Pythonエンジニア必見!Torch Scriptのtorch.jit.ScriptModule.modules()でコードをもっと効率的に
PyTorchのTorch Scriptにおけるtorch.jit.ScriptModule.modules()解説
Torch Scriptは、PyTorchモデルを効率的なC++コードに変換し、推論速度を向上させるためのツールです。torch.jit.ScriptModule
は、Torch Scriptでコンパイルされたモデルを表すクラスです。
torch.jit.ScriptModule.modules()
は、ScriptModule
内のすべてのサブモジュールをジェネレータとして返す関数です。サブモジュールとは、nn.Module
を継承したクラスのインスタンスです。
コード例
import torch
class MyModule(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear1 = torch.nn.Linear(10, 10)
self.linear2 = torch.nn.Linear(10, 10)
model = MyModule()
script_module = torch.jit.trace(model, example_inputs=torch.randn(1, 10))
for module in script_module.modules():
print(module)
上記のコードは、MyModule
クラスのインスタンスを作成し、torch.jit.trace
を使ってScriptModule
に変換します。その後、torch.jit.ScriptModule.modules()
を使って、ScriptModule
内のすべてのサブモジュールをループ処理します。
出力例
Linear(in_features=10, out_features=10, bias=True)
Linear(in_features=10, out_features=10, bias=True)
上記のコードは、MyModule
クラスにはlinear1
とlinear2
という2つのサブモジュールがあることを示しています。
torch.jit.ScriptModule.modules()
は以下の利点があります。
ScriptModule
内のすべてのサブモジュールにアクセスできる。- サブモジュールに対して処理を行うことができる。
- サブモジュールの名前や属性を取得できる。
torch.jit.ScriptModule.modules()
は、Torch Scriptでコンパイルされたモデル内のすべてのサブモジュールにアクセスするための便利な関数です。サブモジュールに対して処理を行う場合や、サブモジュールの名前や属性を取得する場合などに役立ちます。
Torch Scriptのtorch.jit.ScriptModule.modules()のサンプルコード
import torch
class MyModule(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear1 = torch.nn.Linear(10, 10)
self.linear2 = torch.nn.Linear(10, 10)
model = MyModule()
script_module = torch.jit.trace(model, example_inputs=torch.randn(1, 10))
# サブモジュールへのアクセス
linear1 = script_module.modules()[0]
linear2 = script_module.modules()[1]
# サブモジュールの属性へのアクセス
print(linear1.weight)
print(linear2.bias)
サブモジュールに対する処理
import torch
class MyModule(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear1 = torch.nn.Linear(10, 10)
self.linear2 = torch.nn.Linear(10, 10)
model = MyModule()
script_module = torch.jit.trace(model, example_inputs=torch.randn(1, 10))
# サブモジュールに対する処理
def freeze_module(module):
for param in module.parameters():
param.requires_grad = False
for module in script_module.modules():
freeze_module(module)
# 推論
output = script_module(torch.randn(1, 10))
サブモジュールの名前と属性の取得
import torch
class MyModule(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear1 = torch.nn.Linear(10, 10)
self.linear2 = torch.nn.Linear(10, 10)
model = MyModule()
script_module = torch.jit.trace(model, example_inputs=torch.randn(1, 10))
# サブモジュールの名前と属性の取得
for module in script_module.modules():
print(module.name)
print(module.parameters())
サブモジュールを別のScriptModuleに追加
import torch
class MyModule1(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear1 = torch.nn.Linear(10, 10)
class MyModule2(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear2 = torch.nn.Linear(10, 10)
model1 = MyModule1()
model2 = MyModule2()
script_module1 = torch.jit.trace(model1, example_inputs=torch.randn(1, 10))
script_module2 = torch.jit.trace(model2, example_inputs=torch.randn(1, 10))
# サブモジュールを別のScriptModuleに追加
script_module1.add_module("linear2", script_module2.modules()[0])
# 推論
output = script_module1(torch.randn(1, 10))
ネストされたサブモジュールへのアクセス
import torch
class MyModule(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear1 = torch.nn.Linear(10, 10)
self.conv1 = torch.nn.Conv2d(1, 1, 3)
class MyModule2(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear2 = torch.nn.Linear(10, 10)
model = MyModule()
script_module = torch.jit.trace(model, example_inputs=torch.randn(1, 10))
# ネストされたサブモジュールへのアクセス
linear1 = script_module.modules()[0]
conv1 = script_module.modules()[1]
linear2 = script_module.modules()[1].modules()[0]
# サブモジュールの属性へのアクセス
print(linear1.weight)
