QTextCharFormatとQTextTableFormatを組み合わせて、さらに高度な書式設定
Qt GUIにおけるQTextFrameFormatクラス
主な機能
- 枠線
- スタイル、幅、色を設定
- 余白
- 上、下、左、右の余白を設定
- 配置
- フレームをページ内での配置
- 高さ
- フレームの高さを設定
- 背景
- 背景色、画像、パターンを設定
コード例
#include <QTextFrameFormat>
// 新しいフレームフォーマットを作成
QTextFrameFormat format;
// 枠線設定
format.setBorderStyle(QTextFrameFormat::BorderStyle::Solid);
format.setBorderWidth(2);
format.setBorderBrush(Qt::red);
// 余白設定
format.setTopMargin(10);
format.setBottomMargin(10);
format.setLeftMargin(15);
format.setRightMargin(15);
// 配置設定
format.setPosition(QTextFrameFormat::Position::FloatLeft);
// 高さ設定
format.setHeight(100);
// 背景設定
format.setBackground(Qt::gray);
// フレームフォーマットを適用
QTextFrame *frame = new QTextFrame;
frame->setFrameFormat(format);
補足
- QTextFrameFormatは、QTextDocumentクラスと密接に関連しています。
- フレームフォーマットは、QTextCursorクラスを使用して、既存のフレームにも適用できます。
- より複雑な書式設定には、QTextTableFormatクラスなどの他のクラスを使用できます。
関連キーワード
- Qt
- Qt GUI
- QTextFrame
- QTextDocument
- QTextCursor
- 書式設定
QTextFrameFormatクラスのサンプルコード
枠線付きフレーム
QTextFrameFormat format;
format.setBorderStyle(QTextFrameFormat::BorderStyle::Solid);
format.setBorderWidth(2);
format.setBorderBrush(Qt::red);
QTextFrame *frame = new QTextFrame;
frame->setFrameFormat(format);
// テキストを追加
QTextCursor cursor(frame);
cursor.insertText("枠線付きフレーム");
余白付きフレーム
QTextFrameFormat format;
format.setTopMargin(10);
format.setBottomMargin(10);
format.setLeftMargin(15);
format.setRightMargin(15);
QTextFrame *frame = new QTextFrame;
frame->setFrameFormat(format);
// テキストを追加
QTextCursor cursor(frame);
cursor.insertText("余白付きフレーム");
中央配置フレーム
QTextFrameFormat format;
format.setPosition(QTextFrameFormat::Position::Center);
QTextFrame *frame = new QTextFrame;
frame->setFrameFormat(format);
// テキストを追加
QTextCursor cursor(frame);
cursor.insertText("中央配置フレーム");
画像付き背景フレーム
QTextFrameFormat format;
format.setBackground(QPixmap("image.png"));
QTextFrame *frame = new QTextFrame;
frame->setFrameFormat(format);
// テキストを追加
QTextCursor cursor(frame);
cursor.insertText("画像付き背景フレーム");
複数列フレーム
QTextFrameFormat format;
format.setColumnCount(2);
QTextFrame *frame = new QTextFrame;
frame->setFrameFormat(format);
// テキストを追加
QTextCursor cursor(frame);
cursor.insertText("1列目のテキスト\n");
cursor.insertText("2列目のテキスト");
これらのサンプルコードは、QTextFrameFormatクラスの基本的な機能を理解するのに役立ちます。さらに複雑な書式設定には、Qt公式ドキュメントやその他のチュートリアルを参照することを推奨します。
QTextFrameFormatクラスを使用するその他の方法
QTextCharFormatクラスとの組み合わせ
QTextCharFormatクラスは、文字レベルでの書式設定を制御するために使用されます。QTextFrameFormatクラスと組み合わせることで、フレーム内のテキストの書式をさらに細かく制御することができます。
例:
QTextFrameFormat format;
format.setBorderStyle(QTextFrameFormat::BorderStyle::Solid);
format.setBorderWidth(2);
format.setBorderBrush(Qt::red);
QTextCharFormat charFormat;
charFormat.setFontPointSize(16);
charFormat.setFontWeight(QFont::Bold);
QTextFrame *frame = new QTextFrame;
frame->setFrameFormat(format);
// テキストを追加
QTextCursor cursor(frame);
cursor.insertText("枠線付きフレーム", charFormat);
QTextTableFormatクラスとの組み合わせ
