Tensor.sort() の代替方法
PyTorch Tensor.sort() の解説
使用方法
Tensor.sort() の使い方は以下の通りです。
sorted_values, indices = torch.sort(input, dim, descending=False)
input
: 並べ替えたいテンソルdim
: 並べ替えを行う次元descending
: True の場合、降順に並べ替え。False の場合、昇順に並べ替え (デフォルト)sorted_values
: 並べ替え後の要素を含むテンソルindices
: 並べ替え後の要素のインデックスを含むテンソル
例
以下の例では、2次元テンソルの要素を1番目の次元で昇順に並べ替えています。
import torch
# テンソルの作成
input = torch.tensor([[1, 3, 2], [4, 1, 0]])
# 並べ替え
sorted_values, indices = torch.sort(input, dim=1)
# 結果の出力
print(sorted_values)
# tensor([[1, 2, 3],
# [0, 1, 4]])
print(indices)
# tensor([[0, 2, 1],
# [2, 0, 1]])
dim
パラメータは省略可能です。省略した場合、テンソルの最後の次元で並べ替えが行われます。descending
パラメータはデフォルトで False です。True に設定すると、降順に並べ替えられます。- Tensor.sort() は、テンソルの要素を in-place で並べ替えないことに注意してください。
補足
- Tensor.sort() は、テンソルの要素を並べ替えるだけでなく、その要素のインデックスも返す点が重要です。このインデックスは、さまざまな目的に使用できます。例えば、並べ替え後の要素に基づいて、テンソルの他の要素を操作することができます。
- Tensor.sort() は、非常に効率的な関数です。そのため、大規模なテンソルの要素を並べ替える場合にも使用できます。
PyTorch Tensor.sort() は、PyTorch テンソルの要素を効率的に並べ替えるための便利な関数です。この関数の使い方は簡単で、さまざまな目的に使用できます。
Tensor.sort() のサンプルコード
1次元テンソルの並べ替え
import torch
# テンソルの作成
input = torch.tensor([3, 1, 2, 0])
# 昇順に並べ替え
sorted_values, indices = torch.sort(input)
# 結果の出力
print(sorted_values)
# tensor([0, 1, 2, 3])
print(indices)
# tensor([3, 0, 2, 1])
2次元テンソルの並べ替え
import torch
# テンソルの作成
input = torch.tensor([[1, 3, 2], [4, 1, 0]])
# 1番目の次元で昇順に並べ替え
sorted_values, indices = torch.sort(input, dim=1)
# 結果の出力
print(sorted_values)
# tensor([[1, 2, 3],
# [0, 1, 4]])
print(indices)
# tensor([[0, 2, 1],
# [2, 0, 1]])
降順に並べ替え
import torch
# テンソルの作成
input = torch.tensor([3, 1, 2, 0])
# 降順に並べ替え
sorted_values, indices = torch.sort(input, descending=True)
# 結果の出力
print(sorted_values)
# tensor([3, 2, 1, 0])
print(indices)
# tensor([0, 2, 1, 3])
インデックスのみ取得
import torch
# テンソルの作成
input = torch.tensor([3, 1, 2, 0])
# 昇順に並べ替え
_, indices = torch.sort(input)
# 結果の出力
print(indices)
# tensor([3, 0, 2, 1])
最後の次元で並べ替え
import torch
# テンソルの作成
input = torch.tensor([[[1, 3, 2], [4, 1, 0]], [[5, 2, 4], [1, 0, 3]]])
# 最後の次元で昇順に並べ替え
sorted_values, indices = torch.sort(input, dim=-1)
# 結果の出力
print(sorted_values)
# tensor([[[1, 2, 3],
# [0, 1, 4]],
# [[1, 2, 4],
# [0, 3, 5]]])
print(indices)
# tensor([[[0, 2, 1],
# [2, 0, 1]],
# [[1, 2, 0],
# [2, 0, 1]]])
Tensor.sort() の代替方法
torch.argsort() は、テンソルの要素を並べ替えた後の要素のインデックスを返す関数です。Tensor.sort() と異なり、テンソルの要素自体は並べ替えません。
import torch
# テンソルの作成
input = torch.tensor([3, 1, 2, 0])
# 昇順に並べ替え
indices = torch.argsort(input)
# 結果の出力
print(indices)
# tensor([3, 0, 2, 1])
Numpy の sort()
NumPy を使用している場合は、NumPy の sort() 関数を使用して PyTorch テンソルの要素を並べ替えることができます。
import numpy as np
import torch
# テンソルの作成
input = torch.tensor([3, 1, 2, 0])
# NumPy の sort() を使用して昇順に並べ替え
sorted_values = np.sort(input.numpy())
# 結果の出力
print(sorted_values)
# [0 1 2 3]
自作の関数
特定の要件を満たす必要がある場合は、自作の関数を使用して PyTorch テンソルの要素を並べ替えることができます。
import torch
def my_sort(input):
