Qt Widgets サンプルコード集:ボタン、入力、レイアウト、ダイアログ、その他
QButtonGroup::checkedButton() の詳細解説
QButtonGroup::checkedButton()
メソッドは、Qt Widgets モジュールにおける QButtonGroup クラスの重要な機能の一つです。このメソッドは、ボタングループ内に存在するチェックされているボタンを取得することを可能にします。ボタングループは、互いに排他的な動作をする複数のボタンを管理する機能を提供します。
使用方法
checkedButton()
メソッドは、以下の構文で呼び出すことができます。
QAbstractButton* checkedButton() const;
このメソッドは、チェックされているボタンを QAbstractButton
型のポインタとして返します。チェックされていないボタンが存在する場合、またはボタングループ内にボタンが存在しない場合は、nullptr
が返されます。
例
以下のコードは、QButtonGroup
に 3 つのボタンを追加し、checkedButton()
メソッドを使用して、チェックされているボタンを取得する例です。
QButtonGroup buttonGroup;
// ボタンを作成してボタングループに追加する
QRadioButton* radioButton1 = new QRadioButton("Option 1");
QRadioButton* radioButton2 = new QRadioButton("Option 2");
QRadioButton* radioButton3 = new QRadioButton("Option 3");
buttonGroup.addButton(radioButton1);
buttonGroup.addButton(radioButton2);
buttonGroup.addButton(radioButton3);
// ボタンのいずれかをチェックする
radioButton2->setChecked(true);
// チェックされているボタンを取得する
QAbstractButton* checkedButton = buttonGroup.checkedButton();
if (checkedButton) {
qDebug() << "Checked button: " << checkedButton->text();
} else {
qDebug() << "No button is checked";
}
このコードを実行すると、以下の出力がコンソールに表示されます。
Checked button: Option 2
注意点
checkedButton()
メソッドは、チェックされているボタンのみを取得します。チェックされていないボタンを取得するには、buttons()
メソッドと組み合わせる必要があります。checkedButton()
メソッドは、ボタングループ内に存在するボタンのみを取得します。ボタングループに属していないボタンをチェックしても、このメソッドで取得することはできません。
QButtonGroup::checkedButton()
メソッドは、ボタングループ内のチェックされているボタンを取得する際に役立ちます。このメソッドを活用することで、ボタングループの動作をより柔軟に制御することができます。
- この説明が分かりやすく、役に立てば幸いです。
- 上記以外にも、様々な種類のサンプルコードが公開されています。
- 探したいサンプルコードが見つからない場合は、Google 検索で「Qt Widgets」とキーワードを入力して検索してみてください。
-
Using the buttons() method:
The
buttons()
method returns a list of all the buttons in the QButtonGroup. You can then iterate over the list to find the checked button.QList<QAbstractButton*> buttons = buttonGroup.buttons(); for (QAbstractButton* button : buttons) { if (button->isChecked()) { checkedButton = button; break; } }
-
Using the id() method:
Each button in a QButtonGroup has a unique ID. You can use the
id()
method to get the ID of the checked button, and then use thebutton()
method to get the button itself.int checkedId = buttonGroup.checkedId(); if (checkedId != -1) { checkedButton = buttonGroup.button(checkedId); }
-
Connecting to the buttonClicked() signal:
The
buttonClicked()
signal is emitted whenever a button in the QButtonGroup is clicked. You can connect to this signal and use thesender()
method to get the checked button.connect(buttonGroup, &QButtonGroup::buttonClicked, this, &MyClass::onButtonClicked); void MyClass::onButtonClicked(int id) { checkedButton = buttonGroup.button(id); }
Which method you use depends on your specific needs. If you only need to get the checked button once, then the checkedButton()
method is the simplest way to do it. If you need to get the checked button more than once, or if you need to know which button was clicked, then the other methods are better choices.
Here is a table that summarizes the pros and cons of each method:
Method | Pros | Cons |
---|---|---|
checkedButton() | Simple to use | Can only get the checked button once |
buttons() | Can get all of the buttons in the group | More complex to use |
id() | Can get the ID of the checked button | Requires you to know the IDs of the buttons |
buttonClicked() | Can get the checked button whenever it is clicked | Requires you to connect to a signal |
I hope this helps! Let me know if you have any other questions.