print(conv1.kernel
サブモジュールへのアクセス方法
サブモジュールを直接参照する
import torch
class MyModule(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear1 = torch.nn.Linear(10, 10)
self.linear2 = torch.nn.Linear(10, 10)
model = MyModule()
script_module = torch.jit.trace(model, example_inputs=torch.randn(1, 10))
# サブモジュールへの直接参照
linear1 = script_module.linear1
linear2 = script_module.linear2
# サブモジュールの属性へのアクセス
print(linear1.weight)
print(linear2.bias)
torch.jit.get_submodulesを使用する
import torch
class MyModule(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear1 = torch.nn.Linear(10, 10)
self.linear2 = torch.nn.Linear(10, 10)
model = MyModule()
script_module = torch.jit.trace(model, example_inputs=torch.randn(1, 10))
# torch.jit.get_submodulesを使用する
linear1, linear2 = torch.jit.get_submodules(script_module)
# サブモジュールの属性へのアクセス
print(linear1.weight)
print(linear2.bias)
この方法は、サブモジュールの名前が分からなくても使用できます。
torch.jit.ScriptModule.named_modulesを使用する
import torch
class MyModule(torch.nn.Module):
def __init__(self):
super().__init__()
self.linear1 = torch.nn.Linear(10, 10)
self.linear2 = torch.nn.Linear(10, 10)
model = MyModule()
script_module = torch.jit.trace(model, example_inputs=torch.randn(1, 10))
# torch.jit.ScriptModule.named_modulesを使用する
for name, module in script_module.named_modules():
print(name, module)
この方法は、サブモジュールの名前とモジュールオブジェクトのペアをイテレートするのに役立ちます。
その他の方法
- サブモジュールを名前で検索する:
script_module.find_module("linear1")
- サブモジュールのタイプで検索する:
script_module.get_modules(torch.nn.Linear)
サブモジュールへのアクセスにはいくつかの方法があります。どの方法を使用するかは、特定のニーズによって異なります。
パフォーマンス向上:PyTorch Dataset と DataLoader でデータローディングを最適化する
Datasetは、データセットを表す抽象クラスです。データセットは、画像、テキスト、音声など、機械学習モデルの学習に使用できるデータのコレクションです。Datasetクラスは、データセットを読み込み、処理するための基本的なインターフェースを提供します。
線形代数ライブラリtorch.linalgの秘密兵器:torch.linalg.luの全貌
この解説では、torch. linalg. lu の詳細な使い方と、その応用例について説明します。torch. linalg. lu は、入力行列 A を下三角行列 L と上三角行列 U に分解します。この関数は以下の式で表されます。ここで、L は対角成分が全て 1 の下三角行列、U は上三角行列です。
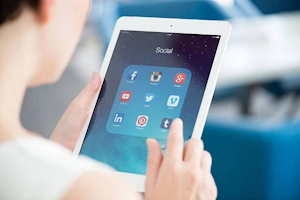
【初心者向け】PyTorch の Linear Algebra モジュール: torch.linalg.cross() 関数を使ってベクトルの外積を計算しよう
torch. linalg. cross() 関数は、PyTorch の Linear Algebra モジュールで提供される機能の一つであり、3 次元ベクトルの外積を計算します。ベクトルの外積は、2 つのベクトルの直交する方向ベクトルを生成するベクトル演算です。
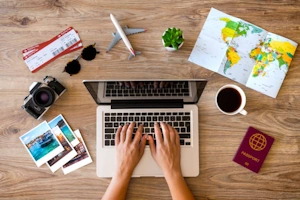
PyTorch Linear Algebra: torch.linalg.vander() の徹底解説
torch. linalg. vander は、Vandermonde行列を生成する関数です。Vandermonde行列は、ベクトルの各要素のべき乗を列ベクトルとして並べた行列です。この関数は、PyTorchの線形代数ライブラリ torch
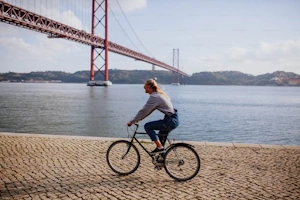
PyTorchで逆行列と行列式を効率的に計算: inv_ex()の使い方
torch. linalg. inv_ex()は、入力された行列の逆行列と行列式を同時に計算します。これは、逆行列と行列式を個別に計算するよりも効率的です。input (Tensor): 逆行列と行列式を計算したい行列**compute_svd (bool

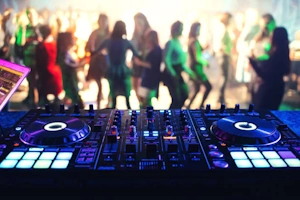
PyTorch Miscellaneous: torch.hub.load()
引数organization_name: モデルを公開している組織の名前 (例: "facebookresearch")model_name: モデルの名前 (例: "resnet18")version: モデルのバージョン (例: "1.0")
PyTorch Probability Distributions:torch.distributions.half_normal.HalfNormal.expand()の徹底解説
torch. distributions. half_normal. HalfNormal. expand()は、PyTorchのProbability Distributionsモジュールにおける、半正規分布の確率密度関数を拡張するための関数です。この関数は、入力されたテンソルの形状に基づいて、新しい形状を持つ半正規分布の確率密度関数を生成します。
コイン投げシミュレーションからベイズ推論まで: PyTorch Tensor.bernoulli_() メソッドの多様な活用例
torch. Tensor. bernoulli_() メソッドは、入力テンソルの各要素をベルヌーイ分布に基づいてランダムな0または1に置き換えます。これは、コイン投げのような2つの状態を持つ事象をシミュレートする際に役立ちます。詳細入力:
PyTorch 量子化: torch.ao.quantization.backend_config.DTypeConfig の詳細解説
DTypeConfig は以下の属性を持ちます。pattern: 量子化対象となるオペレーターパターンの名前を表す文字列。input_dtype: 入力アクティベーションのデータ型を torch. dtype 型で指定。weight_dtype: 重みのデータ型を torch
PyTorch初心者向け:torch.var_mean関数でテンソルの分散と平均値をマスターしよう
この関数を使うと、以下のようなメリットがあります。コードの簡潔化: 分散と平均値を個別に計算する必要がなくなり、コードがスッキリします。効率的な処理: 分散と平均値を同時に計算するため、処理速度が向上します。柔軟な計算: 軸指定やバイアス補正など、さまざまなオプションを指定して計算できます。