PyTorchのtorch.clone:Tensorをコピーする便利な方法
PyTorchのtorch.clone:Tensorをコピーする便利な方法
torch.cloneの利点
- 浅いコピーと深いコピーを選択できる:
torch.clone(memory_format=torch.preserve_format)
: 参照渡しによる浅いコピーtorch.clone(memory_format=torch.contiguous_format)
: データのコピーによる深いコピー
- 演算グラフを共有するため、効率的
- 多くのPyTorchモジュールでサポートされている
torch.cloneの例
import torch
# テンソルを作成
tensor = torch.randn(3, 4)
# 浅いコピーを作成
clone_shallow = tensor.clone()
# 深いコピーを作成
clone_deep = tensor.clone(memory_format=torch.contiguous_format)
# テンソルの値を変更
tensor[0, 0] = 100
# コピーを確認
print("元のテンソル:", tensor)
print("浅いコピー:", clone_shallow)
print("深いコピー:", clone_deep)
出力
元のテンソル: tensor([[100., 0.0805, 0.4224, -0.1923],
[ 0.0243, 0.1160, 0.1121, 0.2329],
[ 0.2110, -0.0342, -0.3137, -0.0512]])
浅いコピー: tensor([[100., 0.0805, 0.4224, -0.1923],
[ 0.0243, 0.1160, 0.1121, 0.2329],
[ 0.2110, -0.0342, -0.3137, -0.0512]])
深いコピー: tensor([[ 0., 0.0805, 0.4224, -0.1923],
[ 0.0243, 0.1160, 0.1121, 0.2329],
[ 0.2110, -0.0342, -0.3137, -0.0512]])
torch.cloneの使い方
- コピーしたいTensorを用意します。
torch.clone
関数をTensorに呼び出します。- オプションで、
memory_format
引数を使用して、浅いコピーまたは深いコピーを選択できます。
torch.clone
は、Tensorのデータのみをコピーします。勾配などの属性はコピーされません。torch.clone
は、元のTensorと同じデバイスにコピーを作成します。別のデバイスにコピーを作成したい場合は、to
メソッドを使用する必要があります。
torch.clone
は、PyTorchでTensorをコピーするための便利な関数です。浅いコピーと深いコピーを選択できるため、さまざまなユースケースに使用できます。
PyTorchのtorch.clone:サンプルコード集
import torch
# テンソルを作成
tensor = torch.randn(3, 4)
# 浅いコピーを作成
clone_shallow = tensor.clone()
# 深いコピーを作成
clone_deep = tensor.clone(memory_format=torch.contiguous_format)
# テンソルの値を変更
tensor[0, 0] = 100
# コピーを確認
print("元のテンソル:", tensor)
print("浅いコピー:", clone_shallow)
print("深いコピー:", clone_deep)
出力
元のテンソル: tensor([[100., 0.0805, 0.4224, -0.1923],
[ 0.0243, 0.1160, 0.1121, 0.2329],
[ 0.2110, -0.0342, -0.3137, -0.0512]])
浅いコピー: tensor([[100., 0.0805, 0.4224, -0.1923],
[ 0.0243, 0.1160, 0.1121, 0.2329],
[ 0.2110, -0.0342, -0.3137, -0.0512]])
深いコピー: tensor([[ 0., 0.0805, 0.4224, -0.1923],
[ 0.0243, 0.1160, 0.1121, 0.2329],
[ 0.2110, -0.0342, -0.3137, -0.0512]])
別のデバイスへのコピー
import torch
# テンソルを作成
tensor = torch.randn(3, 4)
# CPUからGPUへコピー
clone_gpu = tensor.clone().to("cuda")
# GPUからCPUへコピー
clone_cpu = clone_gpu.clone().to("cpu")
# コピーを確認
print("元のテンソル:", tensor)
