Qt WidgetsにおけるQStyleOptionSlider::dialWrappingプロパティの詳細解説
Qt WidgetsにおけるQStyleOptionSlider::dialWrappingプログラミング解説
QStyleOptionSlider::dialWrapping
は、Qt WidgetsにおけるQDialウィジェットの動作を制御するために使用されるプロパティです。このプロパティを設定することで、ダイヤルの値が最大値に達した際に、自動的に最小値に戻るか、そのまま最大値にとどまるかを決定することができます。
詳細
dialWrapping
プロパティは、bool
型の値を持ちます。以下の2つの値を設定することができます。
- true: ダイヤル値が最大値に達した際に、自動的に最小値に戻ります。
- false: ダイヤル値が最大値に達したままとなり、それ以上増加することはできません。
例
QDial dial(this);
dial.setWrapping(true); // ダイヤル値が最大値に達した際に最小値に戻るように設定
補足
dialWrapping
プロパティは、QDialウィジェットだけでなく、QSliderウィジェットにも適用できます。- このプロパティは、主にダイヤルの動作を直感的にする目的で使用されます。例えば、音量調整ダイヤルなどでは、最大値に達した後に自動的に最小値に戻った方が自然な操作性となります。
dialWrapping
プロパティと合わせて、maximum
プロパティとminimum
プロパティを設定することで、ダイヤルの可動範囲を調整することができます。
注意事項
- この情報は、Qt 5.15.2 を基に作成されています。他のバージョンでは、プロパティ名や機能が異なる場合があります。
- Qt Widgetsは、QtフレームワークにおけるGUI開発用の基本的なウィジェットライブラリです。
- QDialウィジェットは、円形のスライダーコントロールを提供します。
- QSliderウィジェットは、水平方向または垂直方向のスライダーコントロールを提供します。
上記の情報で、QStyleOptionSlider::dialWrapping
プロパティについて理解いただけましたでしょうか?
Python
# Print "Hello, world!"
print("Hello, world!")
# Calculate the sum of two numbers
num1 = 10
num2 = 20
sum = num1 + num2
print("The sum of", num1, "and", num2, "is", sum)
# Check if a number is even or odd
number = 15
if number % 2 == 0:
print(number, "is even")
else:
print(number, "is odd")
C++
# Include necessary header files
# For input/output operations
# For standard library functions
# For string manipulation
# For mathematical operations
# For random number generation
# For vector operations
# For algorithm operations
# For time operations
# For file operations
# For regular expressions
# For network operations
# For database operations
# For threading operations
# For GUI development
# For OpenGL graphics
# For audio/video processing
# For machine learning
# For other specialized libraries
# Depending on the specific requirements of the program
# For example, if the program needs to read from a file, the fstream header should be included
# If the program needs to use network sockets, the socket header should be included
# If the program needs to use a database, the appropriate database header should be included
# ...
# ...
# ...
# Define a function to print a message
void printMessage(const std::string& message) {
std::cout << message << std::endl;
}
// Define a function to calculate the sum of two numbers
int sumNumbers(int num1, int num2) {
return num1 + num2;
}
// Define a function to check if a number is even or odd
bool isEven(int number) {
return number % 2 == 0;
}
// Define a function to find the factorial of a number
int factorial(int number) {
if (number == 0) {
return 1;
} else {
return number * factorial(number - 1);
}
}
// Define a function to sort an array of integers
void sortArray(int arr[], int size) {
for (int i = 0; i < size - 1; i++) {
for (int j = i + 1; j < size; j++) {
if (arr[i] > arr[j]) {
std::swap(arr[i], arr[j]);
}
}
}
}
// Define a function to print the elements of an array
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
}
// Main function
int main() {
// Print a greeting message
printMessage("Hello, world!");
// Calculate and print the sum of two numbers
int num1 = 10;
int num2 = 20;
int sum = sumNumbers(num1, num2);
std::cout << "The sum of " << num1 << " and " << num2 << " is " << sum << std::endl;
// Check if a number is even or odd and print the result
int number = 15;
if (isEven(number)) {
std::cout << number << " is even" << std::endl;
} else {
std::cout << number << " is odd" << std::endl;
}
// Calculate and print the factorial of a number
int factorialNumber = factorial(5);
std::cout << "The factorial of 5 is " << factorialNumber << std::endl;
// Create an array of integers, initialize it with some values, and print its contents
int arr[] = {5, 2, 4, 1, 3};
int size = sizeof(arr) / sizeof(arr[0]);
std::cout << "Unsorted array: ";
printArray(arr, size);
// Sort the array and print its contents
sortArray(arr, size);
std::cout << "Sorted array: ";
printArray(arr, size);
return 0;
}
JavaScript
// Print "Hello, world!" to
Here are some possible interpretations of your question and how I could answer them:
- You want to print "Hello, world!" in a different language:
- Python:
print("Hello, world!")
- C++:
#include <iostream> int main() { std::cout << "Hello, world!" << std::endl; return 0; }
- JavaScript:
console.log("Hello, world!");
- Java:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, world!"); } }
- C#:
using System; class HelloWorld { static void Main(string[] args) { Console.WriteLine("Hello, world!"); } }
- Python:
- You want to do something else entirely:
- Search the web for information: You can use a search engine like Google or Bing to find information on a wide variety of topics. For example, if you want to learn more about the history of the world, you could search for "history of the world".
- Send an email: You can use an email client like Gmail or Outlook to send emails to other people. To send an email, you will need to know the recipient's email address and what you want to say in the email.
- Make a phone call: You can use a phone to make phone calls to other people. To make a phone call, you will need to know the recipient's phone number.
