QTextFragment::charFormatIndex()でテキスト断片の書式情報を取得する方法
Qt GUIにおけるQTextFragment::charFormatIndex()解説
概要
- クラス: QTextFragment
- 関数: charFormatIndex()
- 戻り値: int型 - 文字フォーマットのインデックス
- 用途: 特定のテキスト断片に適用される文字フォーマットを取得
詳細
QTextDocumentは、豊富な書式設定機能を備えたテキスト処理クラスです。テキスト文書内の各文字には、フォント、色、サイズなどの書式情報が個別に設定できます。これらの書式情報は、QTextCharFormatクラスによって表現されます。
QTextFragmentは、QTextDocument内の連続したテキスト断片を表します。一つのテキスト断片は、一連の文字フォーマットを持つことができます。charFormatIndex()関数は、指定されたテキスト断片に適用される文字フォーマットのインデックスを取得します。
コード例
// QTextDocumentの作成
QTextDocument document;
// テキストの追加
document.setPlainText("This is a text with different formats.");
// 特定のテキスト断片の取得
QTextFragment fragment = document.findFragment("different formats");
// 文字フォーマットインデックスの取得
int formatIndex = fragment.charFormatIndex();
// インデックスを使用して、文字フォーマットを取得
QTextCharFormat format = document.characterFormat(formatIndex);
// 取得した文字フォーマットで何か処理を行う
// 例:フォントファミリーを変更する
format.setFontFamily("Arial");
document.setCharFormat(formatIndex, format);
補足
- charFormatIndex()関数は、QTextDocument::characterFormat関数と組み合わせて、特定のテキスト断片に適用される文字フォーマットの詳細情報を取得することができます。
- 文字フォーマットは、QTextDocument::setDefaultCharFormat()関数を使用して、デフォルト値を設定することができます。
- QTextDocumentクラスは、豊富なテキスト処理機能を提供しており、さまざまな書式設定や編集操作を行うことができます。
Qt GUIにおけるQTextFragment::charFormatIndex()のサンプルコード
テキストの色とフォントを変更する
QTextDocument document;
document.setPlainText("This is a text with different formats.");
// "different formats"の部分の色とフォントを変更
QTextFragment fragment = document.findFragment("different formats");
int formatIndex = fragment.charFormatIndex();
QTextCharFormat format = document.characterFormat(formatIndex);
format.setFontFamily("Arial");
format.setForeground(Qt::red);
document.setCharFormat(formatIndex, format);
// テキストを表示
QTextEdit textEdit;
textEdit.setDocument(&document);
textEdit.show();
テキストのサイズを変更する
QTextDocument document;
document.setPlainText("This is a text with different sizes.");
// "different sizes"の部分のサイズを変更
QTextFragment fragment = document.findFragment("different sizes");
int formatIndex = fragment.charFormatIndex();
QTextCharFormat format = document.characterFormat(formatIndex);
format.setFontPointSize(16);
document.setCharFormat(formatIndex, format);
// テキストを表示
QTextEdit textEdit;
textEdit.setDocument(&document);
textEdit.show();
太字と下線を設定する
QTextDocument document;
document.setPlainText("This is a text with bold and underline.");
// "bold and underline"の部分に太字と下線を設定
QTextFragment fragment = document.findFragment("bold and underline");
int formatIndex = fragment.charFormatIndex();
QTextCharFormat format = document.characterFormat(formatIndex);
format.setFontWeight(QFont::Bold);
format.setUnderlineStyle(QTextCharFormat::UnderlineSolid);
document.setCharFormat(formatIndex, format);
// テキストを表示
QTextEdit textEdit;
textEdit.setDocument(&document);
textEdit.show();
文字列の一部分のみ書式を変更する
QTextDocument document;
document.setPlainText("This is a text with different formats. This is another part.");
// "different formats"のみ色を変更
QTextFragment fragment = document.findFragment("different formats");
int formatIndex = fragment.charFormatIndex();
QTextCharFormat format = document.characterFormat(formatIndex);
format.setForeground(Qt::blue);
document.setCharFormat(formatIndex, format);
// テキストを表示
QTextEdit textEdit;
textEdit.setDocument(&document);
textEdit.show();
QTextFragment::charFormatIndex() 以外の方法
QTextCursor::charFormat()
QTextCursor クラスは、テキスト文書内のカーソル位置を表します。QTextCursor::charFormat() 関数は、カーソル位置にある文字断片の文字フォーマットを取得します。
QTextDocument document;
document.setPlainText("This is a text with different formats.");
// カーソルを "different formats" の先頭に移動
QTextCursor cursor = document.findCursor("different formats");
// カーソル位置の文字フォーマットを取得
QTextCharFormat format = cursor.charFormat();
// ...
QTextDocument::findCharFormat() 関数は、指定された文字フォーマットを持つ最初のテキスト断片を見つけて、そのインデックスを返します。
QTextDocument document;
document.setPlainText("This is a text with different formats.");
// "different formats" の文字フォーマットを見つける
QTextCharFormat format;
format.setFontFamily("Arial");
int formatIndex = document.findCharFormat(format);
// ...
