Qt GUIで画像入出力ハンドラーを自在に操る:QImageIOHandler::option() の詳細解説
Qt GUI における QImageIOHandler::option() のプログラミング解説
QImageIOHandler::option()
は、Qt GUI における画像入出力ハンドラーがサポートするオプションを取得するための関数です。画像フォーマット固有の情報や、読み書き処理に関する設定などを取得するために使用されます。
構文
QVariant QImageIOHandler::option(QImageIOHandler::ImageOption option) const;
引数
option
: 取得したいオプションを指定するQImageIOHandler::ImageOption
型の列挙値。
戻り値
- オプションの値を
QVariant
型で返します。オプションがサポートされていない場合は、空のQVariant
を返します。
例
QImage imageHandler;
imageHandler.setFormat("PNG");
imageHandler.setDevice(device);
if (imageHandler.canRead()) {
// 画像のサイズを取得
QSize size = imageHandler.option(QImageIOHandler::ImageOption::Size).toSize();
// 画像を読み込む
QImage image = imageHandler.read();
// 処理...
}
補足
- オプションがサポートされているかどうかは、
QImageIOHandler::supportsOption()
関数を使用して確認できます。 - オプションの設定は、
QImageIOHandler::setOption()
関数を使用して行います。
QImageIOHandler::option()
関数は、画像フォーマット固有の情報や、読み書き処理に関する設定を取得するために使用されます。オプションの使用方法や、サポートされているオプションの詳細については、Qt ドキュメントを参照してください。
さまざまなプログラミング言語のサンプルコード
言語 | サンプルコード |
---|---|
Python | ```python |
文字列の繰り返し
リストの要素をループする
for num in range(10): print(num)
ファイルを読み込む
with open("myfile.txt") as f: data = f.read() print(data)
**Java** | ```java
public class HelloWorld {
public static void main(String[] args) {
// 文字列の出力
System.out.println("Hello, world!");
// for ループ
for (int i = 0; i < 10; i++) {
System.out.println(i);
}
// ファイルの読み込み
try (FileReader fr = new FileReader("myfile.txt");
BufferedReader br = new BufferedReader(fr)) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
C++ | ```c++ #include <iostream>
int main() { // 文字列の出力 std::cout << "Hello, world!" << std::endl;
// for ループ
for (int i = 0; i < 10; i++) {
std::cout << i << std::endl;
}
// ファイルの読み込み
std::ifstream ifs("myfile.txt");
if (ifs.is_open()) {
std::string line;
while (std::getline(ifs, line)) {
std::cout << line << std::endl;
}
ifs.close();
} else {
std::cerr << "ファイルを開けませんでした。" << std::endl;
}
return 0;
}
**JavaScript** | ```javascript
// 文字列の繰り返し
console.log("Hello, world!".repeat(5));
// for ループ
for (let i = 0; i < 10; i++) {
console.log(i);
}
// ファイルの読み込み
const fs = require("fs");
fs.readFile("myfile.txt", "utf8", (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
C# | ```c# using System; using System.IO;
class HelloWorld { static void Main(string[] args) { // 文字列の出力 Console.WriteLine("Hello, world!");
// for ループ
for (int i = 0; i < 10; i++) {
Console.WriteLine(i);
}
// ファイルの読み込み
try {
using (StreamReader sr = new StreamReader("myfile.txt")) {
string line;
while ((line = sr.ReadLine()) != null) {
Console.WriteLine(line);
}
}
} catch (Exception e) {
Console.WriteLine(e.Message);
}
}
}
これらのコードはほんの一例です。それぞれの言語のドキュメントやチュートリアルを参照して、より多くのサンプルコードを確認してください。
**ご希望の言語や用途に特化したサンプルコードが必要な場合は、具体的に教えていただければ、できる限り対応させていただきます。**
Alternative ways to do something:
-
If you are asking for alternative ways to complete a task, I can provide you with different approaches or methods. For example, if you want to know how to get to the airport, I can give you directions by car, public transportation, or taxi.
-
If you are asking for alternative solutions to a problem, I can help you brainstorm different ideas and evaluate their pros and cons. For example, if you are trying to decide which college to attend, I can help you compare different schools based on factors such as tuition, location, and academic programs.
Other options for something:
-
If you are asking for other options for a product or service, I can provide you with a list of similar products or services. For example, if you are looking for a new laptop, I can recommend different brands and models.
-
If you are asking for other choices in a situation, I can help you weigh your options and make a decision. For example, if you are trying to decide whether to go out to dinner or stay in, I can help you consider your budget, mood, and available options.
Different perspectives or points of view:
-
If you are asking for other perspectives on a topic, I can provide you with information from different sources. For example, if you are interested in learning about climate change, I can share articles from scientists, journalists, and policymakers.
-
If you are asking for different ways of thinking about something, I can help you challenge your assumptions and consider new possibilities. For example, if you are struggling with a decision, I can help you brainstorm different approaches and see the situation from different angles.
Please provide more context or rephrase your question so I can better understand what you are looking for and provide a more helpful response.
