CMake の共有ライブラリのリンカフラグを初期化する変数 CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT
CMake 変数 CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT の詳細解説
CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT
は、CMake のビルドプロセスにおいて、共有ライブラリのリンカフラグを初期化する変数です。この変数は、toolchain file
内で設定され、CMake がビルドツリーを初めて構成する際に使用されます。CMake は、環境やターゲットプラットフォームに基づいて、この変数の値に内容を前置または付加することがあります。
詳細
- 目的: 共有ライブラリのリンカフラグを初期化します。
- 設定場所:
toolchain file
- 使用タイミング: CMake がビルドツリーを初めて構成する際
- 処理: CMake は、環境やターゲットプラットフォームに基づいて、この変数の値に内容を前置または付加することがあります。
利点
- 異なるプラットフォームやコンパイラ間で共有ライブラリのリンカフラグを統一的に設定できます。
- ビルドプロセスを簡素化し、コードの移植性を向上させることができます。
例
# toolchain file 例
set(CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT "-Wl,-rpath,${CMAKE_INSTALL_PREFIX}/lib")
この例では、共有ライブラリのリンカフラグに -Wl,-rpath,${CMAKE_INSTALL_PREFIX}/lib
が設定されます。これは、CMake がインストールした共有ライブラリを検索するパスをリンカに指示します。
補足
CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT
は、CMake 3.7 以降で使用できます。
関連変数
CMAKE_SHARED_LINKER_FLAGS
: 共有ライブラリのリンカフラグを設定します。CMAKE_TOOLCHAIN_FILE
:toolchain file
の場所を設定します。
CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT
は、CMake における共有ライブラリのリンカフラグ設定を簡素化し、移植性を向上させるための有用な変数です。
- 本解説は、プログラミングの専門知識を持たない方でも理解しやすいように、分かりやすく記述しています。
- 具体的な設定方法や詳細な技術情報については、上記の情報源を参照してください。
Example 1: Setting a library search path
# toolchain file example
set(CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT "-Wl,-rpath,${CMAKE_INSTALL_PREFIX}/lib")
This example sets the library search path for shared libraries to the directory CMAKE_INSTALL_PREFIX/lib
. This means that CMake will look for shared libraries in this directory when linking an executable.
Example 2: Adding a linker flag for a specific platform
# toolchain file example
if(CMAKE_SYSTEM_NAME MATCHES "Linux")
set(CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT "-Wl,--no-as-needed")
endif()
This example adds the linker flag -Wl,--no-as-needed
to the CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT
variable if the system is Linux. This flag tells the linker to not automatically add dependent libraries to the link command.
Example 3: Using a variable to set a linker flag
# toolchain file example
set(LIBRARY_SEARCH_PATH "${CMAKE_INSTALL_PREFIX}/lib")
set(CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT "-Wl,-rpath,${LIBRARY_SEARCH_PATH}")
This example sets the LIBRARY_SEARCH_PATH
variable to the directory CMAKE_INSTALL_PREFIX/lib
and then uses this variable to set the library search path for shared libraries.
Example 4: Using a function to set a linker flag
# toolchain file example
function(add_linker_flag flag)
set(CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT "${CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT} ${flag}")
endfunction()
add_linker_flag("-Wl,-rpath,${CMAKE_INSTALL_PREFIX}/lib")
This example defines a function add_linker_flag()
that takes a linker flag as input and adds it to the CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT
variable. The function is then called to add the library search path flag -Wl,-rpath,${CMAKE_INSTALL_PREFIX}/lib
.
Example 5: Using a CMakeLists.txt file to set a linker flag
# CMakeLists.txt example
set(CMAKE_TOOLCHAIN_FILE path/to/toolchain.cmake)
project(myproject)
add_executable(myprogram myprogram.cpp)
target_link_libraries(myprogram mylibrary)
This example sets the CMAKE_TOOLCHAIN_FILE
variable to the path of the toolchain file and then uses the target_link_libraries()
command to link the mylibrary
library to the myprogram
executable. The linker flags defined in the toolchain file will be used when linking the executable.