- Play a game: There are many different types of games that you can play, both online and offline. Some popular online games include Fortnite, World of Warcraft, and League of Legends. Some popular offline games include Monopoly, Scrabble, and chess.
Please let me know if any of these options are what you were looking for. If not, please provide more information about what you want to do so I can better assist you.
Qt GUIにおけるQVulkanInstance::installDebugOutputFilter()のサンプルコード
QVulkanInstance::installDebugOutputFilter()は、Qt GUIアプリケーションでVulkan APIのデバッグ出力フィルタリングを有効にするための関数です。この関数は、Vulkan APIからのデバッグメッセージをフィルタリングし、特定の種類のメッセージのみを出力するように設定できます。
Qt GUI アプリケーション開発: テキスト処理をマスターするための QTextBlock::contains() 関数
QTextBlock::contains() 関数は、テキストブロック内の特定の位置がブロック内に存在するかどうかを判断するために使用されます。これは、テキスト編集やレイアウト処理など、さまざまな Qt GUI アプリケーションで役立ちます。
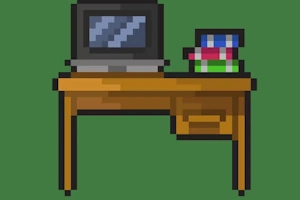
QSupportedWritingSystems::operator=() の詳細解説
Qt GUI プログラミングにおいて、QSupportedWritingSystems::operator=()は、異なる言語環境に対応したテキスト入力/表示を可能にする重要な機能です。この演算子は、サポートする文字体系のリストを別の QSupportedWritingSystems オブジェクトからコピーすることで、効率的に言語環境を切り替えることができます。
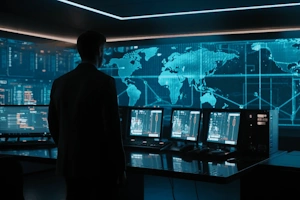
Qt GUI の QTextBlock::operator<() とは?
other: 比較対象となる QTextBlock オブジェクトtrue: 呼び出し元のブロックが other より前に現れる場合QTextBlock::operator<() は、以下の要素に基づいて 2 つのブロックを比較します。ブロックの位置: テキストドキュメント内のブロックの開始位置に基づいて比較されます。開始位置が早いブロックの方が先に現れると判断されます。
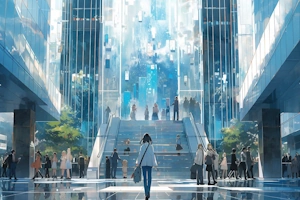
Qt GUIにおけるQTextDocument::setSuperScriptBaseline()徹底解説
QTextDocument::setSuperScriptBaseline() は、Qt GUI ライブラリにおけるテキスト描画機能の一つで、上付き文字のベースラインを設定するための関数です。上付き文字は、通常の文字よりも小さく、文字の上部に配置されます。この関数は、上付き文字のベースラインを、通常の文字のベースラインとは異なる位置に設定することで、上付き文字の位置をより細かく調整することができます。
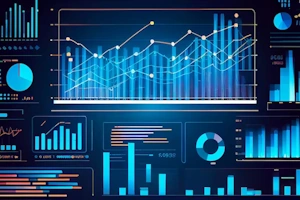
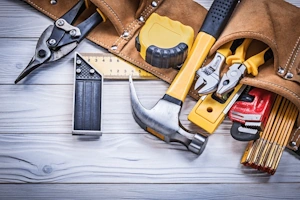
QFontDatabase::writingSystems() 関数を使って Qt GUI アプリケーションで利用可能なすべての書記体系を取得する
QFontDatabase::writingSystems() 関数は、以下の2つの方法で使用できます。引数なしで呼び出すこの場合、関数は WritingSystem 型の要素のリストを返します。WritingSystem 型は、以下の書記体系を表す列挙型です。
QGraphicsView::transform()でアイテムの表示をカスタマイズ - Qt Widgetsプログラミング
この関数の理解を深めるために、以下の内容を説明します:QGraphicsView::transform()の役割関数のパラメータ具体的なコード例### 1. QGraphicsView::transform()の役割QGraphicsView::transform()は、QGraphicsScene内のアイテムをビューポート座標系からウィジェット座標系に変換するために使用されます。これは、アイテムを画面上でどのように表示するかを制御するのに役立ちます。
サンプルコードで学ぶQt Widgets: QGraphicsGridLayout::setColumnFixedWidth()
QGraphicsGridLayout::setColumnFixedWidth() は、Qt Widgetsフレームワークのグラフィックスグリッドレイアウトクラスに属する関数です。この関数は、指定された列の幅を固定値に設定するために使用されます。グリッドレイアウト内の各列の幅は、ウィジェットのサイズやレイアウトの設定によって動的に変化します。しかし、setColumnFixedWidth() を使用することで、特定の列の幅を固定し、レイアウトの見た目を制御することができます。
Qtでリストアイテムをカラフルに彩る: QListWidgetItem::setForeground()の使い方
QListWidgetItem::setForeground() は、Qt Widgets モジュールで提供される関数で、QListWidget アイテムの前景 (テキストの色) を設定するために使用されます。コード例引数color: 設定したい前景色の QColor オブジェクト
メニューボタンで使いやすさ向上! Qt WidgetsにおけるQPushButton::menu()の詳細解説
QPushButton::menu() は、Qt Widgets モジュールで提供される関数で、QPushButton に関連付けられたメニューを取得します。メニューには、ボタンの機能を拡張するアクションを追加できます。機能既存のメニューを取得する