PyTorch Probability Distributionsでサンプル形状を変更する
PyTorch Probability DistributionsにおけるReshapeTransform
使用例
import torch
from torch.distributions import transforms
# 元の分布
dist = torch.distributions.Normal(torch.zeros(2), torch.ones(2))
# サンプル形状:(2,) -> (4,)
reshape_transform = transforms.ReshapeTransform((2, 2))
transformed_dist = dist.transform(reshape_transform)
# サンプル生成
samples = transformed_dist.sample()
print(samples.shape) # (2, 2)
# 異なる形状の分布の比較
dist1 = torch.distributions.Normal(torch.zeros(2), torch.ones(2))
dist2 = torch.distributions.Normal(torch.zeros(4), torch.ones(4))
# 形状を一致させる
reshape_transform = transforms.ReshapeTransform((2, 2))
dist1 = dist1.transform(reshape_transform)
# KLダイバージェンス計算
kl_divergence = torch.distributions.kl_divergence(dist1, dist2)
print(kl_divergence)
主な引数
input_shape
: 入力サンプルの形状validate_args
: 入力形状と出力形状の一貫性を検証するかどうか
ReshapeTransform を使ったサンプルコード
サンプル形状の変更
import torch
from torch.distributions import transforms
# 元の分布
dist = torch.distributions.Normal(torch.zeros(3), torch.ones(3))
# サンプル形状:(3,) -> (2, 3)
reshape_transform = transforms.ReshapeTransform((2, 3))
transformed_dist = dist.transform(reshape_transform)
# サンプル生成
samples = transformed_dist.sample()
print(samples.shape) # (2, 3)
バッチサイズ変更
import torch
from torch.distributions import transforms
# 元の分布
dist = torch.distributions.Normal(torch.zeros(10, 3), torch.ones(10, 3))
# バッチサイズ変更:(10, 3) -> (3, 10)
reshape_transform = transforms.ReshapeTransform((3, 10))
transformed_dist = dist.transform(reshape_transform)
# サンプル生成
samples = transformed_dist.sample()
print(samples.shape) # (3, 10)
異なる形状を持つ分布の比較
import torch
from torch.distributions import transforms
# 元の分布
dist1 = torch.distributions.Normal(torch.zeros(2, 3), torch.ones(2, 3))
dist2 = torch.distributions.Normal(torch.zeros(3, 2), torch.ones(3, 2))
# 形状を一致させる
reshape_transform = transforms.ReshapeTransform((2, 3))
dist2 = dist2.transform(reshape_transform)
# KLダイバージェンス計算
kl_divergence = torch.distributions.kl_divergence(dist1, dist2)
print(kl_divergence)
条件付き分布への適用
import torch
from torch.distributions import transforms, Conditional
# 元の分布
base_dist = torch.distributions.Normal(torch.zeros(3), torch.ones(3))
cond_dist = torch.distributions.Categorical(torch.ones(3))
# 条件付き分布
conditional_dist = Conditional(base_dist, cond_dist)
# サンプル形状:(3,) -> (2, 3)
reshape_transform = transforms.ReshapeTransform((2, 3))
transformed_dist = conditional_dist.transform(reshape_transform)
# サンプル生成
samples = transformed_dist.sample(condition=torch.tensor([0, 1]))
print(samples.shape) # (2, 3)
ReshapeTransform の代替方法
torch.view
関数は、テンソルの形状を変更するために使用できます。これは、ReshapeTransform
よりも簡潔な方法ですが、バッチサイズや条件付き分布などの複雑な形状変更には対応できません。
import torch
# 元の分布
dist = torch.distributions.Normal(torch.zeros(3), torch.ones(3))
# サンプル形状:(3,) -> (2, 3)
samples = dist.sample().view(2, 3)
# サンプル生成
print(samples.shape) # (2, 3)
自作の変換クラス