QWindow::setMouseGrabEnabled() の代替方法:QRubberBand と QGraphicsItem::setFlags() を活用
QWindow::setMouseGrabEnabled() は、マウスイベントを特定のウィンドウに独占的に送信させるための関数です。有効にすると、そのウィンドウがフォーカスを持っていなくても、すべてのマウスイベントを受け取ります。他のウィンドウは、マウスイベントを受け取らなくなります。
Qt GUIでQMatrix4x4::setColumn()の代替方法
QMatrix4x4::setColumn()は、Qt GUIで使用される4x4行列クラスQMatrix4x4のメンバー関数です。この関数は、行列の指定された列の要素をすべて新しい値で設定します。3Dグラフィックスやアニメーションなど、さまざまな場面で活用できます。
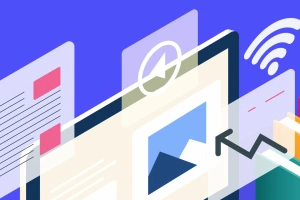
QTextListFormat::style() 関数の使い方
QTextListFormat::style() 関数は、テキストリストのスタイルを取得します。スタイルには、番号付きリスト、箇条書き、段落などがあります。関数宣言引数なし戻り値QTextListFormat::Style 型の値。以下のいずれかになります。
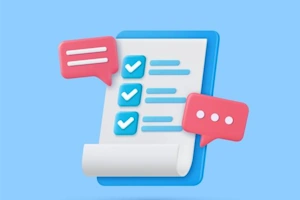
Qt GUIにおけるQStandardItem::setText()の徹底解説
その中でも、setText()メソッドは、アイテムのテキスト内容を設定するために使用されます。このメソッドは、さまざまな引数を受け取り、テキストの書式や配置などを詳細に制御することができます。まず、setText()メソッドの基本的な使い方を説明します。このメソッドには、以下の引数が必要です。
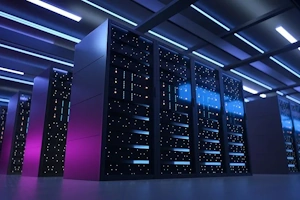
Qt GUIで画像入出力ハンドラーを自在に操る:QImageIOHandler::option() の詳細解説
QImageIOHandler::option() は、Qt GUI における画像入出力ハンドラーがサポートするオプションを取得するための関数です。画像フォーマット固有の情報や、読み書き処理に関する設定などを取得するために使用されます。構文
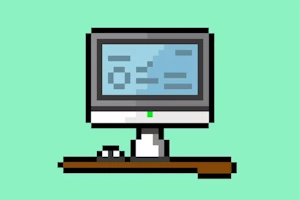

Qt GUIでQOpenGLExtraFunctionsクラスを使ってテクスチャマッピングを行う
QOpenGLExtraFunctionsクラスの利点:OpenGL 3.x/4.xの主要な機能を網羅Qtのオブジェクト指向プログラミングスタイルと自然に統合複雑なOpenGL操作を簡潔なコードで記述可能コードの可読性と保守性を向上開発時間を短縮
Qt Widgetsにおけるフレームの幅を取得する:QFrame::frameWidth()徹底解説
機能: フレームの幅を取得する戻り値: フレームの幅を表す整数値(ピクセル単位)使用例: フレームの幅に基づいてウィジェットのレイアウトを調整する フレームの幅を動的に変更して、視覚的な効果を与えるフレームの幅に基づいてウィジェットのレイアウトを調整する
PythonでWebスクレイピング:BeautifulSoupを使ってh1要素のテキストを抽出
QTreeWidgetItem::clone()は、Qt Widgetsライブラリで提供される、QTreeWidgetItemオブジェクトの完全なコピーを作成する関数です。この関数は、ツリー構造を複製したり、既存のアイテムを別の場所に挿入したりする場合に役立ちます。
Qt GUIで入力エラーを防ぐ:QValidatorの使い方
概要QValidator::~QValidator() は、Qt GUIにおける入力検証クラス QValidator のデストラクタ関数です。この関数は、QValidator オブジェクトが破棄されるときに自動的に呼び出され、オブジェクトが占有していたメモリなどのリソースを解放します。
Qt GUI でグラデーションブラシを作成する方法
QBrush::gradient() 関数は、Qt GUI でグラデーションブラシを作成するために使用します。グラデーションブラシは、複数の色を滑らかに変化させて塗ることができるブラシです。機能QBrush::gradient() 関数は、以下の種類のグラデーションブラシを作成できます。