テキストエディタで選択されたテキストを操作・処理する魔法のメソッド:QTextCursor::selectedText()
Qt GUIプログラミングにおける QTextCursor::selectedText() の詳細ガイド
QTextCursor::selectedText()
メソッドは、Qt GUIアプリケーションにおいて、テキストエディタなどのウィジェットで現在選択されているテキストを取得するために使用されます。このメソッドは、選択されたテキストを操作したり、処理したりする際に非常に役立ちます。
使用方法
QTextCursor::selectedText()
メソッドを使用するには、まず、テキストエディタウィジェットの現在のテキストカーソルを取得する必要があります。これは、textCursor()
メソッドを使用して行うことができます。次に、取得したテキストカーソルを使用して、selectedText()
メソッドを呼び出し、選択されたテキストを取得します。
QTextCursor cursor = textEdit->textCursor();
QString selectedText = cursor.selectedText();
例
以下の例は、テキストエディタウィジェットで現在選択されているテキストを取得し、それをラベルウィジェットに表示する方法を示しています。
void MainWindow::on_textEdit_selectionChanged()
{
QTextCursor cursor = textEdit->textCursor();
QString selectedText = cursor.selectedText();
label->setText(selectedText);
}
詳細
QTextCursor::selectedText()
メソッドは、選択されたテキストだけでなく、そのテキストの書式も返します。書式情報は、QTextCharFormat
クラスを使用して取得できます。
QTextCursor cursor = textEdit->textCursor();
QString selectedText = cursor.selectedText();
QTextCharFormat format = cursor.characterFormat();
// フォント情報
QFont font = format.font();
QString fontFamily = font.family();
int fontSize = font.pointSize();
// テキストの色
QColor textColor = format.textColor();
その他のメソッド
QTextCursor
クラスには、選択されたテキストを操作するための他にもさまざまなメソッドがあります。以下に、よく使用されるメソッドをいくつか示します。
movePosition()
: カーソル位置を移動します。select()
: テキストを選択します。removeSelectedText()
: 選択されたテキストを削除します。insertText()
: 選択されたテキストの代わりに新しいテキストを挿入します。
QTextCursor::selectedText()
メソッドは、Qt GUIアプリケーションにおいて、テキストエディタなどのウィジェットで現在選択されているテキストを取得するために非常に役立つメソッドです。このメソッドを使用することで、選択されたテキストを操作したり、処理したりすることができます。
ファイル入出力
#include <iostream>
#include <fstream>
using namespace std;
int main() {
// ファイルを開く
ofstream ofs("output.txt");
if (!ofs.is_open()) {
cerr << "ファイルを開くことができませんでした" << endl;
return 1;
}
// ファイルに書き込む
ofs << "Hello, World!" << endl;
ofs << "This is a sample text." << endl;
// ファイルを閉じる
ofs.close();
// ファイルを読み込む
ifstream ifs("input.txt");
if (!ifs.is_open()) {
cerr << "ファイルを開くことができませんでした" << endl;
return 1;
}
string line;
while (getline(ifs, line)) {
cout << line << endl;
}
// ファイルを閉じる
ifs.close();
return 0;
}
文字列操作
#include <iostream>
#include <string>
using namespace std;
int main() {
// 文字列の作成
string str = "Hello, World!";
// 文字列の長さ
int length = str.length();
cout << "文字列の長さ: " << length << endl;
// 文字列へのアクセス
char ch = str[5];
cout << "5番目の文字: " << ch << endl;
// 部分文字列
string substring = str.substr(7, 5);
cout << "部分文字列: " << substring << endl;
// 文字列の検索
int pos = str.find("World");
if (pos != string::npos) {
cout << "'World' が見つかりました: " << pos << endl;
} else {
cout << "'World' が見つかりませんでした" << endl;
}
// 文字列の置換
str.replace(7, 5, "C++");
cout << "置換後の文字列: " << str << endl;
// 文字列の大小変換
str.toUpper();
cout << "大文字に変換: " << str << endl;
str.toLower();
cout << "小文字に変換: " << str << endl;
return 0;
}
数値演算
#include <iostream>
using namespace std;
int main() {
// 整数型
int x = 10;
int y = 20;
int sum = x + y;
cout << "x + y = " << sum << endl;
int diff = x - y;
cout << "x - y = " << diff << endl;
int product = x * y;
cout << "x * y = " << product << endl;
int quotient = x / y;
cout << "x / y = " << quotient << endl;
int remainder = x % y;
cout << "x % y = " << remainder << endl;
// 浮動小数点型
double d1 = 3.14;
double d2 = 2.718;
double sumD = d1 + d2;
cout << "d1 + d2 = " << sumD << endl;
double diffD = d1 - d2;
cout << "d1 - d2 = " << diffD << endl;
double productD = d1 * d2;
cout << "d1 * d2 = " << productD << endl;
double quotientD = d1 / d2;
cout << "d1 / d2 = " << quotientD << endl;
return 0;
}
条件分岐
#include <iostream>
using namespace std;
int main() {
int score = 80;
if (score >= 90) {
cout << "A" << endl;
} else if (score >= 80) {
cout << "B" << endl;
} else if (score >= 70) {
cout << "C" << endl;
} else {
cout << "D" << endl;
}
return 0;
}
To give you more specific guidance, I need more context or details about what you're trying to achieve. For instance, do you want to:
-
Perform actions on the selected text: If so, please explain what actions you want to perform, such as replacing, deleting, or formatting the selected text.