より複雑な形状変更を行う場合は、自作の変換クラスを作成することができます。この方法は、柔軟性がありますが、コード量が増加します。
import torch
from torch.distributions.transforms import Transform
class MyTransform(Transform):
def __init__(self, input_shape, output_shape):
super().__init__()
self.input_shape = input_shape
self.output_shape = output_shape
def __call__(self, samples):
return samples.view(self.output_shape)
def inverse(self, samples):
return samples.view(self.input_shape)
# 元の分布
dist = torch.distributions.Normal(torch.zeros(3), torch.ones(3))
# サンプル形状:(3,) -> (2, 3)
my_transform = MyTransform((3,), (2, 3))
transformed_dist = dist.transform(my_transform)
# サンプル生成
samples = transformed_dist.sample()
print(samples.shape) # (2, 3)
これらの方法はそれぞれ、異なる利点と欠点があります。使用する方法は、特定のアプリケーションによって異なります。
パフォーマンス向上:PyTorch Dataset と DataLoader でデータローディングを最適化する
Datasetは、データセットを表す抽象クラスです。データセットは、画像、テキスト、音声など、機械学習モデルの学習に使用できるデータのコレクションです。Datasetクラスは、データセットを読み込み、処理するための基本的なインターフェースを提供します。
画像処理に役立つ PyTorch の Discrete Fourier Transforms と torch.fft.ihfft2()
PyTorch は Python で機械学習を行うためのライブラリであり、画像処理や音声処理など様々な分野で活用されています。Discrete Fourier Transforms (DFT) は、信号処理や画像処理において重要な役割を果たす数学的な変換です。PyTorch には torch
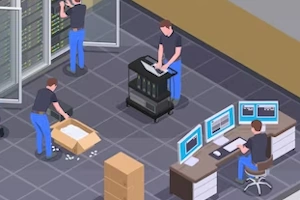
PyTorchで多 boyut DFT:torch.fft.hfftn()の使い方とサンプルコード
torch. fft. hfftn() は、入力テンソルの多 boyut DFT を計算します。この関数は以下の引数を受け取ります。input: 入力テンソル。s: DFT を実行する軸のリスト。デフォルトでは、入力テンソルのすべての軸に対して DFT が実行されます。

PyTorch初心者でも安心!torch.fft.fftnを使ったサンプルコード集
PyTorchは、Pythonにおける深層学習ライブラリであり、科学計算にも利用できます。torch. fftモジュールは、離散フーリエ変換(DFT)を含むフーリエ変換関連の機能を提供します。torch. fft. fftnは、多次元DFTを実行するための関数です。これは、画像処理、音声処理、信号処理など、様々な分野で使用されます。

torch.fft.ifftを使いこなせ!画像処理・音声処理・機械学習の強力なツール
PyTorchは、Pythonにおけるディープラーニングフレームワークの一つです。torch. fftモジュールには、離散フーリエ変換(DFT)と逆離散フーリエ変換(IDFT)を行うための関数群が用意されています。torch. fft. ifftは、DFTの結果を入力として受け取り、IDFTを実行する関数です。
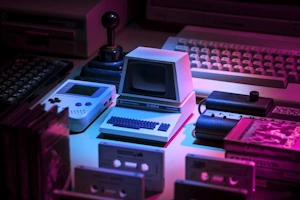
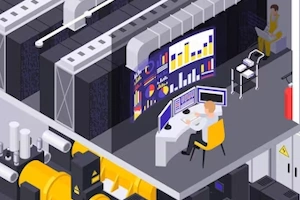
画像処理に役立つ PyTorch の Discrete Fourier Transforms と torch.fft.ihfft2()
PyTorch は Python で機械学習を行うためのライブラリであり、画像処理や音声処理など様々な分野で活用されています。Discrete Fourier Transforms (DFT) は、信号処理や画像処理において重要な役割を果たす数学的な変換です。PyTorch には torch
PyTorch分散学習:Torchelasticと torch.distributed.is_torchelastic_launched()
torch. distributed. is_torchelastic_launched()は、PyTorchの分散通信モジュールtorch. distributedにおける、Torchelasticを使用してプロセスが起動されたかどうかを判定する関数です。
PyTorch Tensor の torch.Tensor.istft() 関数で音声復元:詳細解説とサンプルコード
torch. Tensor. istft() は、短時間フーリエ変換 (STFT) の逆変換を実行する関数です。STFT は、音声を時間周波数領域に変換する処理であり、音声分析や音声合成などに利用されます。istft() 関数は、STFT で得られたスペクトログラムから元の音声を復元するために使用されます。
PyTorchの逆フーリエ変換:torch.fft.ihfftnとその他の方法
torch. fft. ihfftnは、PyTorchにおける多次元逆離散フーリエ変換(IDFT)の実装です。これは、フーリエ変換によって周波数領域に変換されたデータを元の空間に戻すための関数です。使い方引数input: 入力テンソル。複素数型である必要があります。
PyTorchにおける torch.Tensor.to_mkldnn の解説
torch. Tensor. to_mkldnnは、PyTorchにおけるテンソルをIntel® Math Kernel Library for Deep Neural Networks (Intel® MKDNN)形式に変換するためのメソッドです。MKDNNは、畳み込みニューラルネットワーク (CNN) などの深層学習モデルにおける計算効率を向上させるためのライブラリです。