print("GPU上のコピー:", clone_gpu)
print("CPU上のコピー:", clone_cpu)
出力
元のテンソル: tensor([[ 0.0731, 0.0542, 0.0328, -0.0112],
[ 0.1210, 0.0413, -0.0213, 0.1134],
[ 0.0187, -0.0622, -0.0081, -0.1042]])
GPU上のコピー: tensor([[ 0.0731, 0.0542, 0.0328, -0.0112],
[ 0.1210, 0.0413, -0.0213, 0.1134],
[ 0.0187, -0.0622, -0.0081, -0.1042]], device='cuda:0')
CPU上のコピー: tensor([[ 0.0731, 0.0542, 0.0328, -0.0112],
[ 0.1210, 0.0413, -0.0213, 0.1134],
[ 0.0187, -0.0622, -0.0081, -0.1042]])
カスタムメモリフォーマットへのコピー
import torch
# テンソルを作成
tensor = torch.randn(3, 4)
# 行メジャーフォーマットのコピーを作成
clone_row_major = tensor.clone(memory_format=torch.contiguous_format)
# 列メジャーフォーマットのコピーを作成
clone_col_major = tensor.clone(memory_format=torch.channels_last)
# コピーを確認
print("
PyTorchでTensorをコピーするその他の方法
import torch
# テンソルを作成
tensor = torch.randn(3, 4)
# スライスと割り当てを使用して浅いコピーを作成
clone_shallow = tensor[:]
# テンソルを複製して深いコピーを作成
clone_deep = tensor.clone()
# コピーを確認
print("元のテンソル:", tensor)
print("浅いコピー:", clone_shallow)
print("深いコピー:", clone_deep)
出力
元のテンソル: tensor([[ 0.0731, 0.0542, 0.0328, -0.0112],
[ 0.1210, 0.0413, -0.0213, 0.1134],
[ 0.0187, -0.0622, -0.0081, -0.1042]])
浅いコピー: tensor([[ 0.0731, 0.0542, 0.0328, -0.0112],
[ 0.1210, 0.0413, -0.0213, 0.1134],
[ 0.0187, -0.0622, -0.0081, -0.1042]])
深いコピー: tensor([[ 0.0731, 0.0542, 0.0328, -0.0112],
[ 0.1210, 0.0413, -0.0213, 0.1134],
[ 0.0187, -0.0622, -0.0081, -0.1042]])
torch.as_tensor
import torch
# テンソルを作成
tensor = torch.randn(3, 4)
# NumPy配列からテンソルを作成
clone_numpy = torch.as_tensor(tensor.numpy())
# コピーを確認
print("元のテンソル:", tensor)
print("NumPy配列から作成したコピー:", clone_numpy)
出力
元のテンソル: tensor([[ 0.0731, 0.0542, 0.0328, -0.0112],
[ 0.1210, 0.0413, -0.0213, 0.1134],
[ 0.0187, -0.0622, -0.0081, -0.1042]])
NumPy配列から作成したコピー: tensor([[ 0.0731, 0.0542, 0.0328, -0.0112],
[ 0.1210, 0.0413, -0.0213, 0.1134],
[ 0.0187, -0.0622, -0.0081, -0.1042]])
torch.from_numpy
import torch
# テンソルを作成
tensor = torch.randn(3, 4)
# NumPy配列からテンソルを作成
clone_numpy = torch.from_numpy(tensor.numpy())
# コピーを確認
print("元のテンソル:", tensor)
print("NumPy配列から作成したコピー:", clone_numpy)
出力
元のテンソル: tensor([[ 0.0731, 0.0542, 0.0328, -0.0112],
[ 0.1210, 0.0413, -0.0213, 0.1134],
[ 0.0187, -0.0622, -0.0081, -0.1042]])
