プログラミング初心者でも安心!CMake で HIP デバイスコードを生成する方法
CMake 変数 CMAKE_HIP_ARCHITECTURES の詳細解説
CMAKE_HIP_ARCHITECTURES
は、CMake のターゲットプロパティで、HIP デバイスコードを生成する GPU アーキテクチャのリストを指定するために使用されます。これは、HIP ランタイムがサポートするすべてのアーキテクチャをデフォルトで含みますが、必要に応じてユーザーによってオーバーライドできます。
構文
CMAKE_HIP_ARCHITECTURES="<architecture1>;<architecture2>;..."
例
set(CMAKE_HIP_ARCHITECTURES "gfx803;gfx900;gfx908")
この例では、HIP デバイスコードは gfx803
、gfx900
、gfx908
アーキテクチャをターゲットにして生成されます。
詳細
CMAKE_HIP_ARCHITECTURES
変数は、ターゲットが作成されるときに設定されます。- アーキテクチャ名は、
CMAKE_HIP_PLATFORM
に基づいて解釈されます。 - HIP コンパイルモデルには、全体と分離の 2 つのモードがあります。
- 全体コンパイル: コンパイル時にデバイスコードを生成します。
- 分離コンパイル: リンク時にデバイスコードを生成します。
CMAKE_HIP_ARCHITECTURES
変数は、HIP_ARCHITECTURES
ターゲットプロパティを初期化するために使用されます。
補足
CMAKE_HIP_ARCHITECTURES
変数は、CMake 3.21 以降で使用できます。- AMD ROCm プラットフォームを使用している場合、この変数は自動的に設定されます。
- NVIDIA プラットフォームを使用している場合は、この変数を手動で設定する必要があります。
プログラミング例
cmake_minimum_required(VERSION 3.21)
project(hip_example)
set(CMAKE_HIP_ARCHITECTURES "gfx803;gfx900;gfx908")
add_executable(hip_example hip_example.cpp)
target_link_libraries(hip_example ROCm::hip)
この例では、hip_example
という名前のターゲットが作成されます。このターゲットは hip_example.cpp
ファイルからコンパイルされ、ROCm::hip
ライブラリにリンクされます。CMAKE_HIP_ARCHITECTURES
変数は gfx803
、gfx900
、gfx908
アーキテクチャに設定されているため、HIP デバイスコードはこれらのアーキテクチャをターゲットにして生成されます。
CMAKE_HIP_ARCHITECTURES
変数は、HIP デバイスコードを生成する GPU アーキテクチャを指定するために使用されます。これは、CMake のターゲットプロパティで、必要に応じてユーザーによってオーバーライドできます。この変数は、全体と分離の 2 つの HIP コンパイルモードで使用できます。
この説明が、CMAKE_HIP_ARCHITECTURES
変数と CMake で HIP プログラミングを行う方法を理解するのに役立つことを願っています。
Fibonacci sequence
def fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n - 1) + fibonacci(n - 2)
print(fibonacci(10))
This code calculates the Fibonacci sequence up to the 10th term.
Hello world
#include <stdio.h>
int main() {
printf("Hello, world!\n");
return 0;
}
This code prints "Hello, world!" to the console.
Sorting an array
function sortArray(array) {
for (let i = 0; i < array.length; i++) {
for (let j = 0; j < array.length - i - 1; j++) {
if (array[j] > array[j + 1]) {
[array[j], array[j + 1]] = [array[j + 1], array[j]];
}
}
}
return array;
}
console.log(sortArray([5, 2, 4, 1, 3]));
This code sorts an array of numbers using the bubble sort algorithm.
Creating a class
class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
public class Main {
public static void main(String[] args) {
Person person = new Person("John Doe", 30);
System.out.println(person);
}
}
This code creates a class called Person
with two attributes: name
and age
. It also defines methods to get and set these attributes, and a toString()
method that returns a string representation of the object.
Making an HTTP request
import requests
url = "https://www.example.com"
response = requests.get(url)
if response.status_code == 200:
print(response.text)
else:
print("Error:", response.status_code)
This code makes an HTTP GET request to the URL https://www.example.com
and prints the response body to the console.
These are just a few examples of the many different types of code that can be written. The specific code that you need will depend on the specific task that you are trying to accomplish.
Please let me know if you have any other questions.
Here are some possible interpretations of your question and potential responses:
Alternative ways to do something:
If you are asking about alternative ways to do something, I can provide you with different approaches or techniques to achieve the same outcome. For example, if you are asking about how to cook an egg, I could provide you with instructions for boiling, frying, or poaching an egg.
Different types of methods:
If you are asking about different types of methods, I can provide you with examples of methods from various fields or disciplines. For example, if you are asking about different types of scientific methods, I could provide you with examples of experimental, observational, and theoretical methods.
Specific methods for a particular task:
If you are asking about specific methods for a particular task, I can provide you with detailed instructions or code examples. For example, if you are asking about how to create a website, I could provide you with instructions on how to use HTML, CSS, and JavaScript.
