QStyleOptionHeader::SortIndicatorを使用したソートインジケータのカスタマイズ
Qt WidgetsにおけるQStyleOptionHeader::SortIndicator解説
QStyleOptionHeader::SortIndicator
は、Qt Widgetsにおけるヘッダーウィジェットのソートインジケータのスタイルオプションを制御するための列挙型です。このオプションは、ヘッダー内のソート順序と方向を表す視覚的な指標をカスタマイズするために使用できます。
詳細
QStyleOptionHeader::SortIndicator
は以下の値を持ちます:
- NoSortIndicator: ソートインジケータを表示しません。
- SortUp: 昇順ソートインジケータを表示します。
これらの値は、QHeaderView::setSortIndicator
関数を使用してヘッダーウィジェットに設定できます。
例
// ヘッダーウィジェットのソートインジケータを昇順に設定
headerView->setSortIndicator(QHeaderView::SortUp);
// ヘッダーウィジェットのソートインジケータを降順に設定
headerView->setSortIndicator(QHeaderView::SortDown);
スタイルオプションのカスタマイズ
QStyleOptionHeader
クラスには、ソートインジケータの色、サイズ、位置などをカスタマイズするための様々なプロパティが用意されています。これらのプロパティは、QHeaderView::styleOption
関数を使用して取得し、QStyle::drawPrimitive
関数を使用して描画することができます。
スタイルオプションの取得
QStyleOptionHeader option = headerView->styleOption();
スタイルオプションの描画
QStyle *style = headerView->style();
style->drawPrimitive(QStyle::PE_IndicatorHeaderArrow, &option, painter);
Qt WidgetsにおけるQStyleOptionHeader::SortIndicatorを使用したサンプルコード
ソートインジケータの表示
#include <QtWidgets>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// ヘッダーウィジェットの作成
QHeaderView headerView;
// ソートインジケータの表示
headerView.setSortIndicator(QHeaderView::SortUp);
// ヘッダーウィジェットの表示
headerView.show();
return app.exec();
}
ソートインジケータの色の変更
#include <QtWidgets>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// ヘッダーウィジェットの作成
QHeaderView headerView;
// ソートインジケータの色の設定
headerView.setStyleSheet("QHeaderView::indicator:up { color: red; }");
// ソートインジケータの表示
headerView.setSortIndicator(QHeaderView::SortUp);
// ヘッダーウィジェットの表示
headerView.show();
return app.exec();
}
ソートインジケータのカスタマイズ
#include <QtWidgets>
class MyStyle : public QStyle {
public:
void drawPrimitive(PrimitiveElement element, const QStyleOption *option, QPainter *painter) override {
if (element == PE_IndicatorHeaderArrow) {
// ソートインジケータの描画処理
QStyleOptionHeader *headerOption = const_cast<QStyleOptionHeader *>(option);
// ソート方向に応じた三角形の描画
if (headerOption->sortIndicator == QStyleOptionHeader::SortUp) {
painter->drawPolygon(QPolygonF({
QPointF(0, 0),
QPointF(5, 5),
QPointF(10, 0)
}));
} else if (headerOption->sortIndicator == QStyleOptionHeader::SortDown) {
painter->drawPolygon(QPolygonF({
QPointF(0, 5),
QPointF(5, 0),
QPointF(10, 5)
}));
}
} else {
// その他のスタイル要素の描画処理
QStyle::drawPrimitive(element, option, painter);
}
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// ヘッダーウィジェットの作成
QHeaderView headerView;
// カスタムスタイルの設定
headerView.setStyle(new MyStyle);
// ソートインジケータの表示
headerView.setSortIndicator(QHeaderView::SortUp);
// ヘッダーウィジェットの表示
headerView.show();
return app.exec();
}
Qt WidgetsにおけるQStyleOptionHeader::SortIndicator以外のソートインジケータ表示方法
カスタムウィジェット
#include <QtWidgets>
class MyWidget : public QWidget {
public:
MyWidget() {
// ソート方向の初期化
m_sortIndicator = QStyleOptionHeader::SortUp;
}
protected:
void paintEvent(QPaintEvent *event) override {
QPainter painter(this);
// ソート方向に応じた三角形の描画
if (m_sortIndicator == QStyleOptionHeader::SortUp) {
painter.drawPolygon(QPolygonF({
QPointF(0, 0),
QPointF(5, 5),
QPointF(10, 0)
}));
} else if (m_sortIndicator == QStyleOptionHeader::SortDown) {
painter.drawPolygon(QPolygonF({
QPointF(0, 5),
QPointF(5, 0),
QPointF(10, 5)
}));
}
}
void setSortIndicator(QStyleOptionHeader::SortIndicator indicator) {
m_sortIndicator = indicator;
update();
}
private:
QStyleOptionHeader::SortIndicator m_sortIndicator;
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// カスタムウィジェットの作成
MyWidget widget;
// ソートインジケータの表示