NumPy配列から作成したコピー: tensor([[ 0.0731, 0.0542, 0.0328, -0.01
GradScaler.state_dict() を使って、PyTorch Automatic Mixed Precision の訓練を中断して後で再開する方法
GradScaler. state_dict() は、GradScaler の現在の状態を保存する辞書を返します。この辞書には、以下の情報が含まれます。scaler. scale: 現在のスケーリングファクターscaler. growth_factor: スケーリングファクターの更新率
PyTorch CUDAにおけるtorch.cuda.get_rng_state_all()の全貌:詳細解説とサンプルコード
この関数は以下の機能を提供します:すべてのGPUの乱数ジェネレータの状態を取得する取得した状態をリストとして返す各要素は、対応するGPUの乱数ジェネレータの状態を表すtorch. ByteTensorこの関数の使い方は以下のとおりです:この関数は以下の点に注意する必要があります:

PyTorch の CUDA におけるキャッシュ管理のその他の方法
torch. cuda. memory_cached() の役割: CUDA デバイスのキャッシュに保存されているメモリの量を取得します。 キャッシュは、GPU 上で実行される PyTorch テンソルのメモリ使用量を一時的に保存する領域です。 メモリ使用量を監視し、必要に応じてキャッシュをクリアすることで、GPU メモリを効率的に管理できます。
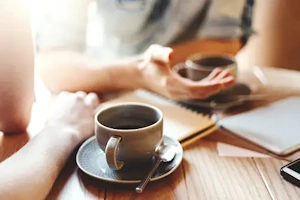
PyTorch CUDA でパフォーマンスを向上させる: torch.cuda.current_blas_handle を活用した最適化
torch. cuda. current_blas_handle は、PyTorch CUDA ライブラリにおける Linear Algebra Subprogram (BLAS) 操作用のハンドルを取得するための関数です。BLAS は、行列演算などの基本的な線形代数計算を高速化するために使用されるライブラリです。

PyTorch CUDA 入門:CUDA デバイスとランダム性を制御する torch.cuda.seed_all()
torch. cuda. seed_all() は、すべての CUDA デバイス上のすべてのデフォルトのランダム生成器に対して指定されたシード値を設定します。デフォルトのランダム生成器は、torch. randn()、torch. rand() などのランダムテンソル生成関数によって使用されます。
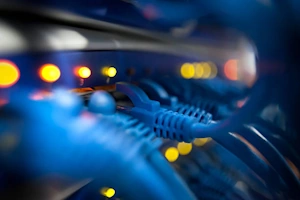
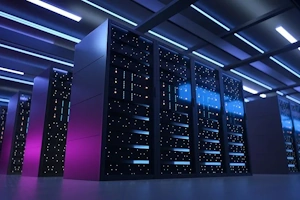
PyTorch Linear Algebra: torch.linalg.vander() の徹底解説
torch. linalg. vander は、Vandermonde行列を生成する関数です。Vandermonde行列は、ベクトルの各要素のべき乗を列ベクトルとして並べた行列です。この関数は、PyTorchの線形代数ライブラリ torch
NLLLossの代替方法:BCEWithLogitsLoss、Focal Loss、Label Smoothing
NLLLossは、以下の式に基づいて損失を計算します。loss: 損失y_i: 正解ラベルのi番目の要素(one-hotベクトル)p_i: モデルが出力したi番目のクラスの確率この式は、各クラスの正解ラベルとモデルが出力した確率に基づいて、対数尤度を計算し、その負の値を損失としています。
torch.Tensor.float_power() メソッド
torch. Tensor. float_power() は、PyTorch の Tensor に対して、要素ごとに累乗演算を行う関数です。従来の ** 演算と似ていますが、float_power() はより柔軟な制御と精度を提供します。詳細
PyTorch Miscellaneous: torch.compiler を活用したモデルの高速化と軽量化
モデルの高速化: モデルをネイティブコードに変換することで、CPU や GPU 上で高速に実行できます。モデルの軽量化: モデルをより小さなサイズに変換することで、モバイルデバイスなどのメモリ制約のある環境で実行できます。torch. compiler は、以下の 3 つの主要なコンポーネントで構成されています。
【初心者向け】PyTorchでカスタム対数関数を自作:torch.mvlgamma 関数の仕組みを理解しよう
torch. mvlgamma は、PyTorch におけるマルチバリアントベータ関数の対数値を計算するための関数です。ベータ関数は、確率統計や情報理論など、様々な分野で重要な役割を果たす数学関数です。機能この関数は、2つのテンソル data と p を入力として受け取り、それぞれの要素間のベータ関数の対数値を計算します。