Please provide more information about your specific question or request so I can give you a more helpful response.
CMakeの"Commands"における"fltk_wrap_ui()"プログラミングを徹底解説!
この解説では、CMakeの"Commands"における"fltk_wrap_ui()"プログラミングについて、分かりやすく説明します。"fltk_wrap_ui()"は、CMakeでFLTK GUIアプリケーションをビルドするために使用されるマクロです。このマクロは、FLTK GUI定義ファイルをC++コードに変換し、プロジェクトに組み込みます。
プログラミング初心者でもわかる!CMake の "set_directory_properties()" コマンドの使い方
set_directory_properties() コマンドは、CMakeプロジェクト内のディレクトリとサブディレクトリにプロパティを設定するために使用されます。これらのプロパティは、ビルドプロセス、インストール、その他の CMake 動作を制御するために使用できます。
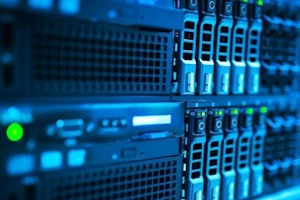
CMakeのCommandsにおけるuse_mangled_mesa()
use_mangled_mesa() は CMake の Commands における関数で、Mesa ライブラリの mangled シンボル名を解決するために使用されます。Mesa は OpenGL の実装であり、古いバージョンの Mesa ではシンボル名が mangled されるため、use_mangled_mesa() を使用してこれらのシンボル名を解決する必要があります。
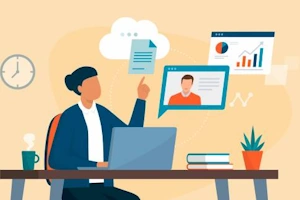
CMake find_file() コマンドの代替方法:もっと柔軟なファイル検索
<variable>: 検索結果を格納する CMake 変数<file_names>: 検索するファイル名のリスト (スペース区切り)<path_list>: 検索するパス名のリスト (スペース区切り)<options>: 検索オプション (後述)
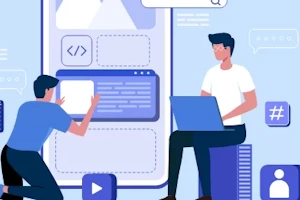
CMakeにおける"get_target_property()"コマンド: ターゲットの情報を自在に操る
get_target_property()コマンドは、CMakeプロジェクトで定義されたターゲットからプロパティを取得するために使用されます。ターゲットプロパティは、ターゲットのビルド方法や動作を制御するために使用される情報です。構文引数VAR: ターゲットプロパティの値を格納する変数名
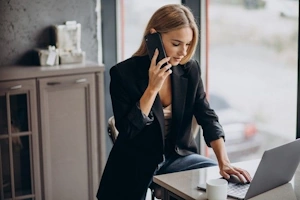
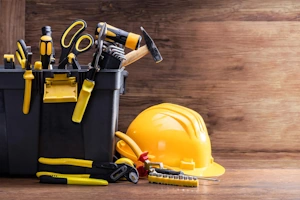
CMake find_libraryコマンドとfind_packageモジュールの比較
find_library() コマンドは、CMake で外部ライブラリを見つけるために使用されます。これは、プロジェクトに必要なライブラリを自動的に検出して、ビルドプロセスを簡略化するのに役立ちます。コマンドフォーマット<VAR>: 検索結果を格納する変数名
CMake の VISIBILITY_INLINES_HIDDEN プロパティとは?
VISIBILITY_INLINES_HIDDEN は、CMake のターゲットプロパティの一つであり、インライン化された関数の可視性を制御します。このプロパティを設定することで、コンパイル時にインライン化された関数を外部モジュールから見えないようにすることができます。
C言語コンパイラとCMakeの連携を強化! CMAKE_C_KNOWN_FEATURES で開発効率アップ
CMAKE_C_KNOWN_FEATURESは、CMakeのグローバルスコーププロパティであり、C言語コンパイラで利用可能なC言語機能のリストを格納します。このプロパティは、ターゲットのコンパイル時に特定の機能を有効化/無効化するために使用できます。
CMakeLists.txtファイルで重複するソースファイルエントリを処理する方法
CMake ポリシー CMP0089 は、target_sources コマンドでソースファイルのリストを指定する際に、重複するエントリを許可するかどうかに影響します。デフォルトでは無効化されており、重複するエントリが存在するとエラーが発生します。
コードブロック内でのHTMLタグ使用
概要CMAKE_OPTIMIZE_DEPENDENCIES は、CMake 3.19 以降で導入された変数で、静的ライブラリの依存関係を最適化するための機能を提供します。静的ライブラリは、実行時に直接リンクされるのではなく、ビルド時に他のライブラリやオブジェクトファイルに埋め込まれます。そのため、静的ライブラリの依存関係を最適化することで、リンク時間の短縮やビルドサイズの削減などが期待できます。