These are just a few examples of how to use the CMAKE_SHARED_LINKER_FLAGS_CONFIG_INIT
variable. There are many other ways to use this variable to configure linker flags for your CMake projects.
I hope this helps! Let me know if you have any other questions.
The CMAKE_SHARED_LINKER_FLAGS
variable can be used to set linker flags for all shared libraries that are linked to a target. This variable can be set in either the CMakeLists.txt file or the toolchain file.
Example:
# CMakeLists.txt example
set(CMAKE_SHARED_LINKER_FLAGS "-Wl,-rpath,${CMAKE_INSTALL_PREFIX}/lib")
project(myproject)
add_executable(myprogram myprogram.cpp)
target_link_libraries(myprogram mylibrary)
This example sets the library search path for shared libraries to the directory CMAKE_INSTALL_PREFIX/lib
. This means that CMake will look for shared libraries in this directory when linking any executable.
Using the target_link_options() command:
The target_link_options()
command can be used to set linker flags for a specific target. This command can be used in the CMakeLists.txt file to set linker flags for executables, shared libraries, and static libraries.
Example:
# CMakeLists.txt example
project(myproject)
add_executable(myprogram myprogram.cpp)
target_link_options(myprogram "-Wl,-rpath,${CMAKE_INSTALL_PREFIX}/lib")
target_link_libraries(myprogram mylibrary)
This example sets the library search path for the myprogram
executable to the directory CMAKE_INSTALL_PREFIX/lib
. This means that CMake will look for shared libraries in this directory when linking the myprogram
executable.
Using a CMakeLists.txt file to set linker flags per source file:
CMake allows you to set linker flags for specific source files using the target_sources()
command. This can be useful if you need to use different linker flags for different parts of your code.
Example:
# CMakeLists.txt example
project(myproject)
add_executable(myprogram myprogram.cpp foo.cpp bar.cpp)
target_sources(myprogram PUBLIC myprogram.cpp)
target_sources(myprogram PRIVATE foo.cpp)
target_sources(myprogram PRIVATE bar.cpp)
target_link_options(myprogram PUBLIC "-Wl,-rpath,${CMAKE_INSTALL_PREFIX}/lib1")
target_link_options(myprogram PRIVATE "-Wl,-rpath,${CMAKE_INSTALL_PREFIX}/lib2")
target_link_libraries(myprogram mylibrary)
This example sets the library search path for the myprogram
executable to the directory CMAKE_INSTALL_PREFIX/lib1
for the myprogram.cpp
source file and to the directory CMAKE_INSTALL_PREFIX/lib2
for the foo.cpp
and bar.cpp
source files.
Using a custom CMake command:
You can also use a custom CMake command to set linker flags. This can be useful if you need to set linker flags that are not supported by the built-in CMake commands.
Example:
# CMakeLists.txt example
project(myproject)
add_executable(myprogram myprogram.cpp)
add_custom_command(TARGET myprogram
COMMAND ${CMAKE_LINKER} ${CMAKE_ARGS} $<TARGET_SOURCES> $<TARGET_LINK_LIBRARIES> -Wl,-rpath,${CMAKE_INSTALL_PREFIX}/lib)
This example sets the library search path for the myprogram
executable to the directory CMAKE_INSTALL_PREFIX/lib
using a custom CMake command.
These are just a few of the many ways to configure linker flags for your CMake projects. The best method for you will depend on your specific needs.
I hope this helps! Let me know if you have any other questions.