-
Manipulate the selected text content: If so, please clarify what kind of manipulations you want to do, such as converting to uppercase, extracting specific information, or performing calculations.
-
Handle user interactions: If so, please describe the user interaction scenario and how you want to use the selected text in response to the user's actions.
Once you provide more details, I can provide you with more tailored examples and explanations.
In the meantime, here are some additional resources that might be helpful:
Please let me know if you have any other questions or need further assistance.
逆変換でQt GUIの2Dグラフィックスを自在に操る:QTransform::adjoint()徹底解説
概要:QTransformクラスは、2D座標系の変換を表すためのクラスです。adjoint()は、QTransformオブジェクトの逆行列の転置行列を計算します。逆行列の転置行列は、逆変換を行うために使用されます。逆変換は、元の座標系に戻すための操作です。
まとめ:QTextDocument::availableRedoSteps() 関数をマスターしてテキスト編集を快適に
QTextDocument::availableRedoSteps() 関数は、テキストドキュメントに対してやり直し可能な操作の数を取得するために使用されます。これは、ユーザーがテキスト編集中に誤った操作を行った場合に、元に戻す操作と同様に、やり直し操作を使用して誤操作を修正するのに役立ちます。
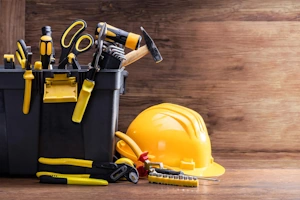
Qt GUIで入力エラーを防ぐ:QValidatorの使い方
概要QValidator::~QValidator() は、Qt GUIにおける入力検証クラス QValidator のデストラクタ関数です。この関数は、QValidator オブジェクトが破棄されるときに自動的に呼び出され、オブジェクトが占有していたメモリなどのリソースを解放します。
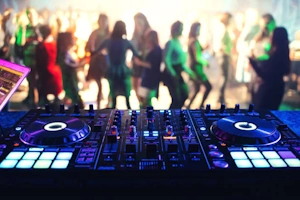
QTextLayout::clearFormats() 関数の詳細解説
QTextLayout は、Qt GUIにおけるテキストレンダリングの基盤となるクラスです。テキストレイアウトは、テキストを画面に表示するための様々な属性を保持します。これらの属性には、フォント、色、サイズ、配置などが含まれます。QTextLayout::clearFormats() は、テキストレイアウトに設定されたすべてのフォーマット設定をクリアします。つまり、テキストはデフォルトのフォント、色、サイズで表示されるようになります。
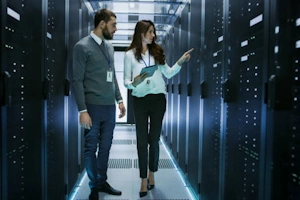
QTextTableFormat::setAlignment() 関数の使い方
QTextTableFormat::setAlignment() は、Qt GUI でテキストテーブルの配置を設定するために使用する関数です。この関数は、テーブル内のテキストを水平方向と垂直方向にどのように配置するかを指定します。引数alignment : テキストの配置を指定する Qt::Alignment 型の値。
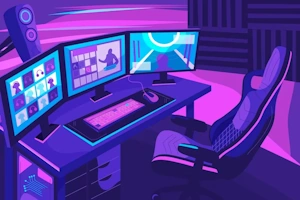
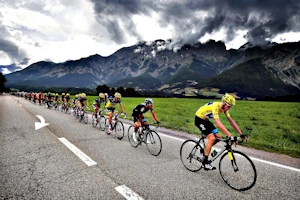
Qt GUI プログラミング:QColor::getHsl() で HSL 値を簡単に取得!
QColor::getHsl() は、Qt GUI における QColor クラスのメソッドであり、指定された色の HSL (Hue, Saturation, Lightness) 値を取得するために使用されます。HSL は、人間の視覚システムに直感的に対応する色空間であり、色相、彩度、明度を表します。
QOpenGLExtraFunctions::glProgramUniform3ui() 関数によるユニフォーム変数の設定
この関数は、3つの整数値をGLuint型ユニフォーム変数に設定するために使用されます。シェーダープログラムでユニフォーム変数を使用する前に、この関数を使って値を設定する必要があります。QOpenGLExtraFunctions::glProgramUniform3ui() の概要:
Qt GUIにおける QSurfaceFormat::setStencilBufferSize() の詳細解説
QSurfaceFormat::setStencilBufferSize() は、Qt GUI アプリケーションでステンシルバッファのサイズを設定するために使用する関数です。ステンシルバッファは、ピクセルごとに 1 ビットの情報を持つバッファで、主に以下の用途で使用されます。
Qt Widgets: ドックウィジェット領域のトラブルシューティング!QMainWindow::dockWidgetArea()のFAQ
QMainWindowは、中央ウィジェットとドックウィジェット領域と呼ばれる複数の領域で構成されています。ドックウィジェット領域は、メインウィンドウの周囲に配置され、ツールバーやパレットなどの補助的なウィジェットを表示するために使用されます。
Qt GUI における Vulkan デバイス取得:QVulkanWindow::device() 関数で実現
概要QVulkanWindow::device() 関数は、Qt GUI における Vulkan アプリケーションで、現在使用されている論理デバイスを取得するためのものです。このデバイスは、Vulkan API を介してグラフィックス レンダリングなどの操作を実行するために使用されます。