This code creates a simple window with three checkboxes. Each checkbox has a label associated with it. The checkboxes are positioned on the window using the move()
method.
Please let me know if you would like to see examples in other languages.
Connecting signals to slots
You can connect the stateChanged()
signal of a QCheckBox
to a slot in your application to perform actions when the checkbox is checked or unchecked. For example, the following code connects the stateChanged()
signal of a checkbox to a slot that prints a message to the console:
QCheckBox checkBox;
checkBox.connect(checkBox.stateChanged(), &Example, &Example::onStateChanged);
void Example::onStateChanged(int state) {
if (state == Qt::Checked) {
qDebug() << "Checkbox is checked";
} else {
qDebug() << "Checkbox is unchecked";
}
}
Using groups
You can group checkboxes together using QButtonGroup
. This can be useful for ensuring that only one checkbox in a group can be checked at a time. For example, the following code creates a group of three checkboxes and ensures that only one checkbox can be checked at a time:
QCheckBox checkBox1;
QCheckBox checkBox2;
QCheckBox checkBox3;
QButtonGroup group;
group.addButton(&checkBox1);
group.addButton(&checkBox2);
group.addButton(&checkBox3);
group.setExclusive(true);
Using styles
You can customize the appearance of a QCheckBox
using styles. For example, the following code sets the font of the checkbox label to bold:
QCheckBox checkBox;
checkBox.setFont(QFont("Arial", 12, QFont::Bold));
Using custom drawing
You can create custom checkboxes by subclassing QCheckBox
and implementing the paintEvent()
method. This allows you to draw the checkbox yourself and control its appearance completely.
In addition to these methods, there are many other features of QCheckBox
that you can explore. For more information, please refer to the Qt Widgets API documentation: https://doc.qt.io/qt-6/qcheckbox.html
Please let me know if you have any other questions.
まとめ:QTextDocument::availableRedoSteps() 関数をマスターしてテキスト編集を快適に
QTextDocument::availableRedoSteps() 関数は、テキストドキュメントに対してやり直し可能な操作の数を取得するために使用されます。これは、ユーザーがテキスト編集中に誤った操作を行った場合に、元に戻す操作と同様に、やり直し操作を使用して誤操作を修正するのに役立ちます。
Qt GUIにおける描画変換:QPainter::combinedTransform()の完全ガイド
QPainter::combinedTransform()関数は、現在のペインター状態におけるワールド変換とビュー変換の積を表すQTransformオブジェクトを取得するために使用されます。これは、描画されるすべての形状とテキストに適用される最終的な変換を理解する上で重要です。
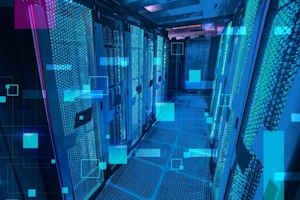
Qt GUIにおけるQFont::Style (enum)の分かりやすい解説
概要QFont::Styleは、Qt GUIで使用されるフォントスタイルを表す列挙型です。この型は、フォントの傾斜と太さを指定するために使用されます。以下の値を持つ: QFont::StyleNormal:通常のスタイル QFont::StyleItalic:斜体 QFont::StyleOblique:斜体 QFont::StyleBold:太字
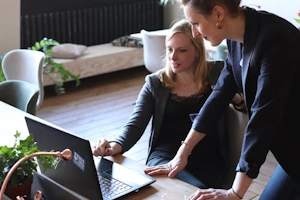
Qt GUI プログラミング:QStandardItem::takeRow() 関数の詳細解説
QStandardItem::takeRow() は、Qt GUI フレームワークの QStandardItemModel クラスで使用される関数です。これは、モデル内の指定された行を削除し、削除された行のアイテムへのポインターのリストを返します。モデルはアイテムの所有権を解放します。
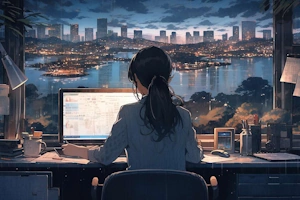
Qt GUI アプリケーションにおけるフォーカス管理:QWindow::focusObjectChanged() シグナルの徹底解説
QWindow::focusObjectChanged() は、Qt GUI アプリケーションにおける重要なシグナルの一つです。これは、フォーカスを受け取るオブジェクトが変更されたときに発生し、開発者がそれに応じて適切な処理を行うための機能を提供します。
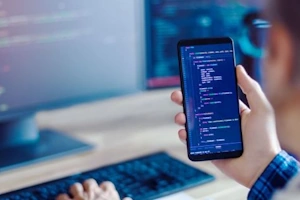
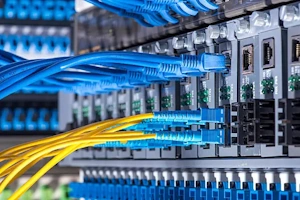
QAbstractItemView::itemDelegateForColumn()の完全ガイド
Qtのモデル/ビューアーアーキテクチャは、データと視覚表現を分離することで、柔軟で再利用可能なUI開発を可能にします。このアーキテクチャでは、以下の主要なコンポーネントが役割を果たします。モデル (QAbstractItemModel): データを格納し、データへのアクセスを提供します。
Qt Widgets: QStatusBar::paintEvent()でステータスバーをカスタマイズ
QStatusBar::paintEvent()は以下の役割を果たします。ステータスバーの背景を描画ステータスバーに配置されたウィジェットを描画一時的なメッセージを描画このイベントは、ステータスバーの状態が変化するたびに発生します。例えば、ウィジェットの追加・削除、メッセージの表示・非表示などです。
Qt Widgets:ステータスバーをマスターしてユーザーインターフェースを改善する
現在の状態や進捗状況に関する情報を表示テキストメッセージ、アイコン、ウィジェットなどを表示複数領域に分割して異なる情報を表示一時的なメッセージと永続的なメッセージを区別QMainWindow::statusBar()を使って、メインウィンドウのステータスバーを取得
言語モデルの応用例:QTabWidget::tabRemoved()シグナルを駆使したQtプログラミング
概要QTabWidget::tabRemoved() は、QTabWidget ウィジェットからタブが削除されたときに発生するシグナルです。このシグナルは、タブの削除に関する情報を提供し、タブの削除に関連する処理を実行するために使用できます。
Qt GUI アプリケーションにおける OpenGL グラフィックスプログラミングの基礎:QOpenGLContext::functions() の使い方
QOpenGLContext::functions() は、Qt GUI で OpenGL グラフィックスを使用する際に、OpenGL 関数へのアクセスを提供する重要な関数です。この関数は、OpenGL バージョンに基づいた適切な関数ポインタを取得し、アプリケーション内で安全に使用できるようにします。