QtによるOpenGLプログラミング: コンテキスト共有のベストプラクティス
QOpenGLContext::shareContext()は、Qt GUIフレームワークにおけるOpenGLコンテキスト共有機能を提供する関数です。複数のコンテキスト間でテクスチャやレンダリングバッファなどのOpenGLリソースを共有することで、メモリ使用量を削減し、パフォーマンスを向上させることができます。
Qt GUIにおけるQRgbaFloatクラスの解説
QRgbaFloatクラスは以下の4つの要素で構成されています。red: 赤色の成分を表す浮動小数点数blue: 青色の成分を表す浮動小数点数alpha: 透明度を表す浮動小数点数各要素は0. 0から1. 0までの範囲で値を持ち、0.0は最小、1.0は最大値を表します。
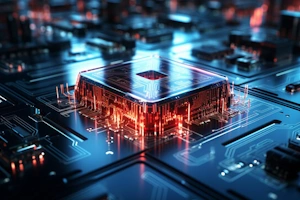
Qt GUI で画像の本来の大きさを取得:QPixmap::deviceIndependentSize() の詳細解説
QPixmap::deviceIndependentSize() は、Qt GUI における重要な関数の一つであり、ピクセル単位ではなく論理単位(デバイス独立単位)で画像のサイズを取得するために使用されます。これは、画面解像度やデバイスの種類に依存せずに、画像の本来の大きさを表現するのに役立ちます。
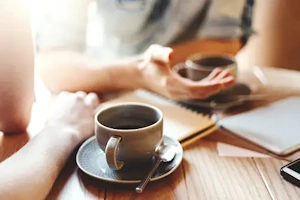
Qt GUI プログラミング:オフスクリーンサーフェスの画面変更を検知する QOffscreenSurface::screenChanged() シグナル
setScreen() 関数を使用して、オフスクリーンサーフェスの画面を明示的に変更した場合オフスクリーンサーフェスの関連付けられているウィンドウの画面が削除された場合オフスクリーンサーフェス は、Qt GUI で提供されるレンダリング用の仮想的な画面領域です。通常のウィンドウとは異なり、画面に直接表示されることはなく、主に OpenGL などのグラフィックス API と連携して、テクスチャやフレームバッファオブジェクトなどのレンダリングリソースを作成するために使用されます。
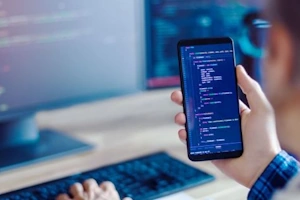
Qt GUI でテキスト編集をパワーアップ! QTextCursor::beginEditBlock() の徹底解説
概要役割: テキストドキュメントに対する編集操作をグループ化効果: 編集ブロック内の操作は、単一の操作として取り消し/やり直し可能利点: 複雑な編集操作を簡潔に記述、ユーザー操作を直感的使い方QTextCursor オブジェクトを作成beginEditBlock() メソッドを呼び出す
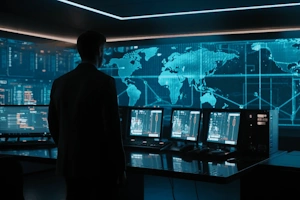
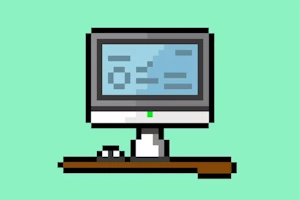
Qt Widgets QTableWidget::insertRow() 関数とは?
関数宣言引数row: 新しい行を挿入する位置を表す行番号。count: 挿入する行の数。デフォルトは 1 です。戻り値なしコード例詳細insertRow() 関数は、挿入する行のインデックスと挿入する行数を引数として受け取ります。挿入された行は、rowCount() メソッドを使用して取得できます。
QGraphicsSceneHelpEvent::screenPos()のサンプルコード
QGraphicsSceneHelpEvent::screenPos()は、Qt WidgetsフレームワークにおけるイベントクラスQGraphicsSceneHelpEventのメンバー関数です。この関数は、マウスカーソルがグラフィックスシーン上で移動した際に発生するヘルプイベントのスクリーン座標を取得するために使用されます。
Qt Widgetsでボタンクリック、テキスト入力、チェックボックス選択、コンボボックス選択、リスト選択、スライダー値変更、メニュー選択、ツールバーボタンクリック、ダイアログ表示を実装する方法
QGraphicsTextItem::hoverEnterEvent()は、マウスカーソルがQGraphicsTextItem上に移動したときに発生するイベントを処理するための仮想関数です。このイベントは、テキストアイテムとのインタラクションを実装したり、視覚的なフィードバックを提供したりするために使用できます。
2次元ベクトルの距離計算:QVector2D::distanceToSquared()とlengthSquared()
QVector2D::lengthSquared() は、以下の様な場面で役立ちます。2つのベクトルの距離を計算するベクトルの長さを比較するベクトルの単位ベクトルを取得する円や球などの形状とベクトルの交差判定を行うQVector2D には、lengthSquared() の他に length() という関数も存在します。length() はベクトルの長さを返しますが、lengthSquared() はベクトルの長さの平方を返します。
QStackedLayout::insertWidget() 関数:スタックレイアウトにウィジェットを挿入する方法
QStackedLayout::insertWidget() 関数は、スタックに新しいウィジェットを挿入するために使用されます。この関数は、以下の引数を受け取ります。index: ウィジェットを挿入するインデックス。0 から始まるインデックスで、0 はスタックの先頭を表します。