CMakeコマンド「ctest_submit()」でテスト結果をCDashサーバーに送信
ctest_submit()は、CMakeの「Commands」カテゴリに属するコマンドで、テスト結果をCDashなどのダッシュボードサーバーに送信するために使用されます。テスト実行後の結果を可視化、共有したい場合に役立ちます。基本構文オプション解説
プログラミング初心者でもわかる!CMake の "set_directory_properties()" コマンドの使い方
set_directory_properties() コマンドは、CMakeプロジェクト内のディレクトリとサブディレクトリにプロパティを設定するために使用されます。これらのプロパティは、ビルドプロセス、インストール、その他の CMake 動作を制御するために使用できます。
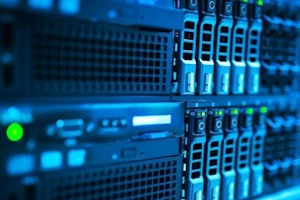
CMake: find_library()とtarget_link_directories()の連携
target_link_directories()コマンドは、CMakeプロジェクト内のターゲットに対して、リンカがライブラリを検索するディレクトリを指定するために使用されます。これは、ターゲットがリンクするライブラリが標準の検索パスに存在しない場合に特に重要です。
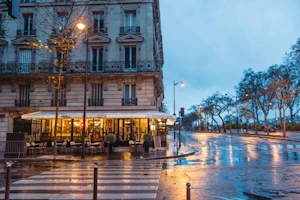
CMakeにおける"get_target_property()"コマンド: ターゲットの情報を自在に操る
get_target_property()コマンドは、CMakeプロジェクトで定義されたターゲットからプロパティを取得するために使用されます。ターゲットプロパティは、ターゲットのビルド方法や動作を制御するために使用される情報です。構文引数VAR: ターゲットプロパティの値を格納する変数名
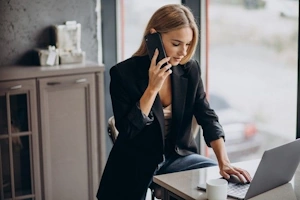
CMakeの"Commands"における"fltk_wrap_ui()"プログラミングを徹底解説!
この解説では、CMakeの"Commands"における"fltk_wrap_ui()"プログラミングについて、分かりやすく説明します。"fltk_wrap_ui()"は、CMakeでFLTK GUIアプリケーションをビルドするために使用されるマクロです。このマクロは、FLTK GUI定義ファイルをC++コードに変換し、プロジェクトに組み込みます。
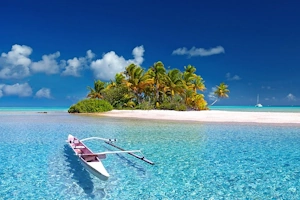
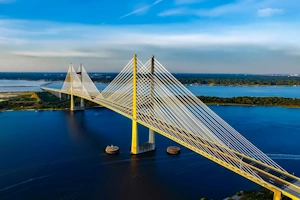
「ATTACHED_FILES」って何?CMakeの「Properties: Tests」でテスト結果を分析しよう!
ATTACHED_FILES は、CMake のテストプロパティの一つであり、テスト実行時にダッシュボードに添付するファイルのリストを設定します。テスト結果に加えて、これらのファイルはダッシュボードに送信され、テストの詳細な分析やデバッグに役立ちます。
CMake の "Properties of Global Scope" における "RULE_LAUNCH_LINK" の詳細解説
CMake の "Properties of Global Scope" における "RULE_LAUNCH_LINK" は、プロジェクト全体のリンクルールを制御するための特殊なプロパティです。これは、特定の条件下で特定のランチャーコマンドを実行するように設定できます。
CMakeLists.txtファイルにおけるCMAKE_LANG_FLAGSのサンプルコード
CMAKE_LANG_FLAGS は、CMake でビルドされる全ての言語に適用されるコンパイルオプションを設定するための変数です。この変数に設定されたオプションは、全てのソースファイルのコンパイル時にデフォルトとして適用されます。設定方法
CMake の "Variables" に関連する "CTEST_CUSTOM_PRE_MEMCHECK" のプログラミング解説
CTEST_CUSTOM_PRE_MEMCHECK の使用方法を理解するには、以下の手順に従ってください。変数を定義する<command> は、CTEST を実行する前に実行するカスタムコマンドを指定します。このコマンドは、シェルスクリプト、実行可能ファイル、またはその他のプログラムであることができます。
WinRTアプリケーション開発におけるVS_WINRT_REFERENCES
VS_WINRT_REFERENCESは、CMakeのターゲットプロパティの一つで、Visual Studioプロジェクトファイル( .vcxproj )にWinRTメタデータ参照を追加するために使用されます。これは、Windows Runtime (WinRT) アプリケーション開発において重要な役割を果たします。