# ここに並べ替えのアルゴリズムを実装
# ...
# テンソルの作成
input = torch.tensor([3, 1, 2, 0])
# 自作の関数を使用して昇順に並べ替え
sorted_values = my_sort(input)
# 結果の出力
print(sorted_values)
# [0, 1, 2, 3]
- Tensor.sort() は、最も一般的で使いやすい方法です。
- torch.argsort() は、テンソルの要素自体は並べ替えたくない場合に便利です。
- NumPy の sort() は、NumPy をすでに使用している場合に便利です。
- 自作の関数 は、特定の要件を満たす必要がある場合に便利です。
それぞれの方法の長所と短所を理解し、状況に応じて適切な方法を選択することが重要です。
パフォーマンス向上:PyTorch Dataset と DataLoader でデータローディングを最適化する
Datasetは、データセットを表す抽象クラスです。データセットは、画像、テキスト、音声など、機械学習モデルの学習に使用できるデータのコレクションです。Datasetクラスは、データセットを読み込み、処理するための基本的なインターフェースを提供します。
PyTorch Miscellaneous: torch.testing.assert_close() の詳細解説
torch. testing. assert_close() は、PyTorch テストモジュール内にある関数で、2つのテンソルの要素がほぼ等しいことを確認するために使用されます。これは、テストコードで計算結果の正確性を検証する際に役立ちます。
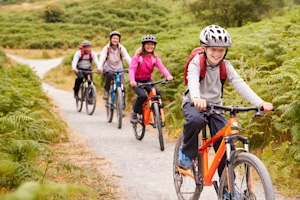
PyTorchのC++バックトレースを取得:torch.utils.get_cpp_backtraceの使い方
torch. utils. get_cpp_backtrace は、PyTorch の C++ バックトレースを取得するための関数です。これは、C++ コードで発生したエラーのデバッグに役立ちます。機能この関数は、現在のスレッドの C++ バックトレースをリストとして返します。各要素は、フレームの情報を含むディクショナリです。
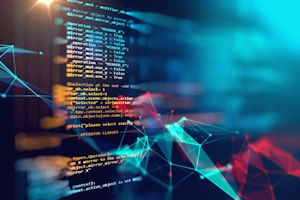
PyTorch C++ 拡張開発をレベルアップ! include パス取得の奥義をマスターしよう
torch. utils. cpp_extension. include_paths() は、PyTorch C++ 拡張をビルドするために必要なインクルードパスを取得するための関数です。 引数として cuda フラグを受け取り、True の場合、CUDA 固有のインクルードパスを追加します。 関数はインクルードパス文字列のリストを返します。
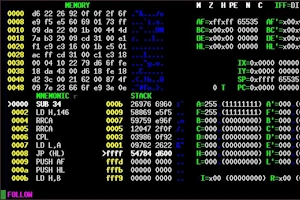
PyTorch Miscellaneous: torch.utils.cpp_extension.get_compiler_abi_compatibility_and_version() の概要
torch. utils. cpp_extension. get_compiler_abi_compatibility_and_version() は、C++ 拡張モジュールをビルドする際に、現在のコンパイラが PyTorch と互換性があるかどうかを確認するために使用されます。
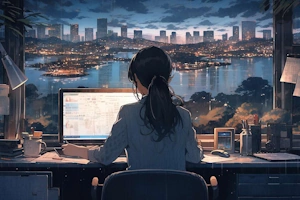
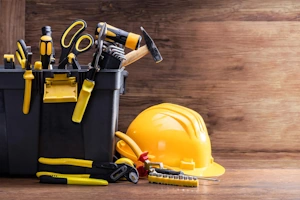
torch._foreach_frac_ 関数のサンプルコード
この関数は、以下の目的で使用できます:Tensorの各要素をランダムにサンプリングするサンプリングされた要素に対して、特定の処理を行うサンプリングされた要素の割合を制御するtorch. _foreach_frac_の引数:input: 処理対象となるTensor
PyTorch Probability Distributionsでサンプル形状を変更する
input_shape: 入力サンプルの形状validate_args: 入力形状と出力形状の一貫性を検証するかどうか
PyTorchでSciPyライクな信号処理:ハミング窓とその他の窓関数
PyTorchは、科学計算と機械学習のためのオープンソースライブラリです。SciPyは、Pythonによる科学計算のためのライブラリです。PyTorchには、SciPyライクな信号処理機能が提供されており、torch. signalモジュールで利用できます。
Python と Torch Script での型チェック: isinstance() と torch.jit.isinstance() の比較
torch. jit. isinstance() の使い方は、Python の isinstance() とほぼ同じです。チェックしたいオブジェクトと、比較したい型を指定します。torch. jit. isinstance() は、以下の型をチェックできます。
PyTorch Backends: torch.backends.cuda.cufft_plan_cache 解説
torch. backends. cuda. cufft_plan_cache は、PyTorch の CUDA バックエンドにおける cuFFT プランキャッシュを管理するためのモジュールです。cuFFT は、CUDA 上で高速なフーリエ変換を行うためのライブラリであり、torch