widget.setSortIndicator(QStyleOptionHeader::SortUp);
// ウィジェットの表示
widget.show();
return app.exec();
}
QGraphicsView
を使用する場合は、QGraphicsItem
を派生したカスタムアイテムを作成し、paint
イベントをオーバーライドしてソートインジケータを描画することができます。
#include <QtWidgets>
#include <QtGraphics>
class MyItem : public QGraphicsItem {
public:
MyItem() {
// ソート方向の初期化
m_sortIndicator = QStyleOptionHeader::SortUp;
}
protected:
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) override {
// ソート方向に応じた三角形の描画
if (m_sortIndicator == QStyleOptionHeader::SortUp) {
painter->drawPolygon(QPolygonF({
QPointF(0, 0),
QPointF(5, 5),
QPointF(10, 0)
}));
} else if (m_sortIndicator == QStyleOptionHeader::SortDown) {
painter->drawPolygon(QPolygonF({
QPointF(0, 5),
QPointF(5, 0),
QPointF(10, 5)
}));
}
}
void setSortIndicator(QStyleOptionHeader::SortIndicator indicator) {
m_sortIndicator = indicator;
update();
}
private:
QStyleOptionHeader::SortIndicator m_sortIndicator;
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// QGraphicsViewの作成
QGraphicsView view;
// カスタムアイテムの作成
MyItem item;
// アイテムの追加
view.scene()->addItem(&item);
// ソートインジケータの表示
item.setSortIndicator(QStyleOptionHeader::SortUp);
// ビューの表示
view.show();
return app.exec();
}
これらの方法は、QStyleOptionHeader::SortIndicator
を使用するよりも柔軟性がありますが、より複雑な実装が必要になります。
- Qt Graphics Frameworkにおけるカスタムアイテムの詳細については、Qt
Qt GUI で QTextList::itemNumber() 関数を使用して特定の項目にアクセスする方法
概要QTextList::itemNumber() 関数は、QTextList オブジェクト内の特定の QTextBlock がリスト内のどの項目に対応しているのかを調べ、そのインデックスを返します。もし、その QTextBlock がリスト内に存在しない場合は、-1 を返します。
Qt GUI でデータのバインディングと QVector2D::operator QVariant()
QVector2D: 2D ベクトルを表すクラスoperator QVariant(): QVector2D オブジェクトを QVariant 型に変換する関数QVariant: Qt のさまざまなデータ型を汎用的に表現する型QVector2D::operator QVariant() は、さまざまな用途で使用されます。
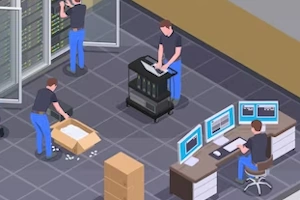
QVector3D::operator QVariant() 関数のサンプルコード
QVector3D::operator QVariant() 関数は、3Dベクトルを表す QVector3D 型を、Qt の汎用データ型である QVariant 型に変換します。これは、3Dベクトルデータを他の Qt オブジェクトとやり取りしたり、シリアル化したり、保存したりする際に役立ちます。
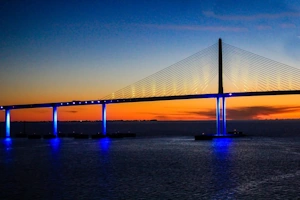
QSyntaxHighlighter::document() を使用してカスタムハイライトルールを実装する方法
QSyntaxHighlighter::document() は、Qt GUI アプリケーションにおけるシンタックスハイライト機能を提供するクラス QSyntaxHighlighter のメンバー関数です。この関数は、ハイライト対象となるテキストドキュメントへのポインタを取得するために使用されます。
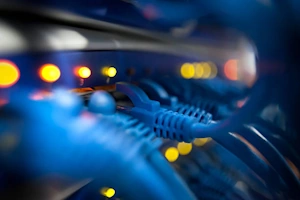
Qt GUI で Vulkan レイヤーの名前を取得するサンプルコード
QVulkanLayer::name の詳細型: QStringデフォルト値: 空の文字列スレッド安全性: スレッドセーフQVulkanLayer::name プロパティは、QVulkanLayer オブジェクトから名前を取得するために使用されます。以下のコード例は、QVulkanLayer オブジェクトの名前を取得する方法を示しています。

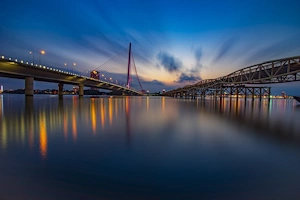
QPainter::setBrushOrigin() メソッドでブラシ原点を設定
QPainter::setBrushOrigin() メソッドは、Qt GUI における描画操作において、ブラシの原点を設定するために使用されます。ブラシの原点は、ブラシのパターンが描画される開始点となる座標を定義します。このメソッドを使用することで、ブラシのパターンの配置をコントロールし、より精度の高い描画を実現することができます。
Qt WidgetsにおけるQProxyStyle::styleHint()徹底解説
QProxyStyle::styleHint()は、Qt Widgetsにおける重要な関数の一つです。この関数は、ウィジェットのスタイルヒントを取得するために使用されます。スタイルヒントは、ウィジェットの外観や動作をどのように変更するかを決定するために使用されます。
Qt Widgetsでカラーマップを自在に操るためのテクニック集:QColormapクラス活用ガイド
本解説では、QColormapクラスの機能と使用方法を、初心者にも分かりやすく丁寧に解説します。QColormapは、Qt Widgetsフレームワークにおけるカラーマップを表現するクラスです。カラーマップは、複数のQColorオブジェクトを連続的に並べたものであり、データの視覚化や画像処理などに使用されます。
Qt Widgets: QListWidget::indexFromItem() 関数の詳細解説
QListWidget::indexFromItem() は、Qt Widgets モジュールの QListWidget クラスで使用される関数です。この関数は、指定されたアイテムに対応するインデックスを取得するために使用されます。機能QListWidget::indexFromItem() は、以下の情報を提供します。
Qt WidgetsにおけるQGraphicsScene::drawForeground()の応用例
QGraphicsScene::drawForeground()は、Qt Widgetsフレームワークにおける重要な関数の一つです。これは、グラフィックスシーンの前面に描画を行うための仮想関数であり、さまざまな用途に使用することができます。