QTextTableFormatクラスは、テーブルレイアウトを持つテキストフレームを作成するために使用されます。QTextFrameFormatクラスと組み合わせることで、テーブルの書式設定を制御することができます。
例:
QTextFrameFormat format;
format.setBackground(Qt::gray);
QTextTableFormat tableFormat;
tableFormat.setBorderStyle(QTextTableFormat::BorderStyle::Solid);
tableFormat.setBorderWidth(1);
tableFormat.setCellSpacing(5);
QTextFrame *frame = new QTextFrame;
frame->setFrameFormat(format);
// テーブルを作成
QTextTable *table = new QTextTable(frame);
table->setFormat(tableFormat);
table->appendRows(2);
table->appendColumns(2);
// セルにテキストを追加
QTextCursor cursor(table->cellAt(0, 0));
cursor.insertText("セル1");
cursor = QTextCursor(table->cellAt(1, 1));
cursor.insertText("セル4");
その他の機能
- テキストの回り込みを設定
- フレームの影を設定
- フレームのフロー制御を設定
これらの機能の詳細については、Qt公式ドキュメントを参照してください。
Qt GUI で Vulkan レンダリングを行うための QVulkanWindow クラス
setFlags() 関数は、以下の引数を受け取ります。flags: 設定するフラグのビットマスクこの関数は、設定されたフラグに基づいてウィンドウの動作を変更します。この例では、ウィンドウを Qt::Window フラグと Qt::Vulkan フラグで初期化しています。
【コード例付き】Qt GUIでセルデータを効率的に扱う!QTextTableCell::operator=()徹底解説
QTextTableCell::operator=()は、Qt GUIライブラリにおける重要な機能の一つであり、テキストテーブルセル内のデータを効率的にコピーおよび割り当てを行うための演算子です。この演算子を用いることで、コードをより簡潔かつ読みやすく保ち、メンテナンス性を向上させることができます。

QTextTableFormat::setAlignment() 関数の使い方
QTextTableFormat::setAlignment() は、Qt GUI でテキストテーブルの配置を設定するために使用する関数です。この関数は、テーブル内のテキストを水平方向と垂直方向にどのように配置するかを指定します。引数alignment : テキストの配置を指定する Qt::Alignment 型の値。
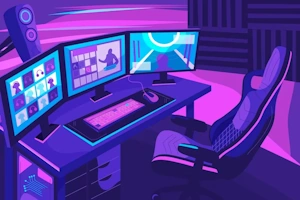
Qt GUIにおけるQTextTableCellFormat::setTopPadding()の詳細解説
QTextTableCellFormat::setTopPadding()は、Qt GUIフレームワークにおいて、テーブルセルの上部余白を設定するための関数です。この関数を用いることで、セル内のテキストと上部の境界線との間に垂直方向のスペースを調整できます。
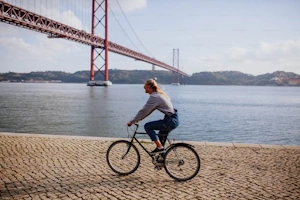
Qt GUIにおけるQVector3D::operator*=()によるスカラー値乗算
役割: ベクトルの各要素をスカラー値または別のベクトルで乗算引数: scalar: スカラー値 vector: 別のQVector3Dオブジェクトscalar: スカラー値vector: 別のQVector3Dオブジェクト戻り値: 現在のベクトル自身 (乗算結果を反映)
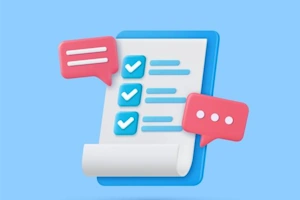
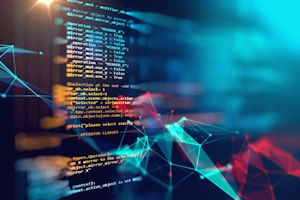
Qt WidgetsにおけるQListWidgetクラスの基本的な使い方
Qt WidgetsのQListWidgetクラスは、項目リストを表示するための便利なウィジェットです。シンプルなテキストリストから、アイコンや画像付きの複雑なリストまで、幅広い用途に使用できます。主な機能項目の追加、削除、編集項目の選択と状態管理
Qtチュートリアル:QWidget::setDisabled() 関数
QWidget::setDisabled() 関数は、Qt ウィジェットを無効または有効にします。無効化されたウィジェットは、ユーザー入力を受け付けなくなり、視覚的にグレーアウトされます。関数宣言引数disabled: ウィジェットを無効にする場合は true、有効にする場合は false を指定します。
Qt Widgetsで日時を操作する:QDateTimeEdit::stepBy()完全ガイド
QDateTimeEdit::stepBy()は、Qt WidgetsのQDateTimeEditクラスで提供される関数です。この関数は、QDateTimeEditウィジェットに表示されている日時を指定されたステップ数だけ増減するために使用されます。
Qt WidgetsにおけるQTableView::setSpan()の徹底解説
QTableView::setSpan()は、Qt Widgets モジュールにおける重要な関数の一つです。これは、テーブルビュー内の複数のセルを結合して、あたかも一つの大きなセルのように表示するために使用されます。この機能は、表内の関連するデータをまとめて表示したり、見やすくするために使用できます。
Qt WidgetsにおけるQProgressBar::sizeHint() 関数の徹底解説
役割: QProgressBarウィジェットの推奨サイズを取得引数: なし戻り値: QSize型の値。ウィジェットの推奨幅と高さを表す関連クラス: QProgressBar関連ヘッダーファイル: <QProgressBar>推奨サイズとはQProgressBar::sizeHint() 関数は、ウィジェットのコンテンツを適切に表示するために必要な最小限のサイズを返します。このサイズは、ウィジェットのスタイル、フォント、テキスト、その他の要素に基づいて計算されます。