QTextDocument::characterAt() 関数は、指定された位置にある文字とその文字フォーマットを取得します。
QTextDocument document;
document.setPlainText("This is a text with different formats.");
// "different formats" の最初の文字とその文字フォーマットを取得
int position = document.find("different formats").position();
QChar character = document.characterAt(position);
QTextCharFormat format = document.characterFormat(position);
// ...
これらの方法は、それぞれ異なるユースケースに適しています。状況に応じて適切な方法を選択してください。
Qt GUI で QTextLayout::drawCursor() を使う
この関数の使い方を理解することで、以下のようなことができます。テキストエディタでカーソル位置をリアルタイムで表示するリッチテキストエディタで選択範囲をハイライトする入力候補を表示するQTextLayout::drawCursor() の基本的な使い方は以下の通りです。
画像の歪み、回転、透視変換... 全部できる! QTransform::quadToQuad() のサンプルコード集
quad1: 変換前の四角形の頂点座標を格納する QPolygonF 型のオブジェクトtransform: 変換行列を格納する QTransform 型のオブジェクトquadToQuad() は、変換が可能な場合は true を返し、不可能な場合は false を返します。
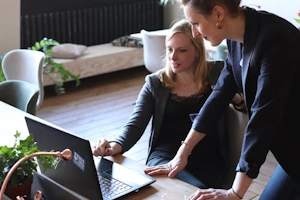
QUndoStack::undoTextChanged()関数によるUndo/Redo機能の実装
QUndoStack::undoTextChanged()は以下の機能を提供します。テキスト編集操作を1つずつ元に戻す/やり直す元に戻す/やり直す履歴を管理元に戻す/やり直す操作をプログラムで制御以下のコードは、QUndoStackとQTextEditを使用して、テキスト編集操作を元に戻す/やり直す機能を実装する例です。
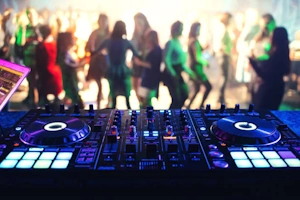
Qt GUI アプリケーションにおける undo/redo 機能のサンプルコード集
QUndoStack::createUndoAction() は、Qt GUI アプリケーションでundo/redo機能を実装するための重要な関数です。この関数は、QUndoStack にプッシュされたコマンドに基づいて、undoアクションを作成します。
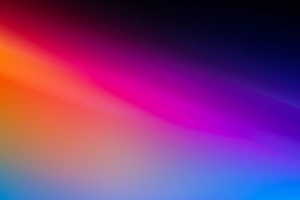
Qt GUI で QTextList::itemNumber() 関数を使用して特定の項目にアクセスする方法
概要QTextList::itemNumber() 関数は、QTextList オブジェクト内の特定の QTextBlock がリスト内のどの項目に対応しているのかを調べ、そのインデックスを返します。もし、その QTextBlock がリスト内に存在しない場合は、-1 を返します。
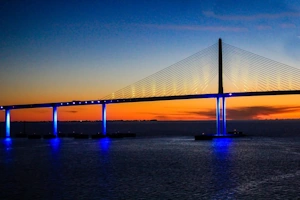
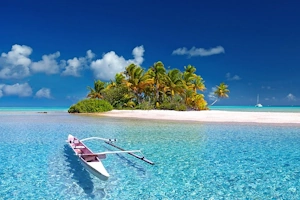
Qt WidgetsにおけるQGraphicsSceneContextMenuEvent::pos()とは?
概要クラス: QGraphicsSceneContextMenuEvent関数: pos()戻り値: QPointF型 - イベントが発生したシーン上の座標用途: コンテキストメニューを表示する場所を決定する詳細QGraphicsSceneContextMenuEvent::pos()は、イベントが発生したシーン座標をQPointF型で返します。この座標は、ウィジェット座標とは異なることに注意が必要です。ウィジェット座標は、ウィジェットの左上隅を原点とする座標系です。一方、シーン座標は、シーンの左上隅を原点とする座標系です。
Qt Widgets の QToolBox ウィジェットのスタイルをカスタマイズする他の方法
QStyleOptionToolBox クラスは、Qt Widgets モジュールにおける QToolBox ウィジェットのスタイルオプションを定義します。このクラスは、QToolBox の外観をカスタマイズするために使用されます。主な機能
QPixmap::transformed() 関数で画像を回転させる
QPixmap::transformed() 関数は、Qt GUI ライブラリにおいて、QPixmap オブジェクト (画像データ) に対して様々な変換を適用し、その結果を新しい QPixmap オブジェクトとして返す機能を提供します。画像の回転、拡大縮小、移動などの操作を簡潔かつ効率的に実現できます。
Qt GUIでクリエイティブな表現を実現:描画と塗りつぶしの可能性
Qtでは、様々な形状をキャンバスに描画することができます。代表的な形状と描画方法は以下の通りです。点:線:四角形:楕円形:ポリゴン:上記以外にも、曲線、テキスト、画像などを描画することも可能です。描画の詳細については、Qtドキュメント https://doc
QScrollerProperties::FrameRatesのサンプルコード
QScrollerProperties::FrameRatesは、Qt Widgetsモジュールでスクロールアニメーションのフレームレートを制御するために使用される列挙型です。スクロールの滑らかさやパフォーマンスに影響を与える重要なプロパティです。